|
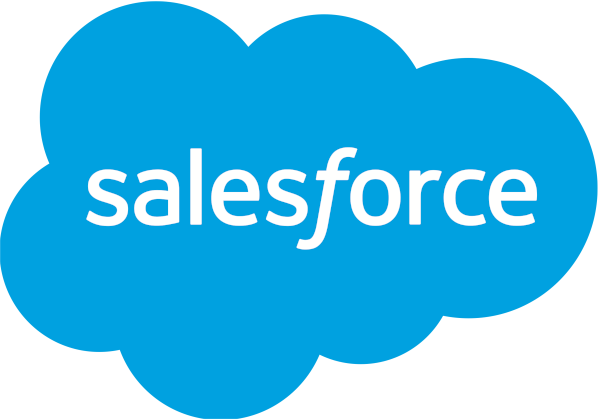
Salesforce is a cloud-based customer relationship management (CRM) platform designed to help businesses manage customer interactions and relationships. It provides a suite of tools for managing sales, marketing, customer service, and analytics, all accessible through a web browser or mobile app.
Salesforce is a highly customizable platform that can be tailored to the specific needs of a business. The platform also offers a marketplace of third-party apps and integrations that can extend its functionality and integrate with other business systems. Salesforce is known for its user-friendly interface and its focus on user adoption, which has helped to make it one of the most popular CRM platforms in the world. It is used by businesses of all sizes, from small startups to large enterprises and across various industries.
This article will help you test Salesforce implementation in your organization. If you are a developer creating or using those Salesforce AppExchange apps, you can use this article to understand how to test them effortlessly.
Amongst the many products and services that Salesforce offers, here are a few examples of Salesforce products that make development easier:
- Lightning Platform: Salesforce Lightning is a UI framework and design system allowing developers and administrators to create modern, responsive, customizable user interfaces for Salesforce applications. In addition to the UI framework and design system, Lightning also includes a range of features and tools for building custom business logic and workflows, such as Lightning Data Service, which provides a standard way to access and manipulate data in Salesforce, and Lightning Flow, which allows developers to create complex, multi-step processes and workflows.
- Salesforce Mobile SDK: It provides development tools and libraries for building mobile applications that integrate with Salesforce. The Mobile SDK supports native and hybrid mobile app development and provides features such as offline data access, push notifications, and secure authentication.
- Heroku: It is a cloud-based application platform that allows developers to build, deploy, and manage applications in various languages, including Java, Ruby, Node.js, and Python. Heroku provides multiple tools and services for building and deploying applications, including a command-line interface, a web-based dashboard, and an add-on marketplace.
- MuleSoft: It is an integration platform that provides various tools and services for connecting applications and data sources. MuleSoft provides an Anypoint Platform for building and deploying integrations and a development platform for creating custom connectors and APIs.
- Tableau: A data visualization and business intelligence platform that helps businesses analyze and visualize their data. Tableau provides various tools and services for creating custom visualizations, building dashboards, and integrating with other data sources.
- Einstein: It is a set of AI-powered features and tools that can be integrated with Salesforce products, including Sales Cloud, Service Cloud, and Marketing Cloud. As a developer, you can use Einstein to build custom AI models and integrations to help automate and optimize various business processes.
- Salesforce DX: Salesforce provides tools and features that make it easier for developers to build and manage applications on the Salesforce platform. It includes scratch orgs, version control integration, and continuous integration and delivery (CI/CD) pipelines. Salesforce DX is designed to improve the development process and enable developers to work more efficiently.
Salesforce testing can be performed manually or through automated testing tools. Automated testing tools can speed up the testing process, improve accuracy, and reduce the risk of human error. When it comes to backend development and testing, Apex is a strongly typed, object-oriented language that is syntactically similar to Java.
For the front end, Salesforce has Lightning frameworks. When it comes to testing, following the testing pyramid is a good idea to ensure maximum coverage and quality. Here is a video to help you create ultra-stable Salesforce test cases.
This means that the following types of testing ought to be done:
Unit Testing
It is meant to test whether each code unit, like classes, methods, or components, works as expected. The focus is strictly on the output of the code here. These kinds of tests are run in isolation and need to be lightweight. This means that your tests should be such that they operate only on a single unit of code, and if there are mandatory dependencies, then they are mocked.
