Microsoft Dynamics GP Testing
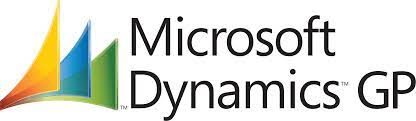
Microsoft Dynamics GP, previously known as Great Plains, goes beyond merely accounting software; it is a comprehensive enterprise resource planning (ERP) solution. With its diverse range of tools and modules, Dynamics GP caters to the needs of businesses across different sectors, including finance, manufacturing, distribution, and professional services.
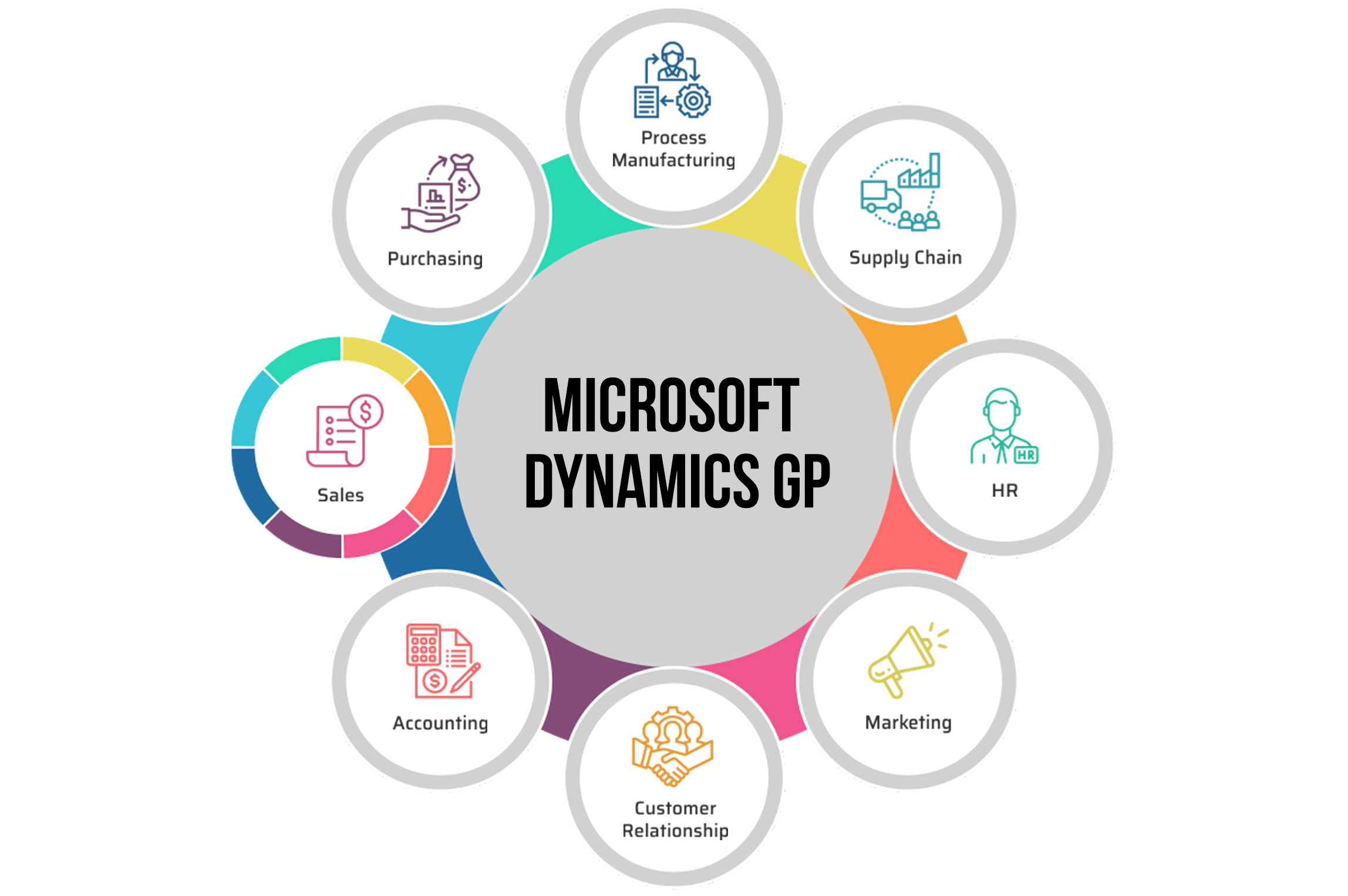
Let us explore how to test Dynamics GP and which tools to use.
How to Perform Testing on Dynamics GP?
Dynamics GP is written in Dexterity, based on C language. It has a user base with significant giants such as Tesla, Subway, Cox Media Group, AAA Northeast, etc.
Broadly, below testing types are required for a Microsoft Dynamics GP platform:
Unit Testing
It concentrates on validating the smallest testable components or units of code, such as functions or methods, independently from the rest of the application. This isolated testing approach helps identify defects early in the development process.
Let's discuss how to perform unit testing for Dynamics GP.
Dexterity Test Dictionary
Dexterity Test Dictionary is a separate test version of a Dynamics GP dictionary file that uses the Dexterity programming language. This test dictionary helps to test changes, customizations, and new features without impacting the live production environment. It's a safe sandbox where you can debug your code, replicate errors, and try solutions.
The Dexterity Test Dictionary uses a separate launch file (.set
), so it doesn't conflict with the production environment. Developers can make and test changes in this test environment and then migrate the final changes to the production dictionary once thoroughly tested and validated.
