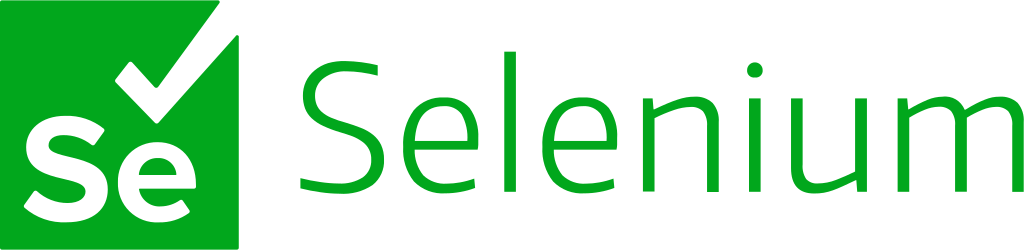
Stale Element Reference Exception in Selenium
org.openqa.selenium. StaleElementReferenceException
This is a comprehensive guide on how to deal with Selenium’s StaleElementReferenceException in Java. StaleElementReferenceException is thrown to indicate that the element that was referenced by a locator is no longer on the page or has become stale.
Main reasons for the StaleElementReferenceException exception
The most common reasons for the exception include:
- There was an action that led to a page change dynamically. Some events came in asynchronously, and the structure of the page changed. For example, new rows appeared dynamically in a table, or the UI framework updated the locators on the page during a refresh.
- There was an action that led the application to navigate to a different page.
- There was an error, and the page changed to indicate the error.
How to identify the exception
Before attempting to resolve the issue, it's crucial to understand the root cause. To do this, follow these steps:
- Repeat the steps to get to the page where you have the exception and monitor what is happening to the element you are trying to interact with.
- Check if the element is present or if the HTML rendering, the page, or any other element changes.
- Determine if there is an error that may cause the exception.
- Taking screenshots of the page might be helpful to assess what happened, as well as using browser developer tools to inspect the page structure and element attributes.
Solutions to handle StaleElementReferenceException
Here are some potential solutions to handle StaleElementReferenceException:
- Refresh the web page before accessing the web element.
- Use the try-catch block to handle the exception and attempt to locate the element again.
- Use explicit wait to ensure the element is present or refreshed before interacting with it.
- Use the Page Object Model (POM) design pattern or principles of Page Factory, which helps update the reference of the web element each time before any action is performed on it, thus reducing the occurrence of StaleElementReferenceException.
Code example:
import org.openqa.selenium.By; import org.openqa.selenium.StaleElementReferenceException; import org.openqa.selenium.By; import org.openqa.selenium.StaleElementReferenceException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class StaleElementExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://example.com"); By elementLocator = By.id("elementId"); WebElement element = findElementWithRetry(driver, elementLocator); // Perform actions on the element driver.quit(); } private static WebElement findElementWithRetry(WebDriver driver, By locator) { final int maxAttempts = 3; int attempts = 0; while (attempts < maxAttempts) { try { WebDriverWait wait = new WebDriverWait(driver, 10); WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(locator)); return element; } catch (StaleElementReferenceException e) { attempts++; if (attempts == maxAttempts) { throw e; } } } throw new RuntimeException("Element not found after maximum retries"); } }In this example, the
findElementWithRetry
method is used to handle the StaleElementReferenceException. This method attempts to locate the web element using the provided locator, and if it encounters a StaleElementReferenceException, it retries the search up to a maximum number of attempts (in this case, 3).
The method uses an explicit wait with WebDriverWait to ensure that the element is present on the page before trying to interact with it. If the element is successfully located, it is returned to the caller. If the maximum number of attempts is reached without success, the StaleElementReferenceException is thrown, indicating that the element could not be located.
This approach can help mitigate the occurrence of this exception when interacting with dynamic web pages. By combining retries, explicit waits, and potentially implementing the Page Object Model or Page Factory principles, you can build more robust and stable tests that can handle dynamic elements on a web page more effectively.
It's essential to understand that while these solutions can help manage StaleElementReferenceException, they might not entirely eliminate the issue in every scenario, particularly when dealing with highly dynamic web applications. It's important to adapt your testing strategy to the specific needs and characteristics of the application under test.
The main reason for StaleElementReferenceException
The primary problem that leads to this exception is Selenium's inability to deal with dynamic pages correctly. Because Selenium hooks up to a locator, which is a detail of implementation, not an end-user's level description. You can learn why Selenium is not an adequate solution for modern websites here. As an alternative solution, you can simply choose testRigor as your test automation tool and forget about exceptions like this forever - since testRigor takes care of locators for you.You're 15 Minutes Away From Automated Test Maintenance and Fewer Bugs in Production
Simply fill out your information and create your first test suite in seconds, with AI to help you do it easily and quickly.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
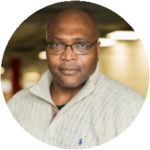
“We spent so much time on maintenance when using Selenium, and we spend nearly zero time with maintenance using testRigor.”
Keith Powe VP Of Engineering - IDT