How to Resolve MoveTargetOutOfBoundsException in Selenium?
|
MoveTargetOutOfBoundsException is thrown when an action attempt is made to an element that is not within the browser’s visible viewport. Usually, this exception occurs when mouse movement actions are involved, such as ‘dragAndDrop‘ or ‘moveToElement‘. The element on which some user action is intended is not within the browser’s visible viewport; hence, some scrolling must be done to access the element.
Reasons for MoveTargetOutOfBoundsException
Below are the common reasons for MoveTargetOutOfBoundsException in Selenium:
Element is out of the browser’s viewport
The web element to be accessed in the Selenium script is present on the screen; however, it is currently out of the visible area of the browser window. The element could be located too far to the right/left or down on the screen and not visible. This requires scrolling through the browser window to bring the element within the browser’s viewport.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); WebElement element = driver.findElement(By.id("offscreenElement")); // Element is off-screen new Actions(driver).moveToElement(element).click().build().perform(); // Throws MoveTargetOutOfBoundsException
Element co-ordinates are not accessible
Consider you are using moveByOffset to provide the element coordinates to move the mouse pointer. In this case, if the element is outside the viewport, MoveTargetOutOfBoundsException is thrown.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); new Actions(driver).moveByOffset(2000,2000).click().build().perform(); // Throws MoveTargetOutOfBoundsException
Dynamic content
In dynamic webpages, the content is dynamic; hence, the position of the web elements is also dynamic and changes. Now, the exception is thrown if the element moves after the dynamic location is calculated, and then the script tries to access the element based on the old calculated location.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/dynamic-content-page"); WebElement element = driver.findElement(By.id("dynamicElement")); // Assuming the element moves due to dynamic content loading new Actions(driver).moveToElement(element).click().build().perform(); // Might throw MoveTargetOutOfBoundsException
Fixed (sticky) UI elements
Some elements are fixed on the UI, such as header/footers. They are called fixed or sticky elements. These elements can interfere with the coordinate calculation of the other UI elements, leading to MoveTargetOutOfBoundsException.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/page-with-fixed-header"); WebElement elementBelowHeader = driver.findElement(By.id("elementBelowFixedHeader")); // The fixed header might interfere with the actual location of this element new Actions(driver).moveToElement(elementBelowHeader).click().build().perform(); // Might throw MoveTargetOutOfBoundsException
iFrame context switch
If the Selenium script tries to interact with an element within an iFrame and the context is not switched. Then, in this case, an exception will occur.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com/page-with-iframe"); // Directly trying to interact with an element inside an iframe WebElement elementInIframe = driver.findElement(By.id("elementInIframe")); // Throws NoSuchElementException or MoveTargetOutOfBoundsException // The correct approach is to first switch to the iframe
Resolution for MoveTargetOutOfBoundsException
Below are a few ways to resolve the MoveTargetOutOfBoundsException in Selenium:
Scroll to the element
The simplest way to handle MoveTargetOutOfBoundsException is to scroll to the element and bring it within the browser’s visible viewport.
import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; WebDriver driver = new ChromeDriver(); WebElement element = driver.findElement(By.id("element_id")); ((JavascriptExecutor) driver).executeScript("arguments[0].scrollIntoView(true);", element); Actions actions = new Actions(driver); actions.moveToElement(element).perform();
Adjust browser window size
Another option is to adjust the browser window size to make sure that the browser window is big enough to include the UI element within the browser’s viewport.
driver.manage().window().setSize(new Dimension(1024, 768));
Use JavaScript direct interactions
If you can still not interact normally with the element, then as a workaround, use JavaScript to interact directly with the element.
import org.openqa.selenium.JavascriptExecutor; WebElement element = driver.findElement(By.id("element_id")); ((JavascriptExecutor) driver).executeScript("arguments[0].click();", element);
Correctly handle dynamic content
To handle the dynamic content and the dynamic locations of the elements, use explicit waits to ensure that the page has finished loading and that dynamic elements have stabilized.
import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; WebDriverWait wait = new WebDriverWait(driver, 10); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("element_id")));
Switch to correct iFrame
If the element is inside an iframe, switch the WebDriver context to that iframe before performing any action on the web element.
driver.switchTo().frame("iframeNameOrID"); WebElement element = driver.findElement(By.id("element_id_inside_iframe")); // Interact with the element here driver.switchTo().defaultContent(); // Switch back to the main document
How testRigor is an Intelligent Alternative?
Selenium has enjoyed being the most popular test automation tool for years. Being open-source and a vast community has contributed to the success. Here is a Selenium with Java cheat sheet for you to refer to.
However, with faster delivery cycles in Agile environments, Selenium is becoming an overhead with enormous exceptions and maintenance issues. Here are the 11 reasons why not to use Selenium anymore.
AI-powered, codeless, intelligent test automation tools such as testRigor are game changers in the test automation paradigm. Anyone in your team can write the test cases in plain English without any pre-requisite programming knowledge using NLP and generative AI capabilities of testRigor.
You need not deliberately put explicit waits in your test case to wait for the elements to load entirely before any interaction. That is the power of AI; testrigor manages all these waits automatically for you. Specify the UI elements as you see them on the screen and not through tedious XPath/ CSS identifiers.
click on "Add to cart"
click on the 3rd "Add to cart"
click on the second "Add to Cart" within the context of "Section 1"
Quickly scroll through the page as testRigor supports scroll in all four directions: up, down, left, and right.
scroll up by ¼ of the screen
scroll up until page contains “Place order”
scroll up on “Contact Us”
Conclusion
Selenium exceptions are annoying and time-consuming. There is no second thought to this. Engineers do not want to waste time resolving these issues using workarounds and get stuck in laborious test script maintenance.
When there are better alternatives that use AI to resolve these issues for you, why not use them? Try testRigor to see how liberating it is when a tool singlehandedly performs all your web, mobile, desktop, and API testing in plain English. Bypass all these Selenium exceptions and errors and only focus on building robust tests, achieving excellent test coverage and scalability.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
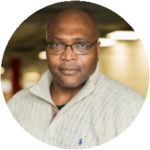