Selenium Test Example
|
What is Selenium?
Selenium is an open-source software tool used to create automated UI tests. It allows you to write scripts in a variety of programming languages to automate interactions with web pages. Selenium can simulate user interactions with a website, such as clicking links and filling out forms.
Selenium provides a range of tools and libraries for automating web browsers, including a WebDriver API, which allows you to control web browsers through programming languages such as Java, Python, and Ruby. It also provides a set of browser-specific drivers for Chrome, Firefox, Edge, Safari, and other popular web browsers.
Selenium WebDriver Overview
The two most popular Selenium offerings are WebDriver and IDE.
Selenium IDE (Integrated Development Environment) is a browser extension that provides a graphical user interface (GUI) for creating and running Selenium tests. It allows users to record, edit, and playback automated tests in a web browser without writing any code. And while it may sound compelling, it has a lot of limitations and typically isn’t advised for any complex scenarios. Therefore, most teams will find WebDriver a better option – which we will be using for writing a test in this article.
How to design a Selenium test
For designing Selenium test we need to familiarize with below:
Locators
- By CSS ID: find_element_by_id
- By CSS class name: find_element_by_class_name
- By name attribute: find_element_by_name
- By DOM structure or XPath: find_element_by_xpath
- By tag Name: find_element_by_tag_name()
- By link text: find_element_by_link_text
- By partial link text: find_element_by_partial_link_text
- By HTML tag name: find_element_by_tag_name
WebDriver Manager
WebDriverManager is an open-source Java library that helps download, setup, and supports maintenance of the drivers required by Selenium WebDriver (e.g., chromedriver, geckodriver, ms edgedriver, etc.) in a fully automated manner. With the use of WebDriver Manager we can avoid installing any device binaries manually. Additionally WebDriverManager helps check the version of the browser installed on the machine and downloads the proper driver binaries into the local cache ( ~/. cache/selenium by default) if not found. Refer here.
Assertions
Assertions are validations that certify if application behavior is working as expected. Asserts in Selenium help testers understand if tests have passed or failed. There are two types of Assertions: Hard Assertions and Soft Assertions.
Assert.assertEquals(actual,expected); Assert.assertNotEquals(actual,expected,Message); Assert.assertTrue(condition); Assert.assertFalse(condition); Assert.assertNull(object); Assert.assertNotNull(object);
softAssert.assertTrue(false); softAssert.assertEquals(1, 2); softAssert.assertAll();
Waits
When a page is loaded by the browser, the elements with which the user wants to interact may load at different time intervals. With this, it becomes difficult to identify the element and in case the element is not located, it will throw an exception. Wait times help resolve this problem.
- Explicit Wait: WebDriver is instructed to wait until a certain condition occurs or is met before proceeding with the execution of the code. It is a conditional wait. Explicit Wait usage is important in scenarios where there are certain elements that by default take more time to load.
- Implicit Wait: WebDriver is instructed to wait for a certain measure of time before throwing an exception. It is a global wait and once this time is set, WebDriver will wait for the element before the exception occurs.
- Fluent Wait: In Fluent Wait, the WebDriver is instructed to wait for a certain condition (web element) to become visible. It also defines how frequently WebDriver will check if the condition appears before throwing the exception.
- Hard Wait: this wait is implemented using Thread.sleep(), and is typically not recommended to use. It adds unnecessary execution time to an automation suite. If your test suite is small, is not run regularly, or you are not under any time restraints – then implicit waits might be a better choice.
Programming languages you can use
Selenium is used to create test scripts using different programming languages such as C#, Groovy, Java, Perl, PHP, Python, Ruby and Scala.
Why is Java widely used with Selenium?
Selenium is one of the most commonly used tools for test automation, and Java is the most popular programming language. So both together make a perfect combination to consider for an example.
Before starting to write tests with Java and Selenium, it is mandatory to understand core Java concepts. It is also important to understand what OOP is and how relevant is its use in Selenium. To understand the core Java concepts, refer here.
What is OOP (OOPs)?
OOP stands for Object-Oriented Programming, which is a programming paradigm that uses objects to represent and interact with data and behavior in a program. In OOP, a program is divided into objects, which have attributes and methods that can be used to represent and manipulate data.
In the context of creating tests, OOP is important because it helps to create maintainable, scalable, and reusable code. OOP provides a modular and organized approach to software development, which allows for code that is easier to understand and maintain.
By using OOP concepts like inheritance, encapsulation, and polymorphism, test automation engineers can create a hierarchy of test cases that can be extended and modified easily as the application evolves over time. OOP also allows for the creation of reusable components, which can be used across multiple tests, reducing the amount of code that needs to be written and maintained.
