WebSocketError in Playwright Explained
|
WebSocket is a communication protocol that enables two-way communication between a client (usually a browser) and a server over a single, long-lived connection. Since Playwright is a framework for web automation testing where we control the browsers via automation scripts. Therefore, a WebSocket error in Playwright means an issue happened when interacting with WebSockets during automation execution.
So, let’s understand the root causes and how we can resolve this issue.
How WebSocketError occurs?
-
Connection Issues: A typical scenario is trying to connect to a WebSocket server with an incorrect URL or an offline server. For instance, if your Playwright script is programmed to interact with a WebSocket at ‘ws://example.com/socket’, but the server at ‘example.com‘ is down, the script will fail to establish the connection, resulting in a WebSocket error. This error signifies that the client (your script) couldn’t initiate a handshake with the server.
-
Timeouts: WebSocket connections are expected to be established within a reasonable timeframe. A timeout error may occur if your Playwright script attempts to connect to a WebSocket server, but the server takes too long to respond (due to network latency, heavy server load, etc.).
This scenario is akin to making a phone call where the call is not picked up in time, leading to a disconnection.
-
Protocol Errors: This happens when there is a mismatch or misconfiguration in the WebSocket protocol used by the client and server. For example, the handshake will fail if your Playwright test is set up to communicate using an outdated WebSocket protocol, but the server expects a newer version.
It’s like conversing in English with someone who only understands French.
-
Server Errors: Imagine your Playwright script sends data to a WebSocket server. Still, the server encounters an internal error while processing this data (e.g., due to a bug or a database outage). The server might then close the connection unexpectedly or send an error message.
This would be similar to a scenario where a customer service representative ends a call abruptly due to system issues at their end.
-
Resource Limitations: If your Playwright script is running on a machine with limited resources (like low memory or CPU) and trying to handle an intensive WebSocket communication, it might fail to maintain the connection effectively.
This is akin to trying to stream a high-definition video on an outdated computer – the system just can’t keep up.
-
Security and Policy Restrictions: Browsers and servers, such as CORS (Cross-Origin Resource Sharing), implement security policies. If your Playwright script tries to establish a WebSocket connection to a server from a disallowed origin, the browser will block it.
This is like a mailroom rejecting a package because it comes from an unapproved sender.
How to avoid WebSocketError?
-
Ensure Correct WebSocket URLs and Server Availability: Before attempting a WebSocket connection, make sure the URL is correct and the server is available. You can use simple HTTP requests to check server availability.
const { webkit } = require('playwright'); // or 'chromium' or 'firefox' (async () => { const browser = await webkit.launch(); const page = await browser.newPage(); try { // Check server availability const response = await page.goto('http://example.com/socket'); // Replace with your WebSocket server URL if (response.ok()) { // Proceed with WebSocket logic } else { console.error('Server is not available'); } } catch (error) { console.error('Failed to connect to the server:', error.message); } await browser.close(); })();
-
Handle Timeouts Gracefully: Implement timeout handling in your script to manage situations where the server takes too long to respond.
page.setDefaultTimeout(10000); // Set a default timeout of 10 seconds // Then, when establishing WebSocket connection: try { // Your WebSocket connection logic } catch (error) { if (error.name === 'TimeoutError') { console.error('WebSocket connection timed out'); } }
-
Manage Protocol Versioning: Ensure that both the client (Playwright script) and server are using compatible WebSocket protocol versions. This usually involves server-side configuration, but you can log the protocol version in your Playwright script for debugging.
page.on('websocket', websocket => { console.log('WebSocket protocol version:', websocket.protocol()); });
-
Handle Server Errors and Disconnections: Implement error handling for server-side errors and unexpected disconnections.
page.on('websocket', websocket => { websocket.on('close', () => console.log('WebSocket closed')); websocket.on('error', error => console.error('WebSocket error:', error.message)); });
- Monitor Resource Usage: Regularly monitor the resource usage of your environment. While this is not directly done in Playwright, ensuring your testing environment has sufficient resources is crucial.
-
Respect Security Policies: Adhere to security policies like CORS (Cross-Origin Resource Sharing). When testing, ensure your Playwright environment is configured to mimic the production environment’s security settings.
const browser = await playwright.chromium.launch({ // Additional options ignoreHTTPSErrors: true, // Only use for testing in a controlled environment });
- Stable Network Conditions: Ensure a stable network connection for both the server and the client running Playwright scripts. While there’s no specific code for this, using network monitoring tools and ensuring a reliable internet connection is vital.
Intelligent Automation: Driving Technology to New Heights
When executing automated tests frequently, it’s frustrating to see WebSocket errors, as this is not related to the application or the script. These errors are mainly caused by the automation tool’s inability to handle them. While using Playwright, we must set up the infrastructure, execution environment, and all other dependencies. So, any mismatch in these can cause tests to fail.
You can easily avoid these hurdles by using testRigor since it has many intelligent features. These capabilities make the testing process easier and failproof.
Let us discuss a few features of testRigor.
- Cloud-hosted: testRigor is a cloud-hosted automated tool, so we don’t have to worry about the infrastructure setup and configuration. That means the cost, time, and effort for initial setup is zero while using testRigor. The initial cost for acquiring the testRigor license will be there, but the ROI is exceptionally high compared to other tools.
- Automatic Test Generation – Leveraging generative AI, testRigor supports the generation of test cases and test data based on the description provided. So this helps to save a lot of time and effort, enabling the team to put more effort into creating more robust test cases.
- Easy Test Script Creation/Maintenance -With testRigor, you can create or edit test cases using plain parsed English, bypassing the complexities of programming languages. This opens doors for every stakeholder to contribute to testing by adding more edge cases quickly.
- Default Wait: testRigor has an intelligent feature that eliminates the need to provide custom waits for every page load. Custom waits may not always be reliable; sometimes, a page may not load fully or load more quickly than expected.
With its smart AI-based capabilities, testRigor automatically executes test scripts when the page load is complete. This ensures that no time is wasted waiting unnecessarily.
- Cross-browser and Cross-platform Support – testRigor provides comprehensive coverage across various browsers, browser versions, and platforms.
- Custom Element Locators – testRigor sidesteps the unreliability of XPaths or CSS selectors. Leveraging its AI-driven algorithms, it uses a distinct technique to pinpoint elements.
click "Cart" click on button "Delete" below "Section Name" to the right of "label"
testRigor offers many other incredible features. You can have a look at testRigor’s top features.
Conclusion
Though it’s the choice of the testing team and QA leadership to decide which tool to use for automation. But when performance and reliability are considered, using intelligent codeless tools is better. They come with built-in AI support, which reduces errors, time, and effort and substantially increases test coverage.
Therefore, the team can ensure they deliver a higher-grade product or service to the customer without falling into the trap of such environment-related errors.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
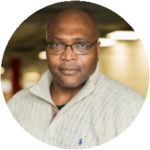