Joomla Testing
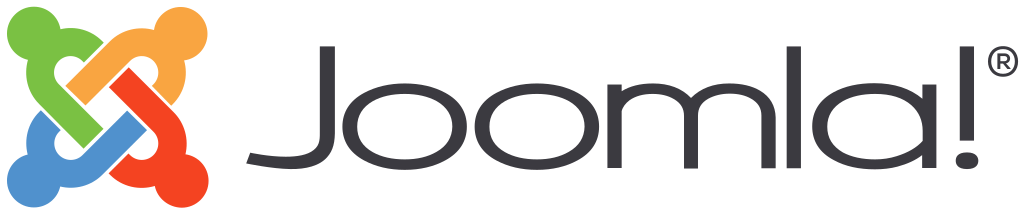
What is Joomla?
Joomla is a popular open-source content management system (CMS) for creating and managing websites and online applications. It is written in PHP and uses MySQL or PostgreSQL as its database management system. Joomla allows users to create and manage websites with little to no technical knowledge, thanks to its user-friendly interface, rich set of features, and extensive library of templates and extensions.
Joomla Unit Testing
Unit tests are designed to check various functions, methods, and classes in isolation. This preliminary testing is crucial in complex Content Management System (CMS) platforms like Joomla. Although the focus is strictly on obtaining the correct output from the code unit, it is an excellent way to determine whether any breaking changes have been introduced into the system. As the emphasis is on isolated and lightweight testing, unit tests often employ mocking to minimize external dependencies.
Joomla uses the PHPUnit framework for unit testing. Here is an example of a test case for a JArrayHelper class in Joomla. The goal of this test is to verify that the getColumn method of the JArrayHelper class returns the correct data. The test case extends the PHPUnit\Framework\TestCase class, which provides methods for setting up and tearing down test fixtures. In this case, the setUp and tearDown methods are left empty.
The test case includes one test method named testGetColumn(). This method creates an array of arrays called $test_array, where each sub-array represents a row of data with values for three columns: 'sport', 'teams', and 'country'. The test method then calls the getColumn method of the JArrayHelper class and passes in the $test_array and the name of the column ('country') to retrieve. The result is stored in $result_array.
Finally, the test method uses the PHPUnit assertion method assertEquals() to compare the $result_array with an expected array of values for the 'country' column. If the two arrays are equal, the test passes. If not, the test fails with an error message.
require_once JPATH_BASE.'/libraries/joomla/utilities/arrayhelper.php'; class JArrayHelperTest extends PHPUnit\Framework\TestCase { /** * Sets up the fixture, for example, opens a network connection. * This method is called before a test is executed. * * @access protected */ protected function setUp(): void { } /** * Tears down the fixture, for example, closes a network connection. * This method is called after a test is executed. * * @access protected */ protected function tearDown(): void { } /** * Simple Test for getColumn method. */ public function testGetColumn(): void { $test_array = [ [ 'sport' => 'football', 'teams' => '10', 'country' => 'United States' ], [ 'sport' => 'badminton', 'teams' => '8', 'country' => 'Germany' ], [ 'sport' => 'basketball', 'teams' => '12', 'country' => 'Canada' ] ]; $result_array = JArrayHelper::getColumn($test_array, 'country'); $this->assertEquals( ['United States', 'Germany', 'Canada'], $result_array, 'We did not get the proper column data back' ); } }
Joomla is also compatible with Codeception which uses PHPUnit under the hood.The syntax is quite similar to PHPUnit. However, Codeception offers some helpful utilities to make testing more straightforward. You can install Codeception in your project using Composer.
namespace Tests\Unit; use Tests\Support\UnitTester; use App\User; class UserTest extends \Codeception\Test\Unit { /** * @var UnitTester */ protected $tester; public function testValidation(): void { $user = new User(); $user->setName(null); $this->assertFalse($user->validate(['username'])); $user->setName('toolooooongnaaaaaaameeee'); $this->assertFalse($user->validate(['username'])); $user->setName('davert'); $this->assertTrue($user->validate(['username'])); } }
The example demonstrates a simple test case for validating a user's username using the User class. The test case checks if the username is valid in three different scenarios: when it's null, when it's too long, and when it's an acceptable length.
You can find in-depth documentation on how to write and run these test cases here.
Joomla Integration Testing
Integration testing is the process of evaluating how different components of a system work together. This is applicable to APIs, forms, and database interactions. Some examples include integration with a third-party system or an API. Let’s take a look at some more scenarios that are specific to applications built using Joomla and where integration testing will come in handy.
- When a Joomla site uses third-party extensions like plugins, modules, or components, it's important to ensure that these extensions work well together and do not conflict with each other.
- Joomla has a powerful access control list system that allows administrators to set granular permissions for different user groups. Integration testing can help ensure that these settings work as intended and that users can access only the content they are authorized to view.
- Joomla sites often use different templates for different pages or sections of the site. Integration testing can help ensure that templates work well with other extensions, and that content is displayed correctly across all templates.
- Joomla has a caching system that can help speed up page load times. Integration testing can ensure that caching works correctly and that cached content is refreshed when necessary.
- Joomla has a multi-language feature that allows sites to be translated into different languages. Integration testing can ensure that all content is correctly translated and that language settings do not conflict with other extensions.
