Headless Browser Testing: When and How to Use It?
|
In automation testing, we try to reduce the execution time as much as possible. To achieve that, usually the parallel execution count is increased. But still, the tests are executed in traditional web browsers, which consumes more time to load and execute the scenarios. This is where headless browser testing emerges as a game-changer.
In this article, let’s understand more about headless browsers, their advantages and disadvantages, and more importantly, how they help to increase the test execution speed.
Understanding Headless Browsers
Headless browsers are regular browsers without a graphical user interface(GUI). It functions similar to a standard browser. Headless browser renders webpages, executes JavaScript and handles network requests. The only difference is the browser operates in the background, executing commands and interacting with web pages just like a regular browser but without displaying the user interface.
This browser is very helpful when you are doing test automation, web scraping or running tests in environments where a GUI is not available or practical. One of the major benefits of using a headless browser is we can run them on servers without any GUI support.
Why Use Headless Browser Testing?
Since headless browsers operate without a graphical user interface, there are many advantages. Let’s go through a few common ones.
Speed and Efficiency
Headless browsers don’t need to render the GUI, which helps in improving test execution speed. This is particularly beneficial for running large test suites or conducting frequent regression testing.
Scalability
Headless browsers are lightweight and consume minimal resources. This allows them to run on servers without dedicated displays, allowing for easy scaling of test environments. Also, this won’t consume much memory of the machine like regular browsers, so you can scale up to double the count of normal browsers.
CI/CD Integration
Headless browsers seamlessly integrate with CI/CD pipelines. Tests can be automated to run after every code commit, providing instant feedback on potential issues.
Cross-Browser Compatibility Testing
Headless browsers can be used to simulate different browser versions and configurations. This helps to ensure that your web application functions flawlessly across various user environments.
Performance Testing
By monitoring resource usage during headless testing, you can identify performance bottlenecks and optimize your web application for faster loading times.
Web Scraping and Monitoring
Headless browsers are very useful in extracting data from websites. This can be valuable for tasks like price comparison, competitor analysis, and website monitoring.
When to Use Headless Browser Testing?
Headless browser testing is ideal for various scenarios:
Automated Testing
- Unit Tests and Integration Tests: Helps to verify small units of code or interactions between components without the overhead of a GUI.
- Regression Testing: Ensure that recent changes do not break existing functionality by running tests efficiently.
- End-to-End (E2E) Testing: You can simulate real user scenarios to validate complete workflows without the need for a graphical interface.
Continuous Integration (CI) and Continuous Deployment (CD)
- CI Pipelines: You can integrate headless browser tests to run automatically with each code commit, ensuring quick feedback on code quality.
- CD Pipelines: Verify that the application is functional before deployment to production environments, streamlining the release process.
Performance Testing
- Backend Performance: Focus on server response times and database performance without the distraction of rendering graphics.
- Load Testing: Simulate high user loads to identify performance bottlenecks and ensure the application can handle peak traffic.
Web Scraping and Data Extraction
- Content Extraction: Using headless browsers we can programmatically retrieve data from web pages, including dynamic content loaded via JavaScript.
- Automated Data Collection: With headless browsers, we can automate the process of logging into websites, navigating pages, and collecting data for analysis or integration.
Development and Debugging
- Non-GUI Environments: Test web applications on remote servers or virtual machines that lack a graphical interface.
- Debugging: Reproduce and diagnose issues in a controlled environment to identify and fix bugs efficiently.
How to Implement Headless Browser Testing
We need to follow a few steps to implement headless browsers into our automation environment. So let’s see how we can do this:
- Choose a Headless Browser: There are several headless browsers available that we can use for automation like – Chrome Headless, Firefox Headless, and Puppeteer (a Node.js library for Chrome Headless).
- Select a Testing Framework: You can use any available frameworks like Selenium, Cypress, and Playwright that provide tools and APIs for scripting and automating browser interactions.
- Develop Test Scripts: Write scripts using the selected framework’s language to simulate user actions and verify expected outcomes.
- Integrate with CI/CD Pipeline (Optional): Integrate your tests with your CI/CD pipeline to automate testing after every code push.
- Execute and Analyze Results: Run the tests and analyze the results to identify bugs and regressions.
Tools for Headless Browser Testing
As we discussed, several tools support headless testing in automation discussions. We can discuss how it’s done in each tool and its limitations.
