HubSpot Testing
|
HubSpot is a customer relationship management (CRM) platform designed to empower businesses of all sizes with inbound marketing, sales and customer service tools. It aims to help companies attract, engage and retain customers by offering a unified suite that streamlines various aspects of customer interaction.
HubSpot was founded in 2006 by Brian Halligan and Dharmesh Shah at the Massachusetts Institute of Technology (MIT). These founders recognized a shift in how people were making buying decisions. Instead of being receptive to interruptive advertising and cold calls, customers increasingly turned to the Internet to research and seek solutions on their own terms.
HubSpot aimed to revolutionize marketing and sales with this insight. Their platform provides tools that help businesses attract visitors through helpful content (blogging, SEO, social media), convert those visitors into leads with forms and email marketing and then nurture them through sales. This “inbound marketing” concept focuses on providing value to customers, ultimately making sales a more natural and seamless experience.
Here’s a breakdown of key features and functionalities:
Marketing Hub
- Landing page creation: HubSpot offers a variety of lead generation tools, including forms, pop-ups and live chat. These tools can be integrated with landing pages and other marketing assets to capture and manage leads efficiently.
- Email marketing: HubSpot allows users to create and manage email campaigns, segment their audience and track the performance of their emails through detailed analytics. The platform supports A/B testing, which enables marketers to test different email variations and determine which performs best.
- SEO tools: HubSpot offers a suite of SEO tools that help users optimize their content for search engines. These tools provide insights into keyword performance, backlinking opportunities and on-page SEO elements, such as meta descriptions, headers and image alt text.
- Social media management: Users can connect their social media accounts to HubSpot and schedule posts, track engagement metrics and monitor brand mentions across multiple social media platforms.
- Content management system (CMS): HubSpot provides tools for creating and managing blog posts, landing pages and email campaigns. The content editor is user-friendly and supports drag-and-drop functionality, making it easy to create professional-looking content without needing advanced design skills.
Sales Hub
- CRM tools: HubSpot’s CRM is the core of the Sales Hub, allowing sales teams to manage their contacts, companies, deals and tasks in one place. The CRM automatically tracks interactions with prospects and customers, providing valuable insights into their behavior and preferences.
- Email tracking and automation: Sales professionals can use HubSpot to track email opens, clicks and replies, allowing them to gauge the effectiveness of their outreach efforts. The platform also supports email templates and sequences, which can be used to automate follow-up communication with prospects.
- Meeting schedule: HubSpot offers a meeting scheduling tool that integrates with popular calendar apps like Google Calendar and Microsoft Outlook. This tool allows prospects to book meetings directly with sales reps based on their availability, eliminating the back-and-forth communication typically associated with scheduling.
- Playbooks and Sales Enablement: HubSpot offers playbooks and sales enablement tools that help sales teams standardize their sales processes, share best practices and provide consistent messaging across the organization.
Read more about testing HubSpot Sales Hub.
Service Hub
- Ticketing system: HubSpot’s ticketing system allows customer service teams to manage and track customer issues and requests. Tickets can be assigned to specific team members, prioritized and tracked through various stages of resolution.
- Live chat: HubSpot provides live chat and chatbot functionality, allowing businesses to engage with customers in real time on their websites. Chatbots can be programmed to answer common questions, qualify leads, or route inquiries to the appropriate team members.
- Service Analytics and Reporting: The platform offers a range of reporting tools that provide insights into customer support performance, including metrics like ticket response time, resolution time and customer satisfaction scores. These reports can be customized to track specific KPIs and help service managers make data-driven decisions.
- Customer feedback tools: The Service Hub includes tools for collecting customer feedback through surveys, NPS (Net Promoter Score) surveys and customer satisfaction surveys. This feedback can be used to identify areas for improvement and enhance the overall customer experience.
CMS Hub
The CMS Hub is designed to help businesses create and manage their websites. Key features of the CMS Hub include:
- Website Builder: HubSpot’s website builder is a drag-and-drop tool that allows users to create and manage web pages without needing technical expertise. The builder includes a variety of pre-designed templates and modules, making it easy to create a professional-looking website.
