InvalidSelectorException: Understanding and Handling the Exception in Selenium
|
To identify web elements on the page and then interact with them, Selenium uses locators or selectors. They are the string expressions that tell Selenium which HTML element it should perform some action such as click, type, drag, etc. They can be of multiple types. Let us know in some detail.
Selectors in Selenium
-
ID Selector: The most common type of selector uses the ‘id’ attribute of an element that is unique.Example:
WebElement element = driver.findElement(By.id("elementId"));
-
Name Selector: Uses the ‘name’ attribute of a web element to identify it uniquely.Example:
WebElement element = driver.findElement(By.name("elementName"));
-
Class Name Selector: Selects elements using their ‘class’ attribute and is useful when the class name is unique. However, the class name is often reused across elements.Example:
WebElement element = driver.findElement(By.className("className"));
-
Tag Name Selector: Locates an element using its HTML tag and usually returns multiple elements sharing the same HTML tag.Example:
List<WebElement> elements = driver.findElements(By.tagName("input"));
-
CSS Selector: It handles complex CSS query expressions to identify elements uniquely.Example:
WebElement element = driver.findElement(By.cssSelector("#id .class"));
-
XPath Selector: It navigates through a webpage and identifies elements in an XML document using complex hierarchical structures.Example:
WebElement element = driver.findElement(By.xpath("//div[@id='id']/span[@class='class']"));
-
Link Text and Partial Link Text Selector: They are helpful specifically for anchor tags (<a> elements). By.linkText looks for exact matches of the link text, and By.partialLinkText partially matches elements containing the specified text.Example:
WebElement link = driver.findElement(By.linkText("Click Here"));
WebElement sameLink = driver.findElement(By.partialLinkText("Click"));
You need to choose selectors (locators) based on the uniqueness of the element and your specific requirements while writing Selenium scripts. So now you know what the different selector types let us move on to InvalidSelectorException.
When Selenium WebDriver cannot interpret the provided selector due to incorrect syntax, expressions, or invalid context, it throws an InvalidSelectorException.
Why does InvalidSelectorException occur in Selenium?
Here are the most common reasons for InvalidSelectorException with examples:
Syntax Errors in Selectors
This is the most common cause for InvalidSelectorException when there is a syntax error in CSS or XPath expressions. For example, missing brackets or typos in the expression.
driver.findElement(By.xpath("//input[@id='testInput'")); // Missing closing bracket
Using Incorrect Selector Types
When a selector type is used which is not valid for a method being called. For example, if you are using a CSS selector string with an XPath-specific method.
driver.findElement(By.xpath("div.classname")); // Using CSS syntax in XPath
Complex/Unsupported Expressions
There can be cases where the selector expressions are either too complex or unsupported by the WebDriver or browser versions.
driver.findElement(By.cssSelector("input:customPseudoClass")); // Unsupported pseudo-class
Invalid XPath or CSS Expressions
XPath or CSS expressions using incorrect axes, functions, or pseudo-classes are invalid according to the standard specifications of XPath/CSS.
driver.findElement(By.xpath("//input[@name='foo' and @id='bar']")); // Incorrect use of 'and' driver.findElement(By.cssSelector("input[name='foo' && id='bar']")); // Incorrect logical operator in CSS selector
Incorrect Use of WebDriver Methods
For example, you provide non-string arguments to a WebDriver method that expects string arguments. This is an incorrect usage of the method, and InvalidSelectorException is thrown.
driver.findElement(By.cssSelector(123)); // Non-string argument where a string is expected
Ways to Avoid InvalidSelectorException
Use the below methods to avoid falling into the trap of InvalidSelectorException and save time:
Verify Your Selector Syntax
Whichever method you are using, double-check the XPath/CSS selector syntax to avoid losing time on debugging it later. They can be pretty complex, and mistakes can happen, resulting in InvalidSelectorException.
// Incorrect: Missing closing bracket // WebElement element = driver.findElement(By.cssSelector("input[name='username'")); // Correct WebElement element = driver.findElement(By.cssSelector("input[name='username']"));
// Incorrect: Using '&&' instead of 'and' // WebElement element = driver.findElement(By.xpath("//input[@name='username' && @type='text']")); // Correct WebElement element = driver.findElement(By.xpath("//input[@name='username' and @type='text']"));
Use the Correct Selector Type
Make sure that you use the correct selector type matching with your selector method. For example, do not mix the CSS selector with the XPath method.
