Overcoming ElementNotVisibleException: Best Practices for Selenium WebDriver
|
You might have encountered various exceptions when executing test automation scripts written in Selenium. These Selenium exceptions are a part of the error-handling mechanism in programming, where the normal flow of a program is disrupted, and the control is transferred to a particular section of code known as an exception handler.
Of the many exceptions, the ElementNotVisibleException exception occurs quite frequently. Irrespective of your proficiency in test script creation, these exceptions can happen. When they do, you need to know how to go about them. Read on to learn how to go about the ElementNotVisibleException exception.
What is ElementNotVisibleException?
In Selenium, the ElementNotVisibleException exception is thrown when an automation script attempts to interact with a web element that is currently not visible on the page, meaning that a real user wouldn’t be able to interact with it.
This exception is in the results – org.openqa.selenium.ElementNotVisibleException
This visibility is determined from the perspective of a user; that is, the element must be not only present in the Document Object Model (DOM) but also visible in the browser viewport.
Causes for ElementNotVisibleException
Here are some of the most common causes that lead to ElementNotVisibleException.
Issues with the XPath of elements
You must be familiar with the concept of XPaths. They are meant to be a way to identify web elements. The XPath you’ve written may have issues like:
- Incorrect XPath: This is straightforward. The XPath you wrote is invalid in the context of the DOM values. Hence, the element is not visible.
- Duplicate XPath: The XPath you’ve written may have other duplicate values; hence, when Selenium looks for the element, it cannot find just 1 match.
For example, suppose you have three buttons at the same level of the DOM. In that case, you need to mention attributes that distinguish which button you are referring to so Selenium can identify the element correctly.
Inaccessible elements
You may have provided the correct XPath to the element, but due to certain conditions, that element is inaccessible to Selenium. This could be possible due to the following conditions:
- Hidden elements: Sometimes, elements are present in the DOM but are hidden due to CSS styles.
- Obstructed elements: Other elements, like pop-ups, menus, or toast messages, might cover the desired element.
- Dynamic elements: Websites with dynamic content can have elements that become visible only under certain conditions or after specific actions.
- Embedded elements: The element might be inside a frame or iframe and not directly accessible from the main page’s DOM.
Timing issues
Most modern websites use dynamic loading of elements to enhance user experience. This means that elements load based on different conditions or backend calls after the initial page load has happened. This behavior is common in modern web applications that use AJAX (Asynchronous JavaScript and XML) and JavaScript. Due to its asynchronous nature, there is a high chance of the ElementNotVisibleException exception occurring. Here are some scenarios where you might see this.
- Element yet to appear: If a script attempts to interact with an element that is supposed to be loaded dynamically but hasn’t yet appeared, Selenium will throw an ElementNotVisibleException. This can happen if the script runs faster than the web application’s dynamic loading process.
- Incorrect timing: When a test script does not account for the time it takes for elements to become visible after being dynamically loaded, it may interact with them before they are visible on the UI.
- Intermittent visibility: In some cases, elements may appear and then quickly change state or be replaced by other elements as part of the dynamic loading process.
Resolution for ElementNotVisibleException
There are ways to ensure this exception does not occur by coding checks and balances in place.
Writing correct XPaths
This may seem like a no-brainer, but it is often the problem. When writing XPaths, try to create unique ones so that Selenium can quickly identify the required web element. Here is your pocket guide about how to write correct XPaths.
driver.findElements()
.driver.findElements(By.xpath("element_locator")).get(3).click();
Checking element state
Before interacting with an element, check that it is not only present in the DOM but also visible and interactable.
Explicit wait
To prevent ElementNotVisibleException in Selenium WebDriver, you can use WebDriverWait along with ExpectedConditions to ensure an element is visible before interacting with it.
WebDriverWait wait = new WebDriverWait(driver, 40); WebElement element=wait.until(ExpectedConditions.elementToBeClickable(driver.findElement(element_locator))); element.click();
Using JavaScript DOM API
The JavaScript DOM API allows direct interaction with the webpage’s HTML and DOM, providing more flexibility and control, especially in complex or highly dynamic web environments. You can manipulate web elements and also check their states, something that may not have been possible with Selenium’s standard methods.
WebElement Element = driver.findElement(By.id("button_1")); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("arguments[0].scrollIntoView();",Element );
Javascript can also be used to tackle the following:
- Polling for elements: JavaScript can be used to poll for the presence or state of elements in a way that’s more tailored to the specific application’s behavior than Selenium’s built-in waits.
- Handling AJAX calls: You can use JavaScript to check for any active AJAX calls (e.g., checking the state of XMLHttpRequest objects) to ensure that all dynamic content has been fully loaded.
A Sample Selenium Java Test
Here, the code sample demonstrates handling ElementNotVisibleException in a Selenium Java test. It imports required classes, initializes WebDriver and WebDriverWait, and uses a try-catch block to navigate to a webpage, wait for an element to become visible, and perform actions on the element. If ElementNotVisibleException is thrown, the error message is printed, and the browser and WebDriver session are terminated in the finally block.
To troubleshoot ElementNotVisibleException effectively, follow these steps:
- Inspect the element: Check the element’s visibility and state (collapsed or expanded) in the browser’s developer tools.
- Wait for the element to become visible: Implement explicit (recommended) or implicit waits to provide sufficient time for the element to become visible before attempting to perform actions on it.
import org.openqa.selenium.By; import org.openqa.selenium.ElementNotVisibleException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class ElementNotVisibleExceptionExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); WebDriverWait wait = new WebDriverWait(driver, 10); // 10-second explicit wait try { driver.get("https://example.com"); // Wait until the element is visible WebElement button = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("buttonId"))); // Perform actions on the element button.click(); } catch (ElementNotVisibleException e) { System.out.println("Element not visible: " + e.getMessage()); } finally { driver.quit(); } } }
Moving beyond the XPath hunts
The ElementNotVisibleException is a common problem that plagues automation testers using Selenium, making them wonder, “Why can’t it do this by itself!”
Luckily, this wish has become a reality, thanks to AI-driven tools like testRigor.
You no longer have to fret over XPaths as testRigor will be the detective and find the web element for you. All you have to do is tell it which element to look for. Here’s an example. Let’s say you want to click on the search button at the top of the web page. The testRigor command for this will be:
click on "Search" enter "macbook" into "Search"
That’s it!
Don’t worry. There are no hidden clauses requiring you to document all web elements in a separate file. Using object recognition and NLP, it can identify the web element you are referring to without needing you to mention the implementation details of said elements.
testRigor uses NLP to let you write test scripts in plain English language, thus making it easier for anyone to automate. The power of generative AI makes testRigor perfect for end-to-end testing of simple and complex web applications across browsers and platforms.
However, if you are comfortable with XPaths and other implementation details of web elements, then you can use the same. Here is an example.
enter "macbook" into "nav-input nav-progressive-attribute"
Besides being able to skip the hassle of working with the implementation details of web elements, testRigor has a lot more to offer. Here are testRigor’s top features.
Avail of the free version to try out the features for yourself.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
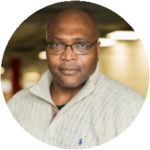