Assertion Testing: Key Concepts and Techniques
|
Never argue, repeat your assertion – Robert Owen.
In real life, you may want to repeat your assertions. However, once is enough in software testing if used correctly at the right checkpoints. Assertions are the validations that are executed in the middle or end of the test cases to evaluate that the actual result is as expected. These checkpoints confirm that the application under test (AUT) is behaving as we expect it to.
These assertions are expressions that validate an application’s state or behavior, and the result is binary/boolean: True or False. While writing an automated test, assertions are included in the test script using various conditions to validate the outcome.
Let us learn about them in detail.
What is an Assertion?
The primary purpose of an assertion is to verify that the application is working correctly at the inserted checkpoints and, hence, overall. It is a way of telling the test script, “I expect the value of application state/behavior to be X at this point.“ Does my expectation match the actual result? Tell me in True or False.
When an assertion is executed, it evaluates a condition (usually a comparison between the actual and expected value). If the condition is true, the test continues to run. If the condition is false, the assertion throws an error, marking the test as failed.
Bake a Cake Example
Let us take an example of baking a chocolate cake and how we insert assertions in between and at the end of the process.
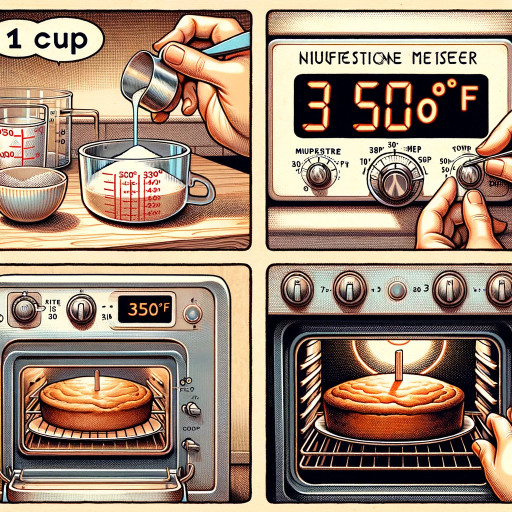
Step 1: Measuring Ingredients
Action: You measure 1 cup of sugar.
Assertion: You verify that the amount of sugar is correct.
Step 2: Preheating the Oven
Action: The recipe says to preheat the oven to 350°F.
Assertion: Verify that the oven thermometer has reached 350°F. In software testing, this is like checking that a condition (in this case, the oven temperature) is exactly as expected.
Step 3: Baking Time
Action: The recipe mentions baking the cake for 30 minutes.
Assertion: You set a timer and check the cake for 30 minutes. This is similar to a timeout assertion, ensuring the process (baking) doesn’t take longer than expected.
Step 4: Final Check – The Toothpick Test
Action: Insert a toothpick into the center to check it is baked.
Assertion: The cake is completely baked if the toothpick comes out clean. This is the same as an assertion where you check the final result (in software, this might be checking that a transaction is completed).
Types of Assertions in Software Testing
Below are the two types of assertions and a comparison table:
Hard Asserts are assertions where the test execution is aborted when the assert conditions are not met and the test case fails. If you want to continue test execution even if one of the assertions fails, use Soft Asserts.
You need to use TestNG with Selenium to set up assertions using org.testng.assert package. By default, the asserts in Selenium are Hard Asserts. To use Soft Asserts (also known as Verify), import the org.testng.asserts.SoftAssert package.