// Incorrect: Using CSS selector syntax with XPath method // WebElement element = driver.findElement(By.xpath("#username")); // Correct WebElement element = driver.findElement(By.cssSelector("#username"));
Test Selectors Before Use
Before using the selectors in Selenium scripts directly, it is advisable to test them first in the developer tools (for example, Chrome DevTools) to ensure they select the intended element. Follow the below steps:
- In Chrome, right-click on the page and select “Inspect”.
- Go to the “Elements” tab.
- Use “Ctrl + F” to bring up the search box and enter your CSS or XPath selector to see if it finds the correct element.
Appropriately Handle Dynamic Content
Dynamic content and elements change as per the user response, which makes it essential to handle them properly in your Selenium scripts. Always wait for the dynamic elements to load entirely before accessing or working on them.
// Wait for an element to be present before selecting it WebDriverWait wait = new WebDriverWait(driver, 10); WebElement dynamicElement = wait.until(ExpectedConditions.presenceOfElementLocated(By.id("dynamicElement")));
Avoid Complex Selector Expressions
CSS and XPath selector expressions are very powerful but can get complex at times. This makes them prone to error if not handled correctly. Always use simplified selectors to keep them easy to debug and use in Selenium scripts.
// Instead of using a very complex selector, try to simplify it WebElement element = driver.findElement(By.cssSelector("div.content > ul.list > li:first-child"));
Follow Standards
Keep yourself up-to-date with the latest CSS and XPath standards. Also, follow the standard for associated browser and WebDriver capabilities to stay away from InvalidSelectorException as much as possible.
Use Relative Paths Over Absolute Paths
Absolute XPath is more prone to break and provide erroneous results with any UI changes. On the other hand, relative XPath is more flexible and less error-prone. Hence, try to use more relative XPath over absolute paths.
// Instead of using absolute XPath // WebElement element = driver.findElement(By.xpath("/html/body/div/form/input")); // Use relative XPath WebElement element = driver.findElement(By.xpath("//input[@id='username']"));
Selenium With Java Code Example
Here, the code sample demonstrates handling InvalidSelectorException in a Selenium Java test. It imports required classes, initializes WebDriver, and uses a try-catch block to navigate to a webpage, locate an element using a valid CSS selector, and perform actions on the element. If InvalidSelectorException is thrown, the error message is printed, and the browser and WebDriver session are terminated in the finally block.
import org.openqa.selenium.By; import org.openqa.selenium.InvalidSelectorException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class InvalidSelectorExceptionExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://example.com"); // Ensure that the selector syntax is correct WebElement element = driver.findElement(By.cssSelector("validCssSelector")); // Perform actions on the element element.click(); } catch (InvalidSelectorException e) { System.out.println("Invalid selector: " + e.getMessage()); } finally { driver.quit(); } } }
With AI Bypass Your Selector Errors Completely
Selectors (locators) are the language through which Selenium scripts communicate with the web page and elements. With so many selector types and the underlying complexity, InvalidSelectorException is quite common and unavoidable.
Engineers lose time and effort in debugging these exceptions, which is a complete nuisance. Read here 11 reasons why not to use Selenium anymore for test automation. The bottom line is why to waste enormous time, effort, and cost in debugging these Selenium exceptions when you can completely get rid of them.
AI-powered, codeless, intelligent test automation tools such as testRigor are here to save your team from all these Selenium exceptions forever. testRigor does not use unstable XPath/CSS locators to identify the web elements. It uses a new concept called testRigor locators, where you, as a human, can rely on a human’s way of referring to elements like sections, relative locations, tables, etc.
enter "Peter" into "First Name" in "From" section
That’s it! Forget all selector exceptions and easily create test cases using plain English. Everyone in your team can contribute to test creation and execution irrespective of their technical expertise in programming.
testRigor lets you create ultra-stable test cases since it doesn’t use XPath or CSS selectors. Even if there are UI changes or changes in element attributes testRigor’s self-healing capabilities automatically incorporate the changes in the test scripts. Can you see the saved maintenance effort and cost here?
Conclusion
Only change is constant, and change for betterment is always a good sign. If there are ways to perform your tasks easily, quickly, and with greater efficiency and stability, why not use such modern tools?
testRigor quickly mitigates the selector errors since it uses an entirely different concept from Selenium for element identification. Try it to experience it! Save on effort, cost, and enormous maintenance hours using testRigor.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
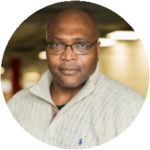