MyBanco Testing
|
MyBanco is a core banking framework that provides the necessary infrastructure and functionalities to support banking operations. Since it is a core banking application, you can expect it to provide a centralized solution for all customer data, including transaction history, account details, and personal information. It also provides the capability to handle various banking services such as account creation, account management, deposits, withdrawals, loan management, payment processing, and other customer-related services.
Being open-source, you can build on top of the core functionalities of MyBanco to customize it into a system that fits your needs. Moreover, you can integrate with other banking systems, financial networks, and third-party services like payment gateways, credit card processing, etc., to provide a seamless banking experience.
MyBanco is built on PHP; hence, you need to use frameworks that work well with PHP to test the code as per the test automation pyramid.
This guide explores how you can test your MyBanco code through:
MyBanco unit testing
Unit testing holds great importance regarding quality assurance. It is the first level of check you can put on your code to ensure it is doing what it should. Unit tests are lightweight tests that tend to focus on validating single outcomes.
For example, if you have a method that debits wallet balance amount, you can write multiple unit tests that check what happens when you provide valid numbers, invalid or borderline values.
Since these tests verify the very behavior of the code up and close, they target various code units, like functions, classes, and APIs. Several tools and frameworks are available for writing unit tests in PHP. Each offers different features and integrates with various development environments.
Here are some of the most commonly used ones.
PHPUnit
PHPUnit is the jack-of-all-trades and the most popular testing framework for PHP. It’s an XUnit-style test runner, and it’s widely used in the PHP community. It offers code coverage analysis, assertions to check for various conditions, and the ability to mock objects.
// File: CalculatorTest.php use PHPUnit\Framework\TestCase; class CalculatorTest extends TestCase { public function testAdd() { $calculator = new Calculator(); $result = $calculator->add(2, 3); $this->assertEquals(5, $result); } }
PHPSpec
PHPSpec is a specification-oriented BDD (Behavior-Driven Development) tool. It’s designed to write clean and working PHP code using the concept of specification. It encourages precise, descriptive testing and drives design through specification. It is more about defining an object’s behavior rather than simply testing methods.
Codeception
Codeception is a full-stack testing framework that encompasses all types of testing: unit tests and application tests, including functional and acceptance tests. It offers a rich set of modules for testing RESTful APIs, browser testing, and many other functions. It also integrates with popular frameworks like Zend Framework, Yii, and Symfony.
Behat
Behat is a BDD framework for PHP. It’s not strictly a unit testing framework but is often used in conjunction with PHPUnit or PHPSpec for behavior-driven development. It offers an easy way to write human-readable stories that describe the behavior of an application.
Mockery
Mockery is a mock object framework that you can use alongside other testing frameworks like PHPUnit. It’s particularly useful for mocking objects in tests. It offers a flexible API for creating mock objects and setting up expectations.
MyBanco integration testing
Now that you’ve tested the various code units in isolation, it’s time to test them together. In integration testing, we do not test all of it simultaneously, only those junctions where noteworthy integrations occur. This could mean an API writing to a database or a front-end element triggering a third-party service or even integrating third-party services like credit card payment gateways with your application.
You can use one or more of the frameworks and tools mentioned above to achieve your needs. For example, you can use Behat to write integration tests in easy language, then use PHPUnit and Mockery to implement these integration tests and subsequently mock required dependencies.
MyBanco end-to-end testing
So far, we have focused on ensuring that the code is well-written and doesn’t give rise to any obvious problems. Now, let’s zoom out and take a look at how the entire system behaves when tested. What this essentially means is that you are now interacting with the system in a way an end user would do.
For example, you might log in using the browser, come to your profile, transfer some funds, or make payments, and then log out.
End-to-end testing is an important part of the QA process as it gives a taste of the user’s experience when working with the system. Quite often than not, as developers and testers, we overlook certain system aspects that we know only because we are familiar with the internal workings. These points may not be obvious to end users and must not be taken for granted.
A simple example of this would be hiding the logout button in a menu option that isn’t visible outright. As the system’s creator, you know where to find it, but a normal user would struggle with completing their workflows just because of such a simple issue.
You will find quite a few tools and frameworks in the market that allow end-to-end testing.