Hard Assert vs. Soft Assert
Insight | Hard Assert | Soft Assert |
---|---|---|
Definition | Execution is stopped immediately on test failure. | Execution is not halted, and all failures are reported at the end of the test execution. Verify is another name for soft assert. |
Behavior | When an assertion fails, the test is marked fail, and no subsequent code is executed. | When an assertion fails, the test execution does not stop, and the test continues. This allows multiple assertions to be checked in a single test run. |
Use Case | Useful when the next test steps depend on the success of the current step. That is, if the execution is required to be stopped during a critical bug, it is useful. | It is useful when running through all steps, and collecting failures for expansive test coverage is important. |
Example | In a login test, if asserting the presence of a login dialog fails, further steps (like entering credentials) are skipped. | In a form validation test, checks for all fields can continue even if one field fails validation, collecting all errors in one go. |
Syntax in Selenium | Assertion hardAssert = new Assertion(); | Assertion softAssert = new SoftAssert(); |
Reporting | Failures are reported immediately after the assertion fails. | All failures are reported together at the end of the test execution. |
Advantages | Quick failure detection, straightforward, and simple. | Efficient for scenarios where there are multiple validations and test results are required in one execution. |
Disadvantages | It does not provide complete test coverage if one of the test cases fails between test executions. Requires more test cases to identify all issues. | More complex to implement. |
TestNG Assert Methods
Syntax: Assert.Method(actual, expected) or Assert.Method(actual, expected, message)
Where
- actual is the actual value received on the application under test (AUT)
- expected is the expected (correct) value on the AUT
- message is the string error message to display in case of test failure
Below are few common TestNG assert methods:
Hard Asserts Syntax
- Assert.assertEquals(actual,expected);
- Assert.assertNotEquals(actual,expected,Message);
- Assert.assertTrue(condition);
- Assert.assertFalse(condition);
- Assert.assertNull(object);
- Assert.assertNotNull(object);
Soft Asserts Syntax
- softAssert.assertTrue(false);
- softAssert.assertEquals(1, 2);
- softAssert.assertAll();
Assert Code Examples
Below are TestNG code examples of hard and soft asserts:
Hard Assert Example
import org.testng.Assert; import org.testng.annotations.Test; public class HardAssertionExample { @Test public void testHardAssertion() { System.out.println("Test started"); Assert.assertEquals(2 + 2, 4, "Expected and actual values are not the same!"); System.out.println("Middle of the test"); Assert.assertTrue("hello".equals("hello"), "Strings are not equal"); System.out.println("Test ended"); } }
Soft Assert Example
import org.testng.annotations.Test; import org.testng.asserts.SoftAssert; public class SoftAssertionExample { @Test public void testSoftAssertion() { SoftAssert softAssert = new SoftAssert(); System.out.println("Test started"); softAssert.assertEquals(2 + 2, 5, "First assertion failed"); System.out.println("After first assertion"); softAssert.assertTrue("hello".equals("world"), "Second assertion failed"); System.out.println("After second assertion"); softAssert.assertAll(); // This will report all assertions System.out.println("Test ended"); } }
See here a Selenium test example to understand better.
A Simple and Quick Approach Using AI
Though you might have an idea about the assertions in software testing and how it works. It is a challenging job to implement it flawlessly. Selenium has its own disadvantages. Here are the 11 reasons not to use Selenium for software testing.
Organizations seek intelligent, fast, and simple solutions for implementing test automation. While the whole world is adopting AI, NLP, and the latest technology to advance, Selenium fails to serve its purpose efficiently. Here are the applications of generative AI in software testing.
Forget complex Selenium, TestNG, log4j, JUnit, integrations, and dependencies. Writing, managing, and maintaining all these scripts together so that they stay relevant and correct is a nightmare for engineers.
Intelligent generative AI-powered testing tools such as testRigor can solve your web, mobile, desktop, and API testing needs singlehandedly in plain English. Learning programming languages and all the syntaxes and dependencies is unnecessary. One testing tool is enough to handle everything in plain English test steps. testRigor works and thinks as a human would. It does not work on XPath and CSS locators instead, it uses UI text as visible on the screen as identifiers. These testRigor locators bring ultra-stability, and the built-in self-healing capabilities incorporate the UI changes in the test steps automatically to a great extent.
open url "https://www.gmail.com/" check that page title contains "Gmail" check that page contains "Email or phone" enter "[email protected]" into "Email or phone" check that page contains "Next" click "Next" check that page contains "Forgot password?" click "Forgot password?" check that page contains "Try another way" enter "testdemEX24$" into "Enter last password" check that page contains "Next" click "Next" check that page contains "Get a verification code"
Can it get simple and straight forward than this? No more confusion between soft assert and hard assert and complex test scripts. With this simplicity in test scripts, anyone in your team can write and execute test cases quickly and easily, irrespective of their technical proficiency. Work together and gain more test coverage with simplicity and stability.
Conclusion
testRigor allows you to write assertions in a natural, human-readable language. This approach is different from typical code-heavy assertion statements. If tools are available to aid you in your testing process, why not use them? testRigor helps you achieve excellent test coverage with minimum effort and test maintenance.
Your team can work together to write simple English test steps that are ultra-stable, unlike Selenium. Try testRigor today to experience its incredible features.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
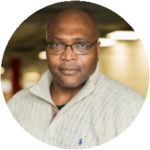