Selenium Test Example
Test case
- Launch Chrome browser using WebDriver Manager.
- Open Gmail Login page.
- Enter email/phone and click Forgot Password.
- Close the web browser.
Pre-requisites – installation and setup details
- Install Java 1.8 or higher. Refer here.
- Install IntelliJ IDE, Community Edition. Refer here.
- Download Selenium jars and Dependencies. Refer here.As an example you can install 3.9 version then you need to install 2 files as highlighted below:
- Download Chrome driver per Google Chrome version as highlighted below. Refer here.
- Download Selenium Java and WebDriver manager dependencies:
- Launch IntelliJ. Create a new Java Project.
- Add Selenium jars and dependencies. Goto File > Project Structure > Modules > Dependencies > Click on ‘+’ Sign > Select for JARs or directories > Add all the selenium. jar files from the directory and subdirectory /lib
Project Structure
Inside the src folder > test , create a Java class file GoogleAccountRecoveryTest.java. Sample code looks like below:
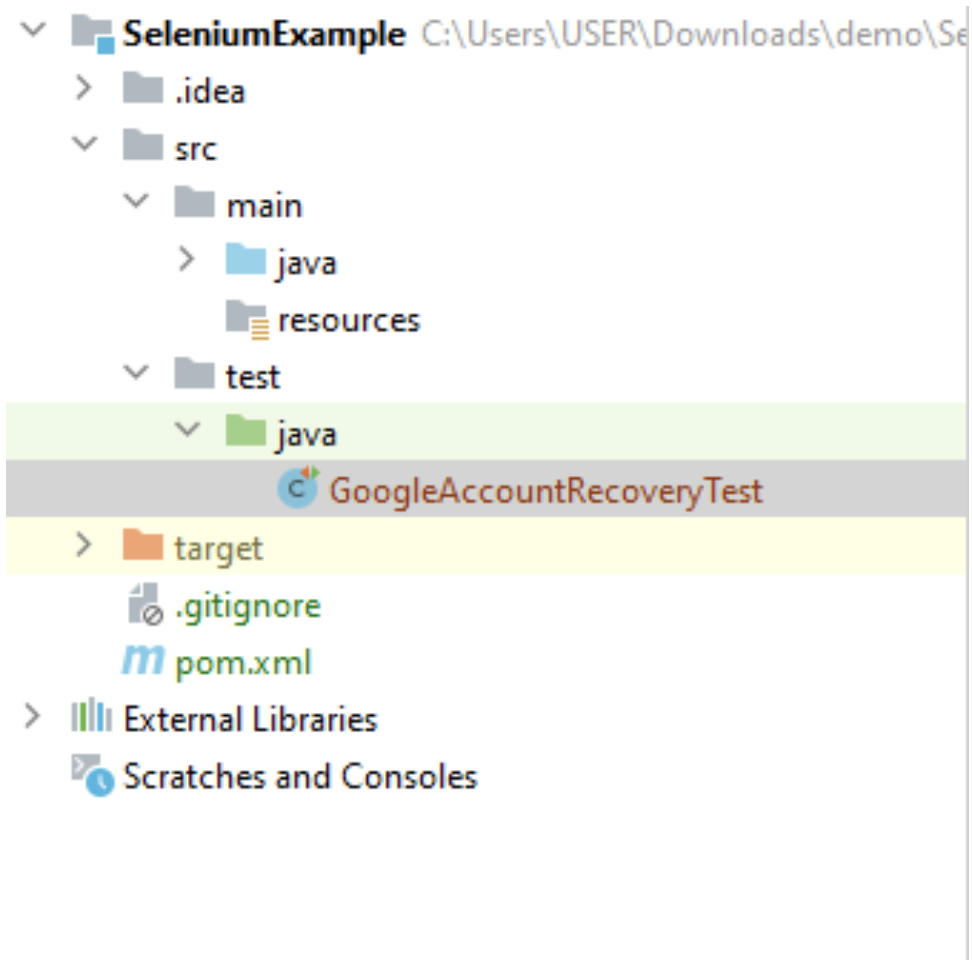
We are using TestNG in this example. Refer here.