- Some Joomla sites use custom code or scripts to add functionality or integrate with other systems. Integration testing can help ensure that custom code works well with other extensions and does not introduce bugs or conflicts.
- Joomla sites often integrate with third-party services like payment gateways, social media platforms, or analytics tools. Integration testing can ensure that these integrations work as intended and that data is properly transmitted and processed.
Integration testing can be performed using automated testing tools, such as Codeception or PHPUnit, or through manual testing. The choice of testing method depends on the complexity of the application, the level of interaction between components, and the resources available for testing.
Joomla End-to-End Testing
End-to-end testing is a comprehensive approach that evaluates an entire system or application from the user's perspective to ensure that it functions as expected. In the context of Joomla applications, end-to-end testing involves testing the complete functionality of the website or application, including the frontend, backend, and all the integrated components, to confirm that they work together seamlessly and meet the desired requirements.
The primary goal of end-to-end testing is to simulate real-world user scenarios and validate that the system behaves correctly under various conditions. This testing type can help uncover issues that might not be detected during unit or integration testing, such as problems with user interfaces, workflows, data handling, or system configurations.
- Testing the user interface (UI) and user experience (UX) to ensure that the site is easy to navigate, responsive, and visually appealing across different devices and browsers.
- Verifying that the frontend and backend components of the application interact properly, including forms, user authentication, content management, and data processing.
- Checking the functionality of any custom or third-party extensions, modules, and plugins, and ensuring that they integrate seamlessly with the Joomla application.
- Assessing the performance, security, and reliability of the application, including load times, data protection, and error handling.
- Selenium with PHPUnit
- Codeception
- testRigor
Using Selenium
Joomla offers support for performing system testing using Selenium. When writing these test cases, you need to think from the user's perspective. Joomla supports using Selenium IDE to record tests which can be converted into PHP code.
PHPUnit also has an extension called Selenium2TestCase which allows QA to write functional tests for web applications using the Selenium WebDriver API. It provides a set of methods for interacting with web pages and simulating user actions. When using PHPUnit_Extensions_Selenium2TestCase, you can define tests that launch a web browser, navigate to a page, and interact with it to test various aspects of the page's behavior. For example, you can test whether certain elements are present on the page, whether form submissions result in the expected behavior, and whether specific interactions trigger expected changes to the page. Unlike the older versions, the latest versions of PHPUnit do not document this class; it is still widely used for automated testing.
The main downside is that this method is not as versatile and does not cover many common use cases that are likely to occur in CMS websites.
Using Codeception
You can use Codeception to write end-to-end tests for your application. This tool also supports the BDD approach for testing. Codeception's BDD support is based on the Gherkin language, which is used to describe the behavior of a system in a structured, human-readable way. Gherkin uses a set of keywords, such as Given, When, Then, And, and But, to describe the steps of a scenario.
Codeception's BDD support allows developers to write Gherkin feature files that describe the system's behavior, and then generate PHP code based on those feature files that can be executed as tests. Let's take a look at the below example.
First, a story is defined using the Gherkin language. Here's an example:
- In order to buy products
- As a customer
- I want to be able to purchase several products at the same time
-
Scenario:
- Given I have a product with a $600 price in my cart And I have a product with a $1000 price When I go to checkout process Then I should see that total number of products is 2 And my order amount is $1600
<?php namespace Step\Acceptance; use AcceptanceTester; class CheckoutProcessSteps extends AcceptanceTester { /** * @Given I have a product with a :price price in my cart */ public function iHaveAProductWithAPriceInMyCart($price) { $I = $this; $I->amOnPage('/product_page_url'); $I->fillField('price', $price); $I->click('Add to cart'); } /** * @And I have a product with :price price */ public function iHaveAProductWithPrice($price) { $I = $this; $I->amOnPage('/product_page_url'); $I->fillField('price', $price); $I->click('Add to cart'); } /** * @When I go to checkout process */ public function iGoToCheckoutProcess() { $I = $this; $I->amOnPage('/checkout'); } /** * @Then I should see that total number of products is :numProducts */ public function iShouldSeeThatTotalNumberOfProductsIs($numProducts) { $I = $this; $I->seeNumberOfElements('.product', $numProducts); } /** * @And my order amount is :orderAmount */ public function myOrderAmountIs($orderAmount) { $I = $this; $I->see($orderAmount, '.order-total'); } }
This step definition file defines a class called CheckoutProcessSteps that extends the AcceptanceTester class, which provides methods for interacting with the application. The methods in this class correspond to the steps in the Gherkin feature file and use Codeception's built-in methods to navigate through the application and perform the necessary actions.
<?php use Step\Acceptance\CheckoutProcessSteps; class CheckoutProcessCest { public function checkoutProcessTest(CheckoutProcessSteps $I) { $I->iHaveAProductWithAPriceInMyCart(600); $I->iHaveAProductWithPrice(1000); $I->iGoToCheckoutProcess(); $I->iShouldSeeThatTotalNumberOfProductsIs(2); $I->myOrderAmountIs(1600); } }
Using testRigor
The primary advantages of testRigor include its simplicity, ease of maintenance, and scalability. Often, the end user's perspective on interacting with a system, which is crucial for creating end-to-end test cases, comes from non-technical individuals such as product owners, experienced manual testers, business analysts, or client-facing teams. However, if test cases must be coded, this perspective may not be accurately translated due to limited engagement from a diverse audience. testRigor addresses this issue by enabling test cases to be written in plain English, facilitating easy collaboration, review, and editing of test scenarios. Additionally, testRigor supports Behavior-Driven Development (BDD) right out of the box - also without the need for coding.
