Apache Struts Testing
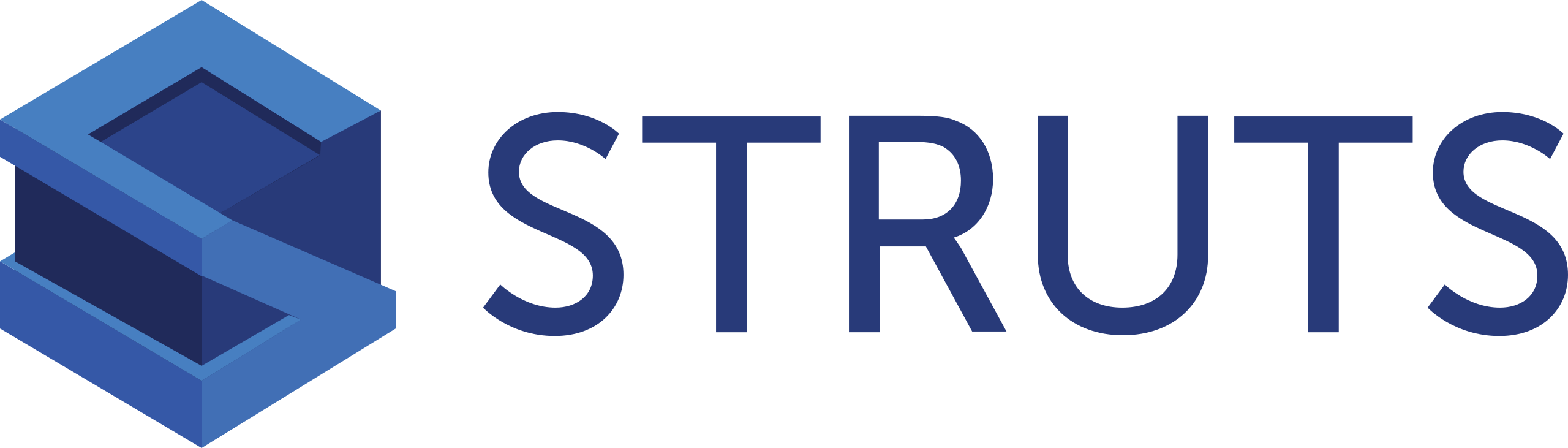
The Apache Struts web framework is a free, open-source MVC (model-view-controller) solution for creating dynamic web applications using Java. It is a well-maintained and full-featured web framework that provides a set of libraries, components, and tools that help developers create web applications with ease. The Apache Struts Project offered two major versions of the Struts framework. Currently, only the Struts 2 version is being maintained.
The framework includes three key components: A "request" handler provided by the application developer that is mapped to a standard URI. A "response" handler transfers control to another resource, which completes the response. A tag library helps developers create interactive form-based applications with server pages. Struts works well with conventional REST applications and technologies like SOAP and AJAX.
Unit Testing
Unit tests are meant to check all the units that are present in the application. Testing each component at a granular level is important as it acts as the very first layer of verification. How these components behave once operated together is checked with the other testing types discussed below. You will need to mock data inputs and services to ensure that each unit can be tested in isolation.
Struts 2 supports running unit tests in the Struts Action class with the Struts 2 JUnit plugin. The Struts 2 JUnit plugin jar file must be on your application's classpath. The JUnit plugin allows you to test the methods of an Action class from within the Struts 2 framework. To use the Struts 2 plugin to ensure the Strut 2 framework runs as part of the test. Also, you need to have your JUnit test class extend StrutsTestCase.
If your Struts 2 application uses Spring to inject dependencies into the Action class, then the Struts 2 JUnit Plugin has a StrutsSpringTestCase that your test class should extend.
@Test public void testExecuteValidationPasses() throws Exception { request.setParameter("personBean.firstName", "Bruce"); request.setParameter("personBean.lastName", "Phillips"); request.setParameter("personBean.email", "[email protected]"); request.setParameter("personBean.age", "19"); ActionProxy actionProxy = getActionProxy("/register.action"); Register action = (Register) actionProxy.getAction() ; assertNotNull("The action is null but should not be.", action); String result = actionProxy.execute(); assertEquals("The execute method did not return " + ActionSupport.SUCCESS + " but should have.", ActionSupport.SUCCESS, result); }
import org.testng.annotations.Test; import com.opensymphony.xwork2.ActionProxy; import org.apache.struts2.StrutsTestCase; import org.junit.Assert; public class ExampleStruts2Test extends StrutsTestCase { @Test public void testLoginAction() throws Exception { request.setParameter("username", "testuser"); request.setParameter("password", "testpassword"); ActionProxy proxy = getActionProxy("/login.action"); String result = proxy.execute(); Assert.assertEquals("success", result); } @Test public void testLogoutAction() throws Exception { request.setParameter("username", "testuser"); ActionProxy proxy = getActionProxy("/logout.action"); String result = proxy.execute(); Assert.assertEquals("success", result); } }
Integration Testing
Using integration tests, you can verify if different components of your system are working correctly together. The test cases target scenarios that involve integrations with databases, file systems, network infrastructure, or other components.
