Selenium with Java Cheat Sheet
|
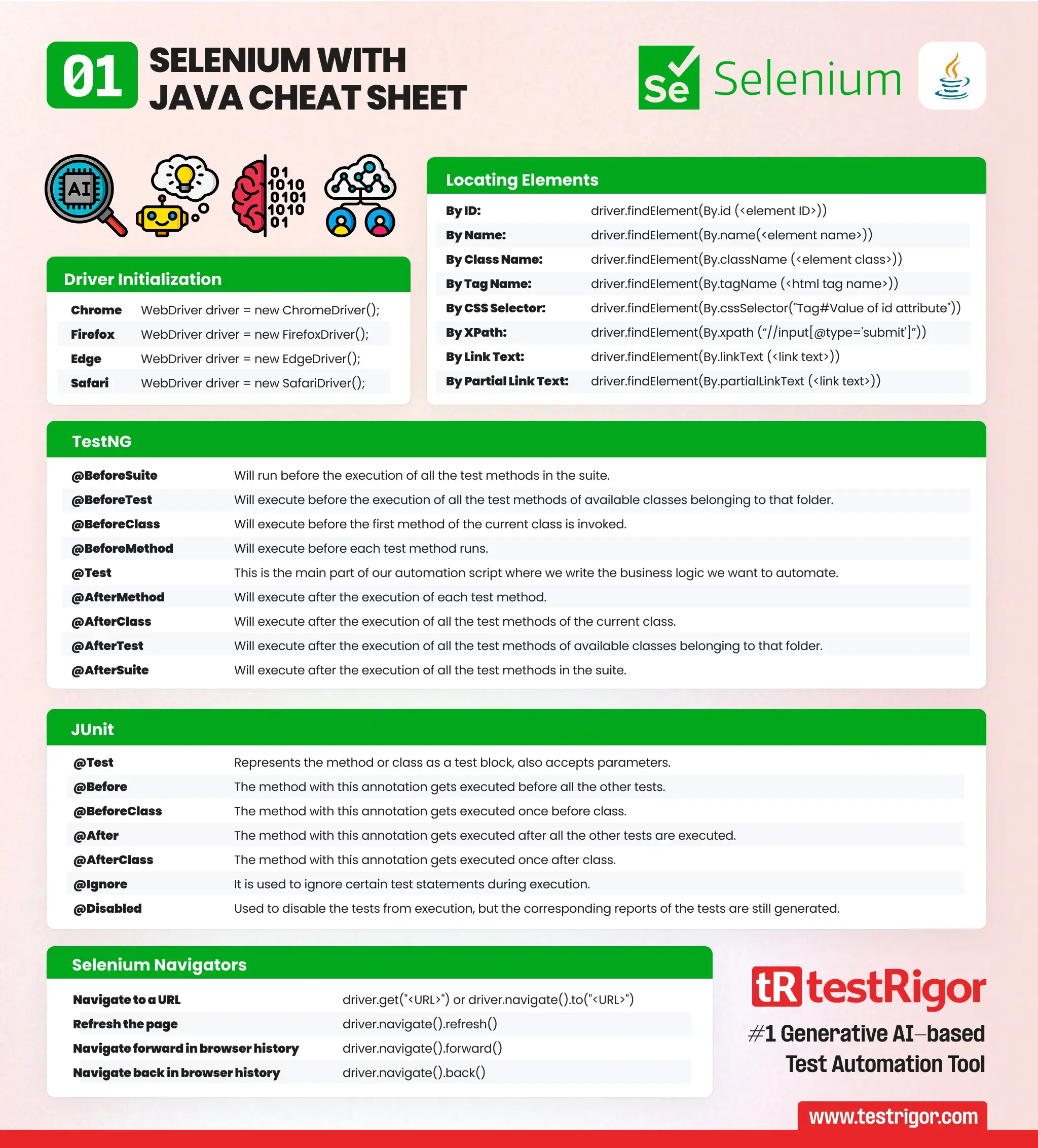
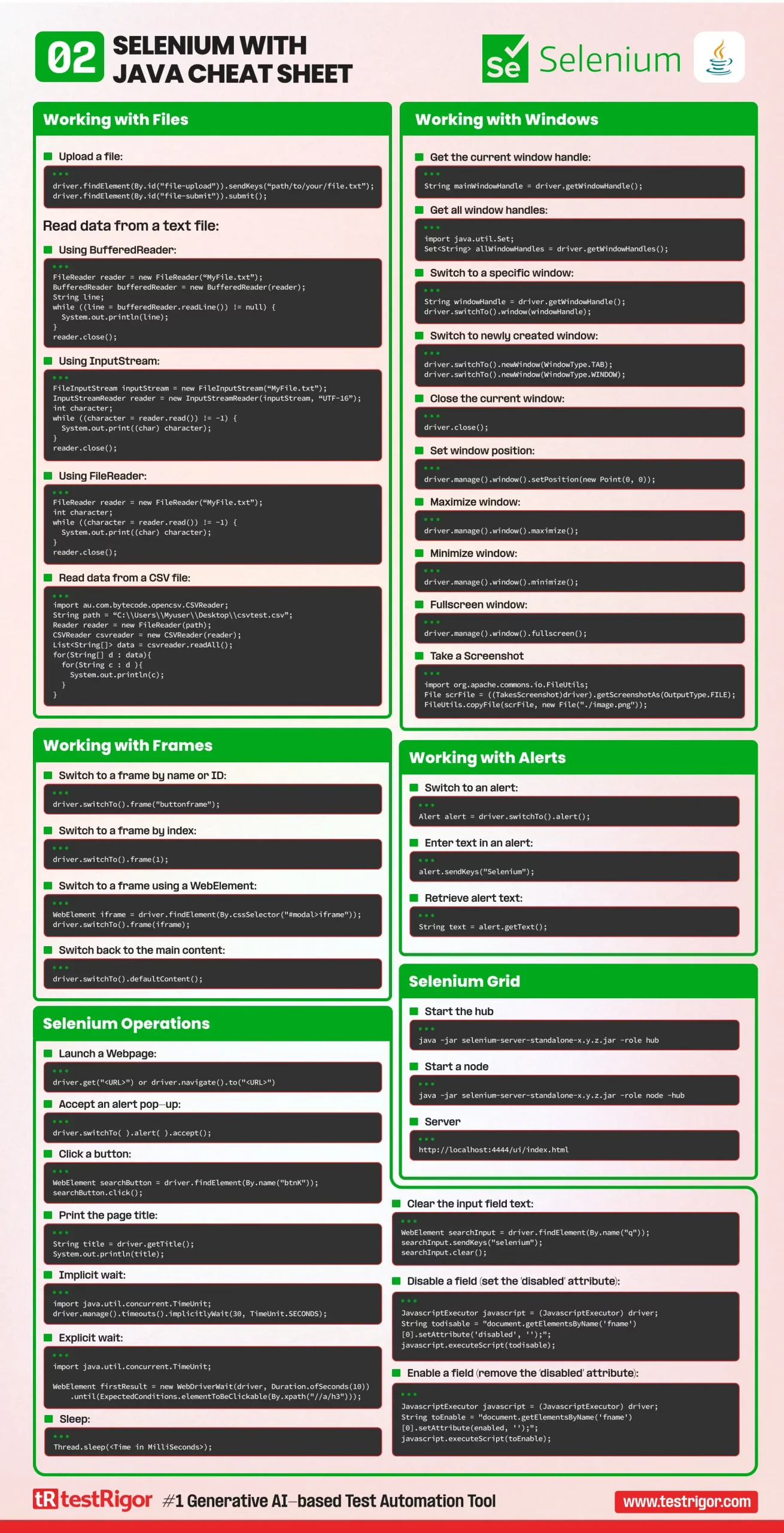
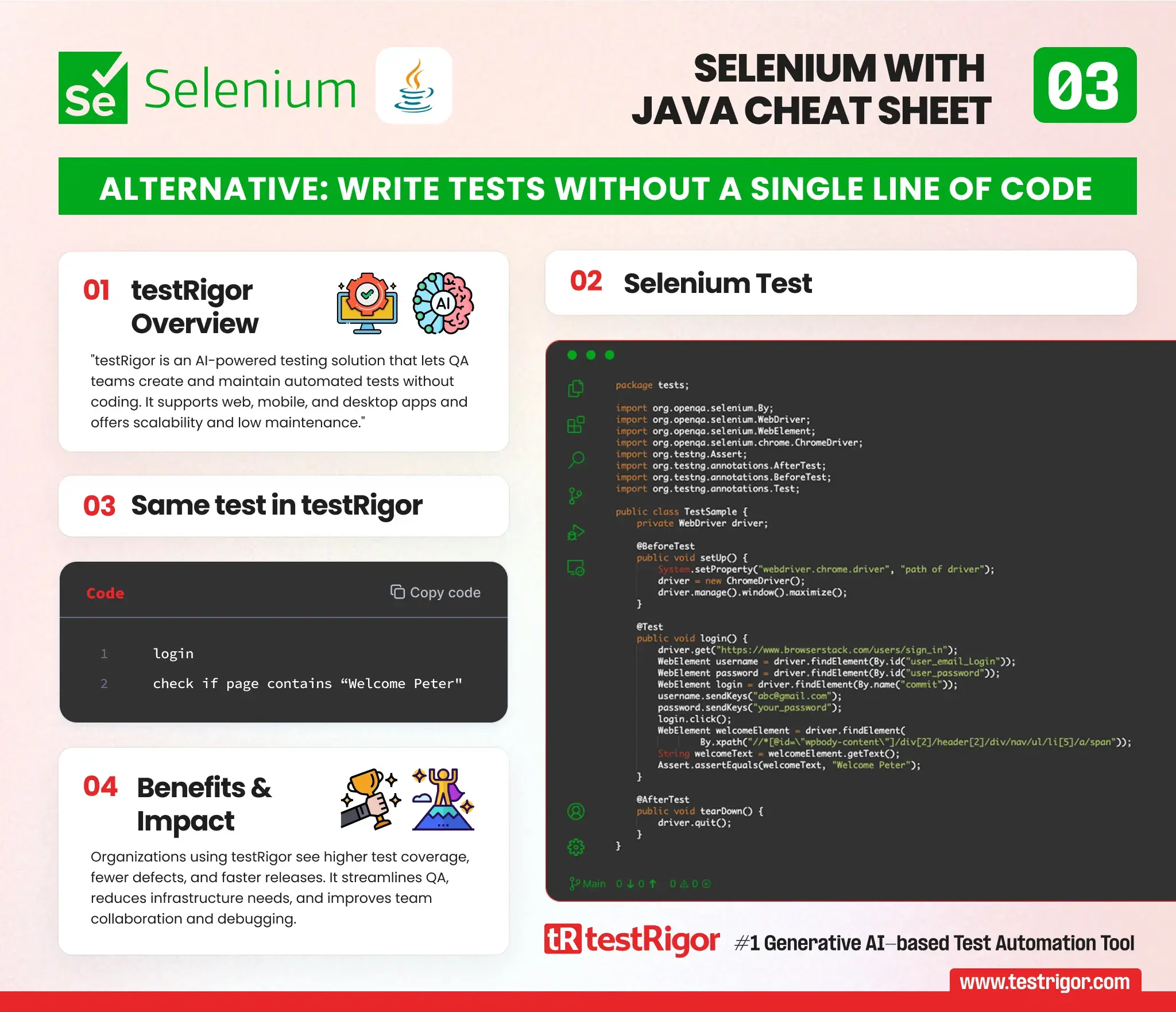
Driver Initialization |
|
Chrome | WebDriver driver = new ChromeDriver(); |
---|---|
Firefox | WebDriver driver = new FirefoxDriver(); |
Edge | WebDriver driver = new EdgeDriver(); |
Safari | WebDriver driver = new SafariDriver(); |
Locating Elements |
|
By ID: | driver.findElement(By.id (<element ID>)) |
By Name: | driver.findElement(By.name(<element name>)) |
By Class Name: | driver.findElement(By.className (<element class>)) |
By Tag Name: | driver.findElement(By.tagName (<html tag name>)) |
By CSS Selector: | driver.findElement(By.cssSelector("Tag#Value of id attribute")) |
By XPath: | driver.findElement(By.xpath (“//input[@type='submit']”)) |
By Link Text: | driver.findElement(By.linkText (<link text>)) |
By Partial Link Text: | driver.findElement(By.partialLinkText (<link text>)) |
TestNG |
|
@BeforeSuite | Will run before the execution of all the test methods in the suite |
@BeforeTest | Will execute before the execution of all the test methods of available classes belonging to that folder |
@BeforeClass | Will execute before the first method of the current class is invoked |
@BeforeMethod | Will execute before each test method runs |
@Test | This is the main part of our automation script where we write the business logic we want to automate |
@AfterMethod | Will execute after the execution of each test method |
@AfterClass | Will execute after the execution of all the test methods of the current class |
@AfterTest | Will execute after the execution of all the test methods of available classes belonging to that folder |
@AfterSuite | Will execute after the execution of all the test methods in the suite |
JUnit |
|
@Test | Represents the method or class as a test block, also accepts parameters. |
@Before | The method with this annotation gets executed before all the other tests. |