{This is the 'PRE' script for the Customer Maintenance form} local string customer_name; local string customer_class; local integer customer_credit_limit; {Get the customer information} customer_name = 'TEST CUSTOMER'; customer_class = 'CLASS1'; customer_credit_limit = 1000; {Set the fields on the form} '(L) Customer Name' of window 'Customer Maintenance' of form 'Customer Maintenance' = customer_name; '(L) Class ID' of window 'Customer Maintenance' of form 'Customer Maintenance' = customer_class; '(L) Credit Limit' of window 'Customer Maintenance' of form 'Customer Maintenance' = customer_credit_limit; {Save the customer} run script '(L) Save Button' of window 'Customer Maintenance' of form 'Customer Maintenance'; {Check that the customer was saved correctly} if '(L) Customer Name' of window 'Customer Maintenance' of form 'Customer Maintenance' <> customer_name then warning "Customer name did not save correctly."; end if; if '(L) Class ID' of window 'Customer Maintenance' of form 'Customer Maintenance' <> customer_class then warning "Customer class did not save correctly."; end if; if '(L) Credit Limit' of window 'Customer Maintenance' of form 'Customer Maintenance' <> customer_credit_limit then warning "Customer credit limit did not save correctly."; end if;
This Test Dictionary script sets up a new customer in the Customer Maintenance form and saves it. Then validates that the customer information was saved correctly. If there are any issues with the save operation, the script will raise a warning.
Integration Testing
While unit testing emphasizes testing each unit or component separately, integration focuses on testing those units when integrated. It ensures that the integrated modules work together as expected. This section discusses the tools for performing integration testing of the Dynamics GP application.eConnect
eConnect by Microsoft is specifically designed for integration with Dynamics GP. It is a middleware or connector that enables seamless interaction between external applications and Dynamics GP, allowing data exchange and transaction processing.using System; using Microsoft.Dynamics.GP.eConnect; using Microsoft.Dynamics.GP.eConnect.Serialization; public void TestCreateCustomer() { // Set up eConnect connection string string connectionString = "data source=SERVER;initial catalog=DATABASE;integrated security=SSPI;persist security info=False;packet size=4096"; // Create a new customer object taCreateCustomerRcd customer = new taCreateCustomerRcd { CUSTNMBR = "TESTCUST01", CUSTNAME = "Test Customer", // Fill out other required fields here... }; // Create an eConnect document representing the new customer eConnectType eConnect = new eConnectType { RMCustomerMasterType = new RMCustomerMasterType[] { new RMCustomerMasterType { taCreateCustomerRcd = customer } } }; // Serialize the eConnect document to an XML string string xml = eConnectSerializer.Serialize(eConnect); // Create a new eConnectMethods object using (eConnectMethods e = new eConnectMethods()) { // Submit the XML document to eConnect e.eConnect_EntryPoint(connectionString, EnumTypes.ConnectionStringType.SqlClient, xml, EnumTypes.SchemaValidationType.None, ""); } // Query the Dynamics GP database to verify the customer was created // This could be done using a SQL query or another eConnect operation // In a real test, you would also compare the new customer's data to the expected values Customer retrievedCustomer = GetCustomerFromDatabase("TESTCUST01"); Assert.IsNotNull(retrievedCustomer); } // This is a placeholder for a method that would retrieve a customer from the Dynamics GP database private Customer GetCustomerFromDatabase(string customerNumber) { // Implementation goes here... return null; }
The above code starts by creating an eConnect document representing a new customer. This document is then serialized to XML and submitted to eConnect using the eConnect_EntryPoint
method. After processing it, the test queries the Dynamics GP database to verify that the new customer was created. A real test would also check that the new customer's data matches the expected values.
Scribe
Scribe is a robust graphical data integration tool for integrating data and testing with Dynamics GP. It provides a user-friendly interface for creating integrations and supports a variety of data sources. With Scribe, you can automate data migrations, integrate applications, and synchronize data.
Scribe allows to set up data mappings using a graphical interface rather than writing code. Testing an integration in Scribe usually involves running the integration and verifying the results manually or with SQL queries or other methods.
Here is an example of how to test a CSV file for Dynamics GP integration:
Prepare Test Data: Prepare a CSV file with test data for new customers. It should include all necessary fields for creating a new customer in Dynamics GP, such as CUSTNMBR, CUSTNAME, etc.
Create the Scribe Integration: Open Scribe Workbench and create a new DTS (Data Translation Specification) file. Set the source as the CSV file and the target as Dynamics GP. In the mappings, map each column in the CSV file to the corresponding field in the Dynamics GP customer table.
Run the Integration: Run the DTS file in Scribe Workbench. Scribe will read each row from the CSV file, convert it to a customer record according to your mappings, and insert it into Dynamics GP.
Verify the Results: Open Dynamics GP and verify that new customers got created for each row in the CSV file. Check each field of the new customers to make sure they match the data in the CSV file. If there are any discrepancies, it might indicate an issue with the integration.
Cleanup: Delete the test customers from Dynamics GP and the CSV file if necessary.
SmartConnect
It is a third-party integration and testing tool provided by eOne Solutions. SmartConnect's integration process allows users to import and export data to and from Dynamics GP. It helps to test a Dynamics GP integration by setting the output to a Dynamics GP file. This feature lets you see the exact data that would be moved into Dynamics GP in XML format. This feature is helpful to check if any changes have been made before transferring the data to GP, especially when dealing with complex integrations.
End-to-end Testing
It validates the complete workflow of a system, and the objective is to simulate real user scenarios. It ensures all interconnected components of the application work together as expected end-to-end. This method detects system flow issues, including communication breakdowns and data integrity problems.
Let's review a few tools primarily used for E2E testing of Dynamics GP apps.
Microsoft EasyRepro
Microsoft's EasyRepro is an open-source framework built on Selenium for automated UI testing for Dynamics GP and Power Platform applications. It allows developers and testers to create UI tests in C# code using a WebDriver API to mimic user interactions with the application.
