Zendesk Testing
|
Zendesk offers a variety of cloud-based software products focused on customer service, sales, and overall customer communication. Zendesk provides a suite of software-as-a-service (SaaS) products that help businesses improve their customer interactions.
Conceived as a software company aiming to simplify the customer support process, Zendesk introduced a cloud-based customer service platform that centralized customer interactions across multiple channels into a single, accessible interface.
Over the years, Zendesk has expanded its product lineup to include a variety of tools focused on improving customer engagement, support, and analytics, catering to an ever-growing global market. The products that Zendesk offers are:
Zendesk Sell
- Boost Sales Productivity: Manages sales pipelines, tracks leads, and helps close deals with features like contact management and opportunity tracking.
- Enhanced Collaboration: Integrates with Zendesk Support for a unified view of customer interactions, fostering better collaboration between sales and support teams.
- Data-Driven Decisions: Provides sales reporting and analytics to identify trends, measure performance, and optimize sales strategies.
- Simplified Sales Process: Offers tools for quote generation, email integration, and task management to streamline the sales workflow.
Zendesk Support
- Central Hub: Manages customer inquiries across various channels like email, phone, chat, and social media in one platform.
- Streamlined Workflow: Offers features like ticketing, automation, and self-service options to improve agent efficiency.
- Improved Customer Experience: Provides tools for knowledge base creation, community forums, and customer satisfaction surveys.
- Scalable Solution: Adapts to businesses of all sizes, from small teams to large enterprises.
Zendesk Sunshine
- Unified Customer Data: Creates a single customer profile by gathering data from all touchpoints, including website visits, support tickets, and purchases.
- Personalized Experiences: Enables businesses to personalize marketing campaigns, product recommendations, and customer support interactions.
- Deeper Customer Insights: Provides data analytics to understand customer behavior, identify trends, and make informed business decisions.
Zendesk Guide
- Knowledge Base Creation: Build a searchable library of articles, FAQs, how-to guides, and troubleshooting steps.
- Content Management: Zendesk Guide provides tools for creating, editing, and publishing content. You can manage content versions, assign ownership to articles, and collaborate with your team.
- Integration with Zendesk Support: Guide seamlessly integrates with Zendesk Support. Support agents can recommend relevant knowledge base articles to customers during interactions.
Zendesk Marketplace
Marketplace offers apps developed by third-party vendors. These apps integrate seamlessly with Zendesk products and extend functionality in various areas. For instance, there are apps for social media integration, project management, and sentiment analysis, allowing you to tailor the Zendesk experience to your specific needs.
Zendesk testing can be performed manually or through automated testing tools. Automated testing tools can speed up the testing process, improve accuracy, and reduce the risk of human error. For the front end, Zendesk uses HTML, CSS, and JavaScript; the server side mainly uses Ruby on Rails.
Testing Zendesk Integrations
Thoroughly testing Zendesk integrations is essential to ensure custom features, third-party apps, and external systems work flawlessly within Zendesk, delivering a smooth and efficient experience for both support agents and customers. These integrations can introduce complexities due to interactions with various systems like CRMs, analytics tools, and communication channels. Testing helps identify and address potential errors or unexpected behaviors before they impact end-users. This proactive approach ensures data accuracy, maintains system security, and preserves workflow efficiency.
To ensure there are no such issues, it’s always suggested that the application goes through different levels of testing, like unit testing, integration testing, and end-to-end testing.
Unit Testing Zendesk Integrations
Unit testing, when applied to Zendesk integrations, focuses on isolating and testing the individual functionalities of the integration within the Zendesk environment. While it doesn’t encompass the entire integration flow, it plays a crucial role in ensuring the building blocks work as expected.
We typically test during Zendesk unit testing:
-
Data Interactions:
- Test how your code retrieves data from Zendesk (tickets, users, etc.) using the Zendesk API.
- Verify data parsing and transformation to the format your application expects.
- Ensure proper data manipulation and updates are sent back to Zendesk.
-
API Calls:
- Validate the construction of API requests according to Zendesk’s documentation.
- Test proper handling of API responses (success, errors, edge cases).
