UnexpectedTagNameException in Selenium: Causes and Remedies
|
The tag name is a property used in Selenium to retrieve the tag name of a web element. Knowing the tag name helps validate or check the element type before performing actions on it.
UnexpectedTagNameException occurs in Selenium when scripts perform an action on the web element with an inappropriate tag name. This error is specific to the scenarios when WebDriver expects a particular tag name and finds another one.
Causes for UnexpectedTagNameException in Selenium
Below are the most common causes for UnexpectedTagNameException in Selenium:
Inappropriate Usage with Select Class
The Select class in Selenium provides methods to interact with dropdown menus.
These dropdown menus are expected to be defined using the <select> HTML tag.
Methods like select_by_value()
, select_by_index()
, or select_by_visible_text()
are intended to be used with <select>
elements. If these methods are used on a different type of element, like <div>
, <span>
, or <input>
, an UnexpectedTagNameException will be thrown.
from selenium import webdriver from selenium.webdriver.support.ui import Select driver = webdriver.Chrome() driver.get("http://example.com") button_element = driver.find_element_by_id("button-id") # Assuming this is a <button> element try: select = Select(button_element) except UnexpectedTagNameException: print("Caught an UnexpectedTagNameException!")
Dynamic Web Content
Sometimes, the structure of a web page might change due to the presence of dynamic content. The script is written considering a different tag and then encounters another tag during execution, causing the exception to be thrown.
Incorrect Locators
If you use incorrect locators in the script that select an element different from what is intended, the UnexpectedTagNameException will be thrown.
Remedies for UnexpectedTagNameException in Selenium
Below are the steps you can take to mitigate UnexpectedTagNameException:
Make Correct Element Selection
from selenium import webdriver from selenium.webdriver.support.ui import Select driver = webdriver.Chrome() driver.get("http://example.com") # Incorrect selection - may cause UnexpectedTagNameException # element = driver.find_element_by_id("non-select-element") # Correct selection element = driver.find_element_by_id("select-element-id") select = Select(element) # Should not raise an exception if element is a <select>
Verify Tag Name
from selenium import webdriver from selenium.webdriver.support.ui import Select driver = webdriver.Chrome() driver.get("http://example.com") element = driver.find_element_by_id("element-id") if element.tag_name == "select": select = Select(element) else: print(f"Expected a <select> element, but got <{element.tag_name}>")
Perform Exception Handling
from selenium import webdriver from selenium.webdriver.support.ui import Select from selenium.common.exceptions import UnexpectedTagNameException driver = webdriver.Chrome() driver.get("http://example.com") try: element = driver.find_element_by_id("element-id") select = Select(element) except UnexpectedTagNameException: print("Element is not a <select> tag, cannot use Select class methods.")
Use testRigor to Bypass Exceptions Forever
Selenium has been a popular test automation tool for a long time. However, today, it might act more as a roadblock in your testing journey. With the plethora of errors and exceptions to handle during script writing, Selenium does not match the Agile and DevOps methodologies of fast releases and short cycles.
Alternatives available can quickly walk in tandem with your CI/CD pipelines, and you can bid goodbye to the irritating exceptions forever. testRigor is a codeless, generative AI-based test automation tool that lets you create test cases in plain English. It doesn’t use unstable XPath and CSS locators; it is based on more humanized element identifiers known as testRigor locators.
In plain English, write the UI text you see on the screen as element identifiers. Tag names or IDs, etc., are not used as element identifiers. As a human, you can rely on a human’s way of referring to elements like sections, relative locations, tables, etc.
click on button "Delete" below "Section Name" to the right of "label" check that checkbox "Keep me signed in" is checked click on table "actions" at row "103" and column "Action"
You can see how easy it is to interact with buttons, checkboxes, and tables using testRigor. It is just plain English, and you are done! testRigor’s advanced features help efficiently execute your web, mobile, desktop, and API tests singlehandedly. It seamlessly integrates with the major test management, CI/CD, and infrastructure providers to expand your testing horizons.
Here is the list of the top testRigor’s features.
Conclusion
If you feel exhausted from troubleshooting and maintaining your Selenium scripts, it is time to shift. Use the power of NLP and AI to fasten and comprehensively improve your test automation activities. With minimum effort and time, achieve more expansive test coverage without getting trapped into errors and exceptions.
Use your precious time to build more robust tests with testRigor than in debugging and exception handling the Selenium scripts.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
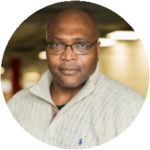