Regex Random String Generation Support
In addition to a simple way to generate random data, testRigor also supports a way to use Regular Expressions (Regex) for more complex scenarios. For instance, you can generate a random tag like this:
generate by regex "<(a|button)>data<\/\1>", then enter into "Tag data" and save as "generatedTag"
which generates <a>data</a>
or <button>data</button>
. Or you might want
generate by regex "https://(www\.)?([a-z][a-z0-9-]{2,30}[a-z0-9]\.){1,5}(com|edu|co\.uk|info|io)", then enter into "URL" and save as "generatedUrl"
which generates a unique URL in the format of “https://www.abc.de.com”.
The rule of thumb to use ReGex is to avoid it as much as possible since it is very hard to read for anyone who is not familiar with it. Always try simple template first. One of the use cases where you might be forced to use ReGex is if what you generate must have a variable length.
testRigor supports both generation of random strings based on Regex as well as validating and extracting data.
Regex Random Data Generation Cookbook
For simple scenarios like generating emails, phone numbers, credit card numbers, etc. please see simple template. The full command will look like this:
generate by regex "", then enter into "Email" and save as "newEmail"
Regex | Use case |
---|---|
[A-Z][a-z]{2,50} |
Random word starting from upper case like “Hello” |
[a-z]{2,50} |
Random word all lower case like “hi” |
[1-2][0-9]{0,8} |
Random number |
((1[0-2])|0[1-9])-((0[1-9])|([1-2][0-8]))-((20[0-2][0-9])|(19[0-9]{2})) |
Dates in mm-dd-yyyy format |
((20[0-2][0-9])|(19[0-9]{2}))-((1[0-2])|0[1-9])-((0[1-9])|([1-2][0-8])) |
Dates in yyyy-mm-dd format |
((1[0-2])|(0[0-9])):([0-5][0-9]) [AP]M |
Time in 11:34 AM AM/PM format |
((2[0-3])|([0-1][0-9])):([0-5][0-9]) |
Time in 14:25 24hr format |
(www\.)?([a-z][a-z0-9-]{2,30}[a-z0-9]\.){1,5}[a-z]{2,3} |
Domain name |
https://(www\.)?([a-z][a-z0-9-]{2,30}[a-z0-9]\.){1,5}[a-z]{2,3} |
URL without path |
https?://(www\.)?([a-z][a-z0-9-]{2,30}[a-z0-9]\.){1,5}[a-z]{2,3}(/[a-z0-9-]{2,10}){0,5}/?(#[a-z0-9])? |
Full random URL |
((25[0-5]|2[0-4]\d|[01]?\d\d?)\.){3}(25[0-5]|2[0-4]\d|[01]?\d\d?) |
IP v4 |
((\+[0-9][0-9]? [0-9]{3})|(\(0[0-9]{3}\))) [0-9]{3} [0-9]{4} |
International phone numbers in a standard E.123 format |
Regex Random Generation Syntax Support
testRigor provides full support for Java-like regular expressions with POSIX character classes and full multibyte Unicode support for international characters. A simple example is:
generate by regex "[A-Z][a-z]{2,50}", then enter into "Name" and save as "generatedName"
Construct | Description | Example |
---|---|---|
A text |
All regular text is copied as is. This is not changeable part. In the example on the right Lakshmi will be posted as is. | generate by regex "Lakshmi", then enter into "Name" and save as "name" |
| |
Select one option from another. testRigor will randomly choose from the options available. In the example on the right, it will use either Lakshmi or Meera | generate by regex "Lakshmi|Meera", then enter into "Name" and save as "name" |
() |
Grouping parts. In case if something needs to be executed on the whole rater than the last symbol. Lakshmi+ will result in “Lakshmiiiiii”, where as (Lakshmi )+ will result in “Lakshmi Laskhmi Lakshmi …” |
generate by regex "(Lakshmi )+", then enter into "Name" and save as "name" |
? |
Add or do not add the part randomly. The example would result in “Lakshmi” about 50% or the time and empty string other 50% | generate by regex "(Lakshmi)?", then enter into "Name" and save as "name" |
+ |
Repeat the part at least one and at most 100 times. The example on the right will have at least one Lakshmi but can also have up to 99 more. | generate by regex "(Lakshmi )+", then enter into "Name" and save as "name" |
* |
Repeat the part at least zero and at most 100 times. The example on the right could produce an empty string or up to 100 “Lakshmi” strings. | generate by regex "(Lakshmi )*", then enter into "Name" and save as "name" |
{N} |
Repeat the part precisely N times. In example on the right “Lakshmi ” will be repeated twice like this: “Lakshmi Lakshmi “. | generate by regex "(Lakshmi ){2}", then enter into "Name" and save as "name" |
{N,M} |
Repeat the part between N and M times. In example on the right “Lakshmi ” can be repeated either once or twice. | generate by regex "(Lakshmi ){1,2}", then enter into "Name" and save as "name" |
[abc] |