- Content Personalization: The CMS Hub allows users to personalize website content based on visitor behavior, location, device type and other criteria. This helps create a more tailored experience for visitors and increases engagement.
- SEO Recommendations: HubSpot provides SEO recommendations and tools to help users optimize their website for search engines. These tools offer insights into on-page SEO elements, backlinking opportunities and content optimization.
- Blogging and Content Creation: The CMS Hub includes tools for creating and managing blog posts, landing pages and other content. The platform supports content versioning, collaboration and approval workflows, making it easy to manage content creation at scale.
- Analytics and Reporting: The CMS Hub provides a variety of reporting tools that offer insights into website performance, including metrics like page views, bounce rate and conversion rates. These reports can be customized to track specific KPIs and help web managers optimize their website for better performance.
Operations Hub
The Operations Hub is designed to help businesses manage their data and streamline their operations. Key features of the Operations Hub include:
- Data Sync: The Operations Hub provides tools for syncing data between HubSpot and other third-party applications. This ensures that data is consistent and up-to-date across all systems, reducing the risk of errors and duplication.
- Data Quality Automation: The platform includes tools for automating data quality tasks, such as deduplication, data enrichment and data validation. This helps businesses maintain clean and accurate data, which is essential for effective decision-making.
- Custom Workflows: The Operations Hub allows users to create custom workflows for automating routine tasks, such as data entry, lead routing and notification management. These workflows can be customized to reflect the unique needs of each business.
- Custom Properties and Data Fields: HubSpot allows users to create custom properties and data fields to capture and store information that is specific to their business. This helps ensure that all relevant data is captured and can be used for reporting and analysis.
- Operations Analytics and Reporting: The Operations Hub includes a range of reporting tools that provide insights into operational performance, including metrics like data accuracy, workflow efficiency and system integration. These reports can be customized to track specific KPIs and help operations managers identify areas for improvement.
HubSpot App Marketplace
This marketplace, accessible within HubSpot, allows users to discover and integrate a vast collection of third-party apps. These apps extend the functionality of HubSpot by connecting to existing tools and services, adding specialized features or catering to specific industry needs.
Hubspot testing can be performed manually or through automated testing tools. Automated testing tools can speed up the testing process, improve accuracy and reduce the risk of human error. For the front end, HubSpot uses HTML, CSS and JavaScript; the back end mainly uses Python, Java and C++.
HubSpot Integrations
HubSpot offers a wide range of integrations with other software platforms, allowing businesses to connect their existing tools and systems to HubSpot. These integrations help create a seamless flow of data across different platforms, improving efficiency and ensuring that all relevant information is accessible in one place.
Some of the most popular integrations include:
- Email and Calendar: HubSpot integrates with popular email and calendar apps like Gmail, Outlook and Office 365. This allows users to sync their email communications, schedule meetings and manage their calendars directly from within HubSpot.
- Marketing Automation: HubSpot integrates with various marketing automation tools, such as Mailchimp, ActiveCampaign and Marketo. This allows users to sync their marketing efforts across multiple platforms and leverage the unique features of each tool.
- Sales and CRM: HubSpot integrates with other CRM platforms like Salesforce, Zoho CRM and Microsoft Dynamics 365. This allows businesses to sync their customer data across different systems and create a unified view of their customers.
- E-commerce: HubSpot offers integrations with popular e-commerce platforms like Shopify, WooCommerce and Magento. This enables businesses to sync their customer data, track purchases and manage their e-commerce operations from within HubSpot.
- Customer Support: HubSpot integrates with customer support tools like Zendesk, Intercom and Freshdesk. This allows businesses to sync their customer support data and manage their support operations more effectively.
Testing HubSpot Integrations
Testing HubSpot integrations is necessary to ensure there are no issues in data flow between HubSpot and the application we integrate with. This is crucial for maintaining data accuracy, reliability and timeliness across the systems. Through testing, companies can verify that data flows correctly between systems, minimizing the risk of errors or data loss that could lead to misinformed decisions or negatively impact customer interactions. Additionally, testing helps identify compatibility issues or bugs within the integration process, ensuring that automation workflows operate efficiently and the overall system remains stable.
