Selenium InvalidCoordinatesException: How to Handle?
|
In Selenium, you will face the InvalidCoordinatesException when the coordinates provided for an action, such as clicking or moving the mouse, are invalid.
Reasons for InvalidCoordinatesException
You might run into this issue for various reasons, such as trying to interact with an element that is not visible on the screen or the coordinates being outside the bounds of the web page or element. Here are the most common causes of this exception:
Element not in the viewport
If the element you are trying to interact with is not in the browser’s currently visible area (viewport), Selenium might be unable to calculate the coordinates correctly. For example, if the element is off-screen, either scrolled out of view or hidden by other elements, Selenium may not be able to find it.
Incorrect coordinate calculation
If you manually calculate coordinates for an action like a mouse move or click, and the calculations are incorrect, it could lead to coordinates outside the bounds of the browser window or the web page.
Element is not rendered
If the element has not been rendered on the page yet, for instance, it might be dynamically loaded, then the coordinates for the element might be invalid or undefined.
Element is hidden or collapsed
If the element is hidden, for example, display: none is set in CSS or collapsed, Selenium cannot interact with it, and attempting to do so might lead to invalid coordinates.
Stale element reference
If the reference to the web element is stale, the element has been reloaded, or the DOM has been updated since the reference was obtained, then the coordinates might no longer be valid.
Incorrect element locator
If the locator used, like XPath or CSS Selector, is incorrect or points to an element different from what was intended, the coordinates derived from this element might not be what you expect.
Changes in page layout
Sometimes, changes in the page’s layout after loading the page due to dynamic content loading, window resizing, etc., can cause previously valid coordinates to become invalid.
Issues with WebDriver
Your browser’s WebDriver implementation might have issues or bugs that can cause unexpected behavior, including invalid coordinate calculations.
Resolution for InvalidCoordinatesException
Here are a few ways to resolve the InvalidCoordinatesException in Selenium:
Make sure the element is visible
element.isDisplayed()
to check if the element is visible on the UI.WebDriver driver = new ChromeDriver(); driver.get("http://example.com/abc-xyz"); WebElement element = driver.findElement(By.id("elementId")); // Check if the element is displayed if (element.isDisplayed()) { element.click();
Wait for the element to be accessible
WebDriverWait,
along with ExpectedConditions
, and wait until the element is ready for interaction.WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); // Create a WebDriverWait instance WebDriverWait wait = new WebDriverWait(driver, 10); // wait for a maximum of 10 seconds // Define the expected condition By locator = By.id("elementId"); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(locator)); // Interact with the element element.click();
Scroll into view
If the element is not in the viewport, then you can use JavaScript or Selenium’s built-in methods to scroll and bring the element into the view.
For example, you can use ((JavascriptExecutor) driver).executeScript("arguments[0].scrollIntoView(true);", element);
in Java.
Check and correct coordinate calculations
Double-check the coordinates calculations when generating them manually for certain actions. Also, make sure they are within the element’s bounds or the browser window. You can use the size and location of elements along with the browser window size to validate your calculations.
Example 1: Checking Coordinates Against Element Bounds
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/abc-xyz"); WebElement element = driver.findElement(By.id("elementId")); // Get element location and size Point location = element.getLocation(); Dimension size = element.getSize(); // Coordinates to check (these should be calculated in your actual code) int xCoordinate = 100; // Example coordinate int yCoordinate = 50; // Example coordinate // Check if coordinates are within element bounds if (xCoordinate >= location.getX() && xCoordinate <= location.getX() + size.getWidth() && yCoordinate >= location.getY() && yCoordinate <= location.getY() + size.getHeight()) {
Example 2: Checking Coordinates Against Browser Window Size
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/abc-xyz"); // Get the browser window size Dimension windowSize = driver.manage().window().getSize(); // Coordinates to check int xCoordinate = 100; // Example coordinate int yCoordinate = 50; // Example coordinate // Check if coordinates are within window bounds if (xCoordinate >= 0 && xCoordinate <= windowSize.getWidth() && yCoordinate >= 0 && yCoordinate <= windowSize.getHeight()) {
Update WebDriver
Sometimes, bugs in the WebDriver can cause such exceptions. To deal with this, ensure that you are using the latest version of the WebDriver that is compatible with your browser.
Use Action Class for complex interactions
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/abc-xyz"); // Locate the source element (element to drag) WebElement sourceElement = driver.findElement(By.id("sourceElementId")); // Locate the target element (where to drop the element) WebElement targetElement = driver.findElement(By.id("targetElementId")); // Create an instance of Actions class Actions actions = new Actions(driver); // Perform the drag-and-drop action actions.dragAndDrop(sourceElement, targetElement).perform();
Handle iFrames
If the element is inside an iframe, switch to that iframe first using driver.switchTo().frame()
before trying to interact with the element.
Handle dynamic elements properly
For dynamically loaded elements (e.g., via AJAX), you can use explicit waits and check the visibility of elements before interacting with them.
Along with the above suggestions, you can further improve the quality of your test code by using debugging and logging to understand the state of the web application when the exception is thrown. Implement try-catch blocks in your code so as to handle exceptions as and when they occur.
testRigor to overcome InvalidCoordinatesException
As popular as Selenium is, it tends to give rise to various issues during the stages of test case creation, execution, and maintenance. You might not want to waste your precious effort and time in resolving Selenium exceptions and errors. Instead of Selenium, you can opt for intelligent tools like testRigor that use AI to make automated testing easy for everyone on the team.
Writing test cases in testRigor is ultra easy as the tool allows you to do so using plain English language. This applies even to identifying elements on the screen. This means that you can forgo the woe of identifying UI elements on the screen through CSS or XPaths.
click on “login”
It is that simple. You need not explicitly write wait statements or scroll down to a particular section if the element is on the page; testrigor automatically manages that for you.
click on "Notes" type "This is a test." send email to "[email protected]" with subject "Test message" and body "Hi, this is a test, this is just a test message." check that page contains "Hello"
testRigor is a modern test automation tool that works on generative AI and NLP and singlehandedly solves your web, mobile, desktop, and API testing needs. It supports cross-browser and cross-platform testing through its powerful integrations with all significant test management, infrastructure providers, and CI/CD tools.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
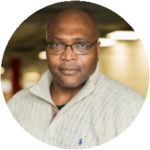