How to Build a Test Automation Framework
|
When you learn about test automation, the term “test automation framework” is unavoidable. This phrase may feel like a nightmare for many of us since it seems to involve complex coding, configurations, and setups that are hard to understand. This guide helps to know how to build a test automation framework.
But before we dive into that, let’s first understand what a test automation framework is and why it’s necessary.
What’s a test automation framework?
A test automation framework is a set of rules and tools that help to automate the testing tasks. It’s like a game plan for running tests: it tells how to organize the tests, manage the data for the tests, and report the results. This way, we don’t have to start from scratch each time we want to test something—we just follow the framework guidelines, making the testing process faster and more consistent.
In simpler terms, think of a test automation framework as a toolkit for testing software. With different tools for different tasks, the framework gives various tools and guidelines to create and run automated tests efficiently. This makes the testing process more efficient, so we can find and fix bugs faster and keep the software running smoothly.
Why do we need a test automation framework?
A test automation framework is necessary for several vital reasons to improve the software testing process’s efficiency, accuracy, and scalability. Let’s see a few reasons why we need a test automation framework:
- Resource optimization – A test automation framework streamlines resource optimization by matching resources to specific requirements through flexible processes. This adaptability allows for more efficient use of resources in alignment with organizational goals.
- Reusable automation code – Reusability is one of the critical advantages of a test automation framework. If one QA team has written test scripts for a particular functionality like login, those scripts can often be reused or adapted for another application with similar login functionality. This saves time and effort, as there is no need to write new test cases from scratch.
- Continuous testing – Runs automated tests continuously and is crucial for a successful CI/CD pipeline to ensure code quality. Test automation frameworks facilitate this by enabling the easy creation and automatic execution of tests.
- Enhanced speed and reliability – Test automation frameworks expedite the testing process by enabling simultaneous execution of multiple scenarios, thus saving time. Automated tools also enhance reliability by minimizing the risk of human error.
Building a test automation framework
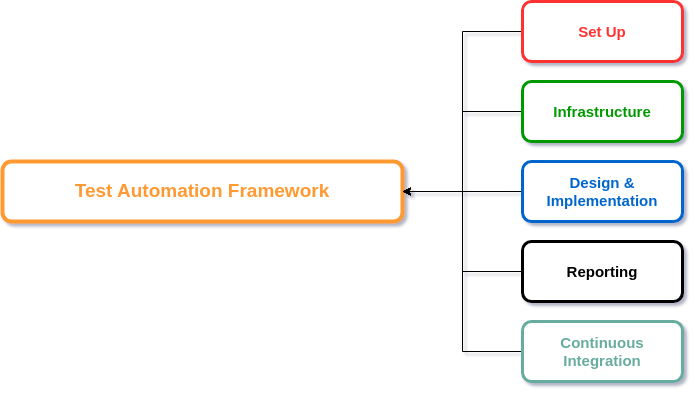
Let’s use Selenium, a popular automation tool for automating web browsers, to build a test automation framework. It works well with various programming languages like Java, Python, C#, etc. Building a test automation framework with Selenium involves a structured approach that includes setting up the environment, designing the infrastructure, generating reports, and integrating with CI/CD pipelines.
Setting up
In this step, we will set up the development environment, the automation tool and its dependencies, and a compatible framework based on the programming languages.
Environment Preparation
To prepare the environment, first install a suitable development environment. We can opt for Eclipse or IntelliJ for Java, while for C#, Visual Studio is a good choice. If we use Python, we can use IDEs like PyCharm or Visual Studio Code. This sets the foundation for the test automation framework.
Setup Build Tools
Installing build automation tools like Maven for Java or pip for Python can help manage dependencies, build the project, and package it for distribution.
Install Selenium WebDriver
Installing Selenium WebDriver involves different steps depending on the programming language and development environment. Below are the installation guides for Java and Python:
Java:
- Download Selenium Jars: Go to the Selenium official website and download the latest Selenium WebDriver Jars.
- Add to Project: Create a new Java project in the preferred IDE (e.g., Eclipse, IntelliJ IDEA). Add the downloaded Selenium Jars to the project’s build path (Right-click on Project -> Properties -> Java Build Path -> Libraries -> Add External JARs).
- Maven: If using Maven, we can simply add Selenium WebDriver as a dependency in the pom.xml:
Python:
- Install via pip: Open the terminal and run the command pip install selenium to install Selenium.
- Check Installation: We can verify the installation by running the command – from selenium import webdriver. If there are no errors, the installation was successful.
Add Dependencies
The next step is to add dependencies related to selenium and the test framework packages. We can add JUnit or TestNG dependencies for Java in Maven’s pom.xml file. For Python, we can use the command install pytest using pip. In C#, using NuGet Package Manager, we can add NUnit.
Infrastructure
We set up object repositories, data management, and version controls in this stage. Let’s see in detail.
Setting up Browser dependencies
Download the necessary browser drivers from their official websites, like ChromeDriver for Chrome or GeckoDriver for Firefox. Place the downloaded driver executables in a directory part of the system’s PATH, or specify the driver’s location within the test script. These drivers bridge the Selenium tests and the web browser, enabling automated control of browser activities.