- Many Struts 2 applications interact with a database to store and retrieve data. Integration testing can be used to ensure that the application is interacting correctly with the database, and that data is being stored and retrieved as expected.
- Struts 2 provides built-in form validation features that allow developers to define rules for validating user input. Integration testing can be used to ensure that these validation rules are working correctly and that users aren't able to submit invalid data.
public void validate(){ if (personBean.getFirstName().length() == 0) { addFieldError("personBean.firstName", "First name is required."); } if (personBean.getEmail().length() == 0) { addFieldError("personBean.email", "Email is required."); } if (personBean.getAge() < 18) { addFieldError("personBean.age", "Age is required and must be 18 or older"); } }
- Many Struts 2 applications interact with external APIs to retrieve data or perform actions. Integration testing can be used to ensure that these API integrations are working correctly and that the application is able to handle different types of responses and errors from the API.
- These applications often involve many steps or pages that need to be navigated through in a specific order. Integration testing can also be used here as a pre-step to end-to-end testing for such situations.
By performing this kind of integration testing, we can ensure that our Struts 2 application is working correctly from end to end and that different parts of the application are interacting correctly.
import com.opensymphony.xwork2.ActionProxy; import org.apache.struts2.StrutsTestCase; import org.junit.Test; import static org.junit.Assert.*; public class ExampleIntegrationTest extends StrutsTestCase { @Test public void testSearch() throws Exception { request.setParameter("query", "test"); ActionProxy proxy = getActionProxy("/search.action"); String result = proxy.execute(); assertEquals("success", result); Object action = proxy.getAction(); assertTrue(action instanceof SearchAction); SearchAction searchAction = (SearchAction) action; assertNotNull(searchAction.getResults()); assertTrue(searchAction.getResults().size() > 0); assertEquals("test", searchAction.getQuery()); } }
End-to-End Testing
End-to-end (or system) testing is a methodology that validates the entire application or system, from start to finish, to ensure that it works as expected in real-world scenarios. This testing approach checks the application's flow, from the user interface (UI) down to the back-end systems and databases, simulating user actions, data inputs, and system interactions to verify that all components work together coherently and efficiently.
And although unit testing and integration testing play a vital role in ensuring defect-free software, they are not enough on their own. This is where end-to-end testing comes into play. These tests are mostly on the UI level, but can also involve API calls when being a part of the user flow.
Selenium-based tools
Selenium is an open-source software testing framework primarily used for web applications. It provides a way to automate browser actions and interactions, enabling testers to simulate user behavior and validate that a web application functions as expected. Selenium supports various programming languages, such as Java, C#, Python, and Ruby, and allows for the creation of test scripts that can be executed across multiple web browsers, including Chrome, Firefox, Safari, and Edge. The complexity of test creation and tedious test maintenance are among the top downsides that you ought to consider when deciding to adopt this framework.
Cucumber
Cucumber is an open-source, behavior-driven development (BDD) testing tool that promotes collaboration between business stakeholders, developers, and testers. It enables teams to write human-readable, executable test specifications using a domain-specific language called Gherkin. Cucumber supports various programming languages, including Java, Ruby, and Python, and integrates with popular testing frameworks like Selenium, JUnit, and TestNG. There's a detailed article on Cucumber that you might be interested in.
testRigor
testRigor is a cloud-hosted AI-driven solution, which incrementally simplifies the entire process of end-to-end testing. From initial setup, to building robust functional scenarios using only plain English commands, to extremely straightforward test maintenance.
This means that you don't need programming skills to create testRigor tests, and the tool also supports BDD out of the box with fewer steps to follow than other tools.
What's more is that this tool comes with many additional features like email and SMS text testing, accessibility testing, easy table content testing, and API testing - to name a few. testRigor also supports visual testing wherein you can directly compare screens and brand their variances based on severity. Besides these capabilities, testRigor also integrates with other tools for test management, CI/CD, and issue management tools. You can read more about the capabilities of this tool over here.
run sql query "select top 1 UserID, LastName, FirstName from Users;" open url "https://www.pivotalaccessibility.com/contact-us" enter stored value "FirstName" into "First Name" check that stored value "LastName" itself contains "Jack Smith" run sql query "insert into Users(UserID, FirstName, LastName) values (3, 'Jane', 'Doe');"
login //pre-defined rule click in the bottom right corner of the screen click "washing machine" click exactly "Technician" check page contains "information will be updated once tracking is started" check page contains "start tracking" click "start tracking" check page contains "the technician will arrive between 4:00 pm and 4:30 pm" check that page contains "share the OTP with the technician to start services"
How to do End-to-end Testing with testRigor
Let us take the example of an e-commerce website that sells plants and other gardening needs. We will create end-to-end test cases in testRigor using plain English test steps.
Step 1: Log in to your testRigor app with your credentials.