Select just one of the characters specified. In the example only a, b, or c will be selected randomly. | generate by regex "[abc]", then enter into "Name" and save as "name" |
[a-c] |
Select just one of the characters in the range specified. In the example only a, b, or c will be selected randomly. | generate by regex "[a-c]", then enter into "Name" and save as "name" |
[^abc] |
If the first symbol in square brackets is ^ , then testRigor will use visible symbols except the ones provided. In the example, it could be any character like ‘A’, ‘7’, ‘d’, ‘&’, etc. This reversal applies universally and can be used with ranges as well, like [^a-c] , which in this case, will have the same effect. |
generate by regex "[^abc]", then enter into "Name" and save as "name" |
\s |
One character of any whitespace like space or tab. | generate by regex "\s", then enter into "Name" and save as "name" |
\w |
One character of lower and upper case Latin letters, digits and ‘_’. Equivalent to [a-zA-Z0-9_] . |
generate by regex "\w", then enter into "Name" and save as "name" |
\d |
One digit character. Equivalent to [0-9] . |
generate by regex "\d", then enter into "Name" and save as "name" |
. |
Any character. testRigor will generate characters that can be typed on a US keyboard randomly. | generate by regex "\d", then enter into "Name" and save as "name" |
\ |
Escapes the following special character. For example, if you’d like to include ‘[‘ and ‘]’ into the set of characters you use, you could specify[\[\]] |
generate by regex "[\[\]]", then enter into "Name" and save as "name" |
\n |
Just one new line character. | generate by regex "\n", then enter into "Name" and save as "name" |
\t |
Just one tab character. | generate by regex "\t", then enter into "Name" and save as "name" |
\N |
A reference to the previously generated Nth group. For example, <(a|button)>data<\/\1> will generate the opening and closing of the same tag (“a” or “button” in this case). |
generate by regex "<(a|button)>data<\/\1>", then enter into "Code" and save as "code" |
Advanced Topics
When we were creating the Regex generator we wanted the syntax to be as close as it is technically possible to Java version of Regex (Pattern). You might find a lot of additional quirks and features to be similar to Java’s version. However, the parser was built from scratch and had to be generation-compatible, so there are some differences. For instance, we included support for almost all POSIX character classes. However, we emulated how Java works with backreferences and group numbering. Also, we tried to build parser in a way that would produce the most readable and understandable error messages we could do.
Question | Answer |
---|---|
How does testRigor generates random? | testRigor generates random numbers with pseudo-random sequence seeded with nanoseconds. |
Is there a bound for unbounded operations like * ? |
Yes, default bound is 100 |
What is I need more than 100 symbols generated? | You can use range, and specify any number as upper bound. testRigor will repeat up to your specified number or 10,000, whichever is smaller. |
Does testRigor support intersections like [a&&[b]] ? |
Yes for validation/search. Not yet for random data generation. |
Does testRigor support multicharacter Unicode symbols? | Yes, naturally out of the box. You can specify 😀{3} to get “😀😀😀” |
Does testRigor support non-capturing groups? | Yes for validation/search. For generation positive non-capturing groups like (?<=group) will generate data, but negative groups like (?!group) will be ignored. |
Does testRigor support flags like (?i) ? |
Yes for validation/search. Not yet for random data generation. |
More simple use cases like phone numbers, passport numbers, SSNs, emails, etc. see simple template.
Regex for validations and search
You can use Regex for search/validation in testRigor. testRigor will use Java 11's Regex Pattern to complile/process the Regex in this case. See the full supported syntax here. Examples of usages are:
check that page has regex "Current time: [0-2][0-9]:[0-5][0-9]" check that page doesn't have regex "fail|crash|500" check that URL matches regex "https://mycompany\.com/list/item/[a-z0-9]{2,30}" check that text below "Current time" matches regex "[0-2][0-9]:[0-5][0-9]" grab value of regex "[0-2][0-9]:[0-5][0-9]" and save it as "currentTime"
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
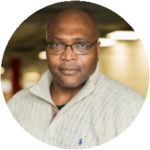