You must write unit tests for the backend and frontend code, depending on your project. Below are the most commonly used tools to do this.
Backend unit testing
Backend work for Salesforce applications is done using Apex, which is a proprietary language. Thus, Salesforce offers certain tools to test this code as well.
Using Apex test execution
Salesforce uses Apex as a programming language to build and customize functionality. It is designed specifically for the Salesforce platform and can create custom business logic, automate processes, integrate with external systems, and create custom user interfaces.
Developers can use Apex to write triggers, which execute custom code before or after data manipulation events such as inserts, updates, or deletions. Apex can also be used to create Visualforce pages, which are custom user interfaces that interact with data on the Salesforce platform. Additionally, Apex can be used to build custom integrations with external systems and to create complex workflows and approval processes.
In Salesforce, the @isTest annotation marks a class/method as a test class/ test method. Test classes and methods are used for unit testing, which is the process of testing individual pieces of code to ensure that they work correctly and meet the specified requirements. You can execute your tests using the following:
- Apex Test Execution page: This feature in the Salesforce UI allows developers to run Apex tests asynchronously and view the test results. Developers can use filters to view test results by class, method, outcome, and more.
- Developer Console: The Developer Console is a tool within Salesforce that provides a range of features for developers, including the ability to create and run tests, execute anonymous Apex code, and debug code.
- Using the API: You can run tests synchronously using the runTests() call. Based on what you specify in the RunTestsRequest object, this single call allows you to execute tests in a specific namespace, tests in a specific subset of classes in a specific namespace, or tests in all classes. You can also choose to run your tests synchronously or asynchronously.
- ApexTestQueueItem: You can use the ApexTestQueueItem to run your tests asynchronously. The tests get added to a job queue, and the results become available once the execution is done. Since this process uses the Apex scheduler, you can schedule your tests to run at specific times.
Here is an example of a unit test written in Apex language for testing the functionality of the “MileageTracker” application. It consists of two test methods: runPositiveTestCases() and runNegativeTestCases().
runPositiveTestCases() method tests the positive scenarios of the application. It creates test data for two users, inserts test mileage records, and verifies if the total mileage is calculated correctly. It also tests the bulk insertion of mileage records and ensures that the daily limit of 500 miles is not exceeded.
@isTest private class MileageTrackerTestSuite { @TestSetup static void setup() { List<User> users = new List<User> { new User(Alias = 'auser', Username = '[email protected]', Email = '[email protected]', EmailEncodingKey = 'UTF-8', LastName = 'Testing', LanguageLocaleKey = 'en_US', LocaleSidKey = 'en_US', ProfileId = [SELECT Id FROM Profile WHERE Name='Standard User' LIMIT 1].Id, TimeZoneSidKey = 'America/Los_Angeles'), }; insert users; } static testMethod void runPositiveTestCases() { Double totalMiles = 0; final Double maxTotalMiles = 500; final Double singleTotalMiles = 300; final Double u2Miles = 100; User u1 = [SELECT Id FROM User WHERE Alias='auser' LIMIT 1]; System.RunAs(u1) { Mileage__c testMiles1 = new Mileage__c(Miles__c = singleTotalMiles, Date__c = System.today()); insert testMiles1; totalMiles = getMilesForUser(u1.Id); Assert.areEqual(singleTotalMiles, totalMiles); List<Mileage__c> testMiles2 = new List<Mileage__c>(); for(integer i=0; i<200; i++) { testMiles2.add( new Mileage__c(Miles__c = 1, Date__c = System.today()) ); } insert testMiles2; totalMiles = getMilesForUser(u1.Id); Assert.areEqual(maxTotalMiles, totalMiles); } } static testMethod void runNegativeTestCases() { User u3 = [SELECT Id FROM User WHERE Alias='tuser' LIMIT 1]; System.RunAs(u3) { Mileage__c testMiles3 = new Mileage__c(Miles__c = 501, Date__c = System.today()); try { insert testMiles3; Assert.fail('DmlException expected'); } catch (DmlException e) { Assert.areEqual('FIELD_CUSTOM_VALIDATION_EXCEPTION', e.getDmlStatusCode(0)); Assert.areEqual(Mileage__c.Miles__c, e.getDmlFields(0)[0]); Assert.isTrue(e.getMessage().contains('Mileage request exceeds daily limit(500): [Miles__c]'), 'DMLException did not contain expected validation message:' + e.getMessage()); } } } private static Double getMilesForUser(Id userId) { Double totalMiles = 0; for(Mileage__c m:[SELECT miles__c FROM Mileage__c WHERE CreatedDate = TODAY AND CreatedById = :userId AND miles__c != null]) { totalMiles += m.miles__c; } return totalMiles; } }
Frontend Unit Testing
Javascript is typically used when it comes to frontend unit testing. Instead of reinventing the wheel, Javascript testing tools like Mocha, Jest, Chai, and Jasmine are available with wrappers. Let’s take a look at these testing alternatives.