Step 2: Set up the test suite for the website testing by providing the information below:
- Test Suite Name: Provide a relevant and self-explanatory name.
- Type of testing: Select from the following options: Desktop Web Testing, Mobile Web Testing, Native and Hybrid Mobile, based on your test requirements.
- URL to run test on: Provide the application URL that you want to test.
- Testing credentials for your web/mobile app to test functionality which requires user to login: You can provide the app’s user login credentials here and need not write them separately in the test steps then. The login functionality will be taken care of automatically using the keyword
login
. - OS and Browser: Choose the OS Browser combination on which you want to run the test cases.
- Number of test cases to generate using AI: If you wish, you can choose to generate test cases based on the App Description text, which works on generative AI.
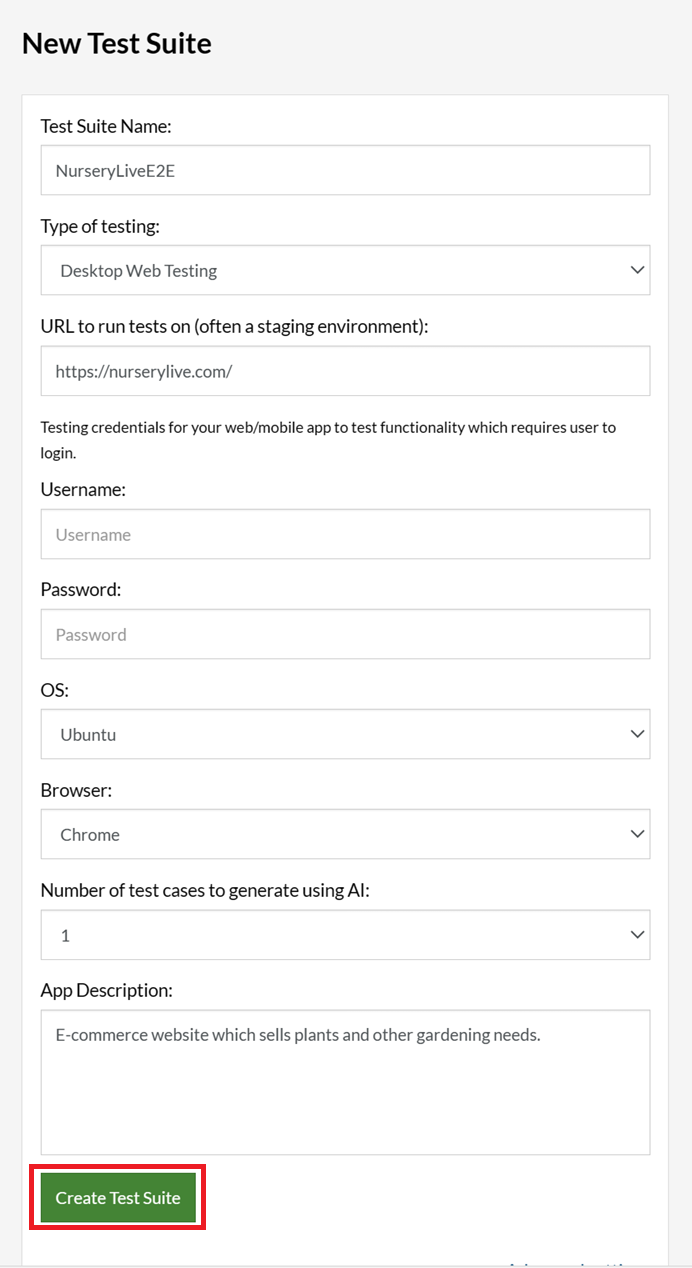
Step 3: Click Create Test Suite.
On the next screen, you can let AI generate the test case based on the App Description you provided during the Test Suite creation. However, for now, select do not generate any test, since we will write the test steps ourselves.
Step 4: To create a new custom test case yourself, click Add Custom Test Case.
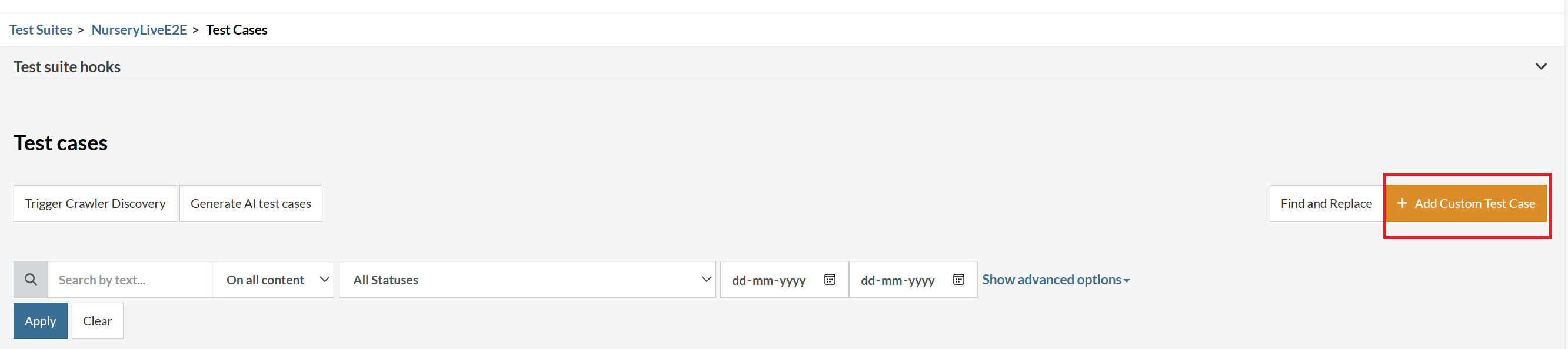
Step 5: Provide the test case Description and start adding the test steps.