To ensure there are no such issues, it’s always suggested that the application goes through different levels of testing, like unit testing, integration testing and end-to-end testing.
Unit testing HubSpot
It refers explicitly to testing the functionality of your custom code that interacts with HubSpot’s APIs. It’s about ensuring your code behaves as expected when working with HubSpot data or functionalities. The primary focus is on isolated units of your code, not the entire integration or HubSpot itself.
You’re testing how your code interacts with HubSpot’s APIs, handles responses and performs the desired actions. In unit testing, what we test is:
-
API Calls:
- Test if your code constructs the correct API requests (e.g., URLs, parameters, headers).
- Verify it handles responses appropriately, parsing data and extracting relevant information.
- Check for error handling mechanisms and how your code reacts to different response codes (success, errors).
-
Data Processing:
- Ensure your code correctly transforms or manipulates data received from HubSpot’s APIs before using it further.
- Test how your code handles unexpected data formats or missing information from the API response.
-
Functionality Specific to Your Integration:
- Beyond fundamental API interactions, test the core functionalities of your custom code related to the HubSpot integration.
- This might involve testing calculations, transformations or actions your code performs based on HubSpot data.
The choice of tools for unit testing HubSpot integrations depends mainly on the technology stack used for the integration. Let’s review a few commonly used unit testing tools based on each framework used for integration.
Junit
The go-to unit testing framework for Java, JUnit, offers a comprehensive suite of features to streamline your testing process.
Features:
- Test Annotations: JUnit uses annotations to simplify test organization and execution. You can mark methods with annotations like @Test to identify them as unit tests.
- Test Runners: JUnit provides a built-in test runner that executes your tests and presents results.
- Assertions: JUnit offers a rich set of assertion methods (e.g., assertEquals, assertTrue) to verify expected outcomes in your tests.
- Test Suites: You can group related tests into suites for better organization and execution control.
How it helps HubSpot unit testing:
JUnit allows you to write clear and concise unit tests for your custom Java code interacting with the HubSpot API. Using JUnit’s mocking libraries like Mockito, you can isolate your code and simulate API interactions to ensure your code functions as expected.
Pytest
A popular unit testing framework known for its flexibility and BDD (Behavior-Driven Development) approach, Pytest makes writing and managing unit tests in Python a breeze.
Features:
- Simple and Concise Syntax: Pytest uses a natural language-like approach for defining tests, improving readability and maintainability.
- Fixtures: It allows you to define fixtures reusable code snippets that set up test environments or provide common test data.
- Parametrization: It supports parametrization, enabling you to run the same test with different data sets for thorough testing.
- Plugins: Pytest has a rich ecosystem of plugins that extend its functionality, such as reporting, integration with continuous integration tools and more.
How it helps HubSpot unit testing:
Pytest allows you to write clear unit tests for your Python code interacting with HubSpot’s API. In combination with mocking libraries like Mock, you can isolate your code and create mock objects to simulate API behavior during testing, ensuring your code interacts with the HubSpot API as intended.
Jest
A beloved choice for JavaScript testing, Jest offers a feature-rich environment for writing, running and managing unit tests. Read more about Jest Testing.
Features:
- Fast Test Execution: Jest is known for its exceptional speed, providing near-instant feedback during test development.
- Test Watchers: It offers a test watcher that automatically re-runs tests whenever your code changes.
- Code Coverage Reports: It can generate comprehensive reports highlighting areas of your code that your tests haven’t exercised.
- Snapshot Testing: Jest supports snapshot testing, a technique for verifying the output of your code components to ensure they continue to render UI elements as expected.
