Element Not Interactable Exception in Selenium: How To Resolve?
|
Element Not Interactable Exception in Selenium
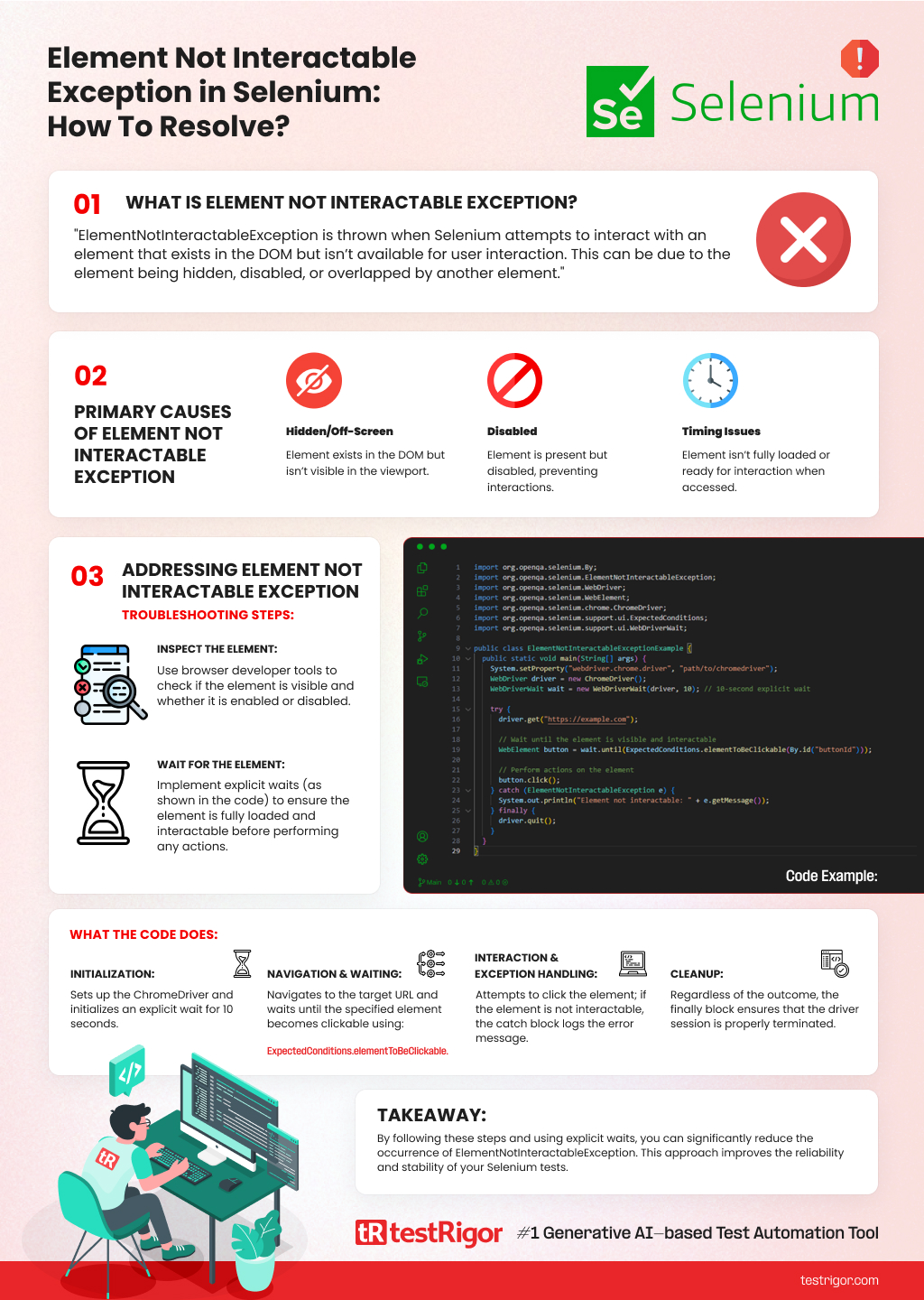
org.openqa.selenium.ElementNotInteractableException
: Understanding and Handling Element Not Interactable Exception in Selenium with Java
Selenium WebDriver is a widely used tool for browser automation across various programming languages, including Java. One common exception developers may encounter when working with Selenium is Element Not Interactable Exception. This article provides a comprehensive understanding of this common exception, its causes, and how to handle it effectively. Additionally, it includes a Java code example to demonstrate handling the exception.
What is Element Not Interactable Exception?
Element Not Interactable Exception is an exception thrown by Selenium WebDriver when an element is present in the DOM, but it is not interactable, meaning that the user cannot perform any action on it, such as clicking or sending keys. This exception indicates that the element you are trying to interact with is either hidden, disabled, or in a state that prevents user interaction.
Primary Causes of Element Not Interactable Exception
- Element visibility: The target element might be hidden or covered by another element, making it non-interactable.
- Element state: The element could be in a disabled state, preventing user interaction. If that is the case, it means that the element is designed to be non-interactable to mimic user interactions.
- Timing issues: The element may be temporarily non-interactable, particularly in dynamic web applications with AJAX and JavaScript. In this case, Selenium might be attempting to interact with the element before it becomes interactable, resulting in the exception.
Addressing Element Not Interactable Exception
- Inspect the element: Check the element’s visibility and state (enabled or disabled) in the browser’s developer tools.
- Wait for the element to become interactable: Implement explicit (recommended) or implicit waits to provide sufficient time for the element to become interactable before attempting to perform actions on it.
Code example:
import org.openqa.selenium.By; import org.openqa.selenium.ElementNotInteractableException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class ElementNotInteractableExceptionExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); WebDriverWait wait = new WebDriverWait(driver, 10); // 10-second explicit wait try { driver.get("https://example.com"); // Wait until the element is visible and interactable WebElement button = wait.until(ExpectedConditions.elementToBeClickable(By.id("buttonId"))); // Perform actions on the element button.click(); } catch (ElementNotInteractableException e) { System.out.println("Element not interactable: " + e.getMessage()); } finally { driver.quit(); } } }
Here, the code sample demonstrates handling Element Not Interactable Exception in a Selenium Java test. It imports required classes, initializes WebDriver and WebDriverWait, and uses a try-catch block to navigate to a webpage, wait for an element to become visible and interactable, and perform actions on the element. If Element Not Interactable Exception is thrown, the error message is printed, and the browser and WebDriver session are terminated in the finally block. This example showcases handling Element Not Interactable Exception and waiting for elements to become interactable before interacting with them in a Selenium test.
By following the recommended practices and using the provided code example as a guide, you can significantly reduce the occurrence of Element Not Interactable Exception and improve the stability of their automated tests.
Why Element Not Interactable Exception is So Common
You can read why Selenium is not an adequate solution for modern websites here. Smart tools such as testRigor don’t depend on any locators, and as a QA professional, you can forget about the underlying infrastructure – and focus on your test having the best assertions and testing for the right things. You can simply switch to testRigor to forever escape element exception issues like the one described in this article.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
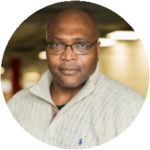