7 Most Common Engineering Bugs and How to Avoid Them
|
Software bugs may seem harmless when seen in isolation. However, even a seemingly minor software error can have catastrophic consequences when it goes undetected.
One infamous example is the Ariane 5 rocket failure that occurred during its maiden flight on June 4, 1996. The rocket exploded just 37 seconds after launch, resulting in the total loss of the vehicle and its payload.
The root cause of the failure was a software overflow error in the rocket’s inertial reference system (IRS). The error occurred when the IRS attempted to convert a 64-bit floating-point number into a 16-bit signed integer, which was necessary for compatibility with older software components.
The error type involved was software overflow, a common programming mistake that occurs when a numerical value exceeds the maximum or minimum value that can be represented in a given data type.
Let’s take a look at some of the most common engineering bugs and ways to avoid them.
Types of Engineering Bugs
Bugs in software development can be categorized in several ways, but here are some of the most common types:
- Syntax errors: They are mistakes in the code that violate the grammar rules of the programming language, such as missing semicolons, unmatched brackets, or incorrect keywords. The compiler or interpreter typically catches these errors and prevents the code from running.
- Logical errors: These errors occur when the code compiles and runs but produces incorrect results due to flaws in the logic. They can be challenging to detect since the program doesn’t crash, but the output is wrong.
- Runtime errors: These are errors that occur while the program is running. They are often caused by invalid operations, such as dividing by zero or accessing out-of-bounds array elements. These usually cause the program to crash or behave unexpectedly.
- Performance bugs: These are issues that cause the application to perform poorly, such as slow response times or excessive memory usage. They can lead to user dissatisfaction, especially in resource-intensive applications.
- Concurrency bugs: These errors occur in multi-threaded or distributed systems when multiple threads or processes access shared resources improperly. Common issues include race conditions, deadlocks, and livelocks.
- Security bugs: These are vulnerabilities that can be exploited by attackers to compromise the system, such as SQL injection, cross-site scripting (XSS) or buffer overflows. They can lead to data breaches, unauthorized access and loss of sensitive information.
- Integration bugs: These are issues that arise when different modules or systems interact, often due to mismatched expectations about data formats or protocols. These can lead to failures in data exchange or functionality across systems.
- Compatibility bugs: These are errors that occur when software does not work as intended on different devices, browsers, or operating systems. These can hinder user experience, especially in web applications.
- Usability bugs: These are problems related to the user interface or user experience that make the application difficult to use. These bugs can lead to user frustration and decreased adoption of the software.
- Data bugs: These are issues arising from incorrect or corrupted data inputs or outputs, which can stem from various sources like database errors or improper data validation. These can lead to inaccurate information being displayed or processed.
Top 7 Engineering Bugs
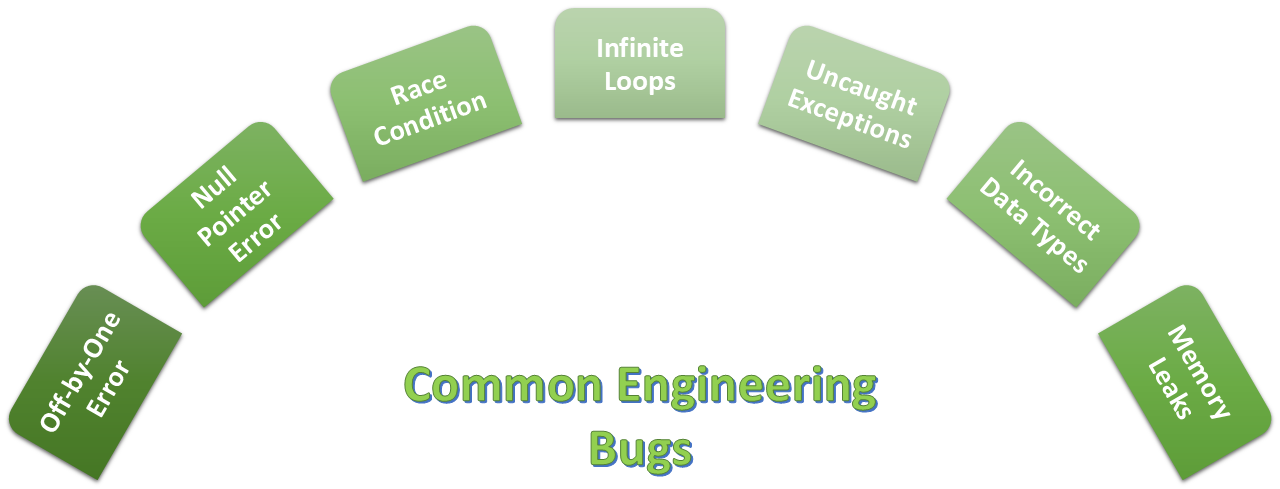
Off-by-one error
Off-by-one errors are a common type of programming mistake that occurs when a loop iterates one time too many or one time too few. This often happens with indexing in arrays or lists where the programmer mistakenly uses the wrong boundary condition.