Selenium
from selenium import webdriver from selenium.webdriver.chrome.options import Options options = Options() options.headless = True driver = webdriver.Chrome(options=options) driver.get("https://example.com") print(driver.title) driver.quit()
Selenium’s Limitations
- Complex setup: Initial setup and configuration can be cumbersome.
- Performance: Slower than some other tools, particularly with heavy tests.
- Verbosity: Test scripts can be verbose and harder to maintain.
Puppeteer
const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch({ headless: true }); const page = await browser.newPage(); await page.goto('https://example.com'); const title = await page.title(); console.log(title); await browser.close(); })();
Puppeteer’s Limitations
- Browser limitation: Only supports Chrome/Chromium, lacking cross-browser testing capabilities.
- Language limitation: Only supports JavaScript/Node.js, limiting its use in projects using other languages.
Playwright
const { chromium } = require('playwright'); (async () => { const browser = await chromium.launch({ headless: true }); const page = await browser.newPage(); await page.goto('https://example.com'); const title = await page.title(); console.log(title); await browser.close(); })();
Playwright’s Limitations
- Language limitation: Playwright is primarily designed for JavaScript/Node.js, though bindings for Python and C# are available.
PhantomJS
var page = require('webpage').create(); page.open('https://example.com', function(status) { console.log("Status: " + status); if(status === "success") { console.log(page.title); } phantom.exit(); });
PhantomJS’s Limitations
- Deprecated: This tool is no longer actively maintained, making it less secure and reliable.
- Limited features: Lacks support for modern web features compared to newer tools.
testRigor
If you have noticed, for all the tools mentioned above, you need to write a few lines of code to enable headless execution. Also for all those tools, we need to manually update the browser version or the headless tool we are using. Often all these lead to additional tasks. But with testRigor, it’s very easy to execute tests in headless mode.
Option 1
If you are creating a new test suite, click Advanced settings and then click General dropdown. In that, there will be a checkbox to enable Desktop headless mode. You can enable that if you need to execute in headless mode.
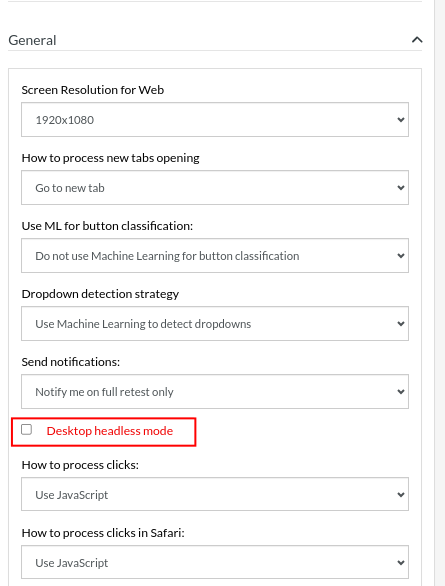
Option 2
If you already have a test suite, and want to run in headless mode, then go to Settings on the left side panel and click Advanced tab, there scroll down to Desktop Web Fine Tuning. There will be a checkbox to enable Desktop headless mode. You can enable that if you need to execute in headless mode.
