|
Heather Brooks
|
What are pop-up alerts?
An alert on a web page is a pop-up window that appears on a website to provide a user with important information or to prompt them to take some action. Alerts can be triggered by different events, such as a user clicking a button or a website encountering an error.
Alerts can be used for a variety of purposes, such as to confirm that a user has successfully completed an action, to provide an error message when something goes wrong, to notify a user of a time-sensitive message, or to request user input.
Some sources mention that there's a difference between alerts and popups, but we would argue that they're essentially the same thing. Regardless, we will discuss all the main types of alerts/pop-ups in this article.
Let's see the alert examples below and how to handle them using Selenium.
Types of Alerts:
There are various types of alerts. Let's see examples below:
Simple Alert
A simple alert will give some information or a warning message on the screen as below with an 'OK' button which needs to be accepted.
Prompt Alert
This alert will ask for some information to be provided or give information before any operation can be performed on the parent window.
Confirmation Alert
This alert is used for task confirmation.
Permission Alert (including Location, Notification, etc.)
For example, when a web application requires access to the camera and microphone, the following pop-ups will appear.
Now that we have seen the above few examples of pop-up alerts, let's find out how to handle them using Selenium.
How to handle alerts?
Using Alert interface:
Alert interface provides below methods to handle alerts:
1. void accept()- driver.switchTo().alert().accept(); //accept alert
2. void dismiss()- driver.switchTo().alert().dismiss(); //dismiss alert
3. void sendKeys(String strToSend)-driver.switchTo().alert().sendKeys(strToSend);// send text to alert input
4. String getText()- driver.switchTo().alert().getText();//get text from alert
Let's take an example of handling a prompt alert on the Rediff website. First, you'll need to make sure that all the Selenium prerequisites are installed (refer to the Selenium
test example article). Once that is done, let's write sample code and execute it to see the test results.
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.util.concurrent.TimeUnit;
public class HandlePromptAlert {
public static WebDriver driver;
public static void main(String[] args) throws InterruptedException {
WebDriverManager.chromedriver().setup();
ChromeOptions options = new ChromeOptions();
options.addArguments("--start-maximized");
driver= new ChromeDriver(options);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
System.out.println("Launch Browser");
driver.get("https://mail.rediff.com/cgi-bin/login.cgi");
driver.findElement(By.className("signinbtn")).click();
System.out.println("Handle Alert");
Alert alert= driver.switchTo().alert();
String alertMessage = driver.switchTo().alert().getText();
System.out.println(alertMessage);
Thread.sleep(3000);
alert.accept();
System.out.println("Alert Handled");
driver.quit();
}
}
In the above example, using
Alert alert= driver.switchTo().alert();
we are switching the control from the parent window to the alert window. Then using
String alertMessage = driver.switchTo().alert().getText();
we are capturing the alert message and storing it in a variable to be displayed using the print method. With
alert.accept()
, we are accepting and handling the alert.
Sample test result:
ChromeDriver was started successfully.
[1678422626.099][WARNING]: virtual void DevToolsClientImpl::AddListener(DevToolsEventListener *) subscribing a listener to the already connected DevToolsClient. Connection notification will not arrive.
Mar 10, 2023 10:00:26 AM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Detected dialect: W3C
Launch Browser
Handle Alert
Please enter a valid user name
Alert Handled
Process finished with exit code 0
Let's now consider another example of handling a pop-up.
Disabling notifications using ChromeOptions
Let's take an example of how to handle a permission pop-up by disabling notifications using ChromeOptions in Google Meet:
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.util.concurrent.TimeUnit;
public class HandlePopups {
public static WebDriver driver;
public static void main(String[] args) {
WebDriverManager.chromedriver().setup();
ChromeOptions options = new ChromeOptions();
options.addArguments("--start-maximized");
options.addArguments("--disable-notifications");
driver= new ChromeDriver(options);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://meet.google.com/iwe-xvak-bhs");
driver.quit();
}
}
In the above example,
options.addArguments("--disable-notifications")
helps to disable the browser-based pop-ups.
