|
Driver Initialization |
|
Chrome | IWebDriver driver = new ChromeDriver(); |
---|---|
Firefox | IWebDriver driver = new FirefoxDriver(); |
Edge | IWebDriver driver = new EdgeDriver(); |
Safari | IWebDriver driver = new SafariDriver(); |
Locating Elements |
|
By ID: | driver.FindElement(By.Id("<element_ID>")); |
By Name: | driver.FindElement(By.Name("<element_name>")); |
By Class Name: | driver.FindElement(By.ClassName("<element_class>")); |
By Tag Name: | driver.FindElement(By.TagName("<html_tag_name>")); |
By CSS Selector: | driver.FindElement(By.CssSelector("Tag#Value_of_id_attribute")); |
By XPath: | driver.FindElement(By.XPath("//input[@type='submit']")); |
By Link Text: | driver.FindElement(By.LinkText("<link_text>")); |
By Partial Link Text: | driver.FindElement(By.PartialLinkText("<link_text>")); |
Working with Files |
|
Upload a file:
using OpenQA.Selenium; IWebElement fileInput = driver.FindElement(By.Id("file-upload")); fileInput.SendKeys(@"path\to\your\file.txt"); driver.FindElement(By.Id("file-submit")).Submit(); Read data from a text file:
using System.IO; string[] lines = File.ReadAllLines(@"path\to\your\file.txt"); foreach (string line in lines) { Console.WriteLine(line); } Read data from a CSV file:
using System.IO; using CsvHelper; using (var reader = new StreamReader(@"path\to\your\csvfile.csv")) using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture)) { var records = csv.GetRecords<dynamic>().ToList(); } Read data from an Excel file:
using OfficeOpenXml; using System.IO; FileInfo file = new FileInfo(@"path\to\your\excelfile.xlsx"); using (ExcelPackage package = new ExcelPackage(file)) { ExcelWorksheet worksheet = package.Workbook.Worksheets["Sheet1"]; int rowCount = worksheet.Dimension.Rows; int colCount = worksheet.Dimension.Columns; for (int row= 1; row <= rowCount; row++) { for (int col = 1; col <= colCount; col++) { Console.Write(worksheet.Cells[row, col].Value.ToString() + "\t"); } Console.WriteLine(); } } |
|
Selenium Navigators |
|
Navigate to a URL | driver.Navigate().GoToUrl("<URL>"); |
Refresh the page | driver.Navigate().Refresh(); |
Navigate forward in browser history | driver.Navigate().Forward(); |
Navigate back in browser history | driver.Navigate().Back(); |
Working with Windows |
|
Get the current window handle:
string mainWindowHandle = driver.CurrentWindowHandle; Get all window handles:
ReadOnlyCollection<string> allWindowHandles = driver.WindowHandles; Switch to a specific window:
string windowHandle = driver.CurrentWindowHandle; driver.SwitchTo().Window(windowHandle); Close the current window:
driver.Close(); |
|
Working with Frames |
|
Switch to a frame by name or ID:
driver.SwitchTo().Frame("frameName"); Switch to a frame by index:
driver.SwitchTo().Frame(1); Switch to a frame using a WebElement:
IWebElement iframe = driver.FindElement(By.CssSelector("#modal>iframe")); driver.SwitchTo().Frame(iframe); Switch back to the main content:
driver.SwitchTo().DefaultContent(); |
|
Working with Dropdowns (Select Elements) |
|
Find the dropdown element:
var dropdown = driver.FindElement(By.Id("dropdown_id")); Create a Select instance for the dropdown element:
var select = new SelectElement(dropdown); Select an option by visible text:
select.SelectByText("Option Text"); Select an option by value:
select.SelectByValue("option_value"); Select an option by index:
select.SelectByIndex(1); Deselect all options (only applicable for multi-select dropdowns):
select.DeselectAll(); Get all selected options:
var selectedOptions = select.AllSelectedOptions; Get the first selected option:
var firstSelectedOption = select.SelectedOption; |
|
Mouse Actions |
|
Click:
var actions = new Actions(driver); actions.Click(element).Perform(); Double click:
actions.DoubleClick(element).Perform(); Right click:
actions.ContextClick(element).Perform(); Drag and drop:
actions.DragAndDrop(sourceElement, targetElement).Perform(); Move to element:
actions.MoveToElement(element).Perform(); |
|
Working with Alerts |
|
Switch to an alert:
IAlert alert = driver.SwitchTo().Alert(); Enter text in an alert:
alert.SendKeys("Selenium"); Dismiss an alert:
driver.SwitchTo().Alert().Dismiss(); Retrieve alert text:
string alertText = alert.Text; |
|
Selenium Operations |
|
Launch a Webpage:
driver.Navigate().GoToUrl("<URL>"); Click a button:
var buttonElement = driver.FindElement(By.CssSelector("<button_element_css_selector>")); buttonElement.Click(); Print the page title:
var title = driver.Title; Console.WriteLine(title); Wait for an element to be visible:
var wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10)); var element = wait.Until(ExpectedConditions.ElementIsVisible(By.Id("<element ID>"))); Wait for an element to be clickable:
var wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10)); var element = wait.Until(ExpectedConditions.ElementToBeClickable(By.Id("<element ID>"))); Implicit wait:
driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(10); // 10 seconds Explicit wait:
var wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10)); var element = wait.Until(ExpectedConditions.ElementLocated(By.CssSelector(“<element_css_selector>”))); // 10 seconds Sleep:
Thread.Sleep(5000); // 5 seconds or 5000 milliseconds Take a screenshot:
var screenshot = ((ITakesScreenshot)driver).GetScreenshot(); screenshot.SaveAsFile("screenshot.png", ScreenshotImageFormat.Png); Clear the input field text:
var inputElement = driver.FindElement(By.CssSelector("<input_element_css_selector>")); inputElement.Clear(); Disable a field (set the ‘disabled’ attribute):
((IJavaScriptExecutor)driver).ExecuteScript(“document.querySelector(‘<element_css_selector>’).setAttribute(‘disabled’, ”);”); Enable a field (remove the ‘disabled’ attribute):
((IJavaScriptExecutor)driver).ExecuteScript("document.querySelector('<element_css_selector>').removeAttribute('disabled');"); |
|
Selenium Grid |
|
Start the hub
java -jar selenium-server-standalone-<version>.jar -role hub Start a node
java -jar selenium-server-standalone-<version>.jar -role node -hub http://localhost:4444/grid/register Server
http://localhost:4444/ui/index.html |
Alternative: write tests without a single line of code
testRigor is an AI-driven, end-to-end testing solution that empowers QA professionals to create and maintain complex automated tests without requiring coding skills. This comprehensive system supports testing of various platforms, including web, mobile, and desktop applications, and is suitable for functional regression, accessibility, load, and performance testing. By leveraging plain English statements and an advanced AI engine, testRigor provides excellent scalability, minimal maintenance, and faster test execution, setting itself apart from coded automation tools such as Selenium.
Organizations that have adopted testRigor have experienced significant improvements in their software testing processes, with increased test coverage, reduced defect escape rates, and accelerated release cycles. The platform not only enhances QA teams and delivery processes but also eliminates the need for maintaining testing infrastructure. The unique approach of testRigor allows for seamless collaboration among team members and ensures tests remain valid even after underlying framework changes or refactoring. This superior choice in test automation provides numerous key benefits, including cost savings, improved communication, and detailed test results for seamless debugging.
Selenium test:
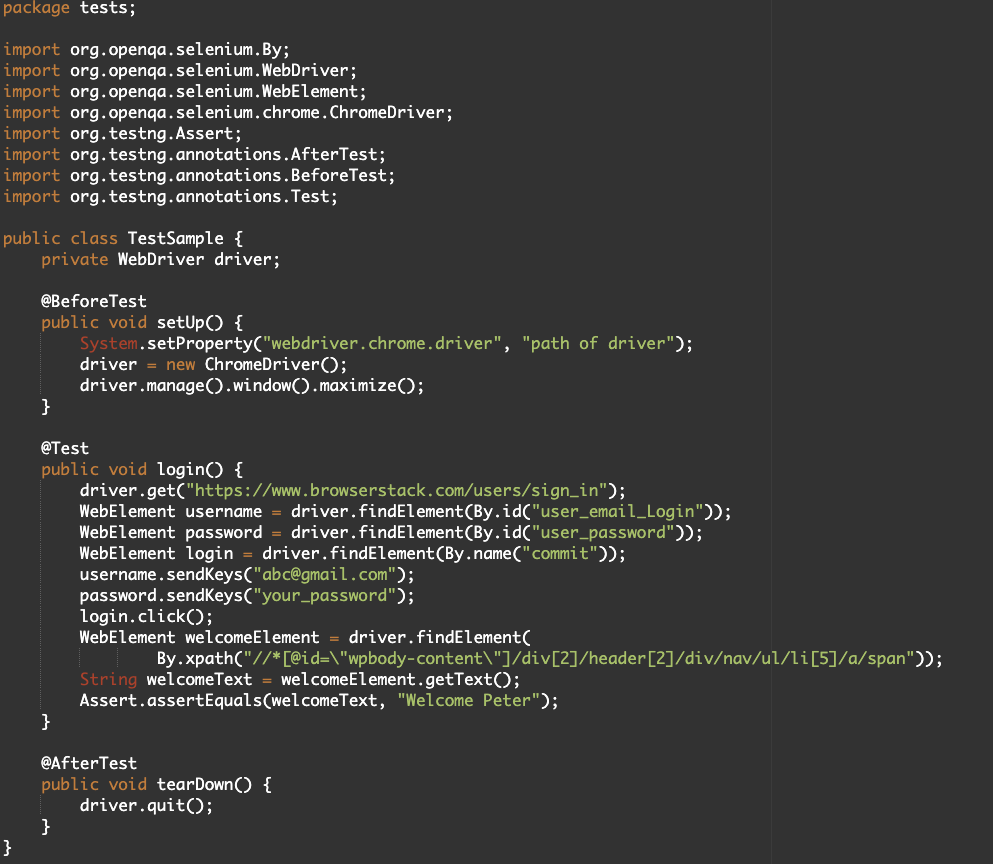
Same test in testRigor:
login check if page contains "Welcome Peter"