How To Deal With No Such Window Exception in Selenium
|
No Such Window Exception in Selenium
org.openqa.selenium.NoSuchWindowException
Selenium WebDriver is a widely adopted tool for automating browsers with multiple programming languages, such as Java. A frequent exception developers might come across when utilizing Selenium is No Such Window Exception. This article offers an in-depth understanding of No Such Window Exception, its primary causes, and effective management techniques. Furthermore, it contains a Java code sample to illustrate how to handle the exception.
What is No Such Window Exception?
No Such Window Exception is is an exception raised by Selenium WebDriver when it cannot find the desired browser window or tab using the specified window handle or name. This exception signifies that the window or tab you are attempting to work with is either not present or has been closed during the test run.
Primary Causes of No Such Window Exception
- Window or tab closure: The target window or tab has been closed during test execution, either through code or user action, rendering it unavailable for further interaction.
- Inaccurate window handle or name: The provided window handle or name employed to switch to the desired window or tab is incorrect or obsolete.
- Timing problems: The window or tab may not be open or fully loaded yet, particularly in dynamic web applications that use AJAX and JavaScript. In this case, Selenium might attempt to switch to the window before it has been opened or loaded, leading to No Such Window Exception.
Addressing No Such Window Exception
- Confirm the window handle or name: Ensure that the window handle or name being utilized is accurate and current. Examine the browser’s window handles to verify that the specified handle or name uniquely identifies the target window or tab.
- Wait for the window or tab: Apply explicit or implicit waits to allow the window or tab to open or load before trying to interact with it. This method ensures that Selenium does not try to switch to the window before it has been opened or loaded.
Code Example:
import org.openqa.selenium.By; import org.openqa.selenium.NoSuchWindowException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class NoSuchWindowExceptionSample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); WebDriverWait wait = new WebDriverWait(driver, 10); // 10-second explicit wait try { driver.get("https://example.com"); // Click a link that opens a new window or tab WebElement link = driver.findElement(By.id("linkId")); link.click(); // Wait for the new window or tab to open wait.until(ExpectedConditions.numberOfWindowsToBe(2)); // Change to the new window or tab for (String windowHandle : driver.getWindowHandles()) { driver.switchTo().window(windowHandle); if (driver.getTitle().equals("New Window Title")) { break; } } // Execute actions in the new window or tab WebElement element = driver.findElement(By.id("elementId")); element.click(); } catch (NoSuchWindowException e) { System.out.println("Unable to find the window: " + e.getMessage()); } finally { driver.quit(); } } }
Here, the code sample illustrates handling No Such Window Exception in a Selenium test. It imports the necessary classes, initializes WebDriver and WebDriverWait, and uses a try-catch block to navigate to a webpage, click a link that opens a new window or tab, and wait for the new window or tab to open. It then switches to the new window or tab and performs actions within it. If No Such Window Exception occurs during execution, the error message is printed, and the browser and WebDriver session are closed in the final block. This example showcases managing No Such Window Exception and waiting for windows or tabs to open before interacting with them.
By adhering to the suggested practices and employing the provided code sample as a reference, you can substantially minimize the occurrence of No Such Window Exception and enhance the reliability of your automated tests.
Why No Such Window Exception is So Common
You can read why Selenium is not an adequate solution for modern websites here. It’s also vital to mention that you can simply switch to a smart test automation tool such as testRigor to forever escape element exception issues like the one described in this article.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
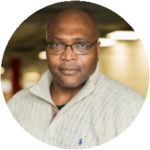