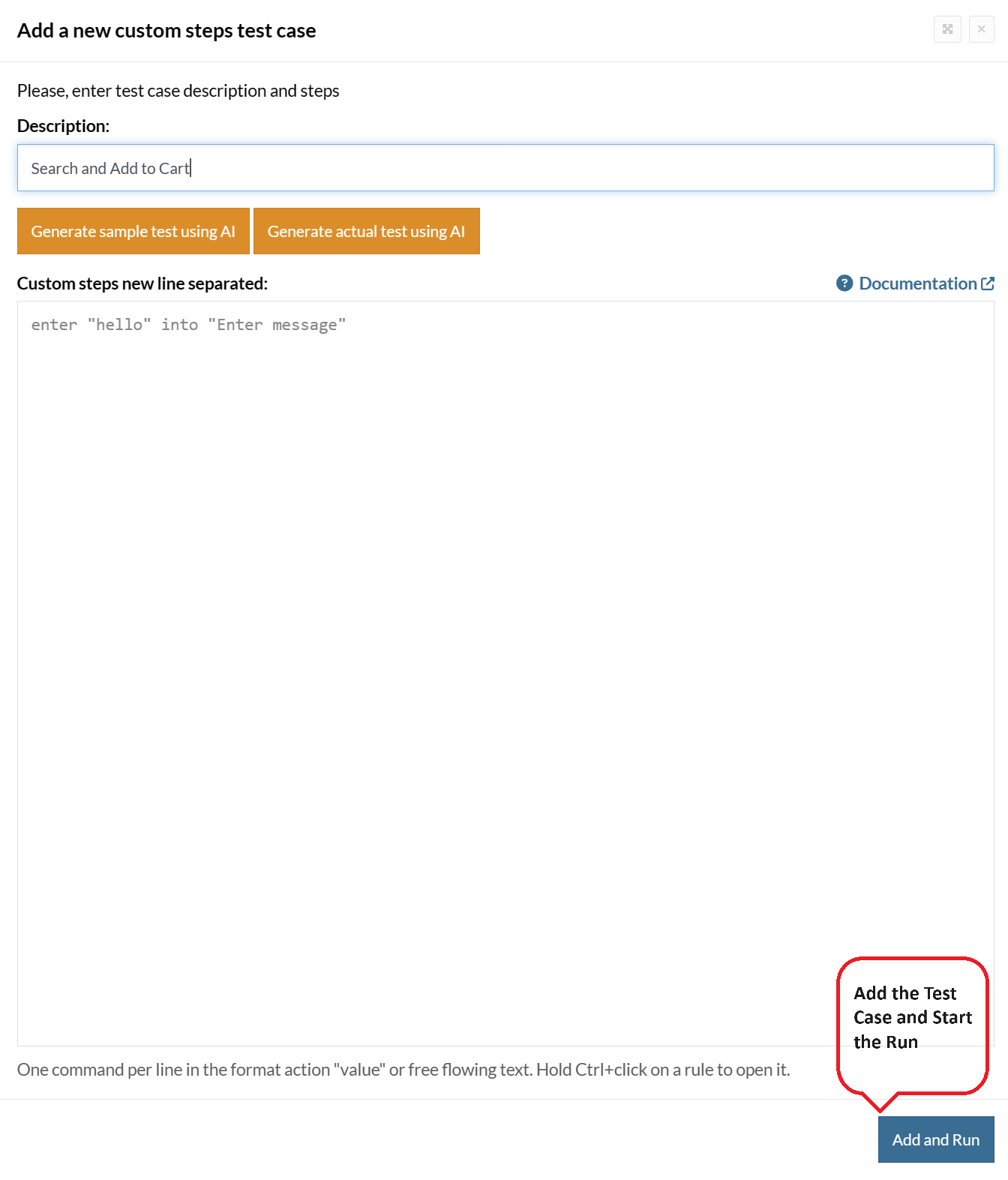
For the application under test, i.e., e-commerce website, we will perform below test steps:
- Search for a product
- Add it to the cart
- Verify that the product is present in the cart
Test Case: Search and Add to Cart
Step 1: We will add test steps on the test case editor screen one by one.
testRigor automatically navigates to the website URL you provided during the Test Suite creation. There is no need to use any separate function for it. Here is the website homepage, which we intend to test.