With its stability, low test maintenance, and straightforward test case creation, testRigor is well-equipped to ensure the quality of your website. Content Management Systems (CMSs) often require testing use cases that involve sending emails or SMS alerts, which testRigor can accommodate. As a cloud-based application, testRigor allows users to begin automating without the need to install and configure software, unlike many other automation tools. The tool supports cross-platform testing across web, mobile, and desktop environments, covering a wide range of testing needs. You can explore more benefits of using testRigor here.
Below is the same test case as above. Notice how clean and concise it is. There are no step definitions or test files needed. This can give you an idea of how manual testers are able to author tests up to 15x faster with testRigor, compared to other tools.
login click on "Cart" grab value from "Item 1 price" and save it as "Price 1" grab value from "Item 2 price" and save it as "Price 2" click on "Check Out" check that page contains "2" to the right of "Total number of items" check that page contains expression "$(Price 1) + $(Price 2)" below "Total Amount"
How to do End-to-end Testing with testRigor
Let us take the example of an e-commerce website that sells plants and other gardening needs. We will create end-to-end test cases in testRigor using plain English test steps.
Step 1: Log in to your testRigor app with your credentials.
Step 2: Set up the test suite for the website testing by providing the information below:
- Test Suite Name: Provide a relevant and self-explanatory name.
- Type of testing: Select from the following options: Desktop Web Testing, Mobile Web Testing, Native and Hybrid Mobile, based on your test requirements.
- URL to run test on: Provide the application URL that you want to test.
- Testing credentials for your web/mobile app to test functionality which requires user to login: You can provide the app’s user login credentials here and need not write them separately in the test steps then. The login functionality will be taken care of automatically using the keyword
login
. - OS and Browser: Choose the OS Browser combination on which you want to run the test cases.
- Number of test cases to generate using AI: If you wish, you can choose to generate test cases based on the App Description text, which works on generative AI.
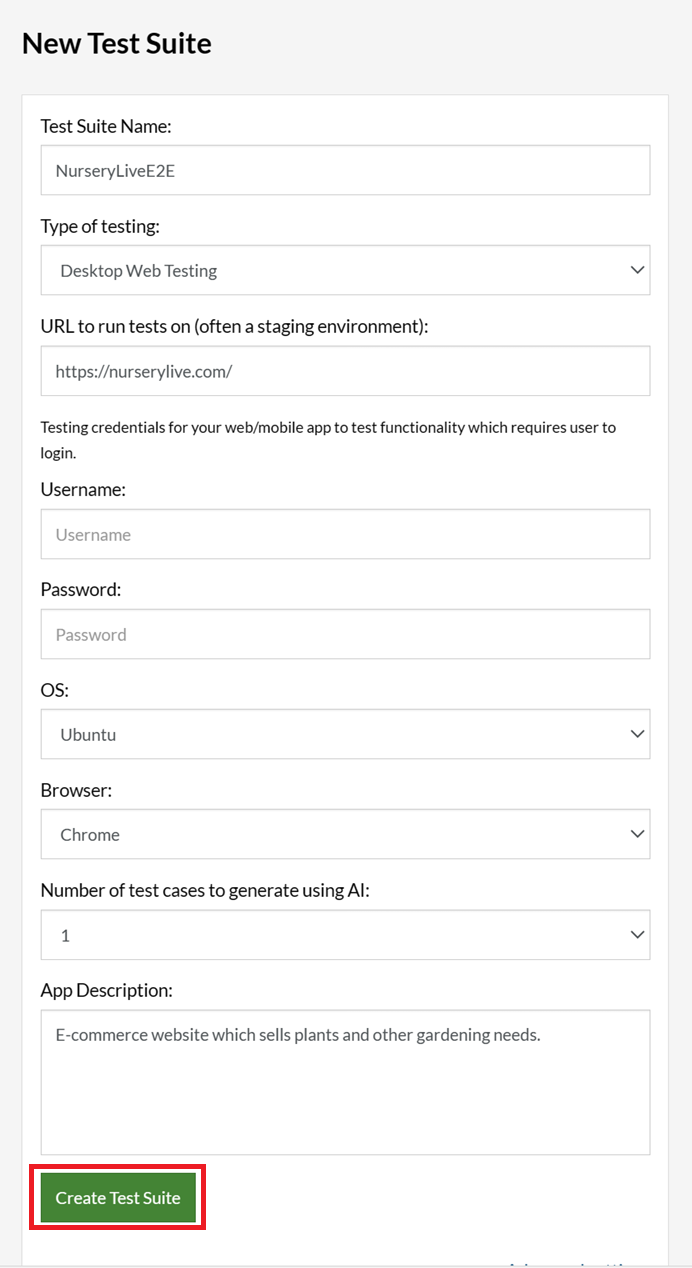
Step 3: Click Create Test Suite.