Common scenarios where this occurs:
- In loop constructs (for, while)
- When accessing elements in arrays or lists
- In mathematical calculations involving ranges
Real-world example
Scenario: E-commerce inventory management
Imagine an e-commerce company that needs to track its inventory levels for various products. The company has an automated system to alert warehouse staff when inventory levels fall below a certain threshold to ensure timely restocking.
# Inventory levels for each product inventory_levels = [5, 10, 0, 3, 7] threshold = 3 # Check inventory levels and send alerts for i in range(len(inventory_levels)): # Off-by-one error if incorrectly implemented if inventory_levels[i] < threshold: send_alert(product_id=i) # Alert for restocking
for i in range(len(inventory_levels) - 1): # Off-by-one error
In this scenario, if the inventory levels are [5, 10, 0, 3, 7], the developer’s logic would lead to the loop checking only the first four products (0 to 3 indices). This means the product at index 4 (inventory level 7) would never be checked, resulting in no alert sent for the product with 3 units left in stock. This can lead to stockouts as the system would fail to alert the warehouse staff about the need to restock a product which can result in lost sales, unhappy customers, and damage to the company’s reputation.
How to prevent off-by-one errors
- Clear boundary understanding: Always clarify the start and end points when dealing with loops. Remember that many programming languages use zero-based indexing, meaning that the first element is at index 0.
- Code reviews: Conduct regular code reviews to catch logical errors. Having a second pair of eyes can often spot off-by-one mistakes.
- Use descriptive variable names: Use names that describe the intended logic (e.g., numElements instead of length) to reduce confusion about what the variable represents.
- Comments and documentation: Write comments that explicitly state the intended loop behavior. This helps clarify the logic for future reference or for other developers.
- Automated unit testing: Implement unit tests that cover edge cases, especially those involving boundaries. For instance, tests should include scenarios where the array is empty, contains one element, and includes the maximum number of expected elements.
- Visual debugging: Use debugging tools that allow you to step through the code and observe the values of variables at each iteration. This can help identify where the logic diverges from expectations.
Null pointer exceptions
A Null Pointer Exception (NPE) occurs when a program attempts to use an object reference that has not been initialized or has been set to null. This error typically arises when accessing methods or properties of an object that doesn’t exist which leads to application crashes and unstable behavior.
Real-world example
Scenario: Customer Relationship Management (CRM) software
Imagine a CRM application used by a sales team to track customer interactions and manage leads. The application retrieves customer data from a database, and one of its features is sending follow-up emails based on customer interactions.
class Customer: def __init__(self, name, email): self.name = name self.email = email def send_follow_up_email(customer): # Attempting to send an email without checking if the customer object is null email_body = f"Hello {customer.name}, thank you for your interest!" send_email(customer.email, email_body) # Simulating a scenario where a customer might not be properly initialized customer = None # This should be fetched from a database send_follow_up_email(customer) # This will raise a Null Pointer Exception
When the sales team attempts to send a follow-up email to a customer who has not been properly initialized, the application crashes with a null pointer exception. This disrupts the workflow and prevents the sales team from performing their tasks. If the sales team cannot follow up with leads due to crashes caused by NPEs, potential sales opportunities could be lost which will impact overall revenue. Frequent crashes and errors can lead to user frustration, which will diminish trust in the software and potentially lead to the abandonment of the application.
How to prevent null pointer exceptions
- Null checks: Implement checks to ensure that an object is not null before accessing its properties or methods.
- Use optional types: In languages that support them (like Java with Optional), consider using optional types to represent values that may or may not be present. This encourages handling the absence of values explicitly.