EasyRepro provides classes, methods, and properties that encapsulate complex UI interactions, such as authenticating with Dynamics GP, opening a record, switching forms, running dialogues, etc. These features make it easier to create automated UI tests without dealing with the complexities of the Dynamics GP UI. One of the main benefits of EasyRepro is that it helps ensure the tests written are resilient to changes in the underlying platform.
using Microsoft.Dynamics365.UIAutomation.Api; using Microsoft.Dynamics365.UIAutomation.Browser; using System; using System.Security; class Program { static void Main(string[] args) { var clientUrl = new Uri("https://your-test-url"); var username = "your-test-username"; var password = ToSecureString("your-test-password"); using (var xrmBrowser = new XrmBrowser(new BrowserOptions { BrowserType = BrowserType.Chrome })) { // Login xrmBrowser.LoginPage.Login(clientUrl, username, password); // Navigate to Sales -> Accounts xrmBrowser.Navigation.OpenSubArea("Sales", "Accounts"); // Click on the 'New' button xrmBrowser.CommandBar.ClickCommand("New"); // Fill in 'Account Name' xrmBrowser.Entity.SetValue("name", "Test Account"); // Save the new account xrmBrowser.CommandBar.ClickCommand("Save"); // Verify that the account was created xrmBrowser.Grid.Search("Test Account"); var results = xrmBrowser.Grid.GetGridItems(); if (results.Count == 0) { throw new Exception("Account creation failed"); } } } private static SecureString ToSecureString(string str) { var secureString = new SecureString(); foreach (var ch in str) { secureString.AppendChar(ch); } return secureString; } }
RSAT (Regression Suite Automation Tool)
The Regression Suite Automation Tool (RSAT) is an integrated tool Microsoft provides for testing Dynamics AX applications. Its primary function is to streamline the testing process by allowing users to record specific business tasks using the Task Recorder feature. These recorded tasks can subsequently be transformed into a collection of automated tests, creating a more efficient testing process.
Underlying each RSAT test case is an individual recording. The tool generates executable files containing C# code and an Excel file that handles test parameters. When a test executes, RSAT capitalizes on the Selenium framework to automatically launch a browser, navigate to the system, and replicate the steps from the specified recording.
However, RSAT's primary limitation is its lack of reusability. Once a test is created, it cannot be repurposed for different test suites. This drawback restricts the testing process flexibility and could impact project timelines. Additionally, it may necessitate allocating extra resources for the testing phase. You should consider this limitation when planning for project resources and schedules.
Traditional (Legacy) Automation Testing Tools
These tools are typically Selenium-based, and there are automation challenges associated with them as below:
Complex UI: Dynamics GP has a rich user interface with many fields, controls, and screens having dynamic elements. Automating UI of this caliber using legacy automation tools is challenging.
Configuration and Data Dependency: There is a significant dependency on system functionality on data interactions and configuration since they align with business processes. The data being processed is gigantic, and this causes reliance on test automation tools which are convergent in dealing with massive datasets. This data size makes the whole testing process difficult with legacy automation tools. Automation scripts must accommodate these customizations, making test maintenance more complex as customizations change over time.
Complex Module Interaction: Integration testing of the complex modules, external systems, APIs, and the communication between them is challenging to script using programming languages in traditional test automation tools. Automating end-to-end integration testing may require coordination with external systems and handling data exchanges effectively.
Performance Testing: Dynamics GP is an extensive system with many simultaneous users, data exchanges, and actions. To test the system's performance, the simulation capabilities of the test automation tool should be top-notch to match the actual user load, which may be lacking with traditional automated testing.
Security and Permissions: There are many users, roles, and permission levels in the system required for the sophisticated functionalities, and this becomes very challenging to automate for testing.
Customized Reporting: Whole Dynamics GP system sustains configuration and customizations. Reporting is heavily customized based on the business requirements and users. Automating different customizations and complex data structures in reports requires ample time and skills.
Test Data Management: First, the amount of data handled is enormous. Secondly, the system contains financial and sensitive business data. Therefore proper test data management techniques are required to collect, mask, edit, and maintain the test data, making it more difficult for legacy test automation tools.
Customization and Updates: Continuous updates and system patches keep the system up-to-date. The test automation should be robust enough to handle all these configuration changes and updates.
testRigor
testRigor is a modern AI-powered no-code automation tool that specializes in end-to-end (system) testing. It is one the fastest and most efficient ways to cover your MS Dynamics GP testing scenarios.
Here are a few distinctive features of testRigor that set it apart from other test automation tools:
- Provide only the test case title and testRigor's generative AI engine automatically creates test steps for you within seconds.
- testRigor helps to write the test scripts in plain English by eliminating the prerequisite to know any programming language. testRigor's AI converts the English test scripts to actual code using advanced Natural Language Processing (NLP).
These test creation features empower manual testers and allow business analysts and other non-technical stakeholders to create automation scripts. Additionally, it accelerates the process for manual testers to generate automation scripts.
Supports desktop testing: Your Dynamic GP testing concerns are solved efficiently by testRigor's desktop testing capabilities. testRigor single-handedly supports testing web, mobile, and desktop applications.
Self-healing tests: The issue of constant updates and test maintenance is expected in Microsoft Dynamics GP as new patches and configuration changes are introduced, given the breadth of the system. testRigor incredibly manages these issues with its automatic self-healing features. Any changes in the element attributes or application are incorporated into the test scripts automatically, saving massive maintenance effort hours.
Automatic wait times: testRigor automatically manages wait times, sparing you from manually inputting them and thus preventing "element not found" errors.
Ultra-stable: As a no-code solution, testRigor eliminates dependencies on specific programming languages. Elements are referenced as they appear on the screen, reducing reliance on implementation details and simplifying test creation, maintenance, and debugging.
Cloud-hosted: Save time, effort, and resources on infrastructure setup. With testRigor, you are ready to start writing test scripts right after signing up, boasting a speed up to 15 times faster compared to other automation tools.
Seamless integrations: Built-in integrations with CI/CD, infrastructure service providers, communication tools, and test management tools ensure a seamless and efficient testing process.
Email, call, and SMS testing: testRigor supports phone calls and SMS testing with the help of Twilio integration. It also supports email testing involving sending, receiving, and validating emails.
Take a look at the testRigor's documentation to get a better understanding of the supported functionality.login create new lead go to leads check that table at row containing stored value "lastName" and column "Status" contains "Created”
You can see how simple and clean the test script looks. The in-built login command takes care of the login automatically. Describe in plain English what you see on the screen as element locators. testRigor serves all your Dynamic GP testing needs with such simplicity, allowing anyone on the team to write and execute test cases in plain English.
See the list of testRigor's features. These features help achieve a broader test coverage before the release deadline, and save on budget. There is no need for separate testing tools and external integrations to perform Dynamic GP testing.
