Selenium with JS (JavaScript) Cheat Sheet
|
Driver Initialization |
|
Chrome | const driver = await new Builder().forBrowser('chrome').build(); |
---|---|
Firefox | const driver = await new Builder().forBrowser('firefox').build(); |
Edge | const driver = await new Builder().forBrowser('edge').build(); |
Safari | const driver = await new Builder().forBrowser('safari').build(); |
Locating Elements |
|
By ID: | By.id('<element ID>') |
By Name: | By.name('<element name>') |
By Class Name: | By.className('<element class>') |
By Tag Name: | By.tagName('<html tag name>') |
By CSS Selector: | By.css('<CSS selector>') |
By XPath: | By.xpath('<XPath expression>') |
By Link Text: | By.linkText('<link text>') |
By Partial Link Text: | By.partialLinkText('<partial link text>') |
Working with Files |
|
Upload a file:
const inputElement = await driver.findElement(By.css('<input_element_css_selector>')); await inputElement.sendKeys('<path_to_your_file>'); Read data from a text file:
const fs = require('fs'); // Read the contents of a file fs.readFile('myfile.txt', 'utf8', (err, data) => { if (err) { console.error(err); return; } console.log(data); }); Read data from a CSV file:
const fs = require('fs'); const parse = require('csv-parse/lib/sync'); // Read the contents of a CSV file const fileContents = fs.readFileSync('mycsvfile.csv', 'utf-8'); const records = parse(fileContents, { columns: true }); console.log(records); Read data from an Excel file:
const XLSX = require('xlsx'); // Read the contents of an Excel file const workbook = XLSX.readFile('myexcelfile.xlsx'); const sheetName = workbook.SheetNames[0]; const worksheet = workbook.Sheets[sheetName]; const jsonData = XLSX.utils.sheet_to_json(worksheet); console.log(jsonData); |
|
Selenium Navigators |
|
Navigate to a URL | await driver.get('<URL>'); |
Refresh the page | await driver.navigate().refresh(); |
Navigate forward in browser history | await driver.navigate().forward(); |
Navigate back in browser history | await driver.navigate().back(); |
Working with Windows |
|
Get the current window handle:
const mainWindowHandle = await driver.getWindowHandle(); Get all window handles:
const allWindowHandles = await driver.getAllWindowHandles(); Switch to a specific window:
await driver.switchTo().window(windowHandle); Switch to newly created window:
await driver.switchTo().newWindow('tab'); await driver.switchTo().newWindow('window'); Close the current window:
await driver.close(); Set window size:
await driver.manage().window().setRect({width: 800,height: 600 }); Maximize window:
await driver.manage().window().maximize(); Set window position:
await driver.manage().window().setRect({x: 0,y: 0 }); |
|
Working with Frames |
|
Switch to a frame by name or ID:
await driver.switchTo().frame('<frame name or ID>'); Switch to a frame by index:
await driver.switchTo().frame(index); Switch to a frame using a WebElement:
await driver.switchTo().frame(driver.findElement(By.css(‘<frame CSS selector>’))); Switch back to the main content:
await driver.switchTo().defaultContent(); |
|
Working with Dropdowns (Select Elements) |
|
Find the dropdown element:
const dropdown = await driver.findElement(By.id('dropdown_id')); Create a Select instance for the dropdown element:
const select = new Select(dropdown); Select an option by visible text:
await select.selectByVisibleText('Option Text'); Select an option by value:
await select.selectByValue('option_value'); Select an option by index:
await select.selectByIndex(1); Deselect all options (only applicable for multi-select dropdowns):
await select.deselectAll(); Get all selected options:
const selectedOptions = await select.getAllSelectedOptions(); Get the first selected option:
const firstSelectedOption = await select.getFirstSelectedOption(); |
|
Mouse Actions |
|
Click:
const actions = driver.actions({bridge: true }); await actions.click(element).perform(); Double click:
await actions.doubleClick(element).perform(); Right click:
await actions.contextClick(element).perform(); Drag and drop:
await actions.dragAndDrop(sourceElement, targetElement).perform(); Move to element:
await actions.move({origin: element }).perform(); |
|
Working with Alerts |
|
Switch to an alert:
const alert = driver.switchTo().alert(); Accept an alert:
await alert.accept(); Dismiss an alert:
await alert.dismiss(); Enter text in an alert:
await alert.sendKeys('Text to enter'); Retrieve alert text:
const alertText = await alert.getText(); |
|
Selenium Operations |
|
Launch a Webpage:
await driver.get('<URL>'); Click a button:
const buttonElement = await driver.findElement(By.css('<button_element_css_selector>')); await buttonElement.click(); Accept an alert pop-up:
const alert = driver.switchTo().alert(); await alert.accept(); Print the page title:
const title = await driver.getTitle(); console.log(title); Wait for an element to be visible:
const element = await driver.wait(until.elementLocated(By.id(‘<element ID>’)), 10000); Wait for an element to be clickable:
const element = await driver.wait(until.elementIsEnabled(driver.findElement(By.id(‘<element ID>’))), 10000); Implicit wait:
await driver.manage().setTimeouts({implicit: 10000 }); // 10 seconds Explicit wait:
const element = await driver.wait(until.elementLocated(By.css(‘<element_css_selector>’)), 10000); // 10 seconds Sleep:
await driver.sleep(5000); // 5 seconds or 5000 milliseconds Clear the input field text:
const inputElement = await driver.findElement(By.css('<input_element_css_selector>')); await inputElement.clear(); Disable a field (set the ‘disabled’ attribute):
await driver.executeScript(“document.querySelector(‘<element_css_selector>’).setAttribute(‘disabled’, ”);”); Enable a field (remove the ‘disabled’ attribute):
await driver.executeScript("document.querySelector('<element_css_selector>').removeAttribute('disabled');"); |
|
Selenium Grid |
|
Start the hub
java -jar selenium-server-standalone-x.y.z.jar -role hub Start a node
java -jar selenium-server-standalone-x.y.z.jar -role node -hub http://localhost:4444/grid/register Server
http://localhost:4444/ui/index.html Note: Replace "x.y.z" with the version number of your Selenium Server Standalone JAR file. |
Alternative: write tests without a single line of code
testRigor is an AI-driven, end-to-end testing solution that empowers QA professionals to create and maintain complex automated tests without requiring coding skills. This comprehensive system supports testing of various platforms, including web, mobile, and desktop applications, and is suitable for functional regression, accessibility, load, and performance testing. By leveraging plain English statements and an advanced AI engine, testRigor provides excellent scalability, minimal maintenance, and faster test execution, setting itself apart from coded automation tools such as Selenium.
Selenium test:
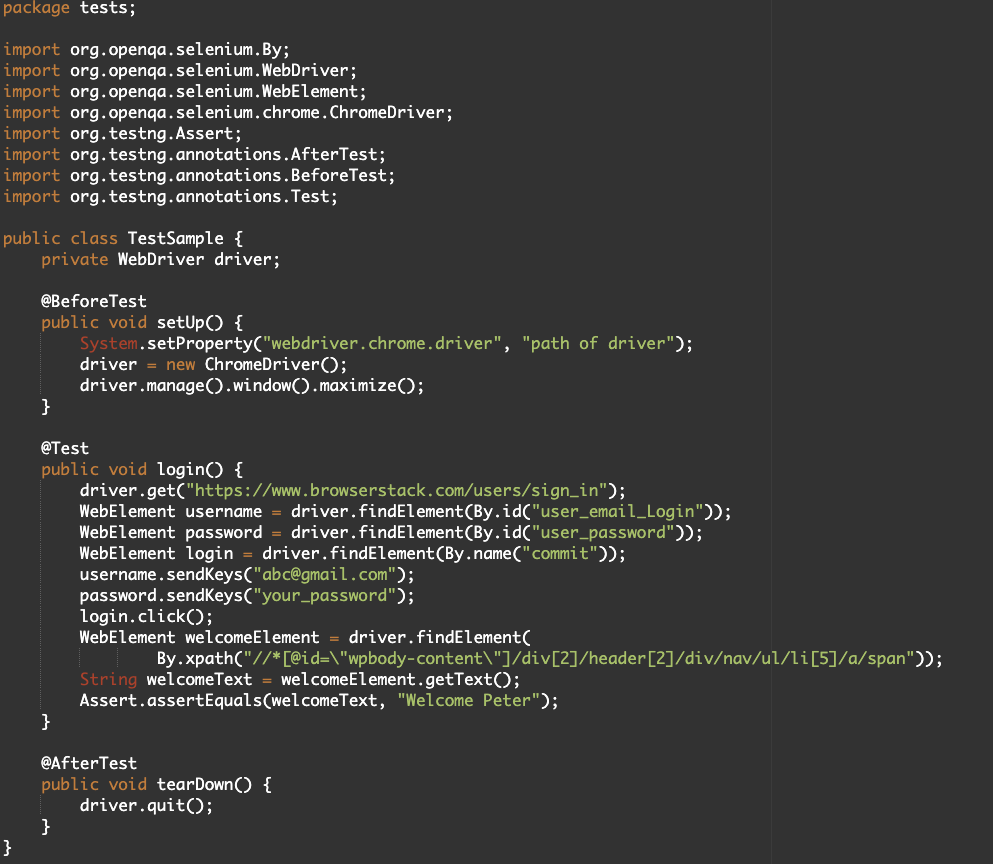
Same test in testRigor:
login check if page contains "Welcome Peter"
Organizations that have adopted testRigor have experienced significant improvements in their software testing processes, with increased test coverage, reduced defect escape rates, and accelerated release cycles. The platform not only enhances QA teams and delivery processes but also eliminates the need for maintaining testing infrastructure. The unique approach of testRigor allows for seamless collaboration among team members and ensures tests remain valid even after underlying framework changes or refactoring. This superior choice in test automation provides numerous key benefits, including cost savings, improved communication, and detailed test results for seamless debugging.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
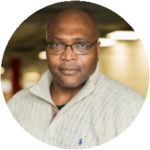