Lightning Testing Service (LTS)
Lightning Testing Service (LTS) is a Salesforce tool that bridges JavaScript testing tools and the Salesforce platform. It provides a way to test the Lightning components and applications developed using the Salesforce Lightning web components framework.
LTS runs the tests in a headless browser environment and supports testing frameworks such as Jest, Mocha, and Jasmine. It enables developers to write unit, integration, and end-to-end tests for their Lightning components and applications.
Jasmine
Jasmine is a behavior-driven development (BDD) framework for testing JavaScript code. These tests are organized into “specs,” which define what is being tested and include one or more “expectations” that describe what the code under test should do. Jasmine provides several matchers that can be used in expectations to check that values are what they should be, such as toEqual, toBe, toContain, toBeGreaterThan, and many others.
It also provides several utility functions for setting up tests, such as beforeEach, afterEach, beforeAll, and afterAll, which allow you to define setup and teardown code that will run before or after each spec or suite of specs. This can be useful for initializing test data, cleaning up after tests, or setting up dependencies shared across multiple specs.
describe("Lightning Component Testing Examples", function(){ afterEach(function() { $T.clearRenderedTestComponents(); }); describe('c:egRenderElement', function(){ it('renders specific static text', function(done) { $T.createComponent("c:egRenderElement", {}, true) .then(function(component) { expect(document.getElementById("content").textContent).toContain("Hello World!"); done(); }).catch(function(e) { done.fail(e); }); }); });
Jest
Salesforce supports Jest as the preferred testing framework for Lightning Web Components (LWC). Jest is a popular JavaScript testing framework allowing developers to write unit and integration tests for LWCs.
Below is a Jest test file for a Salesforce Lightning web component called “c-hello”. The file is named “hello.test.js” and is written in JavaScript. The import statement imports two Lightning Web Components framework functions: createElement and Hello. createElement is used to create the c-hello component, while Hello references the c-hello component’s JavaScript class.
The “describe” function is used to group related test cases together. In this case, all tests will be related to the “c-hello” component. The afterEach function is called after each test case in the “c-hello” group has run. It removes all child elements from the document. body element to ensure that the DOM is clean before each test case. The “it” function defines a single test case. In this case, the test verifies that the component displays the greeting “Hello, World!”.
// hello.test.js import { createElement } from 'lwc'; import Hello from 'c/hello'; describe('c-hello', () => { afterEach(() => { // The jsdom instance is shared across test cases in a single file so reset the DOM while (document.body.firstChild) { document.body.removeChild(document.body.firstChild); } }); it('displays greeting', () => { // Create element const element = createElement('c-hello', { is: Hello }); document.body.appendChild(element); // Verify displayed greeting const div = element.shadowRoot.querySelector('div'); expect(div.textContent).toBe('Hello, World!'); }); });
Mocha and Chai
Mocha is a JavaScript testing framework that provides a flexible and straightforward way to write tests. It allows you to write tests using a variety of testing styles, including BDD (Behavior-Driven Development), TDD (Test-Driven Development), and traditional functional testing. Mocha provides a simple and flexible API for defining and running tests, with support for asynchronous testing and hooks for handling pre- and post-test actions.
