How to Resolve TimeoutException in Selenium
|
Selenium is one of the traditional web automation frameworks that helps the testing team create and execute tests in a browser environment. While executing the tests, it is common to encounter timeout exceptions. Here, we are going into more detail about Selenium time-out exceptions, how they happen, and how we can prevent them.
Different Timeouts in Selenium
In Selenium, we encounter different types of timeout-related exceptions while executing the test cases. The common timeout exceptions that we face are:
- TimeoutException: This exception occurs when an operation takes longer than the implicit or explicit wait time provided to complete. This general timeout error happens when Selenium waits for page loads, element interactions, or other actions to be completed.
- NoSuchElementException: This exception happens when the Selenium WebDriver cannot find the specified web element in the mentioned timeout. This timeout indicates that the element is absent in the DOM within the given time limit. It can be either the loading issue or the element not being present.
- ElementNotVisibleException: This exception is thrown when an element is present in the DOM but not visible on the webpage within a specific time limit. It can occur when we try to interact with hidden elements or lazy loading pages.
- StaleElementReferenceException: This exception is thrown when the previously allocated and stored element becomes no longer valid or stale due to the changes in DOM. The main cause may be a page refresh or dynamic content loading.
- ElementNotInteractableException: This error occurs when an element is present in the DOM and visible but unable to interact. It can happen when the element is disabled or overlapped by other elements.
Root Cause Analysis for Selenium TimeoutException
There are various reasons why Selenium timeout exceptions occur. The root cause is when the web elements or conditions fail to meet the expected conditions mentioned in the test script within the time limit.
Let’s go through a few common reasons for triggering these exceptions.
- Slow Loading: Sometimes, web pages take a longer time to load, so if the script tries to interact with the element before the page is ready, then the timeout error can occur.
- Network Latency: Network delays or issues in internet connection can cause delays in fetching web elements, leading to timeout exceptions.
- AJAX and JavaScripts: Modern web applications use asynchronous JavaScript to load the contents dynamically. If the test scripts are not waiting for the scripts to load, then exceptions will be thrown.
- Page Redirects: A timeout exception is thrown if the page gets redirected and doesn’t wait for the redirect to complete and perform any action or search for an element.
- Unhandled Popups or Alerts: If the automation script encounters unexpected popups or alerts that are not scripted to handle, then an exception is thrown. Read: How to Automate Pop-Up Alert Testing?
- Insufficient Wait Strategies: Using incorrect wait methods, like not using fluent waits or explicit waits, can lead to exceptions.
Debugging Selenium Timeout Errors
Debugging the timeout errors is essential for identifying and resolving the issues in the test automation script. Let’s review some debugging methods we can use.
- Review Error Messages: Selenium usually provides detailed and informative error messages when a timeout error occurs. By understanding the error messages, we can identify the reason that triggered the timeout.
- Logging: Implementing detailed logging in the script will help understand the exception’s root cause. This can be done by changing the Selenium logs or installing third-party loggers.
- Using Try-Catch Blocks: Using Try-Catch blocks, we can handle timeout exceptions gracefully. So, with this method, we can prevent the scripts from failing abruptly and help execute other actions or log the error.
- Increase Timeouts: If the script faces timeouts due to slow loading, we can increase the implicit wait time or configure explicit waits.
- Debugging Statements: We can add print statements at different points in the scripts to track progress and understand where failures happen.
- Validate Selectors: It’s always good to double-check the selectors and ensure we are not using any weak XPath or selector. The selectors should be accurate and unique. Otherwise, it will lead to timeouts.
Tips to Avoid Selenium TimeoutException
-
Explicit Waits: They are mainly used while checking conditions or wait scenarios. Explicit wait is how long the script must wait to meet a particular condition. This is better than implicit wait since the wait can be closed as the element becomes visible or present, reducing timeouts.