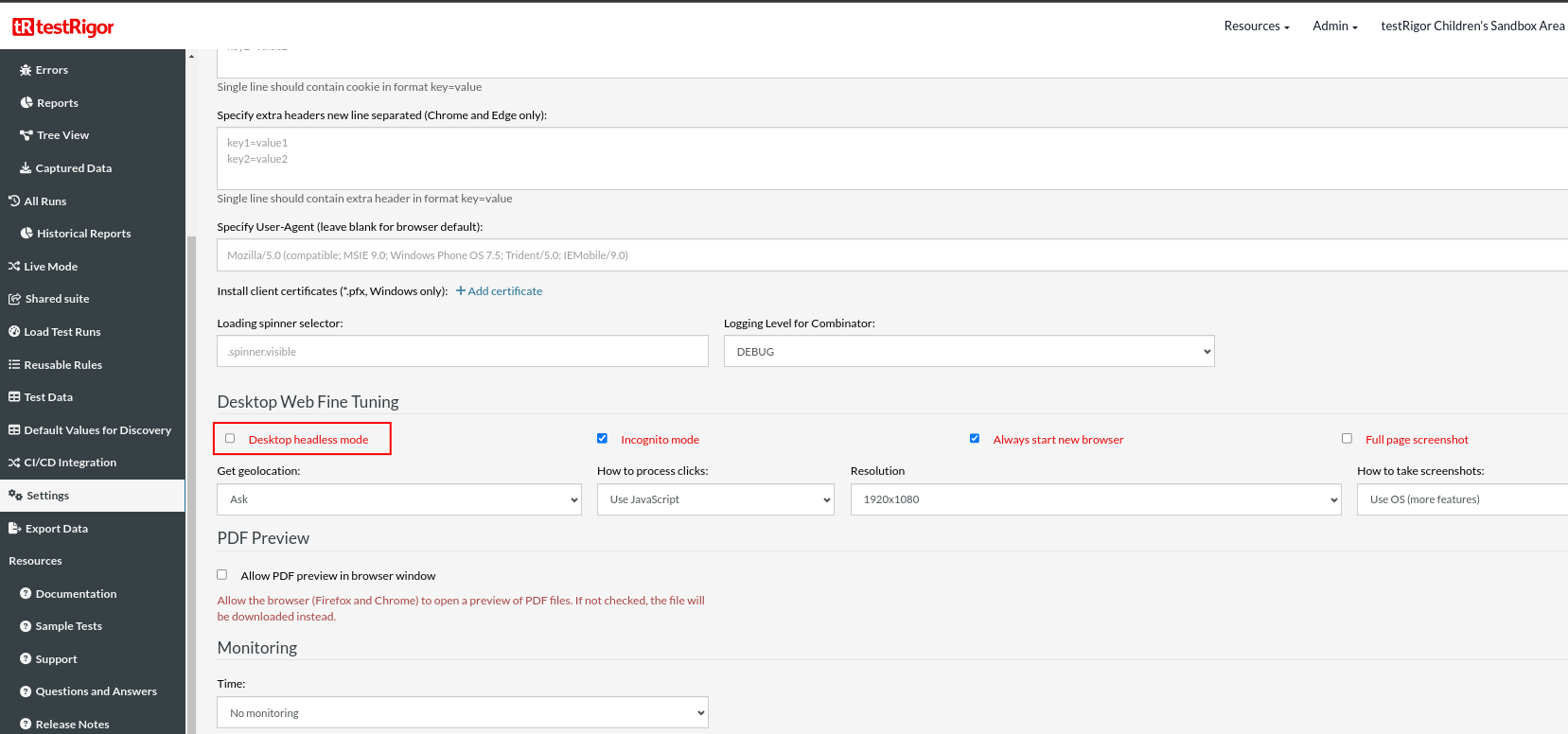
testRigor’s Capabilities
Apart from the simplistic test case design and execution, some advanced features help you test your application using simple English commands.
- Reusable Rules (Subroutines): You can easily create functions for the test steps you use repeatedly. You can use the Reusable Rules to create such functions and call them in test cases by simply writing their names. See the example of Reusable Rules.
- Global Variables and Data Sets: You can import data from external files or create your own global variables and data sets in testRigor for data-driven testing.
- 2FA, QR Code, and Captcha Resolution: testRigor efficiently manages the 2FA, QR Code, and Captcha resolution through simple English commands.
- Email, Phone Call, and SMS Testing: Use simple English commands to test the email, phone calls, and SMS. These commands help validate 2FA scenarios with OTPs and authentication codes sent via email, phone calls, or text.
- File Upload/ Download Testing: Execute the test steps involving file download or file upload without the requirement of any third-party software. You can also validate the contents of the files using testRigor’s simple English commands.
- Database Testing: Execute database queries and validate the results fetched.
testRigor enables you to test web, mobile (hybrid, native), API, and desktop apps with minimum effort and maintenance.
Conclusion
In today’s fast-paced product and service market, the motto is “Quick Release to Market.” To achieve this, every team in an organization needs to work efficiently. Testing often takes a long time because there are many test cases to run across different browsers. Headless browsers can help reduce execution time and increase the number of tests that can run in parallel. Tools like testRigor make it easy to switch to headless browsers without changing any code. This allows QA teams to verify builds quickly, supporting the quick-to-market strategy.
Frequently Asked Questions (FAQs)
Headless browser testing is generally secure, but it’s important to handle sensitive data carefully and ensure that tests do not expose or compromise security credentials or other sensitive information.
Yes, with automation testing tools we can simulate mobile devices by setting viewport sizes and user-agent strings to mimic mobile browsing environments.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
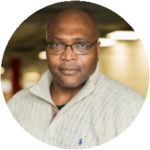