Chai, on the other hand, is an assertion library for JavaScript that can be used with any testing framework, like Mocha. It provides a range of built-in assertions for testing common things like equality, type, and presence, as well as support for custom assertions. Chai’s flexible API also allows for various testing styles, including BDD-style assertions using natural language constructs like “should” and “expect”.
Mocha and Chai provide a powerful and flexible testing solution for JavaScript applications. Mocha provides the framework for organizing and running tests, while Chai provides the syntax for writing assertions within those tests.
Integration Testing
Integration tests form the next layer of control after unit testing. They are fewer than unit tests since they aim to verify if module integrations are working properly. The goal of integration testing is to verify that the different components of the system work together as expected and that data is accurately passed between the different components. This could be integrations between different components, classes, controllers, network systems, and databases. Integration testing can be used to test the integration between different Salesforce components, such as custom code, workflows, triggers, and external systems.
Integration testing should be done in Salesforce when you build an application that integrates with other systems or external services. You can use the same tools as above for backend and frontend test writing and execution. Here are some examples of scenarios where integration testing is typically needed:
- Integrating with third-party APIs: If you are building an application that integrates with third-party APIs, such as payment gateways, email providers, or social media platforms, you need to perform integration testing to ensure that data is being exchanged correctly and that the application is behaving as expected.
- Custom integrations: If you build custom integrations between Salesforce and other systems, such as an ERP or a marketing automation platform, you must perform integration testing to ensure that data is being exchanged correctly and the integration is working as expected.
- Upgrading Salesforce: If you upgrade your Salesforce instance to a new version or add new features, you must perform integration testing to ensure that the changes don’t impact any existing integrations.
- Changes to integrations: If you are making changes to any of the existing integrations, such as adding new fields or updating workflows, you need to perform integration testing to ensure that the changes don’t cause any issues.
- Data migration: If you are migrating data from another system to Salesforce, you need to perform integration testing to ensure that the data is being migrated correctly and that the new data is compatible with the existing data in Salesforce.
We can perform integration testing at the API and UI levels. Let us explore more details about it.
API Integration Testing Tools
- Postman: While not a Salesforce-specific tool, Postman is a popular API testing tool that can test integrations with external APIs. It offers a user-friendly interface for building and sending API requests, making it suitable for developers and non-technical users.
- SoapUI: This commercial tool provides a robust suite for API testing and web services management. It supports various protocols, including SOAP, REST, and GraphQL, and offers security and load-testing features.
UI Integration Testing Tools
- Apex Test: While primarily for unit testing, Apex Test can be used for basic integration testing by simulating calls to external systems. However, it requires significant manual setup and coding, limiting scope and efficiency.
- Selenium: We will discuss Selenium in the next section.
End-to-End Testing
In E2E testing, we test the application’s complete user flow, from user login to the end. Many tools are available for performing E2E testing, so let’s review a few.
Selenium
Selenium has played a significant role in automation testing, offering flexibility and control. However, as technology and user expectations evolve, testing needs have shifted. While Selenium remains a valuable tool, its limitations in certain areas have led testers to explore alternatives.
Several limiting factors of Selenium contribute to the search for new approaches:
- Time-consuming code maintenance: Selenium requires writing and maintaining code, which can be intensive for large test suites.
- Unreliable XPath locators: Brittle locators can lead to flaky test results, impacting trust in automation.
- Limited scope: Selenium primarily focuses on web UI, not encompassing the broader application ecosystem.
testRigor
As we mentioned about the disadvantages of using traditional tools, testRigor is in the process of replacing all these tools. testRigor is a codeless automation tool powered by generative AI. Many codeless automation tools are available in the market, but testRigor stands out from all of them because of its incredible features.
Let’s dive in to learn more about testRigor’s features.
- Cloud-hosted: It is a cloud-hosted tool, so you do not need to invest money and resources to set up an environment and maintain the software. Just subscribe and start creating automation scripts right away.
- Eliminates Code Dependency: testRigor helps create test scripts in parsed plain English, eliminating the need to know programming languages. So, any stakeholder, such as manual QA, BAs, sales personnel, etc., can create test scripts and execute them faster than an average automation engineer using legacy test automation tools. Also, since it works on natural language test steps, anyone can create or update test cases, increasing the test case coverage manifolds.