How it helps HubSpot unit testing:
Jest allows you to write unit tests for your JavaScript code interacting with the HubSpot API. It integrates well with mocking libraries like Sinon.JS, enabling you to create mock objects and simulate API responses during testing. This ensures your JavaScript code interacts with the HubSpot API as intended.
const sinon = require('sinon'); const axios = require('axios'); const { createContact } = require('./hubspotIntegration'); describe('createContact', () => { let axiosPostStub; beforeEach(() => { // Mock the axios.post method before each test axiosPostStub = sinon.stub(axios, 'post').resolves({ data: { id: '123', message: 'Contact created' } }); }); afterEach(() => { // Restore the original axios.post method after each test axiosPostStub.restore(); }); it('should create a new contact and return response data', async () => { const contactData = { firstName: 'John', lastName: 'Doe', email: '[email protected]' }; const result = await createContact(contactData); // Check if axios.post was called with the correct URL and data expect(axiosPostStub.calledWith('https://api.hubapi.com/contacts/v1/contact/', contactData)).toBeTruthy(); // Verify the function returns the correct response data expect(result).toEqual({ id: '123', message: 'Contact created' }); }); it('should throw an error when the API call fails', async () => { axiosPostStub.rejects(new Error('API Error')); await expect(createContact({})).rejects.toThrow('Failed to create contact'); }); });
Explaining the above test suite in detail:
- We use sinon.stub to mock axios.post method before each test, making it resolve with mocked response data or reject it with an error, depending on the test.
- In the first test, we assert that axios.post was called with the correct arguments and that createContact returns the expected data structure.
- In the second test, we make axios.post reject with an error to test the error handling logic of createContact, expecting it to throw an error.
Integration testing HubSpot
There are two main reasons why integration testing is crucial for HubSpot integrations. Firstly, HubSpot itself is a complex system with various features and functionalities. When you add another application on top of that through an integration, you create a new, interconnected system. Integration testing ensures all the different parts communicate seamlessly and behave as expected. This helps avoid data inconsistencies, errors and disruptions in your workflows.
Secondly, integrations are rarely static. Updates to either HubSpot or the integrated application can introduce unforeseen issues. Integration testing helps catch these problems early on before they impact your daily operations. By proactively testing your integrations, you can ensure a smooth flow of information and maintain the overall reliability of your HubSpot ecosystem.
Integration testing can also be performed at the UI and API levels. So, let’s review common tools used for the testing.
API Integration Testing
We discuss Postman and SoapUI for API integration testing here:
Postman
Postman is a popular API development tool developers and testers use to build, test and modify APIs. It supports RESTful and SOAP APIs, making it versatile for various integration scenarios, including those with HubSpot.
Key Features for HubSpot Integration Testing:
- Easy to Use: Postman’s user-friendly interface makes it simple to create and send HTTP requests and then inspect the responses. This is particularly useful for testing the API calls your HubSpot integration makes.
- Collections and Environments: You can organize your API requests into collections and define environments for different stages of development (e.g., development, staging, production). This organization aids in systematically testing your integration under different configurations.
- Pre-request Scripts: These scripts run before an API call is made, allowing you to set up the necessary conditions for your test, such as generating authentication tokens or setting dynamic variables.
SoapUI
It is an open-source web service testing application for SOAP and REST APIs. It’s designed to test API functionality, security and performance, making it a comprehensive tool for integration testing, including with platforms like HubSpot.
Key Features for HubSpot Integration Testing:
- Protocol Support: While its name suggests a focus on SOAP, SoapUI also robustly supports RESTful API testing. This dual support is beneficial for testing integrations with various services, including HubSpot’s REST APIs.
- Functional Testing: SoapUI allows you to create test suites, test cases and assertions to verify the functionality of your HubSpot integration. This includes checking the correctness of API responses and ensuring that your integration handles API requests as expected.
- Security Testing: It offers built-in security tests, allowing you to perform scans for common security vulnerabilities in your integration. This is crucial for ensuring that data exchanged with HubSpot is handled securely.
UI Integration Testing
One commonly used tool for UI integration testing is Selenium. We will discuss it in the E2E testing phase.
End-to-end testing HubSpot
Regular E2E testing is vital for robust HubSpot integrations. It goes beyond individual components, mimicking real user experiences. This broader perspective ensures the entire system functions seamlessly, from user interface to back-end processes. Unlike unit or integration testing, E2E testing reveals issues that might be missed in isolation, like data flow problems or clunky user journeys.