@BeforeClass | The method with this annotation gets executed once before class. |
@After | The method with this annotation gets executed after all the other tests are executed. |
@AfterClass | The method with this annotation gets executed once after class. |
@Ignore | It is used to ignore certain test statements during execution. |
@Disabled | Used to disable the tests from execution, but the corresponding reports of the tests are still generated. |
Working with Files |
|
Upload a file:
driver.findElement(By.id("file-upload")).sendKeys(“path/to/your/file.txt”); driver.findElement(By.id("file-submit")).submit(); Read data from a text file:Using BufferedReader:
FileReader reader = new FileReader(“MyFile.txt”); BufferedReader bufferedReader = new BufferedReader(reader); String line; while ((line = bufferedReader.readLine()) != null) { System.out.println(line); } reader.close(); Using InputStream:
FileInputStream inputStream = new FileInputStream(“MyFile.txt”); InputStreamReader reader = new InputStreamReader(inputStream, “UTF-16”); int character; while ((character = reader.read()) != -1) { System.out.print((char) character); } reader.close(); Using FileReader:
FileReader reader = new FileReader(“MyFile.txt”); int character; while ((character = reader.read()) != -1) { System.out.print((char) character); } reader.close(); Read data from a CSV file:
mport au.com.bytecode.opencsv.CSVReader; String path = “C:\\Users\\Myuser\\Desktop\\csvtest.csv”; Reader reader = new FileReader(path); CSVReader csvreader = new CSVReader(reader); List<String[]> data = csvreader.readAll(); for(String[] d : data){ for(String c : d ){ System.out.println(c); } } Read data from an Excel file:
import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import java.io.File; import java.io.FileInputStream; import java.io.IOException; File file = new File(“E:\\TestData\\TestData.xls”); FileInputStream inputStream = new FileInputStream(file); HSSFWorkbook wb=new HSSFWorkbook(inputStream); HSSFSheet sheet=wb.getSheet(“STUDENT_DATA”); HSSFRow row2=sheet.getRow(1); HSSFCell cell=row2.getCell(5); String address= cell.getStringCellValue(); |