Using methods getWindowHandles() and getWindowHandle()
The below methods can be used to handle pop-ups or unexpected web dialogs using Selenium:
1. Driver.getWindowHandles();
2. Driver.getWindowHandle();
Let's consider an example of how to handle a separate web-based popup using getWindowHandles() on a demo website. In the below scenario (though it's quite rare), three child windows are closed, followed by the parent or main window.
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.util.Iterator;
import java.util.Set;
import java.util.concurrent.TimeUnit;
public class HandleWebBasedPopup {
public static WebDriver driver;
public static void main(String[] args) {
WebDriverManager.chromedriver().setup();
ChromeOptions options = new ChromeOptions();
options.addArguments("--start-maximized");
driver= new ChromeDriver(options);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("http://www.dummysoftware.com/popupdummy_testpage.html");
String mainWindowHandle= driver.getWindowHandle();
Set<String> allWindowHandles= driver.getWindowHandles();
Iterator<String> iterator= allWindowHandles.iterator();
while(iterator.hasNext()){
String childWindow = iterator.next();
if(!mainWindowHandle.equalsIgnoreCase(childWindow)){
driver.switchTo().window(childWindow);
System.out.println("Close child wins:"+ driver.getTitle());
driver.close();
}
}
driver.switchTo().window(mainWindowHandle);
System.out.println("Close parent window:"+ driver.getTitle());
driver.close();
}
}
In the above example using
String mainWindowHandle= driver.getWindowHandle();
we are getting the handle of the parent window and storing it in a variable mainWindowHandle.
Next, using
Set<String> allWindowHandles = driver.getWindowHandles();
we get the handles of all the windows that are currently open which returns the set of handles. We are iterating through the handles using
Iterator<String> iterator= allWindowHandles.iterator();
and based on the condition applied and using
driver.switchTo().window(childWindow);
we switch to the desired child window and close it. Finally, we switch to the parent window using
driver.switchTo().window(mainWindowHandle);
and close it.
Sample test result:
ChromeDriver was started successfully.
[1678424740.624][WARNING]: virtual void DevToolsClientImpl::AddListener(DevToolsEventListener *) subscribing a listener to the already connected DevToolsClient. Connection notification will not arrive.
Mar 10, 2023 10:35:40 AM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Detected dialect: W3C
Close child wins:Screen 2
Close child wins:Screen 3
Close child wins:Screen 1
Close parent window:PopupDummy! Popup Test Page
Process finished with exit code 0
Using Robot class
What is Robot class?
A Robot class in Selenium is used to generate and handle native system input events for test automation, self-running demos, and other applications where control is needed over the mouse and keyboard. It enables automated testing for implementations of the Java platform.
When and why to use Robot class?
WebDriver cannot handle the OS popups. So Robot class was introduced in Java version 1.3. It can handle the keyboard and mouse functions, but tests can be flaky and unstable - since it uses coordinates.
Sample code below with Robot class:
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.awt.*;
import java.awt.event.InputEvent;
import java.util.concurrent.TimeUnit;
public class HandlePopupUsingRobot {
public static WebDriver driver;
public static void main(String[] args) throws InterruptedException, AWTException {
WebDriverManager.chromedriver().setup();
ChromeOptions options = new ChromeOptions();
options.addArguments("--start-maximized");
driver= new ChromeDriver(options);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("Webpage link");
driver.manage().window().maximize();
Thread.sleep(2000);
driver.findElement(By.id("Popup")).click();
Robot robot= new Robot();
robot.mouseMove(400,10);
robot.mousePress(InputEvent.BUTTON1_DOWN_MASK);
Thread.sleep(2000);
robot.mouseRelease(InputEvent.BUTTON1_DOWN_MASK);
Thread.sleep(2000);
driver.quit();
}
}
In the above example, we can see that the use of coordinates using
robot robot.mouseMove(400,10);
can make tests unstable - which might run fine in one iteration but these tests are bound to fail in subsequent iterations making them not reliable.