Execution Infrastructure
We have two main options to run tests across various browsers and mobile devices: local and cloud-based testing.
Local testing may require maintaining multiple versions of browsers and mobile device emulators, which can become complex and resource-intensive.
Cloud-based services like BrowserStack and LambdaTest simplify this process by providing a range of browsers and mobile devices for testing. These platforms offer compatibility across various versions and configurations, saving the hassle of maintaining the test environment.
Selenium Grid
If the organization requires tests to run from a central repository, Selenium Grid is an ideal solution. Its hub-node architecture allows the hub to act as a centralized server, coordinating the execution of tests across various nodes or test environments. This parallel execution speeds test runs and enhances test coverage across multiple machines and browsers.
Design & Implementation
Below are the components associated with the design and implementation of a test automation framework:
Framework Architecture
Choosing the type of framework is a strategic decision that can affect the success of the test automation efforts. Let’s review the most commonly used frameworks.
Data-Driven Framework: This approach allows to run the same test script with multiple data sets. It separates test data from test logic, making maintaining and extending the tests easier.
Keyword-Driven Framework: In this model, keywords represent different operations or activities within the test. The test data and keywords are stored in an external data source like an Excel file, allowing non-technical stakeholders to contribute to test creation.
Hybrid Framework: As the name suggests, this combines elements of both Data-Driven and Keyword-Driven frameworks. It provides the flexibility to use the most effective techniques from each type for different scenarios.
Page Object Model (POM): This pattern is based on creating an object repository to store all the web elements. It allows the separation of the layout and the test logic, making the tests more maintainable and scalable.
Object Repository
An Object Repository is a centralized storage location for web element locators used in the test scripts. By keeping all locators in a central repository, we simplify test maintenance and improve code reusability. When changes occur in the web application’s UI, we only need to update the locators in this central location rather than throughout multiple test scripts. This approach makes it easier to manage and maintain the test automation framework.
Test Data Management
Managing test data is critical to building a robust test automation framework. The test data management strategy will depend on factors such as the complexity of the test cases, team skill set, and specific testing requirements. Below are the different types of data sources:
External Data Sources
- Excel/CSV Files: Data can be read from Excel or CSV files using libraries like Apache POI for Java or pandas for Python. This makes it easy to maintain and update the test data.
- Databases: For more dynamic and complex scenarios, using a database like MySQL, SQLite, or MongoDB is beneficial. The Selenium scripts can then query the database to retrieve the required data.
- JSON/XML Files: Storing data in JSON or XML formats is convenient for API testing or configurations. Parsing libraries can be used to read and manipulate this data.
In-Code Solutions
- Data Providers: Test frameworks like TestNG offer @DataProvider annotations, which can supply multiple data sets directly to test methods, enabling data-driven testing.
- Constants/Enums: For fixed sets of data that won’t change frequently, defining them as constants or enums within the test code can be straightforward and convenient.
Here are a few effective test data management tools.
Version Control
Utilizing a version control system offers a structured way to keep track of any changes made to the test automation framework. This tracking is critical for debugging, feature enhancement, and understanding the framework’s evolution. Additionally, version control ensures that everyone on the team is working on the most up-to-date version of the framework, which promotes consistency and helps prevent conflicts that can arise from working on different versions. Popular platforms for version control like BitBucket, GitHub, or GitLab offer centralized places where the codebase can be stored and managed.
Reporting
Logging
Accurate and detailed logging is vital for tracking and debugging test executions. Using libraries like Log4j, we can effectively capture logs at different levels, such as INFO, DEBUG, WARN, and ERROR. Log4j offers flexibility regarding log formatting, destinations (like files, console, database), and more. This helps quickly identify issues, understand the flow of test cases, and ensure that all steps are recorded for future reference.
Test Reports
A well-structured test report aids stakeholders in assessing the quality and reliability of the software under test. Libraries like Extent Reports and TestNG Reports offer detailed test execution insights, including pass-fail metrics, logs, and screenshots for failed tests. Here is a detailed blog to get you started on test reports.
Extent Reports provide rich, visual feedback about the tests, including pie charts, system info, and logs.
TestNG Reports come by default with the TestNG framework and provide a hierarchical view of the tests executed and logs.
Popular Tools:
For both logging and reporting, there are several popular tools available in the industry:
- For Logging: Apart from Log4j, there’s SLF4J, Logback, and JUL (Java Util Logging).
- For Reporting: Allure Reports, JUnit Reports, and Cucumber Reports are among the popular choices, in addition to Extent Reports and TestNG Reports.
Continuous Integration
Integrating a test automation framework with a CI/CD pipeline is vital for enabling continuous testing. This integration is crucial for achieving continuous integration and deployment, ensuring that code changes are automatically tested and ready for production. Here’s how to go about it:
- Select a CI/CD Tool: Choose a CI/CD tool that suits the project’s needs. The popular choices are Jenkins, Travis CI, CircleCI, and GitLab CI/CD. Many of these tools integrate easily with source control management systems like Git.