E2E testing validates critical aspects for smooth CRM, marketing and sales. It verifies data accuracy, authentication flows and third-party integrations. This proactive approach ensures the integration remains reliable and adapts to updates, fostering user trust and business success. Let us go through the tools that we can use for E2E testing.
Selenium
Selenium was a commonly used UI automation tool by many companies. The main advantage of Selenium is that it is open-source; anyone can customize the framework. However, using Selenium has many disadvantages, such as code complexity. As the automation code count increases, debugging becomes impossible and more time will be spent on maintenance tasks.
There are many other reasons why it is not a good idea to use Selenium for automation testing. Here, we are not covering much detail about Selenium, but if you would like to know about building Selenium tests, you can refer to this blog.
One of the most commonly used tools explicitly designed for E2E testing is testRigor. Let us learn about it in detail.
testRigor for E2E testing
A common question that comes to everyone’s mind would be, what is unique about testRigor that makes it stand out from other tools? The answer is it’s packed with many powerful features, making it a well-crafted E2E testing tool. So, let us go through a few features:
- Plug and Play tool: testRigor is a cloud-hosted tool. You just need to sign up, purchase a plan and use it. There is no need to worry about setting up the infra, installation, etc. testRigor saves a lot of time and money here.
- Comprehensive Integrations: The next headache will be integrations once the installation is done. We must find the proper integration versions and ensure integrations won’t fail during an upgrade. testRigor is packed with almost every integration you would need. You can read more about supported testRigor integrations here.
- No-code Automation: testRigor simplifies the pre-requisite of having programming language skills and lets you create test scripts in plain parsed English. That means manual testers can create test scripts faster than an automation engineer automating using a traditional automation framework. That is why it is a test automation tool for manual testers. Also, business analysts or stakeholders who review the test scripts can easily modify the test cases. With generative AI, you can generate tests or test data based on the description you provide.
-
Custom Element Locators: testRigor does not rely on flaky XPath or CSS selectors; instead, it uses its stable way of identifying elements, i.e., testRigor locators. This process has become more straightforward and human, thanks to AI algorithms. You just mention the UI text that you see on the screen or its position to identify the element, such as:
click "cart" click on button "Delete" below "Section Name"
-
Testing Powerhouse: Another headache is that you may need to keep multiple frameworks for each type of testing, such as mobile, web and desktop. However, testRigor is one tool that supports most types of testing. So, there is no need to keep multiple frameworks. You can perform the below testing types using plain English commands:
These are just the tip of the iceberg. Read to know the top features of testRigor.
How to use testRigor for Hubspot Testing?
It is really straightforward to test Hubspot with testRigor and its plain English commands. Here is a step-by-step guide to doing so. Let’s get started:
Step 1: Log in to your testRigor app with your credentials.
Step 2: Set up the test suite for the website testing by providing the information below:
- Test Suite Name: Provide a relevant and self-explanatory name.
- Type of testing: Select from the following options: Desktop Web Testing, Mobile Web Testing, Native and Hybrid Mobile, based on your test requirements.
- URL to run test on: Provide the Salesforce application URL you want to test.
- Testing credentials for your web/mobile app to test functionality which requires user to login: You can provide the app’s user login credentials here and need not write them separately in the test steps then. The login functionality will be taken care of automatically using the keyword login. Filling out this field is optional and not mandatory.
- OS and Browser: Choose the OS Browser combination on which you want to run the test cases.
- Number of test cases to generate using AI: You can simplify your test creation further by opting to generate test cases based on the App Description text. This feature works on generative AI.
- App Description: Provide the description of the Application you are testing.