How to do End-to-end Testing with testRigor
Let us take the example of an e-commerce website that sells plants and other gardening needs. We will create end-to-end test cases in testRigor using plain English test steps.
Step 1: Log in to your testRigor app with your credentials.
Step 2: Set up the test suite for the website testing by providing the information below:
- Test Suite Name: Provide a relevant and self-explanatory name.
- Type of testing: Select from the following options: Desktop Web Testing, Mobile Web Testing, Native and Hybrid Mobile, based on your test requirements.
- URL to run test on: Provide the application URL that you want to test.
- Testing credentials for your web/mobile app to test functionality which requires user to login: You can provide the app’s user login credentials here and need not write them separately in the test steps then. The login functionality will be taken care of automatically using the keyword
login
. - OS and Browser: Choose the OS Browser combination on which you want to run the test cases.
- Number of test cases to generate using AI: If you wish, you can choose to generate test cases based on the App Description text, which works on generative AI.
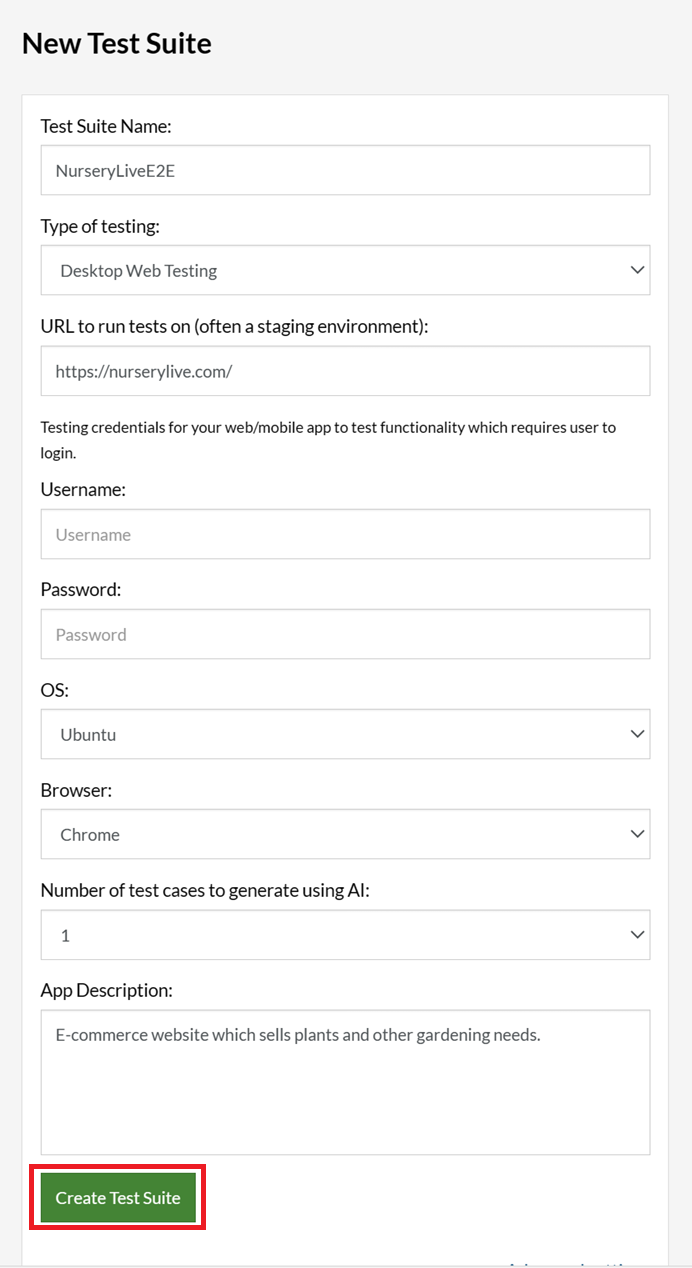
Step 3: Click Create Test Suite.
On the next screen, you can let AI generate the test case based on the App Description you provided during the Test Suite creation. However, for now, select do not generate any test, since we will write the test steps ourselves.
Step 4: To create a new custom test case yourself, click Add Custom Test Case.
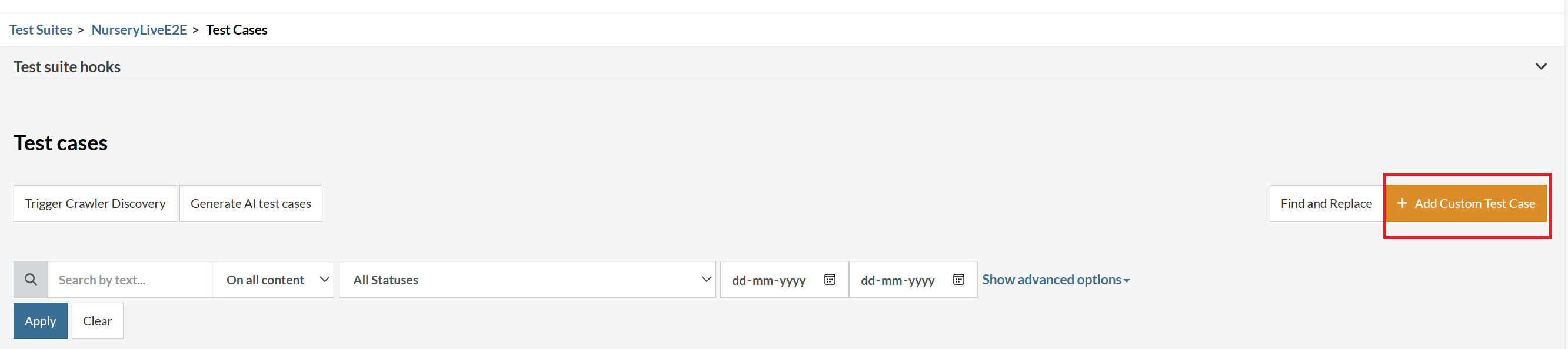
Step 5: Provide the test case Description and start adding the test steps.