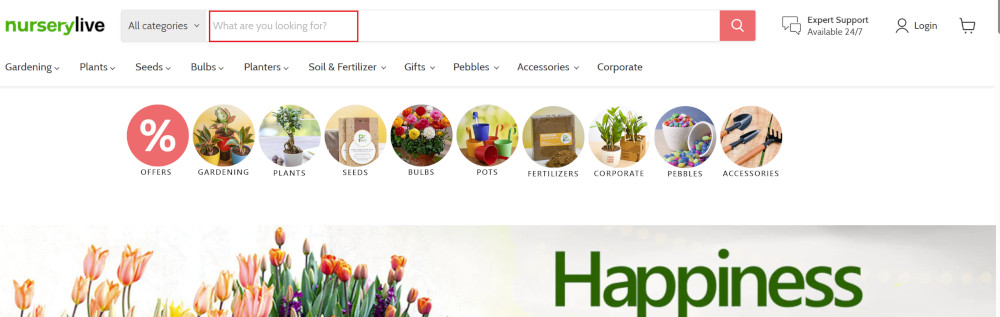
First, we want to search for a product in the search box. Unlike traditional testing tools, you can identify the UI element using the text you see on the screen. You need not use any CSS/XPath identifiers.
click "What are you looking for?"
Step 2: Once the cursor is in the search box, we will type the product name (lily), and press enter to start the search.
type "lily" enter enter
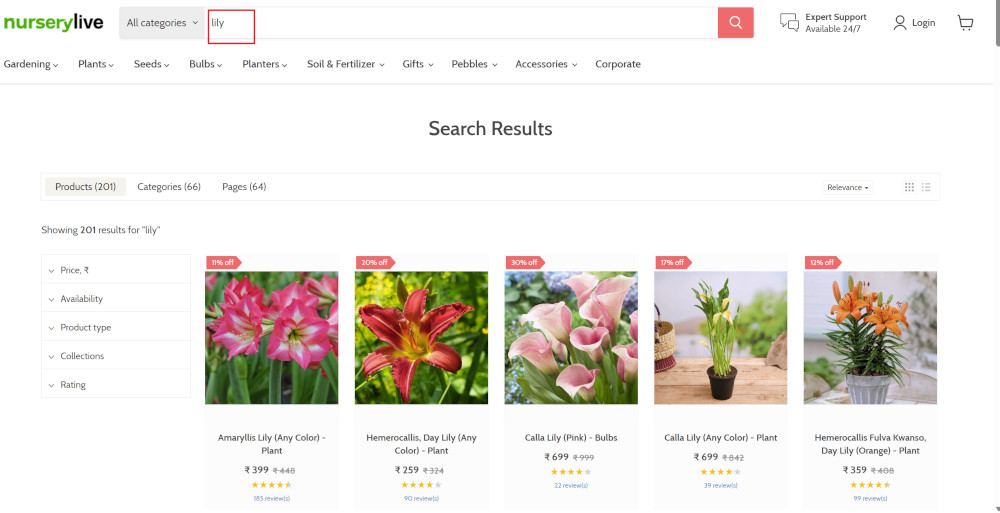
Search lists all products with the “lily” keyword on the webpage.
Step 3: The lily plant we are searching for needs the screen to be scrolled; for that testRigor provides a command. Scroll down until the product is present on the screen:
scroll down until page contains "Zephyranthes Lily, Rain Lily (Red)"
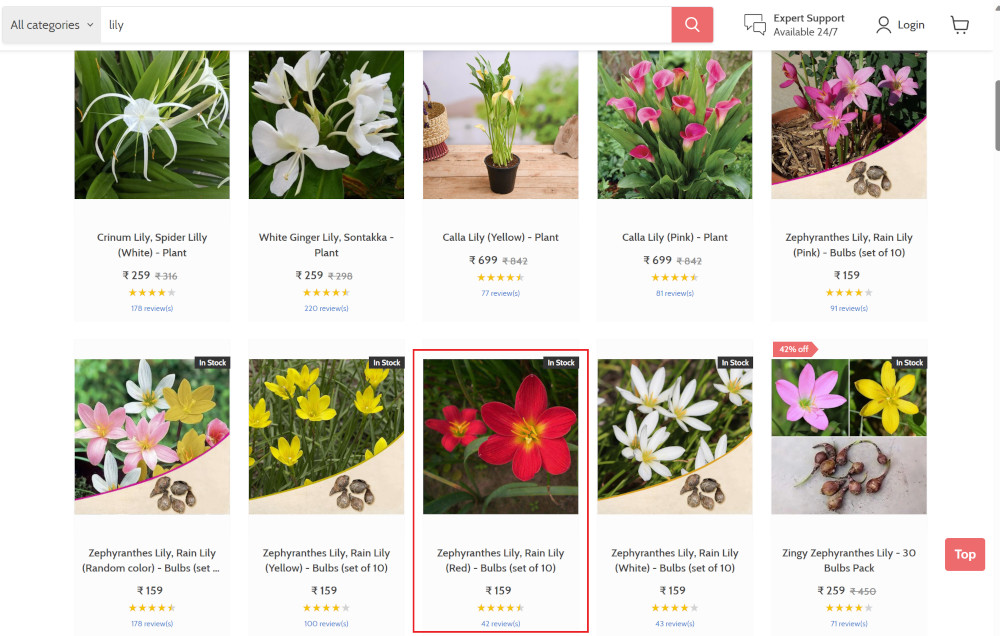
When the product is found on the screen, testRigor stops scrolling.