On the next screen, you can let AI generate the test case based on the App Description you provided during the Test Suite creation. However, for now, select do not generate any test, since we will write the test steps ourselves.
Step 4: To create a new custom test case yourself, click Add Custom Test Case.
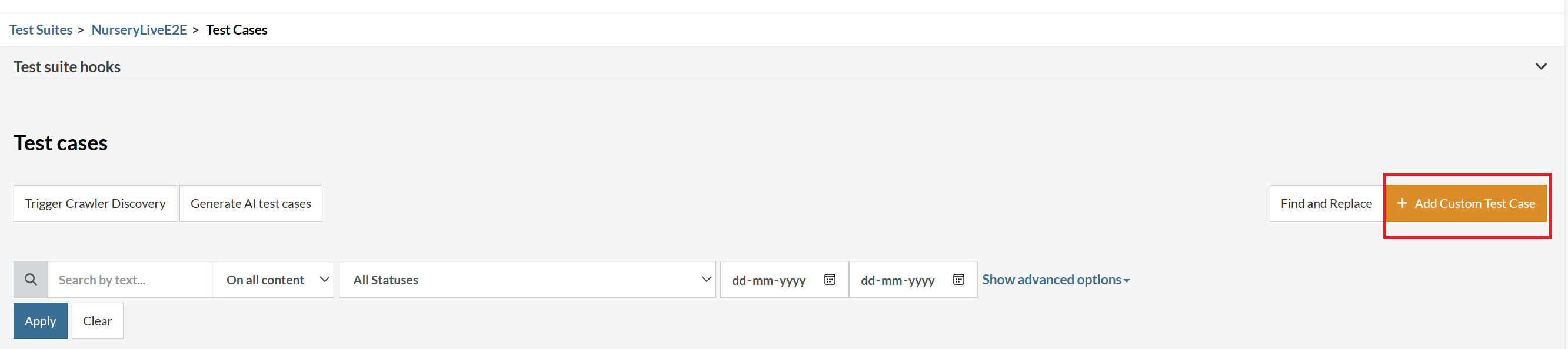
Step 5: Provide the test case Description and start adding the test steps.
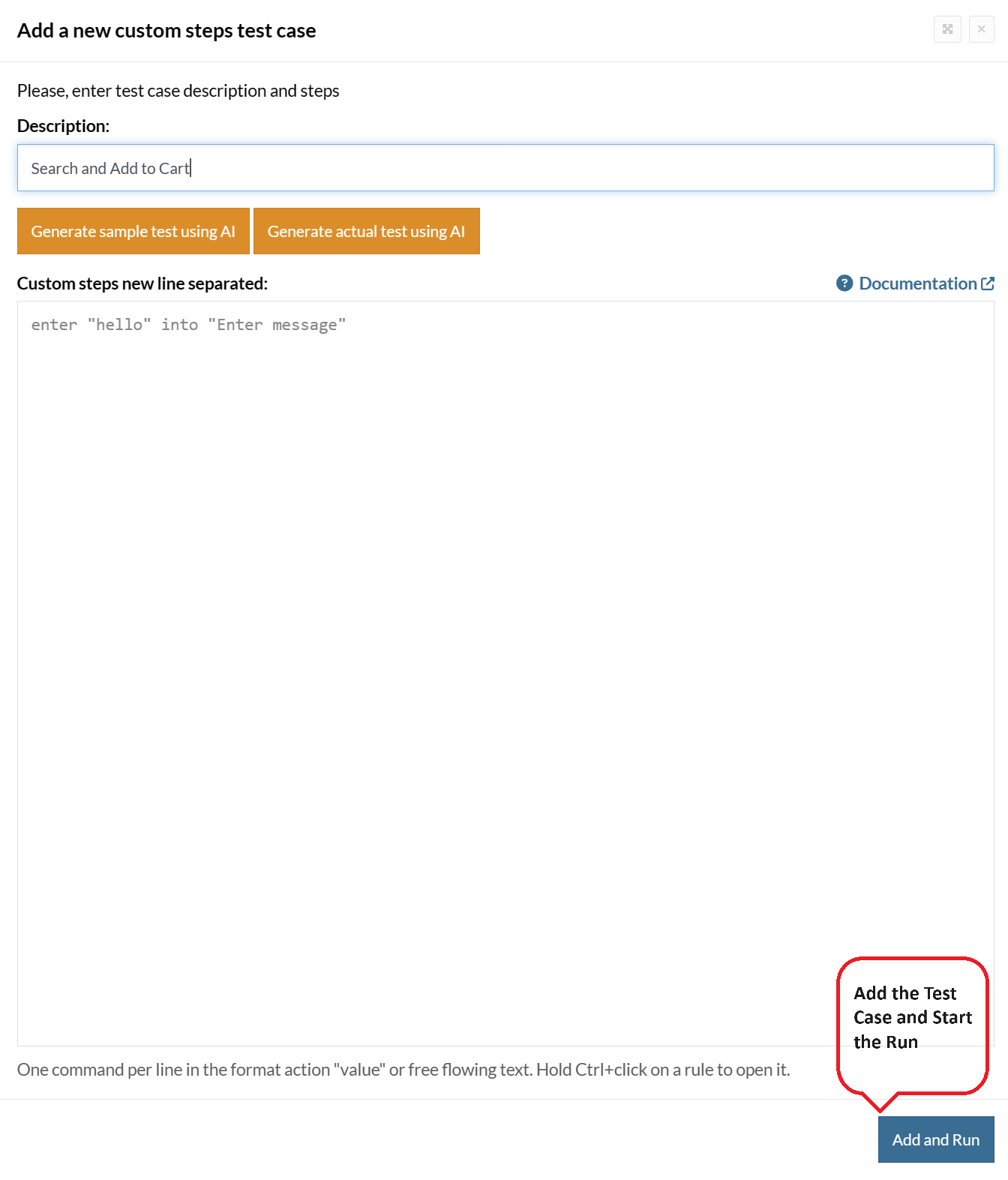
For the application under test, i.e., e-commerce website, we will perform below test steps:
- Search for a product
- Add it to the cart
- Verify that the product is present in the cart
Test Case: Search and Add to Cart
Step 1: We will add test steps on the test case editor screen one by one.