- Tool Integration: Set up a job or workflow in the chosen CI/CD tool:
- Configure it to pull the latest code from the repository.
- Set it up to install any dependencies that the project requires.
- Configure it to execute the test scripts whenever there’s a change in the application or whenever a new build is created.
- Automation Script Execution: In the CI/CD pipeline, including a step to execute the Selenium automation scripts. This can be done by invoking the build tool (Maven, Gradle, etc.) or running a script that triggers the test runner.
- Feedback Loop: Ensure that the results of the test runs are communicated back to the team, especially when tests fail. This can be through emails, Slack notifications, or dashboard reports.
- Parallel Execution: If the test suite grows and takes longer, consider integrating with Selenium Grid or cloud services like Sauce Labs or BrowserStack to run tests in parallel and reduce execution time.
Drawbacks with legacy frameworks
Selenium frameworks have disadvantages, and their popularity has declined due to various factors:
- Delay in Automation: For the automation team, it’s not possible to immediately start creating automation scripts. They first need to plan the framework’s architecture as a preliminary step. Once that’s in place, they can design and build the framework. Initiating this activity as early as possible is crucial so the team can start writing scripts from the first sprint.
- Time and Resource Consumption: Developing a framework is a time-consuming process that involves multiple steps. Initially, one must select the proper tool framework and dependencies for reporting, screenshot capturing, and video recording modules. Additionally, it’s crucial to have modules that integrate with test management tools and CI/CD systems.
Completing an entire framework can take weeks, and it will likely continue to evolve even after its initial development. Moreover, building such a framework requires individuals with solid coding skills.
- Maintenance-intensive: As the number of integrations grows, maintaining the framework becomes increasingly challenging. For example, changes in integration plugins can lead to compatibility issues or introduce known bugs. These problems require frequent modifications to the framework, making it a complex and demanding task to manage effectively.
- Dependency Issues: Many dependencies can reach their End of Life (EOL), necessitating searching for new dependencies as replacements. This often leads to code changes, as the methods used by new dependencies may differ from those in the deprecated ones, adding another layer of complexity to maintenance. Also, when the upgrade happens to dependencies, it is mandatory to check the functionality every time, as there can be compatibility issues or known bugs.
Here are the 11 reasons why you should not use Selenium for test automation.
How testRigor makes a difference
testRigor is a next-generation automation tool that integrates generative AI technology and offers a codeless approach to testing. testRigor breaks the traditional framework creation and scripting approach, introducing a fresh perspective through its innovative features. testRigor comes equipped with a pre-built framework, allowing testers to start writing test scripts right from Day 1. Let’s go into detail about the features.
- Cloud-hosted: testRigor is a cloud-hosted tool, so there is no headache in installing and setting up the tool.
- Codeless tool: testRigor is a codeless automation tool enabling QA teams to craft test scripts using plain English. This eliminates the need for complex programming languages, making test script creation more accessible and efficient for a broader range of team members.
- No Object Repository: Unlike Selenium and other legacy frameworks that rely on XPath, IDs, or CSS selectors for element identification, testRigor employs a unique approach. It typically identifies elements based on their displayed names or relative positions. This approach eliminates the need for XPath, which often relies on DOM element properties, reducing the likelihood of flaky tests caused by changes in DOM elements.
- Reports – testRigor provides live, real-time reports refreshed with every executed test step. Additionally, testRigor enhances the reporting process by including video recordings of the entire test execution, detailed step-by-step execution reports containing screenshots, and comprehensive logs that pinpoint the exact cause of failures.
- Inbuilt Integrations: testRigor has native integrations for various systems and platforms, eliminating external integration challenges like version compatibility and End-of-Life issues. A few built-in integrations are listed below:
- Leading test case management systems, including TestRail, Zephyr, XRay, etc
- Ticketing systems like Jira, Pivotal Tracker, Azure DevOps, etc
- All CI platforms, including Jenkins, CircleCI, Azure DevOps, etc
- Major infrastructure providers, including LambdaTest, BrowserStack, SauceLabs, etc
The above listed are just a few features. Here are testRigor’s top features.
open url "https://www.amazon.com" click "Sign In" enter stored value "email" into "email" click "Continue" enter stored value "password" into "password" click "Login" enter "Men's Printed Shirt" roughly to the left of "search" click "Search" click on image from stored value "Men's Printed Shirt" with less than "10" % discrepancy click "Add to Cart" click "Cart" click "Checkout"
The above example illustrates how testRigor simplifies test case creation and debugging with minimal and straightforward steps.
Conclusion
While legacy frameworks built with tools like Selenium were once popular, they often incurred significant rework and maintenance expenses. Their added complexity to the automation testing process further contributed to their limitations. These frameworks fall short in today’s agile world, where organizations aim for frequent upgrades.
This is where testRigor steps in. With its innovative features, testRigor empowers QA teams to create automation scripts on the go without the need for framework configuration, perfectly aligning with industry demands and supporting the “early to market” approach for swift product launches.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
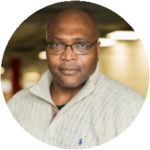