Selenium ElementClickInterceptedException Explained
|
As we know, the exceptions in Selenium are used to handle any unexpected situation while executing the test scripts. So, these exceptions gracefully handle the errors, or the test cases fail abruptly. Here, we will be discussing ElementClickInterceptedException. We will be going through what this exception is, how this exception occurs, and how we can avoid this exception.
ElementClickInterceptedException in Detail
ElementClickInterceptedException occurs when we attempt to click on an element in the webpage, but it becomes unsuccessful because another element intercepts or covers the element we try to click.
<!DOCTYPE html> <html> <head> <title>Click Intercept Example</title> </head> <body> <button id="dropdown-button">Click Me</button> <div id="dropdown-menu" style="display: none;"> <ul> <li>Option 1</li> <li>Option 2</li> <li>Option 3</li> </ul> </div> </body> </html>
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC # Create a WebDriver instance (you need to have the appropriate WebDriver executable in your PATH) driver = webdriver.Chrome() # Navigate to the web page driver.get("http://example.com") try: # Find and click the "Click Me" button button = driver.find_element(By.ID, "dropdown-button") button.click() # Wait for the dropdown menu to become visible (use WebDriverWait) wait = WebDriverWait(driver, 10) dropdown_menu = wait.until(EC.visibility_of_element_located((By.ID, "dropdown-menu"))) # Now, you can interact with the dropdown menu options option = dropdown_menu.find_element(By.XPATH, "//li[text()='Option 1']") option.click() # Perform further actions or assertions as needed except Exception as e: print(f"An exception occurred: {e}") finally: # Close the browser window driver.quit()
When we execute the script, ElementClickInterceptedException is thrown because the dropdown menu is initially hidden. So we need to first click on the “Click Me” button, then wait for the dropdown to be visible, and finally select the dropdown.
Now, let’s check the common causes for this exception.
Causes for ElementClickInterceptedException
ElementClickInterceptedException occurs for various reasons, so let’s go through a few common causes for this exception:
- Overlapping UI Elements: One of the common causes for this exception to occur is when another element in a web page partially or fully covers the element we are trying to interact with. These overlapping elements can be buttons, images, divs, etc, with a higher “z-index” value.
- Element Visibility: If the element is not visible when we try to interact, we can encounter this issue. Elements with display: none; or visibility: hidden; styles are not clickable until they become visible.
- Animations and Transitions: Most web pages include animations or transitions that modify or move the elements. We may encounter this exception if we try to click an element in the middle of an animation or transition.
- Dynamic Content Loading: The web pages that dynamically load the content using AJAX or JavaScript may not need an element when we try to access it. So, it’s always better to ensure the element’s visibility and perform interactions.
- Popup Windows or Modals: If clicking an element triggers the opening of a popup window or modal dialog, you may need to switch the WebDriver’s focus to the new window or frame before interacting with the elements inside it.
- Slow Page Loading: If the web page is slow to load, and you attempt to click an element before it’s fully loaded and ready for interaction, you may encounter this exception. Waiting for the page to load completely can help.
There are many other reasons why ElementClickInterceptedException can be triggered, but the above ones are common.
Resolving ElementClickInterceptedException
Resolving this exception means identifying and addressing the root cause of this exception. Let’s look at some strategies we can use to fix this exception.
-
Use Explicit Waits: Use explicit waits with conditions like
ExpectedConditions.elementToBeClickable
to ensure the element is ready for interaction before clicking it. This is one of the most reliable ways to handle this exception.from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC wait = WebDriverWait(driver, 10) element = wait.until(EC.element_to_be_clickable((By.XPATH, "//button[@id='myButton']"))) element.click()
-
Scroll to Element: There could be cases when the desired element is not in the browser’s viewport, and Selenium is unable to find it. To fix this, scroll to the element using JavaScript or Selenium’s built-in scrolling functionality and then try to click on it.
driver.execute_script("arguments[0].scrollIntoView();", element) element.click()
- Check for Overlapping Elements: You can inspect the web page’s HTML DOM structure and check if there are other elements that overlap the intended element. If you see an overlap, make the necessary changes to the test script.
-
Increase Wait Time: Sometimes, the page may take longer to load or render elements, and when the Selenium script tries to access it, it fails. Use the explicit wait to give the page more time so that all the elements are fully loaded and present for interaction.
wait = WebDriverWait(driver, 20) # Increase the timeout to 20 seconds
-
Use Retry Mechanism: Implement a retry mechanism to click the element multiple times with short pauses between attempts. Sometimes, the issue may be intermittent, and retrying can help.
from selenium.common.exceptions import ElementClickInterceptedException from time import sleep attempts = 3 for _ in range(attempts): try: element.click() break # Successful click, exit the loop except ElementClickInterceptedException: sleep(2) # Wait for a while before retrying
Avoid Exceptions Using Intelligent AI
As mentioned earlier, Selenium has many disadvantages because it is a legacy automation tool. They still use the old mechanisms of identifying elements or for the page waits. It is the tester's responsibility to check if the application page is loaded fully. This increases the complexity of test scripts, as more conditions need to be added to the script. Read here the 11 reasons why NOT to use Selenium for automation testing.click "cart" click on button "Delete" below "Section Name" to the right of "label"
Also, using testRigor, you don't need to add any condition using programming scripts to check if the page is loaded fully. testRigor will perform actions to execute test steps only after the page is loaded fully. You need not add explicit waits in test cases; you can easily avoid ElementClickInterceptedException.
Also, you don't have to create a retry mechanism. testRigor will retry the failed cases to check if the step passes during retry. testRigor supports test scripts in plain English so that your team doesn't need to be proficient in programming languages. Your manual testers can create test scripts faster than automation testers with other tools like Selenium.
In short, everyone on your team can write and execute tests in plain English using the generative AI- and NLP-powered capabilities of testRigor. Its self-healing features automatically incorporate the functional and element attribute changes in the test scripts. This lifts enormous maintenance burden off your shoulders, saving time, effort, and cost.
Summing Up
Selenium exceptions help the tester understand what error occurred in the test scripts. Still, when we check with the current market expectation, it may be like the automation tool should fix this exception or have mechanisms to avoid it.
The current motto of Agile and DevOps environments is frequent releases with excellent product quality. These exceptions should be handled wisely to achieve these goals, keeping the QA team on par with the development team. Selenium fails to impress here, and testRigor goes beyond a mile.
If you want to save time and effort on debugging and maintaining Selenium scripts and use your precious time to create more robust tests to achieve higher coverage, try intelligent tools such as testRigor. To learn more about testRigor, you can sign up for a free trial.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
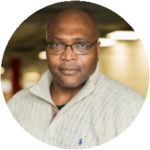