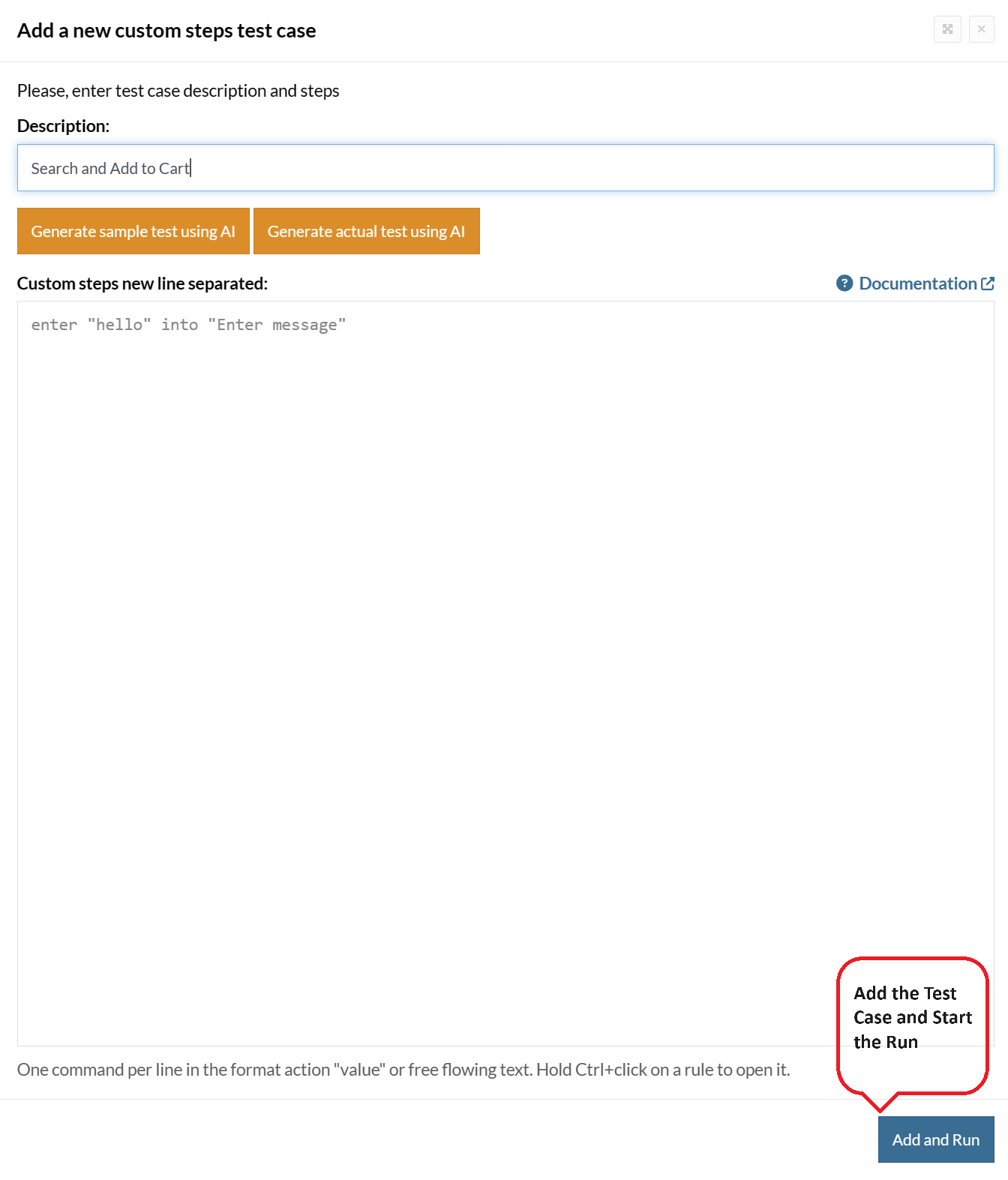
For the application under test, i.e., e-commerce website, we will perform below test steps:
- Search for a product
- Add it to the cart
- Verify that the product is present in the cart
Test Case: Search and Add to Cart
Step 1: We will add test steps on the test case editor screen one by one.
testRigor automatically navigates to the website URL you provided during the Test Suite creation. There is no need to use any separate function for it. Here is the website homepage, which we intend to test.
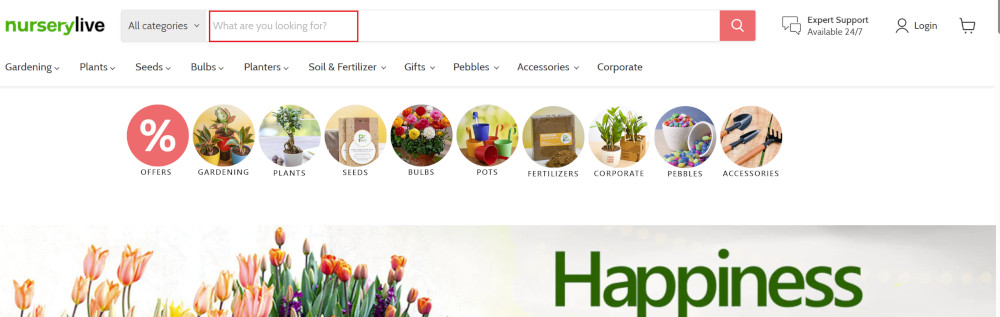
First, we want to search for a product in the search box. Unlike traditional testing tools, you can identify the UI element using the text you see on the screen. You need not use any CSS/XPath identifiers.
click "What are you looking for?"
