Reasons for ScriptTimeoutException in Selenium
|
Timeouts are the mechanisms Selenium uses to let the WebDriver know how much maximum time to allocate for a particular operation or condition. An exception is thrown when the operation is not completed within the specified time.
These timeouts help identify the situations where a script takes too long to execute, or some operation takes longer than expected, such as element loading.
A script timeout is used to set the time for an asynchronous script to finish execution. If the script does not finish execution within the specified time, then a ScriptTimeoutException is thrown. This timeout is specifically helpful while executing executeAsyncScript to run JavaScript.
Let us have a look at the reasons for ScriptTimeoutException:
Why does ScriptTimeoutException Happen?
Here are a few reasons for ScriptTimeoutException in Selenium:
Long-running scripts
There could be cases when the script takes longer than expected time to execute, such as when processing large amounts of data. Another example is when a script is waiting for some other conditions, and there is no proper exit strategy, and it keeps waiting. In such situations, the ScriptTimeoutException is thrown in Selenium.
WebDriver driver = new ChromeDriver(); driver.manage().timeouts().setScriptTimeout(2, TimeUnit.SECONDS); try { // A script that is expected to take more than 2 seconds driver.executeAsyncScript("window.setTimeout(arguments[arguments.length - 1], 3000);"); } catch (ScriptTimeoutException e) { System.out.println("Script took too long to run and timed out"); }
In the above example, the script is set to complete in 2 seconds, while the script will take 3 seconds to execute as in executeAsyncScript. Therefore, a ScriptTimeoutException is thrown because the script cannot be completed in the stipulated time.
Asynchronous operations waiting
When scripts involve asynchronous operations such as AJAX calls, these operations take longer than expected. Another case is to keep the script waiting for completion, leading to an indefinite wait. Such scenarios cause ScriptTimeoutException in Selenium.
WebDriver driver = new ChromeDriver(); driver.manage().timeouts().setScriptTimeout(5, TimeUnit.SECONDS); try { // Script with an AJAX call that might take an unknown amount of time String script = "var callback = arguments[arguments.length - 1];" + "fetch('https://some-api.com/data').then(function(response) {" + " callback(response.json());" + "}).catch(function(error) {" + " callback('Error');" + "});"; driver.executeAsyncScript(script); } catch (ScriptTimeoutException e) { System.out.println("Script timed out due to a long-running AJAX call"); }
Incorrect timeout
If you have set a shorter timeout for the script and the script usually takes longer than it, then the exception is bound to be thrown. Hence, properly evaluate the time required for the script to run and then set the timeout accordingly successfully.
Unresponsive web elements or browser
If the web elements or the browser are unresponsive, that may cause the script to hang. Since the script has stopped running, the execution gets halted, the timeout exceeds, and the exception occurs.
Network issues or delays
The script might fetch and wait for remote resources where the communication is through networks. If there happens to be any delay or issue in the network communication, the script will continue to wait indefinitely. In such cases, the timeout will be exceeded, and Selenium throws ScriptTimeoutException.
Ways to Resolve ScriptTimeoutException
The most important technique to avoid ScriptTimeoutException is to manage allocated script execution time effectively in the test scripts. Follow the below methods to prevent and resolve ScriptTimeoutException:
Increase script timeout value
WebDriver driver = new ChromeDriver(); // Setting the script timeout to 60 seconds driver.manage().timeouts().setScriptTimeout(60, TimeUnit.SECONDS); try { driver.executeAsyncScript("window.setTimeout(arguments[arguments.length - 1], 50000);"); } catch (ScriptTimeoutException e) { System.out.println("Script execution took too long"); }
Review and optimize the script
You can review and optimize the script to make it quicker and avoid falling into the ScriptTimeOutException. Some optimizations could be to reduce complexity, optimize loop processing, enhance data processing, etc.
Properly handle the asynchronous operations
String asyncScript = "var callback = arguments[arguments.length - 1];" + "fetch('https://api.example.com/data')" + ".then(response => response.json())" + ".then(data => callback(data))" + ".catch(error => callback('Error'));"; try { Object result = driver.executeAsyncScript(asyncScript); } catch (ScriptTimeoutException e) { System.out.println("Asynchronous script timed out"); }
Use explicit waits
WebDriverWait wait = new WebDriverWait(driver, 20); wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("someId"))); // Now perform actions with the element
Split longer scripts into smaller ones
// Executing a long script in smaller parts for (String part : scriptParts) { driver.executeAsyncScript(part); }
Handle network issues and delays
String networkDependentScript = "var callback = arguments[arguments.length - 1];" + "fetch('https://api.example.com/data', { timeout: 10000 })" + // Setting a timeout for the fetch request ".then(response => response.json())" + ".then(data => callback(data))" + ".catch(error => callback('Error'));"; try { Object result = driver.executeAsyncScript(networkDependentScript); } catch (ScriptTimeoutException e) { System.out.println("Network-dependent script timed out"); }
Instead, use an Intelligent AI-powered Solution
If you are fed up with the enormous and never-ending Selenium exceptions and looking for alternatives, we have a piece of good news. Now, you need not waste time debugging and resolving Selenium errors and exceptions. Also, you can avoid working on indefinite maintenance. Here are the 11 reasons NOT to use Selenium for automation testing anymore.
enter "TV" into "Search" enter enter click "TV" click "Add To Cart" check that page contains "Item Added To Cart"
As you can see in the above example, CSS/XPath locators are not used. Directly write test steps in English with what you see on the UI for element identification. testRigor automatically handles the waits; hence, you need not add any explicit waits for page/element loading. This reduces the enormous load off your shoulders and eases the whole process.
Regardless of their technical competency, anyone in your team can write and execute test cases in English using the generative AI and NLP capabilities of testRigor. Quicken your web, mobile, desktop, and API testing with broader test coverage and minimum maintenance effort.
Conclusion
It is not the software testing that is giving you sleepless nights. It is the choice of testing tool that is causing all the trouble. Double-check your team’s skills, deadlines, coverage requirements, and required time for creating test scripts before you decide upon a test automation tool.
You can save effort, time, and cost with the right choice. With testRigor, your testing team can focus on what they are meant for, i.e., creating incredible, robust tests. Use your testing skills in the right direction to gain superior quality products quickly and not on debugging and exception handling.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
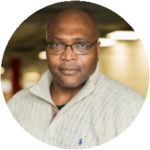