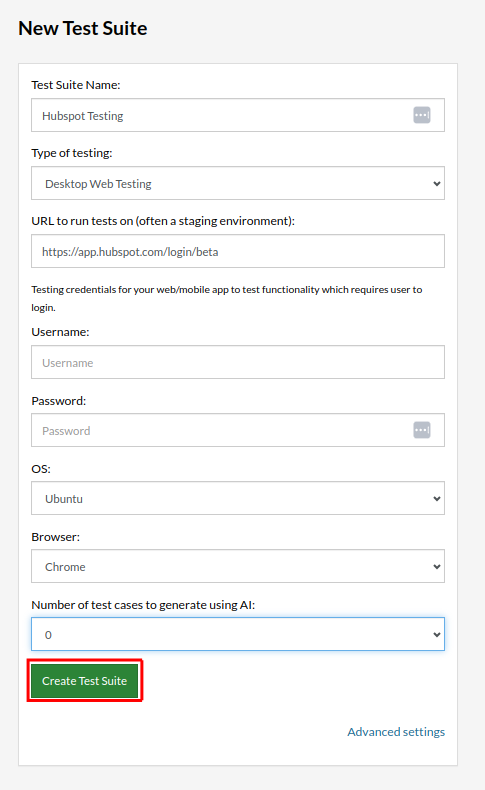
On the next screen, you can let AI generate the test case based on the app description you provided while creating the Test Suite. However, for now, select do not generate any test, since we will write the test steps ourselves.
Step 4: To create a new custom test case, click Add Custom Test Case.
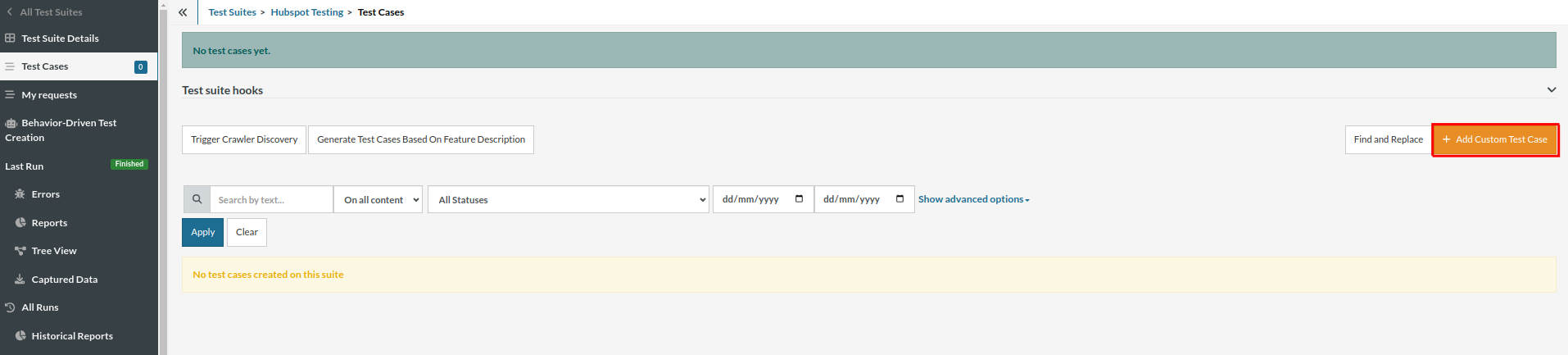