|
Selenium Navigators |
|
Navigate to a URL | driver.get("<URL>") or driver.navigate().to("<URL>") |
Refresh the page | driver.navigate().refresh() |
Navigate forward in browser history | driver.navigate().forward() |
Navigate back in browser history | driver.navigate().back() |
Working with Windows |
|
Get the current window handle:
String mainWindowHandle = driver.getWindowHandle(); Get all window handles:
import java.util.Set; Set<String> allWindowHandles = driver.getWindowHandles(); Switch to a specific window:
String windowHandle = driver.getWindowHandle(); driver.switchTo().window(windowHandle); Switch to newly created window:
driver.switchTo().newWindow(WindowType.TAB); driver.switchTo().newWindow(WindowType.WINDOW); Close the current window:
driver.close(); Set window position:
driver.manage().window().setPosition(new Point(0, 0)); Maximize window:
driver.manage().window().maximize(); Minimize window:
driver.manage().window().minimize(); Fullscreen window:
driver.manage().window().fullscreen(); Take a Screenshot
import org.apache.commons.io.FileUtils; File scrFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(scrFile, new File("./image.png")); |
|
Working with Frames |
|
Switch to a frame by name or ID:
driver.switchTo().frame("buttonframe"); Switch to a frame by index:
driver.switchTo().frame(1); Switch to a frame using a WebElement:
WebElement iframe = driver.findElement(By.cssSelector("#modal>iframe")); driver.switchTo().frame(iframe); Switch back to the main content:
driver.switchTo().defaultContent(); |
|
Working with Alerts |
|
Switch to an alert:
Alert alert = driver.switchTo().alert(); Enter text in an alert:
alert.sendKeys("Selenium"); Retrieve alert text:
String text = alert.getText(); |
|
Selenium Operations |
|
Launch a Webpage:
driver.get("<URL>") or driver.navigate().to("<URL>") Click a button:
WebElement searchButton = driver.findElement(By.name("btnK")); searchButton.click(); Accept an alert pop-up:
driver.switchTo( ).alert( ).accept(); Print the page title:
String title = driver.getTitle(); System.out.println(title); Implicit wait:
import java.util.concurrent.TimeUnit; driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); Explicit wait:
import java.util.concurrent.TimeUnit; WebElement firstResult = new WebDriverWait(driver, Duration.ofSeconds(10)) .until(ExpectedConditions.elementToBeClickable(By.xpath("//a/h3"))); Sleep:
Thread.sleep(<Time in MilliSeconds>); Clear the input field text:
WebElement searchInput = driver.findElement(By.name("q")); searchInput.sendKeys("selenium"); searchInput.clear(); Disable a field (set the ‘disabled’ attribute):
JavascriptExecutor javascript = (JavascriptExecutor) driver; String todisable = "document.getElementsByName('fname')[0].setAttribute('disabled', '');"; javascript.executeScript(todisable); Enable a field (remove the ‘disabled’ attribute):
JavascriptExecutor javascript = (JavascriptExecutor) driver; String toEnable = "document.getElementsByName('fname')[0].setAttribute(enabled, '');"; javascript.executeScript(toEnable); |
|
Selenium Grid |
|
Start the hub
java -jar selenium-server-standalone-x.y.z.jar -role hub Start a node
java -jar selenium-server-standalone-x.y.z.jar -role node -hub Server
http://localhost:4444/ui/index.html |
Alternative: write tests without a single line of code
testRigor is an AI-driven, end-to-end testing solution that empowers QA professionals to create and maintain complex automated tests without requiring coding skills. This comprehensive system supports testing of various platforms, including web, mobile, and desktop applications, and is suitable for functional regression, accessibility, load, and performance testing. By leveraging plain English statements and an advanced AI engine, testRigor provides excellent scalability, minimal maintenance, and faster test execution, setting itself apart from coded automation tools such as Selenium.