- Default values: When initializing objects, provide default values to avoid null references. For example, create a default customer object if the actual customer cannot be retrieved.
- Error handling: Implement robust error handling using try-catch blocks to manage exceptions gracefully. This can prevent the application from crashing and allow for logging and user notifications.
- Unit testing: Write unit tests that include scenarios for null inputs. This helps ensure that the application behaves correctly when encountering unexpected null values.
- Code reviews: Encourage regular code reviews where peers can check for potential null reference issues. This provides an extra layer of scrutiny before the code is deployed.
- Use of IDE features: Utilize Integrated Development Environment (IDE) features that can help identify potential null pointer issues such as static analysis tools and warnings.
Race conditions
A race condition occurs in a multi-threaded or concurrent programming environment when two or more threads or processes access shared resources simultaneously, and the outcome depends on the order of execution. If not properly managed race conditions can lead to unpredictable behavior, data corruption or system crashes.
Real-world example
Scenario: Online banking system
Consider an online banking system where users can transfer money between accounts. The system allows simultaneous transactions to be processed to improve user experience.
Here’s how a race condition might occur:
- Account balance: A user wants to transfer $100 from their account (Account A) to another account (Account B) which currently has a balance of $50.
-
Concurrent transactions:
- Transaction 1 (T1): A user initiates a transfer of $100 from Account A to Account B.
- Transaction 2 (T2): At the same time, another user attempts to transfer $30 from Account B to their own account.
-
Execution steps:
- Both transactions read the current balance of Account B (which is $50) almost simultaneously.
- T1 checks if Account A has enough funds (which it does) and proceeds to deduct $100.
- T2 also checks the balance of Account B and sees $50 which is sufficient to transfer $30.
- Both transactions proceed which leads to an inconsistency where Account B ends up being overdrawn, which violates banking rules.
The final balance in Account B may reflect incorrect amounts which can cause confusion and financial discrepancies. If users encounter issues such as incorrect balances or failed transactions, it can erode trust in the banking system, potentially leading to loss of customers. Moreover, financial institutions are subject to strict regulations. Race conditions leading to financial discrepancies can result in legal repercussions and penalties.
How to prevent race conditions
- Locking mechanisms: Use locking mechanisms to ensure that only one thread can access the critical section of code that modifies shared resources at a time.
- Atomic operations: Use atomic operations to read and write shared data. This ensures that operations are complete without interruption, which prevents inconsistencies.
- Thread-safe data structures: Utilize thread-safe data structures provided by many programming languages or frameworks, which manage internal synchronization automatically.
- Avoid shared state: Where possible, design systems to minimize shared state. Immutable data structures or copies of data can reduce the need for synchronization.
- Transaction management: Implement transaction management systems that can roll back transactions if an error occurs, thus ensuring data integrity.
- Testing for race conditions: Conduct thorough testing: functional and non-functional. This includes stress testing and race condition testing to identify potential issues under concurrent loads.
- Code reviews: Regularly review code for potential race conditions, especially in areas that handle shared resources or critical transactions.
Memory leaks
A memory leak occurs when a program allocates memory for use but fails to release that memory back to the system after it is no longer needed. Over time, memory leaks can consume an increasing amount of system resources that can lead to decreased performance, application crashes or system instability.
Real-world example
Scenario: Customer support application
Imagine a customer support application that handles support tickets for a large organization. The application is designed to allow agents to view and respond to customer inquiries. The application dynamically loads customer data and ticket information whenever an agent opens a support ticket. A developer inadvertently writes code that loads customer data into memory but fails to release that memory after the ticket is closed.
class SupportTicket: def __init__(self, customer_data): self.customer_data = customer_data # Customer data loaded into memory # Function to open a ticket def open_ticket(customer_data): ticket = SupportTicket(customer_data) # Ticket processing logic # Forgetting to delete or free the ticket object when done # Example usage for ticket_id in range(10000): # Processing many tickets open_ticket(load_customer_data(ticket_id)) # Memory used for each ticket is not released
As the application continues to run and more tickets are processed without releasing memory, the application may slow down due to high memory usage. Eventually, the memory usage may exhaust available resources and lead to crashes. Customer support agents may find themselves unable to access tickets, disrupting business operations.