Step 2: Once the cursor is in the search box, we will type the product name (lily), and press enter to start the search.
type "lily" enter enter
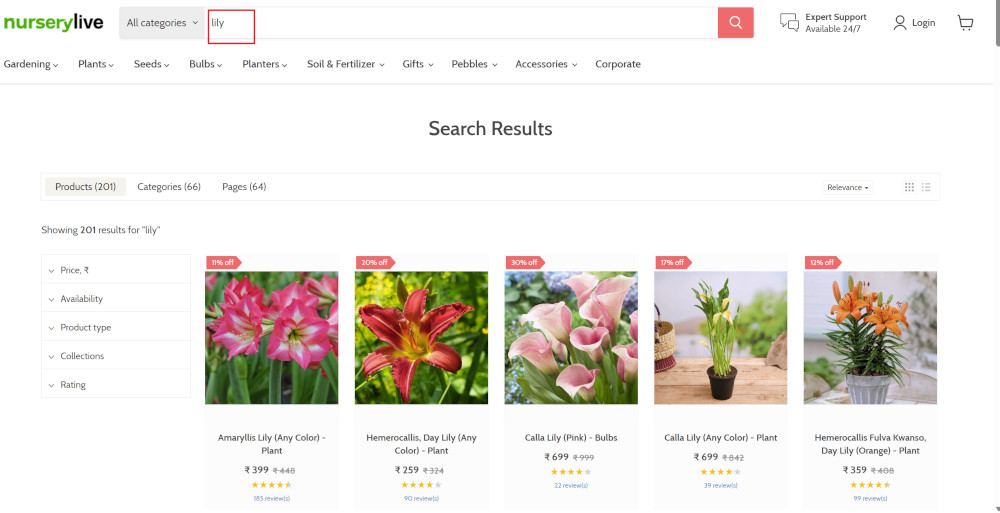
Search lists all products with the “lily” keyword on the webpage.
Step 3: The lily plant we are searching for needs the screen to be scrolled; for that testRigor provides a command. Scroll down until the product is present on the screen:
scroll down until page contains "Zephyranthes Lily, Rain Lily (Red)"
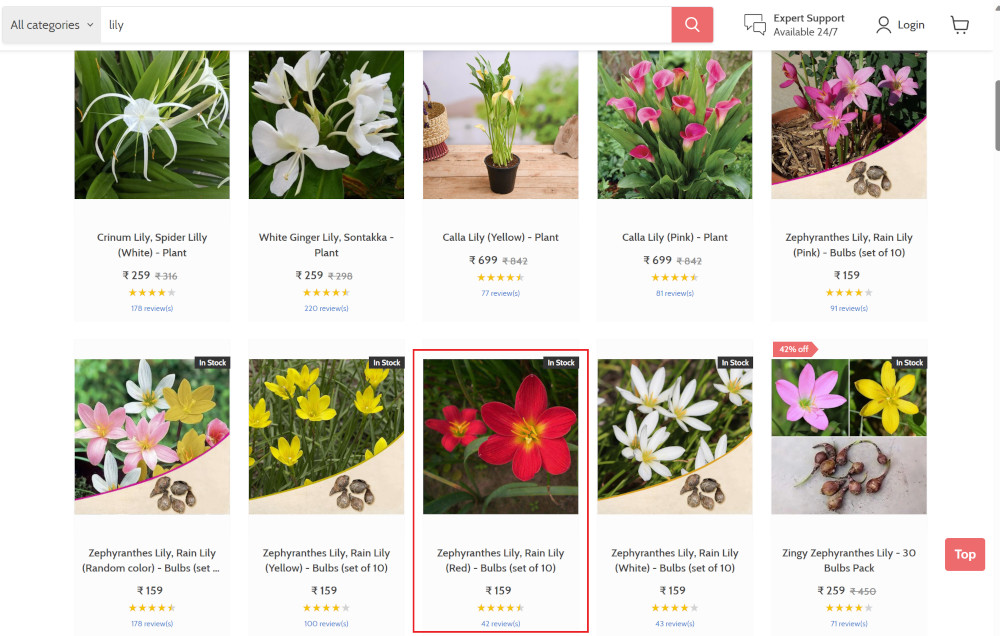
When the product is found on the screen, testRigor stops scrolling.
Step 4: Click on the product name to view the details:
click "Zephyranthes Lily, Rain Lily (Red)"
After the click, the product details are displayed on the screen as below, with the default Quantity as 1.
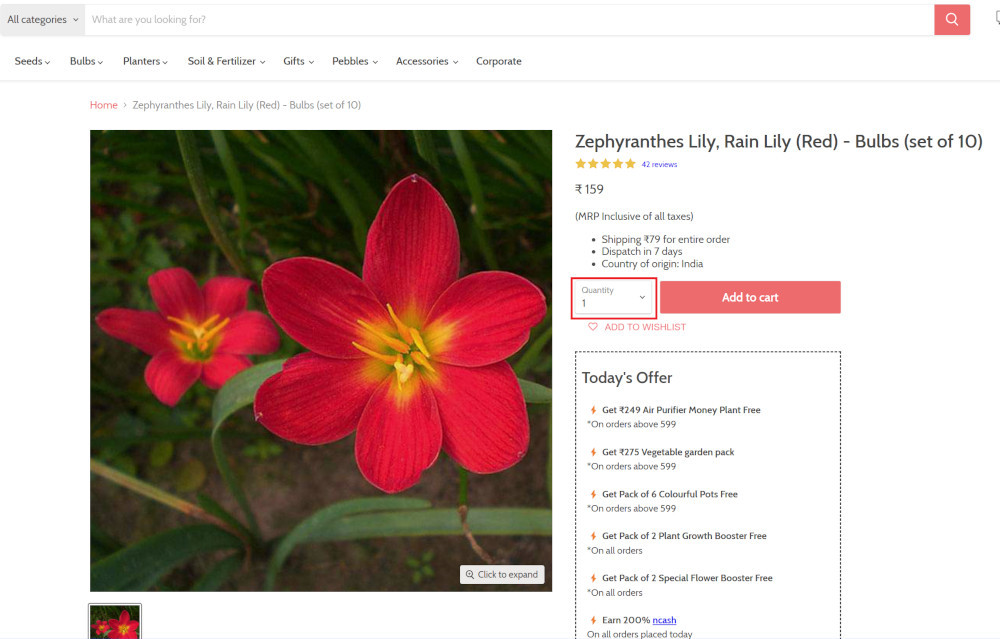
Step 5: Lets say, we want to change the Quantity to 3, so here we use the testRigor command to select from a list.
select "3" from "Quantity"
click "Add to cart"
The product is successfully added to the cart, and the “Added to your cart:” message is displayed on webpage.