-
Authentication:
- Verify that your code securely retrieves and uses Zendesk access tokens.
- Test handling of token expiration and refresh mechanisms.
-
Error Handling:
- Ensure your code gracefully handles potential errors during Zendesk interactions (network issues, API errors).
- Test that proper error messages are logged or returned to the user.
There isn’t one specific tool solely for unit testing Zendesk integrations. Instead, developers leverage general-purpose unit testing frameworks alongside potential mocking libraries depending on their programming language. Let’s review a few of the most popularly used tools.
JUnit
JUnit is a popular unit testing framework specifically designed for Java programming. When working with Zendesk integrations written in Java, JUnit provides a structured approach to write and execute tests that ensure your integration functions as expected. Improved code quality and faster development are a few benefits of using JUnit.
Let’s see how JUnit helps with unit testing Zendesk integrations:
- Test Case Organization: JUnit allows you to define individual test cases as methods within a Java class. Each test case focuses on a specific functionality of your integration code.
- Assertions: JUnit provides a set of assertion methods that verify the expected behavior of your code. You can use assertions to check if data retrieved from Zendesk matches what you anticipate or if actions performed on Zendesk (simulated through mocking) produce the desired outcomes.
- Test Runners: JUnit integrates seamlessly with most Java IDEs (like Eclipse and IntelliJ IDEA). These IDEs provide test runners that execute your JUnit test cases. The runner displays the results, highlighting any failed tests and pinpointing the line of code that caused the failure.
- Annotations: JUnit offers annotations like @Test and @BeforeClass/@AfterClass to organize your tests further. These annotations help specify which methods are actual test cases, and some can set up or tear down resources needed for testing (like establishing mock connections to Zendesk).
Pytest
Pytest is a powerful and popular unit-testing framework specifically designed for Python. When working with Zendesk integrations written in Python, pytest offers a flexible and comprehensive approach to writing and executing tests that ensure your integration functions as expected.
We have the following advantages of using Pytest for unit testing:
- Simple and Concise Syntax: Pytest allows you to define test cases directly as functions decorated with the @pytest.mark.test decorator (or simply def test_something()). This approach promotes readability and makes writing tests intuitive.
- Fixtures: Pytest provides a powerful fixture mechanism for managing shared test resources like mocked Zendesk connections or data setups. Fixtures can be scoped to individual test functions, modules, or even entire test sessions, ensuring efficient resource management.
- Automatic Discovery: Pytest automatically discovers test cases by searching for files and functions starting with test_ within your project directory. This eliminates the need to manually configure test runners, making it easier to get started with testing.
import pytest import requests_mock from zendesk_integration import create_ticket @pytest.fixture def ticket_data(): return { "subject": "Test ticket", "comment": { "body": "This is a test ticket" }, } def test_create_ticket_success(requests_mock, ticket_data): subdomain = "example" api_token = "test_api_token" mock_url = f"https://{subdomain}.zendesk.com/api/v2/tickets.json" # Mocking the POST request to return a success status code requests_mock.post(mock_url, json={"ticket": {"id": 123, "status": "created"}}, status_code=201) response = create_ticket(subdomain, api_token, ticket_data) assert response.status_code == 201 assert response.json()["ticket"]["status"] == "created" def test_create_ticket_failure(requests_mock, ticket_data): subdomain = "example" api_token = "test_api_token" mock_url = f"https://{subdomain}.zendesk.com/api/v2/tickets.json" # Mocking the POST request to return an error status code requests_mock.post(mock_url, json={"error": "Invalid request"}, status_code=400) response = create_ticket(subdomain, api_token, ticket_data) assert response.status_code == 400 assert "error" in response.json()
This code demonstrates how to use pytest and requests_mock to test the success and failure scenarios of the create_ticket function without actually making any real API requests to Zendesk. The requests_mock fixture is used to intercept and mock the requests made by the requests library, allowing you to specify the expected response data and status codes for different test cases.
Integration Testing Zendesk Apps
Integration testing for Zendesk integrations verifies how your custom functionality connects and interacts with the Zendesk platform and potentially other external systems. It goes beyond individual code units and focuses on ensuring a smooth flow of information between these systems.