Frequent crashes or slow performance can lead to frustration among customer support agents, negatively impacting their productivity and the overall customer experience. Moreover, if the organization relies on cloud services to host the application, memory leaks can lead to increased costs due to higher resource consumption and the need for scaling.
How to prevent memory leaks
- Proper memory management: Always ensure that allocated memory is released once it is no longer needed. In languages like C or C++, this means using free() or delete.
- Automatic garbage collection: Use programming languages with automatic garbage collection (e.g., Java, Python) that help manage memory allocation and deallocation. However, developers should still be aware of how objects are referenced and ensure they are no longer needed.
- Weak references: Utilize weak references when dealing with caches or observer patterns to allow the garbage collector to reclaim memory when objects are no longer in use.
- Memory profiling tools: Use memory profiling tools (such as Valgrind for C/C++ or memory profilers in integrated development environments) to identify and analyze memory leaks during the development and testing phases.
- Code reviews: Conduct regular code reviews to ensure best practices for memory management are being followed and that potential memory leaks are identified early.
- Unit testing: Implement unit tests that specifically check for memory leaks, especially in areas that handle dynamic memory allocation.
- Monitor application performance: Continuously monitor application performance in production to identify unusual spikes in memory usage. Use performance monitoring tools to track memory usage over time.
Infinite loops
An infinite loop occurs when a sequence of instructions in a program continues to execute indefinitely because the terminating condition is never met. This can happen due to logical errors in loop constructs and lead to unresponsive applications or excessive resource consumption.
Real-world example
Scenario: E-commerce checkout process
Consider an e-commerce platform where users can add items to their cart and proceed to checkout. During the checkout process, a loop is intended to ensure that the user has successfully completed their order before the application finalizes the transaction.
A developer writes a loop that checks whether the payment has been confirmed, but due to a logical error, the loop condition is never evaluated as false.
def checkout(): payment_confirmed = False while not payment_confirmed: # Intended to keep checking for payment confirmation # Code to process payment payment_confirmed = check_payment_status() # Assume this checks the payment status # Example function to simulate payment checking def check_payment_status(): # Logic to check payment, but due to a bug, it always returns False return False # Simulating a failure in payment processing
In this case, the checkout process becomes unresponsive as the application continuously checks the payment status without end. Users may see a loading spinner indefinitely. The infinite loop consumes CPU resources, potentially leading to degraded performance of the entire application.
This can affect other users trying to access the site. Customers may abandon their carts due to the frustrating experience which will lead to lost sales and revenue for the business. If users frequently encounter issues with the checkout process, it can damage the brand’s reputation and drive potential customers to competitors.
How to prevent infinite loops
- Clear loop conditions: Ensure that loop conditions are well-defined and that they will eventually be evaluated as false. Always check that the logic leading to the loop’s termination is sound.
- Use timeouts: Implement timeouts for long-running loops. If a certain condition is not met within a specific time frame, the loop should exit and handle the situation gracefully, such as prompting the user for action.
- Debugging tools: Use debugging tools and logging to trace the flow of execution. This can help identify potential infinite loops during development. Set breakpoints and examine variable states.
- Code reviews: Regularly conduct code reviews with peers to catch logical errors in loops. Fresh eyes can often spot issues that the original developer might overlook.
- Automated unit testing: Implement unit tests that cover edge cases and scenarios that could lead to infinite loops. Tests should simulate various conditions to ensure proper handling.
- Monitor application behavior: Continuously monitor application performance in production. Set up alerts for excessive CPU usage, which could indicate potential infinite loops or other performance issues.
- Design considerations: Consider designing systems that minimize reliance on loops for critical operations. For example, using event-driven architectures can help avoid continuous checking and enhance responsiveness.
Uncaught exceptions
An uncaught exception occurs when an error or unexpected condition arises during the execution of a program, and the program does not have a mechanism in place to handle it. This can lead to application crashes, unpredictable behavior, and a poor user experience. Read this informative article about UX testing.
