API Contract Testing: A Step-by-Step Guide to Automation
|
In modern software development, APIs (Application Programming Interfaces) enable the interactions among applications, services, and systems. It is vital to keep these APIs intact and reliable. This is where the API contract testing comes into play API contract testing ensures that the API works based on a predefined contract, which means keeping everything consistent in all interactions. By automating this process, you are both making the process more efficient and ensuring that validation is thorough and repeatable.
In this article, we will discuss the step-by-step process, best practices, and tools for automating API contract testing.
API contracts establish the structure and behavior of an API and serve as a binding contract between the API provider and the consumer. Such contracts can differ depending on whether they are for the consumer (API consumer) or provider (API provider). It is very important to understand what types of API contracts are helpful in design, development, and testing.
Let us understand the types of API contracts.
Consumer-Driven Contracts
Consumer-driven contracts (CDCs) are based on the needs of the API consumers. The contracts are written according to the way consumers want to consume the API, i.e., the expected endpoints, expected data formats, etc. The provider makes sure that you have complied with these requirements and prevents what could have been integration bugs. This allows us to avoid breaking changes when multiple consumer applications depend on the shared API, extending the API according to the consumer’s needs through CDCs.
Provider Contracts
The API provider defines provider contracts and describes the API’s capabilities. We mention the available endpoints, request/response structures, and error codes. These contracts are comprehensive and cover all possible API use cases. They are then shared with consumers to guide their integration efforts. Provider contracts are particularly effective for public APIs or scenarios where the API serves a large or undefined number of consumers. It is required since they provide a standardized approach to API usage.
What is API Contract Testing?
It is a type of software testing that focuses on the communication between different services or systems. It adheres to a predefined agreement known as a contract. This contract specifies how an API behaves and what it expects when interacting with another system. These are the details present in it:
- Endpoints: The API’s available URLs for interaction (e.g., /users, /orders).
- HTTP Methods: The allowed operations on those endpoints (e.g., GET, POST, PUT, DELETE).
- Request and Response Formats: The structure and data types of the requests sent to and responses received from the API (e.g., JSON, XML).
- Headers: Provide the metadata in API requests or responses (e.g., Authorization, Content-Type).
- Status Codes: The HTTP status codes returned by the API (e.g., 200 OK, 404 Not Found, 500 Internal Server Error).
- Error Responses: Mention how the API handles and communicates errors.
Features of API Contract Testing
- Validation of Agreements: The contract ensures that both the provider (the system exposing the API) and the consumer (the system using the API) agree with the contract on the defined structure and behavior.
- Focus on Interface, Not Implementation: Unlike functional or integration testing, contract testing is not at all concerned with verifying the underlying logic or how the data is handled. Rather, it verifies that the API’s interface confirms its specification.
- Ensures Consistency Across Changes: APIs evolve, and contract testing ensures backward compatibility. It informs teams when a breaking change has occurred.
Why is API Contract Testing Important?
In distributed systems, especially those built on microservices architectures, APIs act as the glue connecting various services. Misalignments in communication can lead to:
- Service failures
- Data mismatches
- Poor user experience
API contract testing addresses these risks by validating the API’s interface early and consistently. It makes sure that services can communicate reliably even as they evolve independently.
Challenges in Manual Contract Testing
Manual contract testing involves verifying that the interaction between APIs adheres to a predefined contract. While it ensures that APIs meet consumer and provider expectations, the manual process often introduces inefficiencies and errors.
Here are the key challenges associated with manual contract testing:
- Time-Consuming: It is a time-intensive effort that involves testing each endpoint, request and response, as well as metadata. In larger systems or in a microservices setup where multiple APIs are wired together, this process becomes even more tedious.
- Error-Prone: Humans can make errors, especially when dealing with complex data structures or larger numbers of test cases. Therefore, it can lead to missed differences. Which we definitely do not want in production environments.
- Inefficient for Iterative Changes: In today’s development cycles, projects are expected to have regular updates and iterations. Every single modification to an API requires the contract to be validated again. Manual checks can slow development cycles and create bottlenecks, particularly during fast-paced releases.
- Lack of Scalability: Manual testing simply cannot scale. More so with the expanding number of APIs and their many integrations. It is impossible to cover all endpoints, error cases, and edge scenarios.
Benefits of Automating API Contract Testing
Automation not only addresses the challenges of manual testing but also offers significant advantages. Here are the key benefits of automating API contract testing:
- Speed: Automated contract tests execute much faster than manual tests. This allows for rapid validation of API changes. This speed is crucial in agile development environments where quick feedback loops are essential.
- Consistency: Automation eliminates human error by applying a uniform testing process across all API endpoints. This ensures that all interactions are validated reliably and consistently, regardless of complexity.
- Scalability: Automated testing can efficiently handle the increasing number of APIs and integrations in modern systems. It allows teams to maintain comprehensive test coverage even as the system grows.
- Early Feedback: Automated tests can be integrated into the development process, enabling contract violations to be identified during development rather than after deployment. This reduces the cost and effort of fixing issues later in the software lifecycle. Read: Minimizing Risks: The Impact of Late Bug Detection.
- Cost-Effective: By minimizing manual efforts and preventing post-release bugs, automation reduces the overall cost of testing. It allows teams to allocate resources to other critical development tasks. Thus, improves overall productivity. Read: What is the Cost of Quality in Software Testing?
Step-by-Step Guide to Automate API Contract Testing
Let’s go through setting up automated API contract testing.
Step 1: Define Your API Contract
The first step is to establish a clear API contract. This serves as the agreed-upon standard between API providers and consumers.
- Use API specification formats like OpenAPI (Swagger), RAML, or GraphQL schemas to document endpoints, request/response structures, headers, and status codes.