Selenium WebDriver limitations of popup alert handling
When working with alerts and pop-ups using Selenium, unexpected situations can arise that can be difficult to handle. This is because each scenario is unique, and there is no clear-cut solution for dealing with pop-ups, as demonstrated in the examples provided. Pop-ups can appear at random, making it challenging to fix issues programmatically. It is important to handle pop-ups properly to continue with the test execution, as failure to do so can prevent further operations on the parent window. To address these challenges, it is advisable to consider alternative solutions such as testRigor. Let's take a closer look at how testRigor handles these situations.
How does testRigor handle pop-ups and alerts?
If you're not familiar with testRigor, it is a codeless solution that was created to make your life easier. That, of course, includes scenarios such as encountering and handling pop-up alerts. testRigor can identify an alert via visible text using a feature called OCR, which works well even for scenarios where the pop-up automatically disappears from the screen in a few seconds. To use this feature, we just need to add the command 'using OCR' or 'using OCR only'. The difference between the two options is that for 'OCR only', testRigor will only consider the visual part and won't pay any attention to identifying element locators for a given command. Let's see an example test below.
Using OCR
Let's take the same example of how to handle a prompt alert and pop-up using OCR for a pizza delivery application.
check that page contains "LOCATE ME"
check that page contains "We would like to send you some cheesy offers!"
check that page contains "Notifications can be turned off anytime from browser"
check that page contains "Don't Allow"
click "Allow" using OCR only
click "ORDER NOW"
check that page contains "BESTSELLERS"
Comparison of pop-up and alerts handling by Selenium vs testRigor
Let's now compare both tools for alert handling
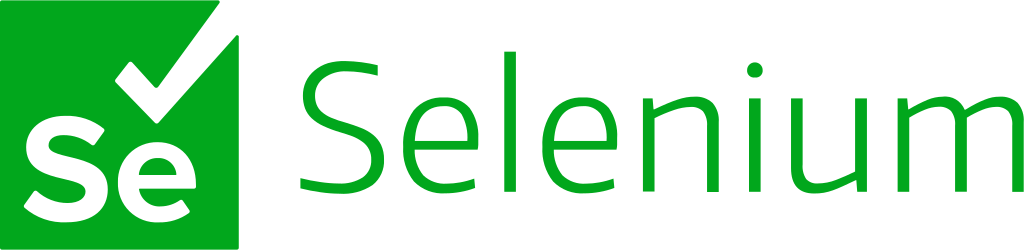 |
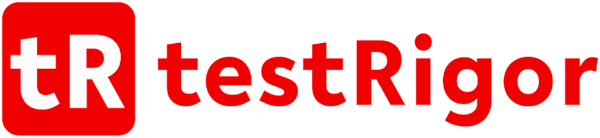 |
Needs a lot of coding to handle alerts and pop-ups |
No coding is required, plain English executable specifications are enough to handle tricky scenarios |
There is a list of prerequisite steps, such as installation of the needed dependencies and jars, and configurations to make Selenium up and running after which code for the feature can be developed and tested. |
No prerequisites are needed since testRigor is a cloud-hosted tool. Users can start writing their test automation starting from day one. |
Different scenarios are handled with different coding logic |
'Using OCR' or 'using OCR only' covers all pop-up/alert scenarios |
Screenshots with test results need to be configured separately |
Screenshots for each test step are automatically generated during the execution |
Use of certain classes (like Robot class) can make tests flaky |
Tests are ultra-stable and robust |
Conclusion
To conclude, Selenium might be a good choice for handling scenarios for pop-ups and alerts - but it can sometimes be tricky, plus such tests can be unstable. Conversely, testRigor makes the task a lot easier and can easily handle pop-up/alert test automation scenarios. You're welcome to try it out yourself!