Step 4: Click on the product name to view the details:
click "Zephyranthes Lily, Rain Lily (Red)"
After the click, the product details are displayed on the screen as below, with the default Quantity as 1.
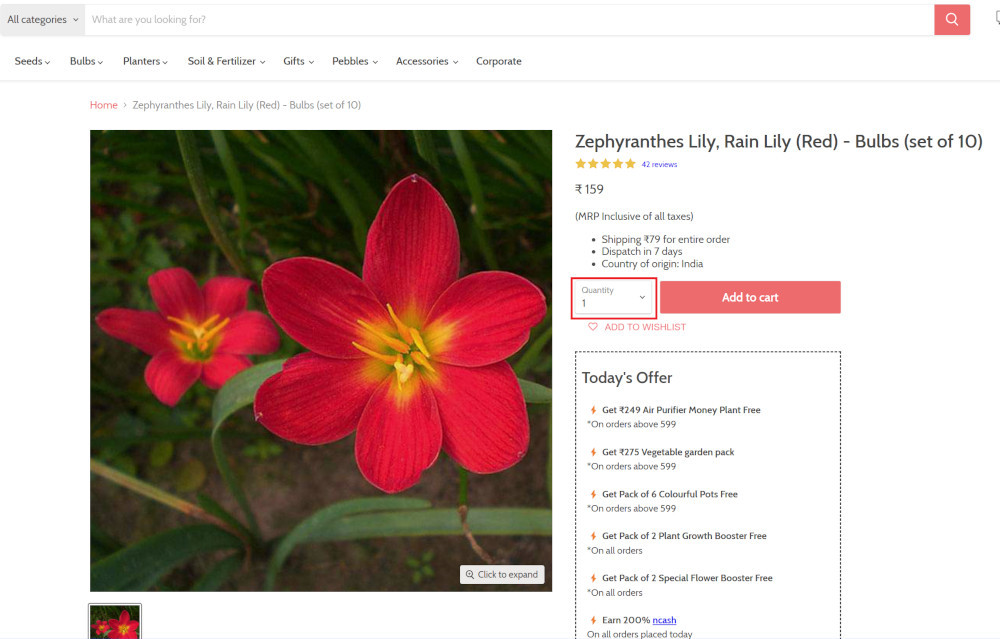
Step 5: Lets say, we want to change the Quantity to 3, so here we use the testRigor command to select from a list.
select "3" from "Quantity"
click "Add to cart"
The product is successfully added to the cart, and the “Added to your cart:” message is displayed on webpage.
Step 6: To assert that the message is successfully displayed, use a simple assertion command as below:
check that page contains "Added to your cart:"
Step 7: After this check, we will view the contents of the cart by clicking View cart as below:
click "View cart"
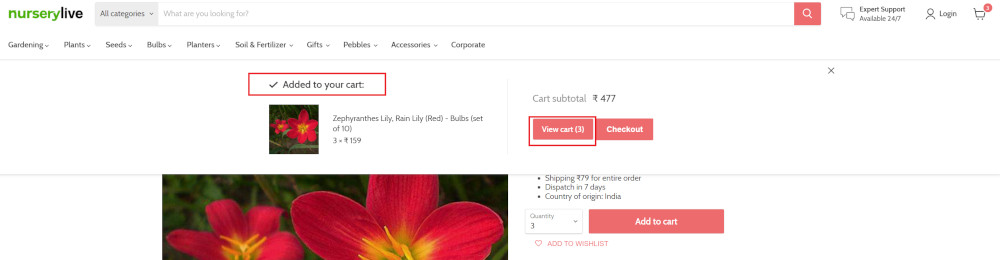
Step 8: Now we will again check that the product is present in the cart, under heading “Your cart” using the below assertion. With testRigor, it is really easy to specify the location of an element on the screen.
