What is InvalidCookieDomainException in Selenium?
|
Cookies are small pieces of data sent from a website and stored on the user’s computer. These cookies are helpful for websites to remember stateful information (such as items added to the shopping cart in an online store) or to record the user’s browsing activity (including clicking particular buttons, logging in, or recording which pages were visited in the past).
Cookies are domain-specific and are associated with a specific domain (website). A website can only read the cookies set by its own domain and cannot access cookies from other websites. InvalidCookieDomainException in Selenium is related to managing cookies in a web browser through WebDriver commands.
This exception is thrown when you try to add a cookie under a domain that is different from the domain of the current page the WebDriver is on. In Selenium, cookies are associated with the domain of the web page, and this association is essential for the security and integrity of web sessions.
Why does InvalidCookieDomainException Occur in Selenium?
Mismatched domain from the current domain
WebDriver driver = new ChromeDriver(); driver.get("http://www.example1.com"); // Trying to add a cookie for a different domain Cookie cookie = new Cookie("name", "value", "example2.com", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Cannot add cookie to a domain different from the current domain"); }
Setting cookies for the parent domain from a subdomain
For example, when you are currently on a subdomain such as ‘sub.example.com’. From here, you try to set cookies for the parent domain of ‘subdomain.com’. This causes InvalidCookieDomainException in Selenium.
WebDriver driver = new ChromeDriver(); driver.get("http://sub.example.com"); // Trying to add a cookie for the main domain Cookie cookie = new Cookie("name", "value", "example.com", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Cannot add cookie for the parent domain while on a subdomain"); }
Setting secure cookies on a non-secure page
WebDriver driver = new ChromeDriver(); driver.get("http://www.example.com"); // Trying to add a secure cookie on a non-secure page Cookie secureCookie = new Cookie.Builder("secureName", "secureValue") .secure(true) .build(); try { driver.manage().addCookie(secureCookie); } catch (InvalidCookieDomainException e) { System.out.println("Cannot add a secure cookie to a non-secure page"); }
Incorrect cookie domain format
WebDriver driver = new ChromeDriver(); driver.get("http://www.example.com"); // Incorrect domain format Cookie cookie = new Cookie("name", "value", "examplecom", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Incorrect domain format for the cookie"); }
How to Handle InvalidCookieDomainException?
The first step is to make sure that the cookies you are trying to manipulate are compatible with the current page’s domain, i.e., they should match. Below are the ways to handle InvalidCookieDomainException:
Match the cookie and page domain
WebDriver driver = new ChromeDriver(); driver.get("http://www.example.com"); Cookie cookie = new Cookie("name", "value", "www.example.com", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Cookie domain does not match the page domain"); }
Dynamically use the current domain
You can dynamically set the cookie’s domain to match the current page’s domain in the script. This bypasses the requirement of hardcoding the domain name in the script and helps avoid the exception altogether.
Handle secure cookies appropriately
WebDriver driver = new ChromeDriver(); driver.get("https://www.example.com"); Cookie secureCookie = new Cookie.Builder("secureName", "secureValue") .secure(true) .domain("www.example.com") .build(); try { driver.manage().addCookie(secureCookie); } catch (InvalidCookieDomainException e) { System.out.println("Error adding secure cookie on a non-secure page"); }
Have exception handling in place
WebDriver driver = new ChromeDriver(); driver.get("http://www.example.com"); Cookie cookie = new Cookie("name", "value", "www.anotherdomain.com", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Caught InvalidCookieDomainException: " + e.getMessage()); // Additional handling logic here }
Correct cookie domain format
WebDriver driver = new ChromeDriver(); driver.get("http://www.example.com"); // Incorrect domain format can lead to exceptions Cookie cookie = new Cookie("name", "value", "incorrect-format", "/", null); try { driver.manage().addCookie(cookie); } catch (InvalidCookieDomainException e) { System.out.println("Cookie domain format is incorrect"); }
Use AI and Forget Selenium Exceptions
Selenium exceptions are annoying and time-consuming to debug. In today’s Agile and DevOps environments, wasting time on script errors and exceptions is a nightmare. Fast releases require quick testing and great coverage, which is difficult to achieve with Selenium test automation.
Read here the 11 reasons why not to use Selenium for automation testing.
click on "Notes" type "This is a test." check that page contains "Hello" open new tab scroll down until page contains "Submit"
This is the clarity level you can achieve through testRigor in your test cases, no fuss and complex programming scripts. You can use below straightforward commands to manage cookies as shown below:
-
You can set/get/clear browser cookies like this:
set cookie "cookie value" as "cookie-name" get cookie "cookie-name" and save it as "variableName" clear cookies
-
set/get/clear browser localStorage and sessionStorage items like so:
set item "item-data" in sessionstorage as "item-name"
See more cookie commands here. Using testRigor, everyone in your team can write and execute test cases easily which helps gain more test coverage. Most of the UI and element changes are automatically incorporated into the test scripts by testRigor through self-healing, which minimizes your maintenance effort, unlike Selenium.
Conclusion
Using age-old automation tools for new software products may put you in a tight situation. With testRigor, use the ease of codeless test automation to quicken the product releases with great product quality and coverage. Save enormous time because you need not debug the neverending Selenium exceptions. Writing test steps in testRigor is super easy and intuitive, just like a human. Use your precious time in creating more powerful and robust test cases than wasting time in exception handling and script maintenance.
Choose the correct test automation tool and stay ahead in achieving your testing goals, that too within budget!
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
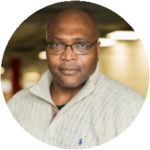