Real-world example
Scenario: Online ticket booking system
Imagine an online ticket-booking platform that allows users to purchase tickets for events such as concerts, movies or sports. The system processes user requests and communicates with a payment gateway to complete transactions. During the checkout process, if there is an error in processing the payment (e.g., network failure or invalid payment details) and the application does not handle this error properly, an uncaught exception can occur.
def process_payment(user_payment_details): # Code to interact with payment gateway response = payment_gateway.process(user_payment_details) return response def book_ticket(user_details): # Attempt to process payment payment_response = process_payment(user_details.payment_info) # Possible uncaught exception # Code to finalize booking print("Ticket booked successfully!") # Example usage user_details = get_user_details() # Assume this retrieves user information book_ticket(user_details)
If an uncaught exception occurs, the entire application may crash. This prevents users from completing their transactions. This can further lead to lost sales opportunities. Frequent crashes or errors can tarnish the business’s reputation which can make potential customers hesitant to use the service in the future.
How to prevent uncaught exceptions
- Robust error handling: Implement try-catch blocks to handle exceptions gracefully. This allows the application to respond appropriately to errors instead of crashing.
- User-friendly feedback: Provide clear and informative error messages to users when exceptions occur. This helps them understand what went wrong and what actions they can take next.
- Logging and monitoring: Implement logging to capture uncaught exceptions and their context. Use monitoring tools to track application performance and error rates in production. This can help identify patterns and issues that need addressing.
- Testing for edge cases: Conduct thorough testing, including unit tests, integration tests, and user acceptance tests, to simulate various error conditions and ensure that exceptions are handled correctly. Use smart test automation tools to make this happen.
- Failover mechanisms: Implement failover mechanisms for critical operations such as payment processing. If an operation fails, the system can retry the operation or revert to a backup plan to minimize disruption.
- Continuous improvement: Review exception logs regularly to identify recurring issues and make necessary code improvements. This proactive approach can help reduce the occurrence of uncaught exceptions over time.
Incorrect Data Types
Incorrect data types occur when a variable is assigned a value of a type that does not match the expected data type for that variable. This can lead to runtime errors, unexpected behavior or incorrect results in applications, particularly in strongly typed languages where data types are strictly enforced.
Real-world example
Scenario: Financial reporting application
Consider a financial reporting application used by an accounting firm to generate monthly reports. The application processes various numerical inputs, such as revenue, expenses, and profit margins, to provide insights to clients. A developer is responsible for calculating the total revenue based on user inputs. However, they mistakenly use a string input where a numeric type is expected.
def calculate_total_revenue(revenue_list): total_revenue = 0 for revenue in revenue_list: total_revenue += revenue # Here, revenue should be a float or int return total_revenue # Example usage monthly_revenue = ["1000", "2000", "1500"] # Incorrect data types: strings instead of numbers total = calculate_total_revenue(monthly_revenue) print("Total Revenue:", total)
In this example, the attempt to add string values results in a TypeError (or equivalent error in other languages), causing the application to crash or behave unexpectedly. If the application does not crash but instead coerces types (e.g., concatenating strings), it may produce incorrect revenue calculations and give flawed financial reports.
Clients relying on accurate financial data may lose trust in the application and the firm if reports contain errors, which can result in a potential loss of business. Fixing data type issues after deployment can be costly as it requires additional time and resources to identify, correct, and retest the application.
How to prevent incorrect data types
- Data validation: Implement input validation to ensure that data entered into the system matches expected types. For example, convert strings to numbers and validate their range before processing.
- Use of strong typing: Utilize strongly typed programming languages (e.g., Java, C#) where possible, which enforce data type checks at compile time. This reduces the chances of incorrect data types being used.
- Type annotations: In dynamically typed languages (e.g., Python), consider using type annotations to clarify expected types and improve code readability.
- Comprehensive testing: Develop unit tests that cover various scenarios, including edge cases where incorrect data types might be passed.
- Code reviews: Encourage regular code reviews to identify potential type-related issues. Peers can provide valuable insights and help enforce best practices.
- Documentation and standards: Maintain clear documentation outlining the expected data types for functions and methods. Establish coding standards to ensure consistency across the codebase.
Conclusion
In this fast world of technology, the presence of bugs is an inevitable challenge that developers face. By recognizing these common pitfalls and prioritizing preventive measures, software engineers can build resilient applications that meet user expectations and maintain business integrity.
Remember, an ounce of prevention is worth a pound of cure; investing time in understanding and addressing these bugs today will save countless hours of debugging and rework tomorrow.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
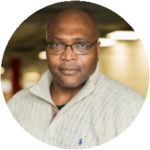