import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.Assert; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; import java.util.concurrent.TimeUnit; public class GoogleAccountRecoveryTest { public static WebDriver driver = null; @BeforeTest public void setUp() throws Exception { WebDriverManager.chromedriver().setup(); driver = new ChromeDriver(); } @Test public void firstTestCase() { try { System.out.println("Launch Gmail"); driver.get("https://www.gmail.com/"); Assert.assertEquals("Gmail", driver.getTitle()); System.out.println("Enter email"); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); WebElement email_phone = driver.findElement(By.xpath("//input[@id='identifierId']")); Assert.assertEquals(true, email_phone.isDisplayed()); email_phone.sendKeys("[email protected]"); System.out.println("Click Next button"); WebElement nextBtn = driver.findElement(By.id("identifierNext")); Assert.assertEquals(true, nextBtn.isDisplayed()); nextBtn.click(); System.out.println("Click Forgot Password link"); WebDriverWait wait = new WebDriverWait(driver, 20); WebElement forget_password = driver.findElement(By.xpath("//span[text()='Forgot password?']")); wait.until(ExpectedConditions.visibilityOf(forget_password)); Assert.assertEquals(true, forget_password.isDisplayed()); forget_password.click(); System.out.println("Enter last known password"); WebElement tryLink = driver.findElement(By.xpath("//span[text()='Try another way']")); wait.until(ExpectedConditions.visibilityOf(tryLink)); Assert.assertEquals(true, tryLink.isDisplayed()); WebElement lastPasswd = driver.findElement(By.name("password")); lastPasswd.sendKeys("testdemEX24$"); System.out.println("Click Next"); WebElement pwd_next = driver.findElement(By.xpath("//*[@id='passwordNext']/div/button/span")); Assert.assertEquals(true, pwd_next.isDisplayed()); pwd_next.click(); System.out.println("Check Get a verification code shown"); WebElement get_ver_code = driver.findElement(By.xpath("//*[@id='view_container']/div/div/div[2]/div/div[1]/div/form" + "/span/section/header/div/h2/span")); wait.until(ExpectedConditions.visibilityOf(get_ver_code)); Assert.assertEquals(true, get_ver_code.isDisplayed()); } catch (Exception e) { } } @AfterTest public void closeBrowser() { driver.close(); System.out.println("The driver has been closed."); } }
Code Walkthrough
import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.Assert; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; import java.util.concurrent.TimeUnit;
@BeforeTest
is an annotation provided by testNG. This will be executed before the first @Test
annotated method. Here we use the setup method to initialize WebDriverManager
download and setup the chromedriver.@BeforeTest public void setUp() throws Exception { WebDriverManager.chromedriver().setup(); driver=new ChromeDriver(); }
@Test
is an annotation in TestNG that is used to identify a test method. Here we also use the Explicit and Implicit waits.
@AfterTest
is an annotation provided by TestNG which will be run after all the test methods in the current class have been executed. Here we are closing the browser instance.@AfterTest public void closeBrowser() { driver.close(); System.out.println("The driver has been closed."); }
SLF4J: No SLF4J providers were found. SLF4J: Defaulting to no-operation (NOP) logger implementation SLF4J: See https://www.slf4j.org/codes.html#noProviders for further details. Starting ChromeDriver 110.0.5481.77 (65ed616c6e8ee3fe0ad64fe83796c020644d42af-refs/branch-heads/5481@{#839}) on port 44803 Only local connections are allowed. Please see https://chromedriver.chromium.org/security-considerations for suggestions on keeping ChromeDriver safe. ChromeDriver was started successfully. [1677289460.573][WARNING]: virtual void DevToolsClientImpl::AddListener(DevToolsEventListener *) subscribing a listener to the already connected DevToolsClient. Connection notification will not arrive. Feb 25, 2023 7:14:20 AM org.openqa.selenium.remote.ProtocolHandshake createSession INFO: Detected dialect: W3C Launch Chrome browser Enter email Click Next button Click Forgot Password Link Enter last Password Click Next Check Get a verification code shown The driver has been closed. =============================================== Default Suite Total tests run: 1, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
testRigor with example
You can write Selenium code as described above, or you can instead create the same tests in testRigor using plain English commands. testRigor is a cloud-based AI-driven tool that makes the entire testing process an order of magnitude more straightforward. Let’s automate the same use case "GoogleAccountRecoveryTest"
test using testRigor code.
open url "https://www.gmail.com/" check that page title contains "Gmail" check that page contains "Email or phone" enter "[email protected]" into "Email or phone" check that page contains "Next" click "Next" check that page contains "Forgot password?" click "Forgot password?" check that page contains "Try another way" enter "testdemEX24$" into "Enter last password" check that page contains "Next" click "Next" check that page contains "Get a verification code"
No code, no dependencies to think about, no wait times, no element locators (testRigor takes care of everything for you). The result? Cleaner and easier to understand tests that are ultra-stable. Feel free to check us out and experience yourself!