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC element = WebDriverWait(driver, 10). until(EC.presence_of_element_located((By.ID, "elementID")))
Key Points:- Explicit waits should be used for elements that take time to load or become ‘interactable’.
- The ExpectedConditions class provides predefined conditions, such as elementToBeClickable, visibilityOf, presenceOfElementLocated, etc.
-
Retry Mechanisms: They perform the same action multiple times if the particular scenario or test case fails. This will be helpful when the initial failure is due to any network latency or improper page refresh and reduces a lot of exceptions.
from selenium.common.exceptions import TimeoutException max_attempts = 3 for _ in range(max_attempts): try: element = WebDriverWait(driver,5).until( EC.presence_of_element_located((By.ID, "elementID")) ) # Perform actions on the element break # Exit the loop if successful except TimeoutException: continue # Retry if a timeout occurs
-
Handle Stale Element Reference: If the DOM changes after an element is located, a stale element reference exception may occur. To resolve this, re-fetch the element before interacting with it.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.id("dynamic-element"))); element = driver.findElement(By.id("dynamic-element")); // Re-fetch the element element.click();
- Page Load Strategy: In Selenium, we can set the page load strategy as “normal”, “eager”, or “none”, based on the application’s behavior. This will help to control the Selenium waits.
-
Use Fluent Wait: Fluent wait provides more control over the waiting mechanism by allowing you to define the polling frequency and ignore specific exceptions.
Wait<WebDriver> fluentWait = new FluentWait<>(driver) .withTimeout(Duration.ofSeconds(30)) .pollingEvery(Duration.ofSeconds(2)) .ignoring(NoSuchElementException.class); WebElement element = fluentWait.until(driver -> driver.findElement(By.id("example-id"))); element.click();
-
Failure Handling: Implementing an error-handling mechanism will handle the timeout exceptions. The Try-Catch method we mentioned above will be the best option.
from selenium.common.exceptions import TimeoutException try: element = WebDriverWait(driver,10).until( EC.presence_of_element_located((By.ID, "elementID"))) # Perform actions on the element except TimeoutException: # Handle the timeout error here (e.g.log the error or take alternative actions)
Handling Timeout Errors Efficiently
Selenium is a traditional automation tool that came to market when test automation was evolving. Though initially, many companies used that, slowly, they started moving out from Selenium. Here are the 11 reasons why not to use Selenium for automation testing.
Selenium is considered a popular programming test automation tool, but more than the advantages, there are many disadvantages to using the Selenium framework. Because of all these reasons, you may want to consider a shift from Selenium to modern and intelligent test automation tools like testRigor, which are powered by generative AI.
Let’s see how testRigor tackles timeout errors efficiently and quickly.
-
Custom Element Selector: testRigor doesn’t rely on unstable locators like XPath or CSS selectors; it utilizes AI to identify elements. Therefore, you only need to provide the name as it is visible on UI or its position as below:
click "cart" click on button "Delete" below "Section Name" to the right of "label"
- Inbuilt Waits: You don’t need to set any implicit wait for page loads with testRigor; its intelligent features manage that automatically for you. It captures whether the page is fully loaded and only then executes the next test step. This approach helps avoid most timeout errors related to page loads easily without letting the test execution stop or fail. Read: How to add waits using testRigor?
- Parallel Execution: With testRigor, users can execute test scripts concurrently across different browsers or platforms, minimizing timeout errors.
Read here how testRigor is different from Selenium.
End Notes
Test automation frameworks are mainly used to ease the job of testing. So, the effort to maintain the test framework or scripts should be minimal, which is missing in Selenium.
testRigor fills the gap here with its advanced features. It eliminates the maintenance effort, and with generative AI, codeless test creation in plain English, test recorder, and live mode testing, it also reduces the script creation effort. Everyone in your team can contribute to test creation and execution now, irrespective of their technical expertise.
To learn more about testRigor, you can sign up for a free trial.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
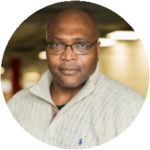