Step 6: To assert that the message is successfully displayed, use a simple assertion command as below:
check that page contains "Added to your cart:"
Step 7: After this check, we will view the contents of the cart by clicking View cart as below:
click "View cart"
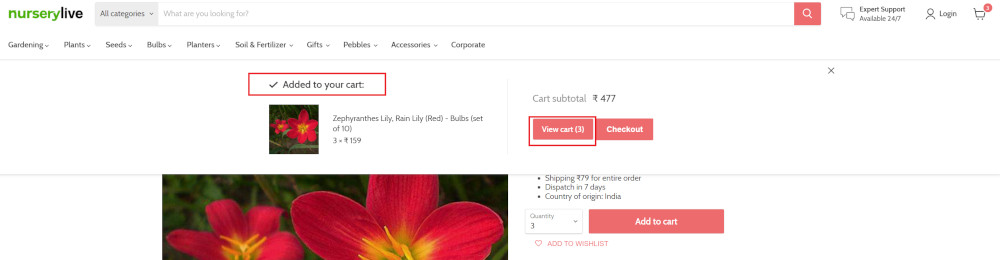
Step 8: Now we will again check that the product is present in the cart, under heading “Your cart” using the below assertion. With testRigor, it is really easy to specify the location of an element on the screen.
check that page contains "Zephyranthes Lily, Rain Lily (Red)" under "Your cart"
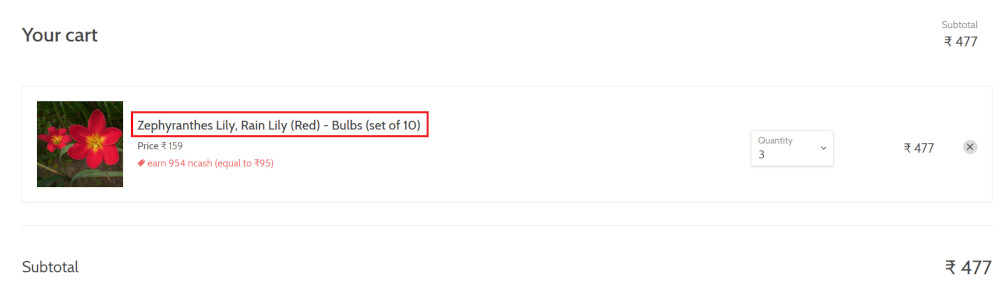
Complete Test Case
Here is how the complete test case will look in the testRigor app. The test steps are simple in plain English, enabling everyone in your team to write and execute them.
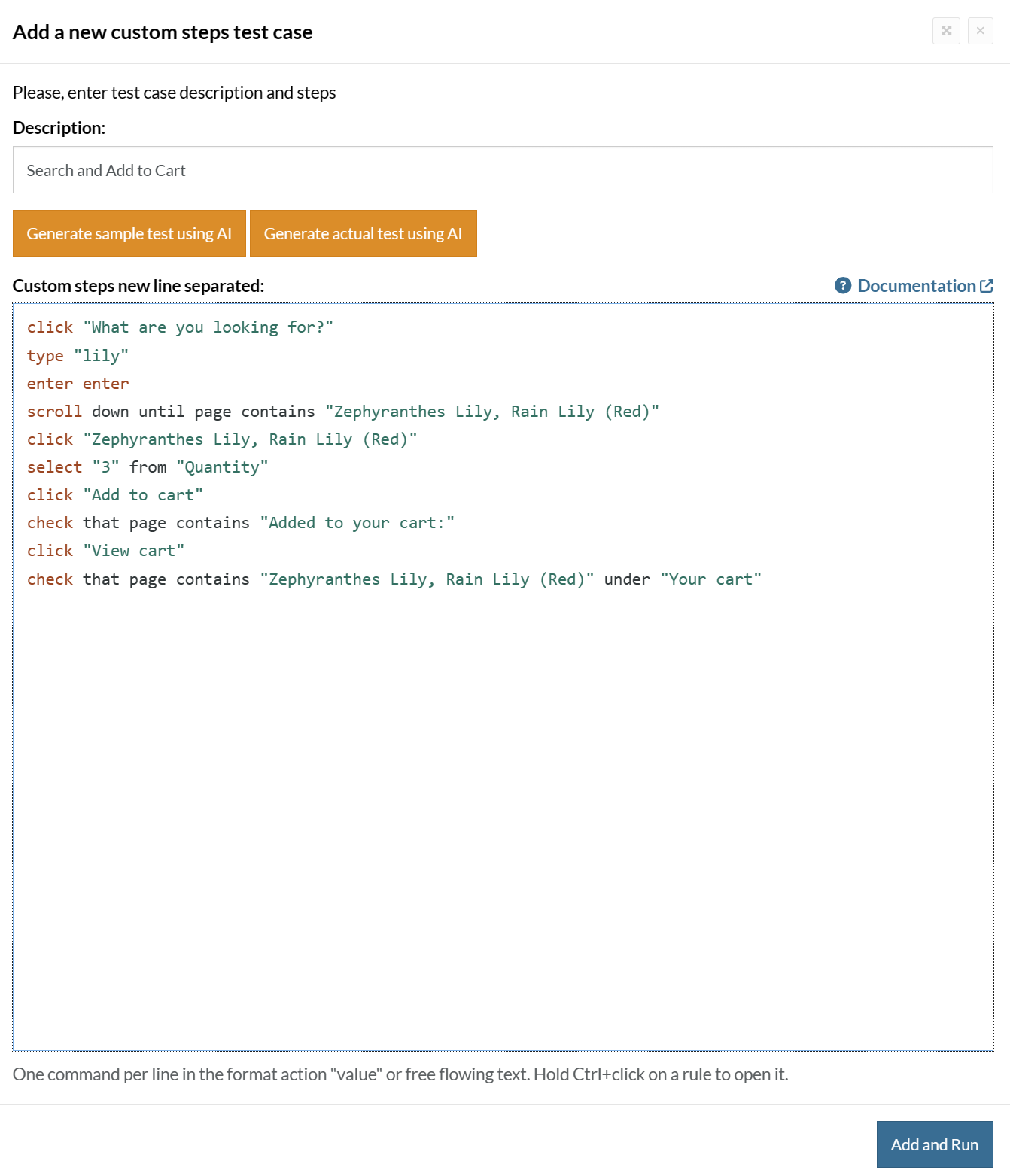
Click Add and Run.