Selenium test:
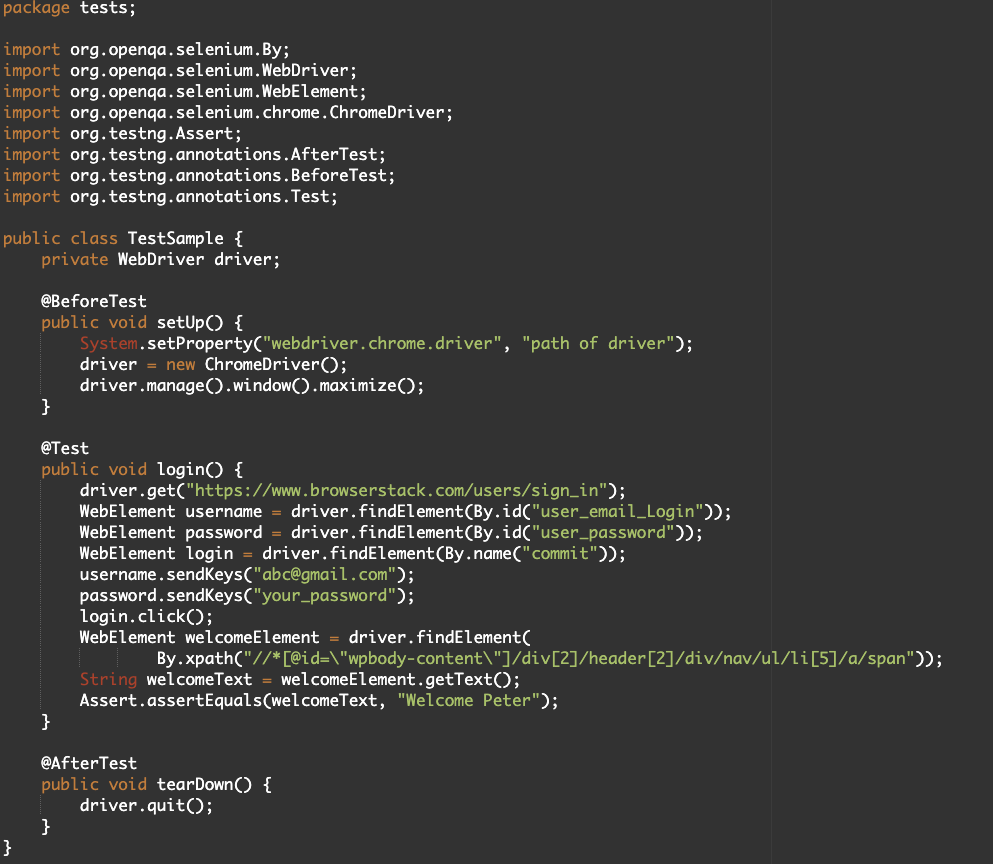
Same test in testRigor:
login check if page contains “Welcome Peter"
Organizations that have adopted testRigor have experienced significant improvements in their software testing processes, with increased test coverage, reduced defect escape rates, and accelerated release cycles. The platform not only enhances QA teams and delivery processes but also eliminates the need for maintaining testing infrastructure. The unique approach of testRigor allows for seamless collaboration among team members and ensures tests remain valid even after underlying framework changes or refactoring. This superior choice in test automation provides numerous key benefits, including cost savings, improved communication, and detailed test results for seamless debugging.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
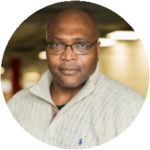