testRigor automatically navigates to the website URL you provided during the Test Suite creation. There is no need to use any separate function for it. Here is the website homepage, which we intend to test.
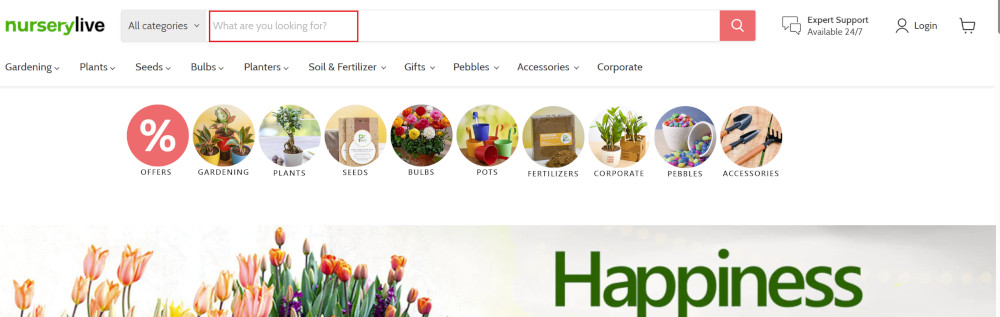
First, we want to search for a product in the search box. Unlike traditional testing tools, you can identify the UI element using the text you see on the screen. You need not use any CSS/XPath identifiers.
click "What are you looking for?"
Step 2: Once the cursor is in the search box, we will type the product name (lily), and press enter to start the search.
type "lily" enter enter
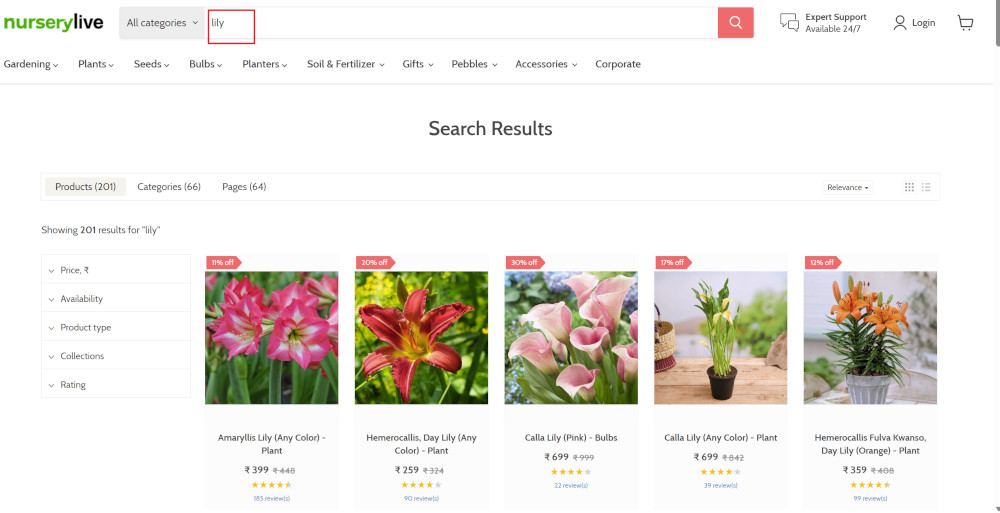
Search lists all products with the “lily” keyword on the webpage.