Here, you’ll test scenarios like data exchange accuracy in both directions (sending data to Zendesk and receiving data from it). Error handling is crucial, so you’ll simulate issues like network failures or authentication problems to see how your integration responds and if it provides clear user feedback. Security is also a significant concern, so you’ll want to test for vulnerabilities that could expose sensitive information during data exchange.
Here are some tools and services that can help facilitate integration testing for Zendesk integrations:
Zendesk API Sandbox
Zendesk provides a sandbox environment that closely mirrors the production environment. It’s designed for testing and development purposes, allowing developers to test their integrations without affecting live data. It creates a separate, isolated environment that mimics your production Zendesk instance.
Zendesk API sandbox offers several advantages like:
- Safe Experimentation: Unlike testing in your production Zendesk, the Sandbox provides a completely isolated environment. You can freely test your integration’s functionalities without any risk of affecting real user data or workflows. This allows you to create test tickets, manipulate data, and simulate various scenarios without worries.
- Early Issue Detection: By testing in the Sandbox, you can identify and fix integration bugs much earlier in development. This saves time and resources compared to catching issues after deploying to production, which could potentially disrupt real users.
- Realistic Testing: The Sandbox closely mimics your Zendesk setup, allowing you to test your integration in a familiar environment. This increases the confidence that your integration will function smoothly when deployed to production.
- Improved Integration Quality: By thoroughly testing your integration in the Sandbox, you can ensure it interacts with the Zendesk API as expected. This leads to a more robust and reliable integration that delivers a better user experience.
Postman
Postman is a popular API testing tool that plays a vital role in integration testing for Zendesk integrations. It allows you to simulate real-world interactions between your custom functionality and the Zendesk API. There are many advantages of using Postman for Zendesk integration testing:
- Simulating API Interactions: Postman allows you to build requests that mirror how your Zendesk integration would typically communicate with the Zendesk API. You can specify request methods (GET, POST, PUT, etc.), URLs, headers, and body parameters like your integration code would.
- Sending and Inspecting Responses: Once you’ve built your request, Postman sends it to the Zendesk API (or the Zendesk API Sandbox for testing purposes) and displays the response. This lets you see exactly what data the Zendesk API returns based on your request.
- Verifying Data Exchange: By examining Postman’s request and response payloads, you can verify if data is being exchanged accurately between your integration and Zendesk. This helps ensure your integration retrieves or sends the intended information.
- Identifying Integration Issues: By inspecting responses and status codes, you can identify discrepancies or errors in how your integration interacts with the Zendesk API. This allows you to troubleshoot and fix issues before deploying your integration to production.
The tools that we discussed above are mainly for API integration testing. So, when it comes to UI integration testing, the commonly used tool is Selenium, which we can discuss in E2E testing.
E2E testing for Zendesk Integrations
End-to-end (E2E) testing for Zendesk integrations goes beyond individual components and the integration itself. It simulates real user scenarios, testing how data flows across your system and into Zendesk. This might involve creating a test user, performing actions within your application that trigger interactions with Zendesk (like submitting a support ticket), and verifying if those actions are reflected accurately in Zendesk. E2E testing helps identify issues related to data consistency, user workflows, and overall integration functionality within the broader context of your application and Zendesk.
Let’s check out a few commonly used E2E testing tools.
Selenium
While Selenium has been a popular choice for UI automation testing due to its open-source nature and customizability, it has limitations. As the number of automated tests using Selenium grows, maintaining them can become challenging. Complex code structures can make debugging difficult and time-consuming.
Below are some reasons why some organizations might choose to explore alternatives to Selenium for UI automation testing:
- Scalability Issues: Managing an extensive suite of Selenium tests can become cumbersome. Complex code and intricate test dependencies can make it challenging to scale your test suite efficiently.
- Maintenance Burden: Debugging intricate Selenium tests can be a time-consuming process. As your test suite grows, the maintenance overhead associated with Selenium can become significant.
- Limited Scope: Selenium primarily focuses on web application UIs. It’s not ideal for testing mobile apps, desktop applications, or API interactions, requiring additional tools for comprehensive testing.
