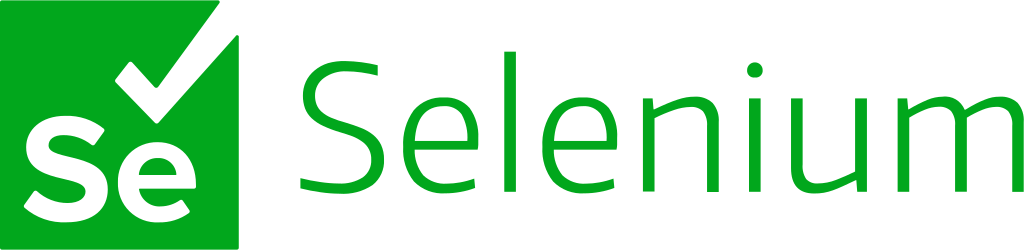
NoSuchElementException in Selenium
org.openqa.selenium.NoSuchElementException
Selenium WebDriver is a widely used tool for automating browsers through various programming languages, including Java. One common exception that engineers encounter when working with Selenium is NoSuchElementException. This article provides a comprehensive understanding of NoSuchElementException, its causes, and how to handle it effectively. Additionally, it includes a Java code example to demonstrate handling the exception.
What is NoSuchElementException?
NoSuchElementException is thrown by findElement()
method in Selenium WebDriver when the desired element cannot be located using the specified locator (such as an ID, name, class, CSS selector, or XPath). This exception indicates that the web element you are trying to interact with is not present on the web page at the time the method is executed.
Primary Causes of NoSuchElementException
- Changes in HTML/XML structure: The target element now has a different locator than it did previously. This situation is especially common in actively developed projects, where UI elements and their locators might change frequently.
- Element no longer present on the page/screen: The element was previously on the current page but can no longer be located. This issue may stem from the current step or a prior step, leading to a mismatch between the expected and actual page states.
- Timing issues: The element might not yet be loaded on the page, especially in dynamic web applications with AJAX and JavaScript. In this case, Selenium might be attempting to find the element before it has been rendered, resulting in NoSuchElementException.
How to deal with the NoSuchElementException exception
To troubleshoot NoSuchElementException effectively, follow these steps:
- Verify the locator: As a first step, you ought to find out what kind of issue you are dealing with. Is the element still there and can't be found, or is it no longer present on the page? To do this, you can setup Selenium to not to close the browser after the test failure, and run the test. Once you get the exception, you can inspect the page that is open in the browser. Double-check that the locator being used is correct and up-to-date. Inspect the HTML source code to confirm that the locator uniquely identifies the target element on the page.
- Wait for the element: Implement implicit (not recommended unless necessary) or explicit (recommended) waits to give the element time to load before attempting to interact with it. This approach helps to ensure that Selenium does not attempt to find the element before it has been rendered on the page.
Code example:
import org.openqa.selenium.By; import org.openqa.selenium.NoSuchElementException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class NoSuchElementExceptionExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); WebDriverWait wait = new WebDriverWait(driver, 10); // 10-second explicit wait try { driver.get("https://example.com"); // Wait until the element is present and visible WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId"))); // Perform actions on the element element.click(); } catch (NoSuchElementException e) { System.out.println("Element not found: " + e.getMessage()); } finally { driver.quit(); } } }
Here, the example demonstrates handling NoSuchElementException in a Selenium WebDriver test. It imports necessary classes, initializes WebDriver and WebDriverWait, and uses a try-catch block to navigate to a webpage, wait for an element to be visible, and perform actions on the element. If NoSuchElementException is thrown, the error message is printed, and the browser and WebDriver session are terminated in the finally block.
By using this code example as a guide, you can implement error handling for NoSuchElementException in your Selenium tests, while ensuring that your automation script waits for the elements to be visible before interacting with them.
The main reason for NoSuchElementException
The main problem that leads to this exception is that in Selenium, you hook up to details of implementation. Because Selenium hooks up to a locator, which is a detail of implementation, not end-user's level description. You can learn why Selenium is not an adequate solution for modern websites here.
To give you a different perspective, testRigor users will never face exception errors like the one discussed in this article - since testRigor's engine scans the page for you, identifies all possible locators for each element, and machine learning algorithms fine-tune wait times as needed. You will never have to worry about element locators with testRigor.