-
One Tool Solves Everything: testRigor performs not just web automation; it can help you run the following test types seamlessly:
- Web and Mobile browser testing
- Mobile app testing
- Desktop app testing
- API testing
- Visual testing
- Accessibility testing
So you don’t have to install different tools for different types of testing; testRigor takes care of all your testing needs singlehandedly.
- Stable Element Locators: Unlike traditional tools that rely on specific element identifiers, testRigor uses a unique approach for element locators. You simply describe elements by the text you see on the screen, leveraging the power of AI to find them automatically. This means your tests adapt to changes in the application’s UI, eliminating the need to update fragile selectors constantly. This helps the team focus more on creating new use cases than fixing the flaky XPaths/CSS locators.
- Integrations: testRigor offers built-in integrations with most of the popular CI/CD tools like Jenkins and CircleCI, test management systems like Zephyr and TestRail, defect tracking solutions like Jira and Pivotal Tracker, infrastructure providers like AWS and Azure, and communication tools like Slack and Microsoft Teams.
upload file from saved value "Profile_photo" to mobile device AppLogin // pre-defined rule to perform login press "Profile" press "Get Started" if present check that page contains "Years of Experience" click "close" in the context of "complete your profile" if present click "Camera" click "Photo albums" click middle of screen check that page contains "Years of Experience" click "Done" at the bottom click on image from stored value "Profile_photo" with less than "10" % discrepancy click "Add Bio" enter stored value "Bio" into "Enter your bio here" click "Save" check that "Bio is too long!" color is "ff0000"
Learn here about helpful features of testRigor.
Salesforce Beyond CRM
Salesforce’s usage and application are far ahead of being just a CRM. Here are some Salesforce areas that function beyond CRM:
Salesforce Cloud: Salesforce caters to diverse needs with a comprehensive suite of cloud applications:
- Sales Cloud: Streamline sales processes and manage pipelines efficiently.
- Service Cloud: Deliver exceptional customer support and resolve issues effectively.
- Marketing Cloud: Execute targeted digital marketing campaigns and measure results.
- Commerce Cloud: Build and manage seamless online storefronts.
- Salesforce Platform: Develop custom applications to extend CRM capabilities.
- Analytics Cloud: Gain valuable insights from customer data to make informed decisions.
- Community Cloud: Foster vibrant online communities for customers, partners, and employees.
- Integration Cloud: Connect Salesforce with various external systems and data sources.
Expanding Ecosystem: The AppExchange, a marketplace for third-party applications, lets businesses further customize their CRM experience by integrating additional tools and services.
Committed to Social Impact: Salesforce embodies its values through its 1-1-1 model, dedicating 1% of its products, equity, and employee time to social good. This commitment to philanthropy has earned the company recognition for its positive impact on society.
About Salesforce AppExchange
Salesforce AppExchange is an app store designed specifically for the Salesforce platform. It’s a marketplace where businesses can find and install various pre-built solutions to extend and customize their Salesforce experience. Think of it like the Google Play Store for Salesforce users. Here’s a breakdown of what you can find on AppExchange:
- Apps: Pre-built applications that address specific business needs, such as marketing automation, project management, reporting, and industry-specific solutions.
- Components: Reusable building blocks for customizing the look and feel of Salesforce pages and applications.
- Flow Solutions: Pre-built automation processes and templates to streamline workflows.
- Bolt Solutions: Industry-specific templates and pre-built communities to accelerate your Salesforce implementation.
- Lightning Data: Real-time data enrichment to enhance your Salesforce data capabilities.
- Consulting services: Connect with certified Salesforce partners to help with implementation, customization, and integration.
How AppExchange Validates Apps
Salesforce’s validation process for apps on the AppExchange is rigorous and thorough, designed to ensure the highest security, functionality, and compatibility standards. The process aims to protect Salesforce customers from security vulnerabilities and to guarantee that the apps integrate seamlessly with Salesforce’s ecosystem. Here’s a detailed look at each process step:
Security Review
It’s designed to identify and mitigate potential security vulnerabilities to protect user data and ensure compliance with best practices. This review includes:
- Static Code Analysis: Salesforce uses automated tools to scan the app’s code for common security issues, such as SQL injection, cross-site scripting (XSS), and insecure data handling.