- Browser Compatibility Challenges: Maintaining compatibility with different browsers and their frequent updates can add complexity to Selenium test maintenance.
Above are a few reasons why Selenium is not used for automation testing. Here, we are not covering much detail about Selenium, but if you would like to know about building Selenium tests, you can refer to this blog.
One of the most commonly used tools explicitly designed for E2E testing is testRigor. Let us learn about it in detail.
testRigor for E2E Testing
As we know, many codeless automation tools are available in the market. Still, testRigor distinguishes itself through a combination of features designed to streamline the testing process and empower a broader range of users.
Let’s check what makes testRigor a standout choice:
- Effortless Setup: Forget time-consuming infrastructure setup and installation hassles. testRigor is a cloud-hosted solution that allows you to sign up, purchase a plan, and start testing immediately. This translates to significant cost and time savings compared to traditional tools.
- Integration Arsenal: No more scrambling to find compatible integrations after installation. testRigor boasts a comprehensive library of pre-built integrations, eliminating compatibility headaches and ensuring smooth operation even during upgrades. You can find a detailed list of supported integrations here.
- Plain English Power: testRigor breaks the barrier of programming expertise. You can create test scripts using natural language, similar to describing test steps in plain English. This empowers manual testers to create automated scripts faster than traditional automation tools engineers use. Additionally, business analysts and stakeholders can easily understand and modify these scripts, fostering better collaboration.
- Generative AI Boost: Leverage the power of generative AI to streamline test creation. Simply provide a description, and testRigor can generate test cases or test data automatically. This reduces manual effort and ensures your test suite covers a broader range of scenarios.
-
Say Goodbye to Flaky Locators: Unlike traditional tools that depend on fragile XPath or CSS selectors, testRigor simplifies element identification for a more human-like experience. You simply describe the element you want to interact with by mentioning the text you see on the screen or its relative position. We can say this as testRigor locators. For example,
click "cart" click on button "Delete" below "Section Name"
-
Unified Testing Platform: Eliminate the hassle of managing multiple testing frameworks for web, mobile, and desktop applications. testRigor acts as a comprehensive testing powerhouse, supporting a wide range of testing needs within a single platform. This simplifies your testing process and reduces tool sprawl and associated maintenance overhead. You can perform the below testing types using plain English commands:
- Web and Mobile browser testing
- Mobile testing
- Desktop app testing
- API testing
- Visual testing
- Accessibility testing
If you are excited to learn more about the features of testRigor, you can visit this page.
open url "https://www.loginurl.com" enter stored value "UserName" into "User Name" enter stored value "password" into "Password" click "Sign in" click “Employees” click “Add New Employee” enter "John" into "First Name" enter "Doe" into "Last Name" click "Submit"
The example above clearly shows the simplicity of testRigor test script creation, maintenance, and other test activities as automation progresses to the next level.
Zendesk Marketplace Certification process
Once you create a Zendesk marketplace account and develop the app using Zendesk guidelines, you can submit your integration on Zendesk Marketplace for review.
App Review Process
The Zendesk Marketplace team will review your app for the following criteria:
- Security: Ensures your app follows security best practices and doesn’t introduce vulnerabilities to Zendesk environments.
- Functionality: Verifies your app functions as advertised and integrates seamlessly with Zendesk.
- User Experience (UX): Evaluate your integration’s user interface and overall user experience.
- Documentation: Check if you provide clear and comprehensive documentation for users to understand and install your app.
- Compliance: Ensures your app adheres to Zendesk’s Marketplace policies and guidelines.
Approval and Publication
- The Zendesk Marketplace team might provide feedback on your app and request changes before approval.
- Once your app successfully passes the review process, the Zendesk Marketplace team will publish it on the Marketplace.
- Your app will become available for discovery and installation by Zendesk users.
Resources:
- Zendesk Marketplace Seller Guide: https://support.zendesk.com/hc/en-us/articles/4408823868954-Working-with-Zendesk-Marketplace-apps-in-Sell
- Zendesk App Security Review: http://developer.zendesk.com/
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
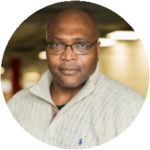