check that page contains "Zephyranthes Lily, Rain Lily (Red)" under "Your cart"
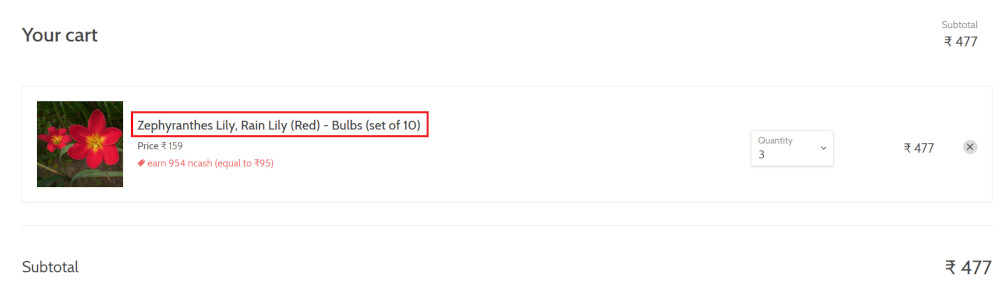
Complete Test Case
Here is how the complete test case will look in the testRigor app. The test steps are simple in plain English, enabling everyone in your team to write and execute them.
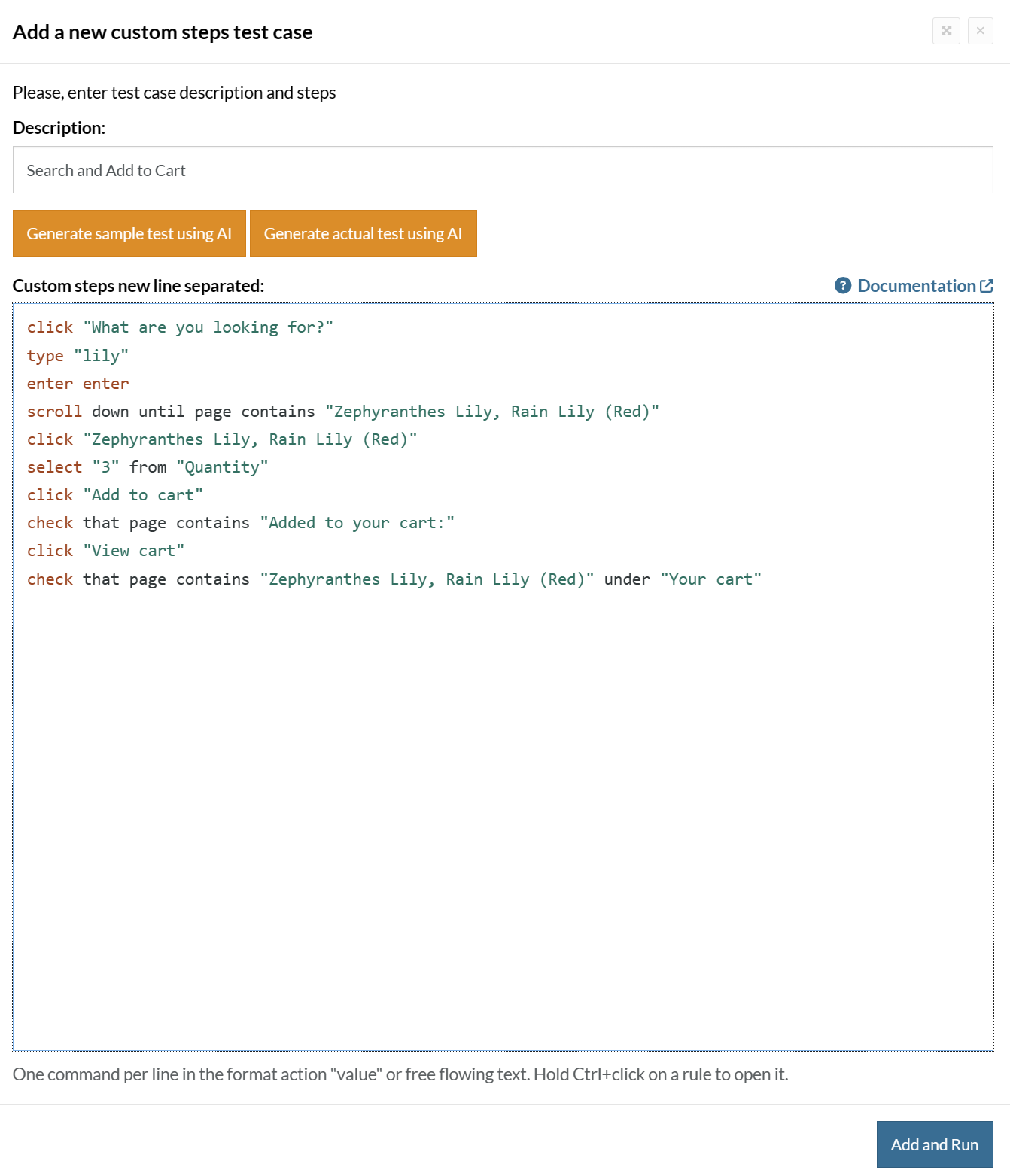
Click Add and Run.
Execution Results
Once the test is executed, you can view the execution details, such as execution status, time spent in execution, screenshots, error messages, logs, video recordings of the test execution, etc. In case of any failure, there are logs and error text that are available easily in a few clicks.
You can also download the complete execution with steps and screenshots in PDF or Word format through the View Execution option.