Step 3: The lily plant we are searching for needs the screen to be scrolled; for that testRigor provides a command. Scroll down until the product is present on the screen:
scroll down until page contains "Zephyranthes Lily, Rain Lily (Red)"
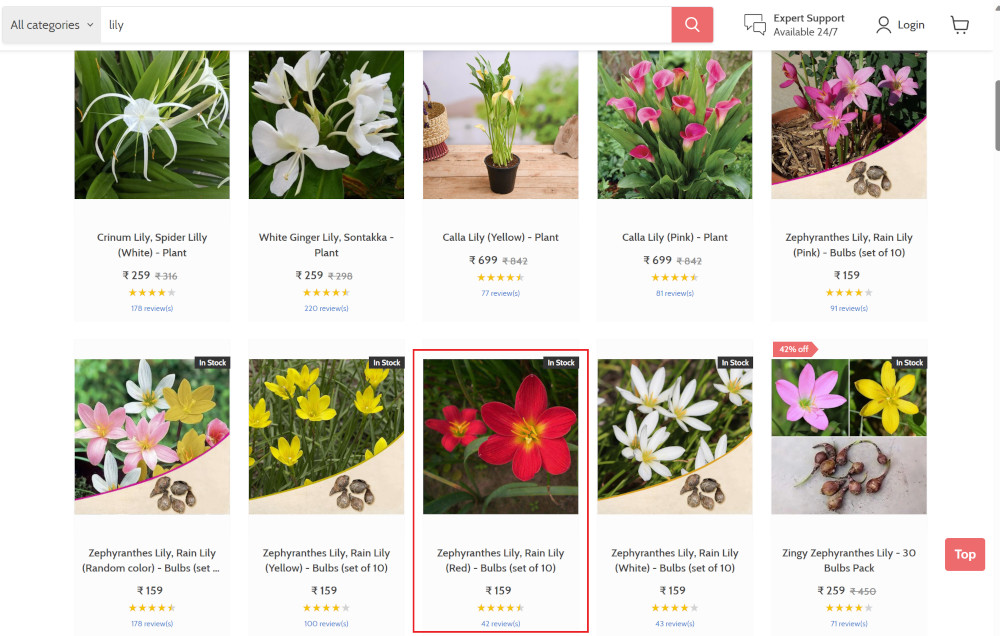
When the product is found on the screen, testRigor stops scrolling.
Step 4: Click on the product name to view the details:
click "Zephyranthes Lily, Rain Lily (Red)"
After the click, the product details are displayed on the screen as below, with the default Quantity as 1.
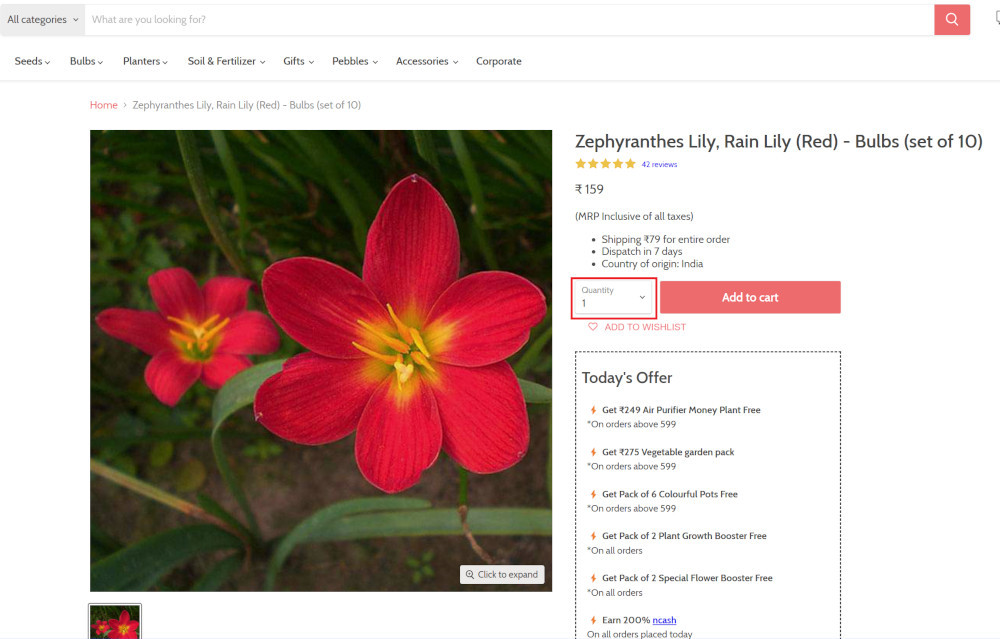
Step 5: Lets say, we want to change the Quantity to 3, so here we use the testRigor command to select from a list.
select "3" from "Quantity"
click "Add to cart"
The product is successfully added to the cart, and the “Added to your cart:” message is displayed on webpage.
Step 6: To assert that the message is successfully displayed, use a simple assertion command as below:
check that page contains "Added to your cart:"
Step 7: After this check, we will view the contents of the cart by clicking View cart as below:
click "View cart"
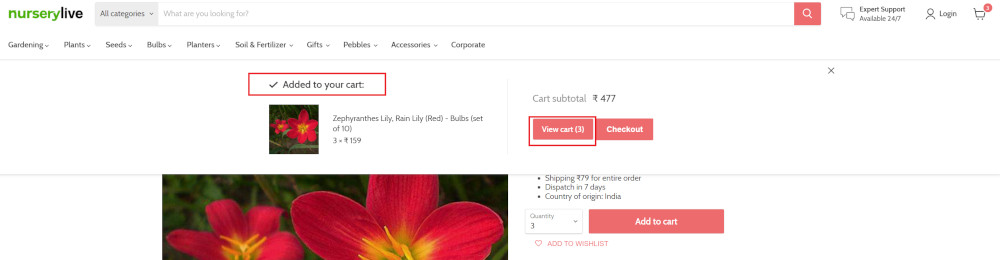
Step 8: Now we will again check that the product is present in the cart, under heading “Your cart” using the below assertion. With testRigor, it is really easy to specify the location of an element on the screen.