Behat
Behat is well-suited for end-to-end testing because it allows you to write tests in a language that non-technical stakeholders understand, which is Gherkin. It is a plain-text language that allows you to describe software behaviors without detailing how that functionality is implemented.
Gherkin uses a set of special keywords to give structure and meaning to executable specifications. The main keywords are:
- Feature: A high-level description of a software feature.
- Scenario: A single path or behavior through the feature.
- Given, When, Then, And, But: Steps used to describe an event or a state. “Given” is used to describe the initial context, “When” describes an action, and “Then” the outcome or result. “And” and “But” are used to add more steps.
Behat integrates with your PHP application and allows you to define, in your feature files, how your application should behave. Each step in a Gherkin scenario is matched to a PHP function that Behat calls when it executes the scenario. These functions are known as step definitions, allowing Behat to interact with your application.
# File: features/account_management.feature Feature: Account Management In order to manage my bank accounts As a bank customer I need to be able to view my account balance and recent transactions Scenario: Viewing account balance Given I am logged in as a customer And I have an account with a balance of $1000 When I navigate to the account summary page Then I should see a balance of $1000 Scenario: Viewing recent transactions Given I am logged in as a customer And I have recent transactions in my account When I navigate to the recent transactions page Then I should see a list of recent transactions
For UI-based testing, you can integrate Behat with Selenium. However, this entire setup has its drawbacks as it makes the testing process lengthy and difficult to maintain, requiring a niche group of professionals who can code everything here. Read here the 11 reasons why not to use Selenium.
Codeception
Codeception is particularly powerful for end-to-end testing because it is designed to simplify and streamline the process of writing and running tests. It extends the standard testing frameworks like PHPUnit to provide additional functionality and syntax that are optimized for writing such tests. It is built to be flexible and is compatible with many popular PHP frameworks and libraries.
Codeception supports Behavior-Driven Development (BDD) through a simple and expressive PHP DSL (Domain-Specific Language). You can descriptively write your tests, making it easier to understand what the test does. It even supports browser testing with WebDriver (Selenium) and PhpBrowser, allowing you to perform or emulate tests in real browsers.
Codeception can be integrated with tools like Selenium for web browser automation and PhantomJS for headless browser testing. It also works well with many continuous integration systems.
testRigor
When it comes to end-to-end testing of web applications, especially banking applications, the user-specific test cases are often known to team members from non-technical backgrounds like manual QA, business analysts, client-facing analysts, etc. In such situations, you are better off using a testing tool everyone can use.
Though the BDD tools offer this, they involve a lot of coding efforts to convert the BDD statements into executable code. Luckily, there are tools in the market like testRigor that provide this without the hassles involved in using the above-mentioned tools.
testRigor is a one-of-a-kind AI-based test automation tool that can be used to automate simple as well as complex end-to-end test cases. This can be done for applications operating across platforms.
Writing tests in testRigor is very easy. Write test steps in plain English using its generative AI capabilities without writing any other code to make those plain English statements work. The AI engine is smart enough to understand and identify what web element you are talking about and execute test cases.
login as customer click on “My Profile” click on “Check Balance” check that page contains “Balance = $1000” navigate to account summary page check that page contains “Balance = $1000”
The use of AI does not stop at this. testRigor uses AI to make test maintenance, which tends to be a big complaint for test automation engineers, negligible. This helps you continue to focus on creating quality test cases rather than fixing exceptions and XPath/CSS identifiers of elements.
This was just the tip of the iceberg. You can do a lot more with testRigor. Check out this tool’s features over here.
Conclusion
When testing a banking application, you’re not just testing the code; you’re ensuring that the application behaves as expected from the user’s perspective, which is crucial for applications dealing with sensitive financial transactions.
For such sensitive applications, opt for a testing tool that lets you focus on identifying test cases rather than spending time writing and maintaining them. Also, the tool should be intelligent enough to handle complex testing such as database, 2FA, SMS, file, visual, email, QR code, geolocation testing, etc. testRigor helps you run all these complicated test steps in plain English, saving you enormous time, effort and cost, securely.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
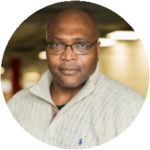