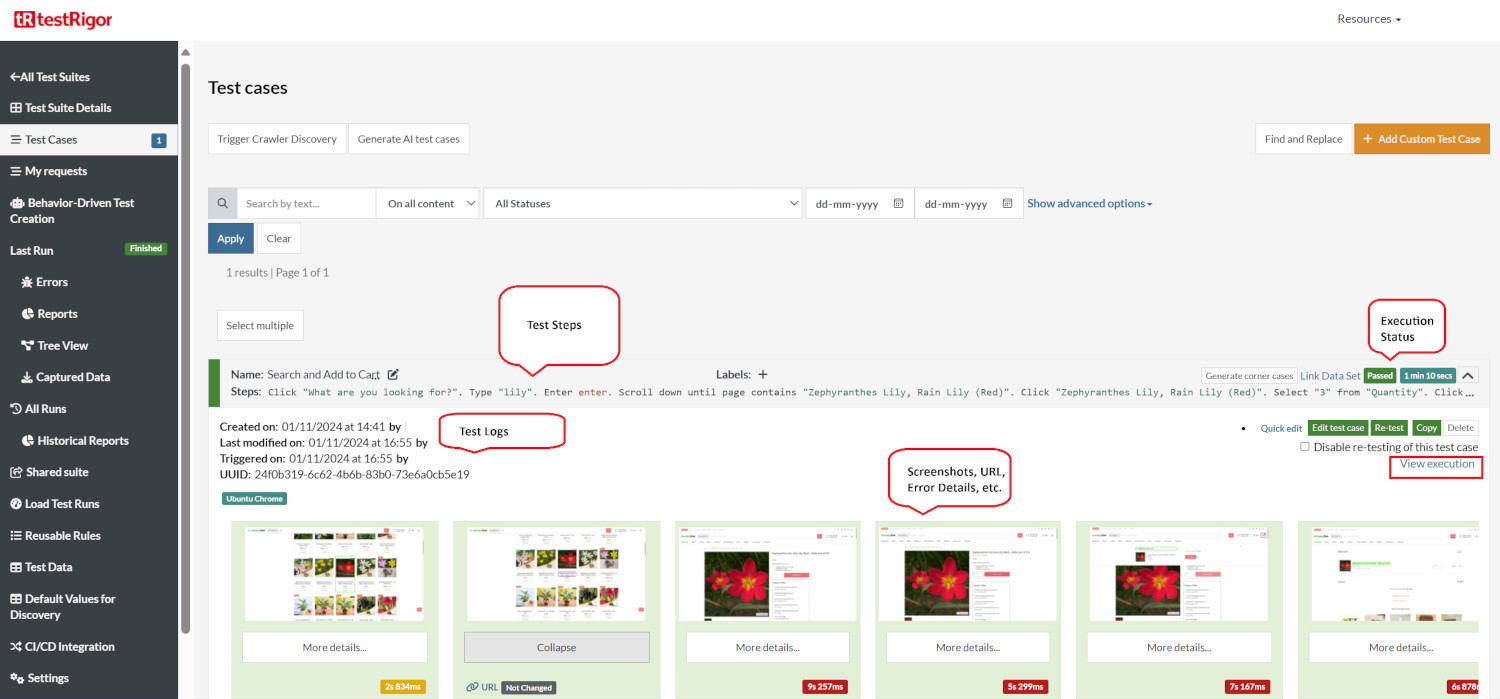
testRigor’s Capabilities
Apart from the simplistic test case design and execution, there are some advanced features that help you test your application using simple English commands.
- Reusable Rules (Subroutines): You can easily create functions for the test steps that you use repeatedly. You can use the Reusable Rules to create such functions and call them in test cases by simply writing their names. See the example of Reusable Rules.
- Global Variables and Data Sets: You can import data from external files or create your own global variables and data sets in testRigor to use them in data-driven testing.
- 2FA, QR Code, and Captcha Resolution: testRigor easily manages the 2FA, QR Code, and Captcha resolution through its simple English commands.
- Email, Phone Call, and SMS Testing: Use simple English commands to test the email, phone calls, and SMS. These commands are useful for validating 2FA scenarios, with OTPs and authentication codes being sent to email, phone calls, or via phone text.
- File Upload/ Download Testing: Execute the test steps involving file download or file upload without the requirement of any third-party software. You can also validate the contents of the files using testRigor’s simple English commands.
- Database Testing: Execute database queries and validate the results fetched.
testRigor enables you to test web, mobile (hybrid, native), API, and desktop apps with minimum effort and maintenance.
Additional Resources
- Access testRigor documentation to know about more useful capabilities
- Top testRigor’s features
- How to perform end-to-end testing
Conclusion
Java developers can create versatile web applications using Apache Struts. With proper quality assurance practices and the latest testing tools, you can also ensure the application is in great shape and ready for end users.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
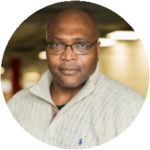