- Collaborate with stakeholders (developers, QA, and consumers) to ensure the contract is accurate and comprehensive.
Step 2: Choose the Right Tools
Selecting the appropriate tool is crucial for efficient automation. Consider the following:
- testRigor: Best tool for automating native desktop, web, mobile, API, visual, exploratory, AI features, and accessibility testing in plain English. This helps anyone in the project, even without knowledge of the programming language, to create API tests easily.
- Pact: Ideal for consumer-driven contract testing in microservices.
- Postman: Useful for automating tests based on OpenAPI specifications.
- Swagger/OpenAPI Validator: Ensures API implementations align with OpenAPI contracts.
- Dredd: Tests APIs against contracts written in OpenAPI, API Blueprint, or other formats.
Choose tools that align with your technology stack and team expertise.
Step 3: Set Up Your Testing Environment
Create an environment where automated tests can run reliably:
- Use mock servers to simulate the provider or consumer for isolated testing.
- Configure test environments to mimic production settings to catch potential issues early.
- Ensure necessary dependencies and configurations are included.
Step 4: Write Contract Tests
Begin implementing test cases to validate the API contract.
- Test the structure of requests and responses, ensuring they match the contract.
- Validate required headers, status codes, and error messages.
- Include tests for edge cases and invalid inputs.
- For consumer-driven contracts, verify that providers meet the consumer’s expectations.
Step 5: Integrate with CI/CD Pipelines
Automate the execution of contract tests by integrating them into your CI/CD pipelines.
- Configure your pipeline to run contract tests on each pull request or build.
- Fail builds if any test violates the contract, ensuring issues are caught early.
- Use tools like Jenkins, GitHub Actions, or CircleCI for seamless integration.
Step 6: Monitor and Maintain Tests
As APIs evolve, contracts and tests need to be updated:
- Version Control: Use versioning for API contracts to manage changes and ensure backward compatibility.
- Regular Updates: Modify tests whenever there are changes to the API contract.
- Monitor Test Results: Regularly review test reports to identify patterns or recurring issues.
Common Challenges in API Contract Testing
Automating API contract testing simplifies and enhances the validation process, but it is not without challenges. Teams often encounter issues such as managing API changes, dealing with complex dependencies, and ensuring test reliability. Below are these challenges explained, along with the strategies to overcome them.
Handling API Changes
API changes, whether due to new features or improvements, can break existing contracts and disrupt integrations. To overcome these challenges:
- Use Version Control: Maintain versioned API contracts to track changes and ensure backward compatibility.
- Employ Deprecation Strategies: Gradually phase out outdated APIs while providing consumers time to migrate to newer versions.
Dealing with Complex Dependencies
Dependencies on external systems or APIs can make testing fragile and unreliable. This is especially true when those systems are unavailable or prone to changes. To overcome these challenges:
- Utilize Mocks and Stubs: Simulate external dependencies to isolate tests and focus solely on the API being tested. This ensures tests remain unaffected by external factors.
Ensuring Test Reliability
Flaky tests can undermine the confidence in automation, often caused by unstable environments or inconsistent data. To overcome these challenges:
- Stabilize Test Environments: Ensure that the testing environment mirrors production to reduce variability.
- Use Consistent Data: Employ predictable test data and avoid relying on dynamic or random values that can cause discrepancies. Read: How to do data-driven testing in testRigor.
Future Trends in API Contract Testing Automation
The landscape of API contract testing is continually evolving with advancements in technology and shifts in software development practices. Below are the emerging trends that are shaping the future of API contract testing automation, with a special focus on how tools like testRigor are contributing to these developments.
AI-Driven Contract Validation
The integration of Artificial Intelligence (AI) technology is transforming contract testing by automating the generation and validation process for API contracts. Through usage patterns, historical data, and interactions with intent systems, these AI tools can either propose or validate API definitions.
How testRigor Fits In:
testRigor uses AI to reduce the maintenance overhead of automated tests. By adapting test scripts to minor changes in APIs, testRigor ensures that your contract tests remain relevant and effective without requiring manual intervention. Read: Decrease Test Maintenance Time by 99.5% with testRigor.
Shift-Left Testing
Shift-left testing emphasizes performing contract testing earlier in the development lifecycle. By integrating contract validation during development or even at the design stage, teams can detect and resolve issues before they propagate downstream.
How testRigor Fits In:
testRigor’s ease of use and natural language capabilities make it an excellent choice for integrating contract testing early in the development process. Its ability to create tests without coding enables developers and non-technical stakeholders to participate in testing from the beginning.
Event-Driven APIs
The rise of event-driven architectures and asynchronous communication has led to a growing focus on testing APIs defined by the AsyncAPI specification. These APIs handle events and message streams, requiring unique testing strategies to ensure compliance and reliability.
How testRigor Fits In:
While testRigor excels in RESTful API testing, its flexible framework can be extended to accommodate event-driven APIs. By integrating with tools that simulate events, testRigor can validate the contract for AsyncAPI-based systems. Read: How to do API testing using testRigor?
Here is an All-Inclusive Guide to Test Case Creation in testRigor.
Conclusion
API contract testing automation is essential in modern software projects. It can provide great value to teams with reliability, compatibility, and scalability. It does so by validating how application programming interfaces (APIs) interact with each other and are based on predefined contracts. By following this guide, you will be able to implement a solid automated contract testing workflow, enabling your organization to release better software much faster. Do follow the recommended tools, best practices, and methodologies shared above to make your API testing faster and more reliable!
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
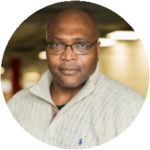