check that page contains "Zephyranthes Lily, Rain Lily (Red)" under "Your cart"
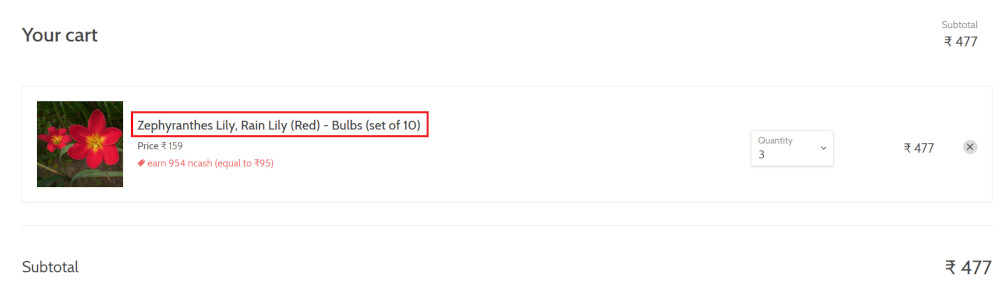
Complete Test Case
Here is how the complete test case will look in the testRigor app. The test steps are simple in plain English, enabling everyone in your team to write and execute them.
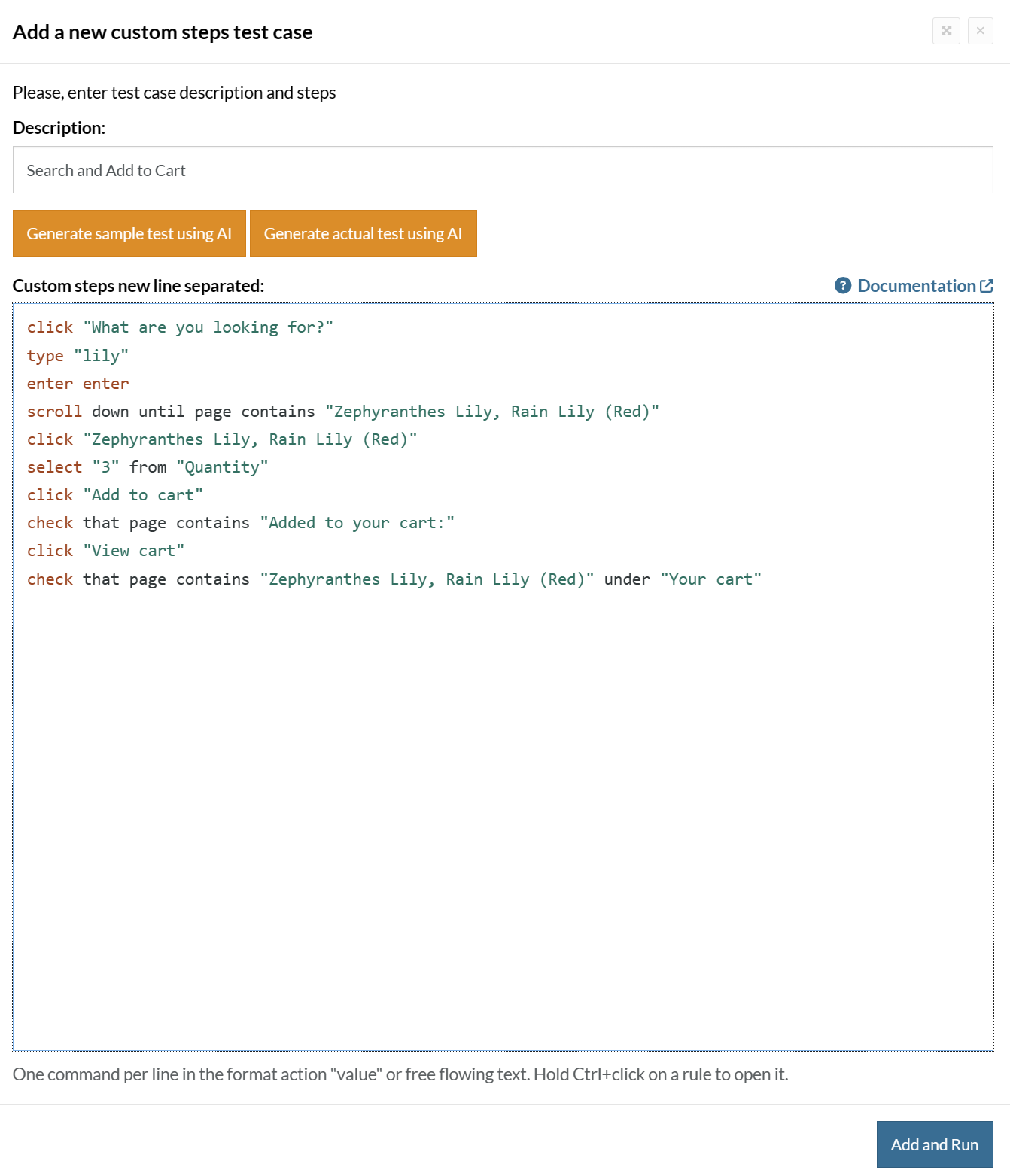
Click Add and Run.
Execution Results
Once the test is executed, you can view the execution details, such as execution status, time spent in execution, screenshots, error messages, logs, video recordings of the test execution, etc. In case of any failure, there are logs and error text that are available easily in a few clicks.