Execution Results
Once the test is executed, you can view the execution details, such as execution status, time spent in execution, screenshots, error messages, logs, video recordings of the test execution, etc. In case of any failure, there are logs and error text that are available easily in a few clicks.
You can also download the complete execution with steps and screenshots in PDF or Word format through the View Execution option.
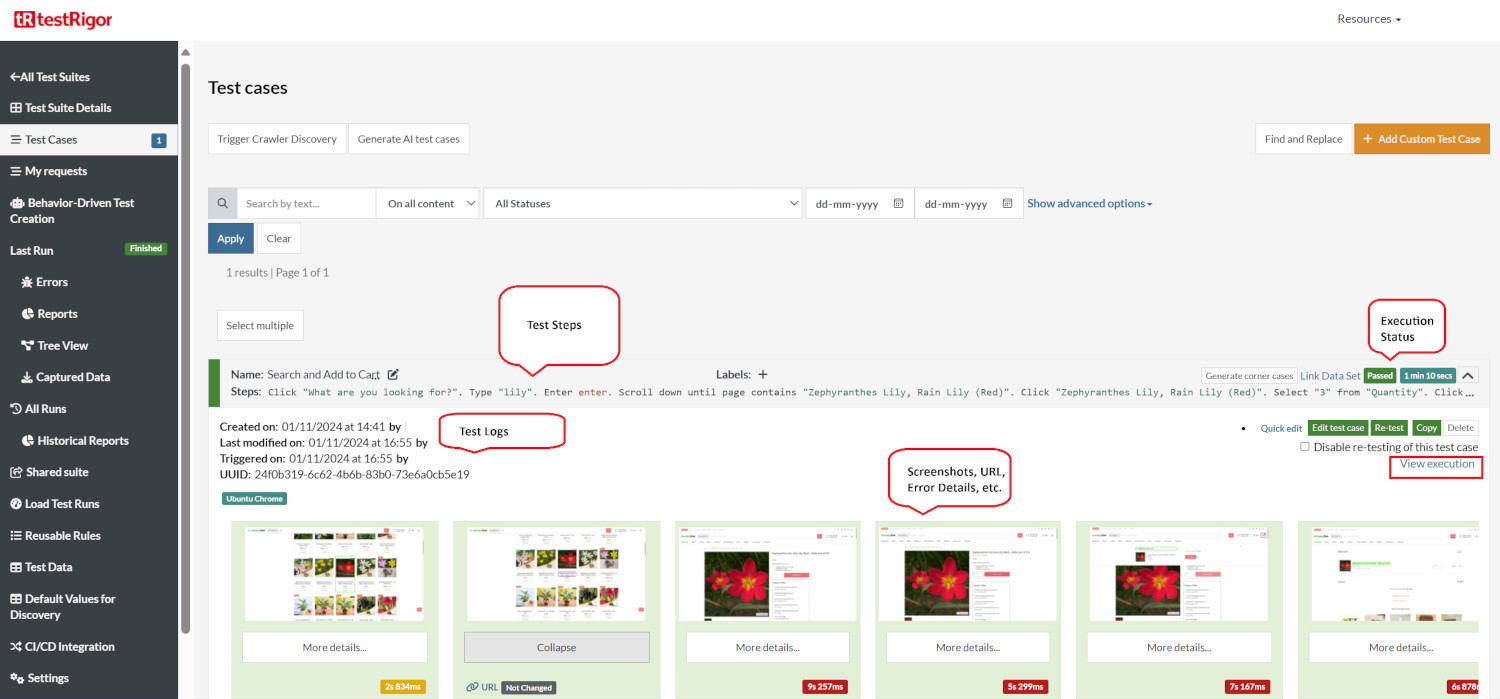
testRigor’s Capabilities
Apart from the simplistic test case design and execution, there are some advanced features that help you test your application using simple English commands.
- Reusable Rules (Subroutines): You can easily create functions for the test steps that you use repeatedly. You can use the Reusable Rules to create such functions and call them in test cases by simply writing their names. See the example of Reusable Rules.
- Global Variables and Data Sets: You can import data from external files or create your own global variables and data sets in testRigor to use them in data-driven testing.
- 2FA, QR Code, and Captcha Resolution: testRigor easily manages the 2FA, QR Code, and Captcha resolution through its simple English commands.
- Email, Phone Call, and SMS Testing: Use simple English commands to test the email, phone calls, and SMS. These commands are useful for validating 2FA scenarios, with OTPs and authentication codes being sent to email, phone calls, or via phone text.
- File Upload/ Download Testing: Execute the test steps involving file download or file upload without the requirement of any third-party software. You can also validate the contents of the files using testRigor’s simple English commands.
- Database Testing: Execute database queries and validate the results fetched.
testRigor enables you to test web, mobile (hybrid, native), API, and desktop apps with minimum effort and maintenance.
Additional Resources
- Access testRigor documentation to know about more useful capabilities
- Top testRigor’s features
- How to perform end-to-end testing
Conclusion
Microsoft Dynamics GP is a vast application; comprehensive testing at various levels is critical for organizations. It is imperative to rectify potential issues before they impact your operations, business, and customer base. Utilizing specialized and intelligent automation testing solutions enhances testing quality and provides excellent test coverage and efficiency. Frequent configuration changes and patches make it compulsory to have robust test automation in place to keep your business running smoothly.
Effective testing practices contribute to a seamless Dynamics GP deployment, allowing your business to manage its financials, supply chain, human resources, and other core processes confidently and precisely. testRigor is a single solution for all your Dynamics GP automation testing needs, which helps you attain all these milestones with perfection, ease, and within budget and timelines.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
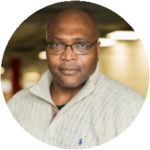