- Manual Testing: Besides automated tools, security experts perform manual testing to uncover vulnerabilities that automated scans might miss.
- Third-party Libraries and Dependencies Review: Salesforce evaluates the security of any third-party libraries or dependencies used by the app to ensure they do not introduce vulnerabilities.
- OAuth and Data Security: For apps that use OAuth for authentication or accessing Salesforce data, the review includes an assessment of the app’s authentication flows and data handling practices to ensure they are secure.
You can read more about AppExchange security requirements and the AppExchange security review process here.
Technical Review
The technical review assesses the app’s adherence to Salesforce’s technical standards and best practices, including:
- API Usage: Ensuring the app uses Salesforce APIs efficiently and does not exceed API rate limits.
- Performance Impact: Evaluating the app’s impact on the overall performance of the Salesforce environment to ensure it does not degrade user experience.
- Compatibility: Checking the app for compatibility with various Salesforce editions and versions to ensure it works as intended across the platform.
Business Review
The business review evaluates the app’s business model, market viability, and the developer’s support structure. Key aspects include:
- Pricing and Packaging: Review the app’s pricing structure to ensure transparency and fairness.
- Support Model: Evaluating the developer’s plans for customer support, including responsiveness and availability.
- Market Need: Assessing the app’s relevance and utility to ensure it meets a genuine customer need.
Listing and Documentation Review
This step involves reviewing the app’s AppExchange listing and accompanying documentation for clarity, completeness, and accuracy. This includes:
- App Description and Images: Ensuring the app’s listing clearly and accurately describes its features, benefits, and high-quality images.
- Installation and Configuration Guides: Review the documentation to ensure it provides clear instructions for installing and configuring the app.
- Support and Troubleshooting Resources: Ensuring the developer provides adequate resources for users to get help and troubleshoot common issues.
Compatibility Check
Salesforce verifies that the app is compatible with the platform and does not interfere with Salesforce updates or functionality. This includes:
- Testing Across Salesforce Editions: Verifying that the app works across different Salesforce editions (e.g., Essentials, Professional, Enterprise).
- Salesforce Release Compatibility: Ensuring the app is compatible with new releases and updates.
Beta Testing
Selected users test the app in a real-world setting, providing feedback on functionality, usability, and potential issues. Here is more about alpha and beta testing.
Partner Review: Certified Salesforce partners may beta test and provide feedback on the app’s technical aspects and adherence to best practices.
Final Steps
- Salesforce Approves or Requests Changes: Based on all assessments, Salesforce either approves the app or requests changes if any issues are identified. Developers iterate and address feedback until the app meets all requirements.
- Ongoing Monitoring: Even after listing, Salesforce monitors apps for security vulnerabilities, performance degradation, and adherence to guidelines.
- Factors Impacting Validation Time: The validation process can take anywhere from a few weeks to several months, depending on the app’s complexity, completeness of documentation, and identified issues during testing.
Ongoing Compliance
Once an app is listed on the AppExchange, the developer must maintain compliance with Salesforce standards. This includes:
- Regular Updates: Developers must update their apps in response to new Salesforce releases and security patches.
- Periodic Reviews: Salesforce may periodically re-review apps to ensure they continue to meet security and compatibility standards.
Find more details on the validation guide here.
Conclusion
The testing process is vital for building dependable and robust applications on the Salesforce platform. This testing can take several forms, including unit testing, integration testing, and end-to-end testing, each playing a distinct role in guaranteeing the quality of the application. Whether you are developing Salesforce apps or using them in your organization, this article might help you to test the app effectively.
Choosing the right testing tool is critical, and you should consider the specific needs of the testing scenario and the capabilities offered by a specific tool. The testing tool should prove helpful in your testing process and should not elevate the issues with exceptions, coding errors, and instabilities. Make an informed decision and choose the best path to deliver a quality product quickly and efficiently.