You can also download the complete execution with steps and screenshots in PDF or Word format through the View Execution option.
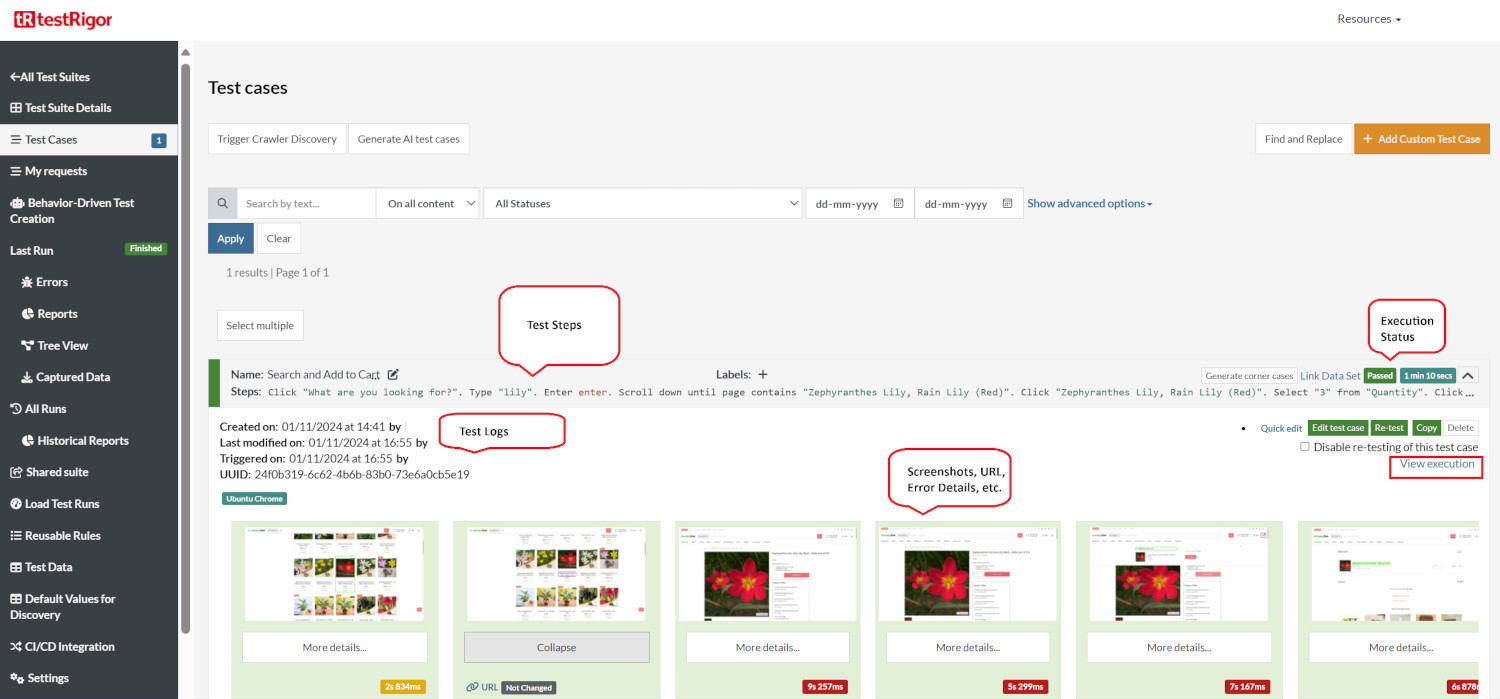
testRigor’s Capabilities
Apart from the simplistic test case design and execution, there are some advanced features that help you test your application using simple English commands.
- Reusable Rules (Subroutines): You can easily create functions for the test steps that you use repeatedly. You can use the Reusable Rules to create such functions and call them in test cases by simply writing their names. See the example of Reusable Rules.
- Global Variables and Data Sets: You can import data from external files or create your own global variables and data sets in testRigor to use them in data-driven testing.
- 2FA, QR Code, and Captcha Resolution: testRigor easily manages the 2FA, QR Code, and Captcha resolution through its simple English commands.
- Email, Phone Call, and SMS Testing: Use simple English commands to test the email, phone calls, and SMS. These commands are useful for validating 2FA scenarios, with OTPs and authentication codes being sent to email, phone calls, or via phone text.
- File Upload/ Download Testing: Execute the test steps involving file download or file upload without the requirement of any third-party software. You can also validate the contents of the files using testRigor’s simple English commands.
- Database Testing: Execute database queries and validate the results fetched.
testRigor enables you to test web, mobile (hybrid, native), API, and desktop apps with minimum effort and maintenance.
Additional Resources
- Access testRigor documentation to know about more useful capabilities
- Top testRigor’s features
- How to perform end-to-end testing
Conclusion
In conclusion, testing is an essential aspect of developing and maintaining high-quality Joomla applications. By incorporating various testing approaches, such as unit, integration, and end-to-end testing, you can ensure that your applications function correctly and meet the desired requirements. Utilizing tools like PHPUnit, Codeception, and testRigor, you can create comprehensive test suites, collaborate effectively, and reduce the time spent on manual testing. Embracing these testing practices not only leads to a more reliable and efficient development process but also guarantees a better user experience for the audience, ultimately contributing to the project's success.