Step 5: Next step is to provide the test case Description and add the test steps as below:
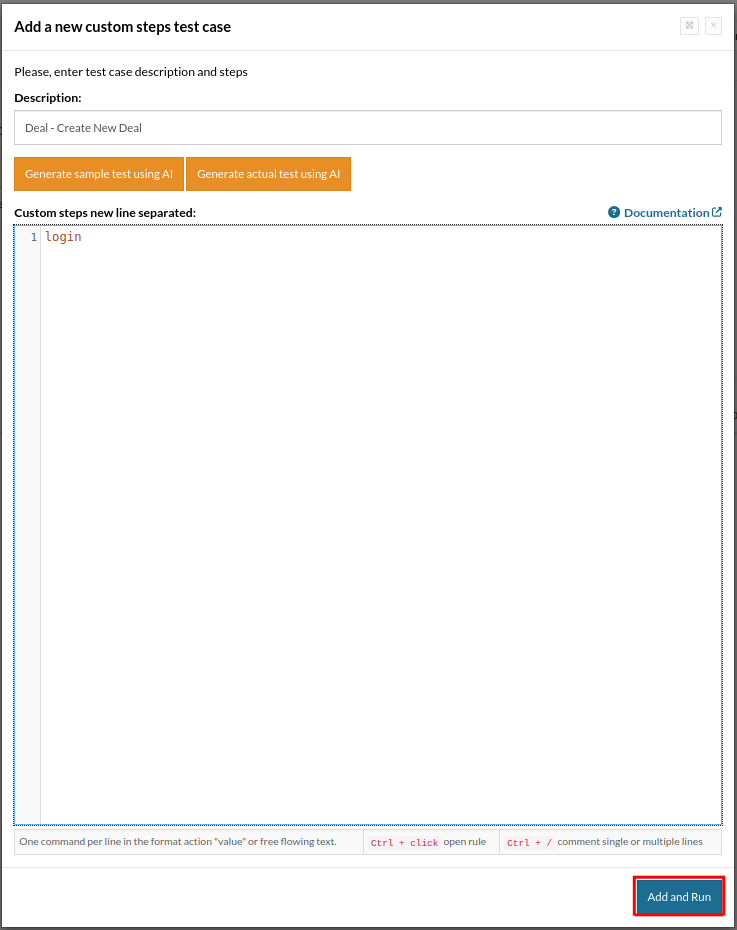
Step 6: As we have mentioned in Step 2, ‘login’ keyword will automatically login you to the application, using the credentials provided at the time of test suite creation.
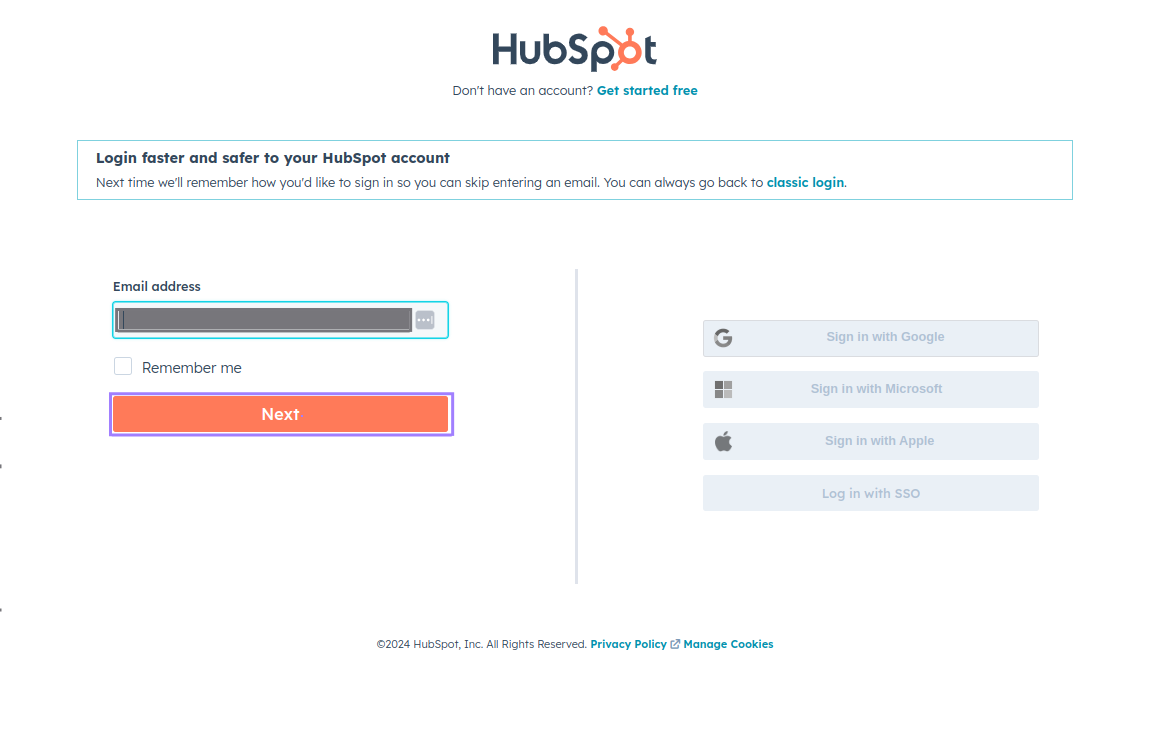
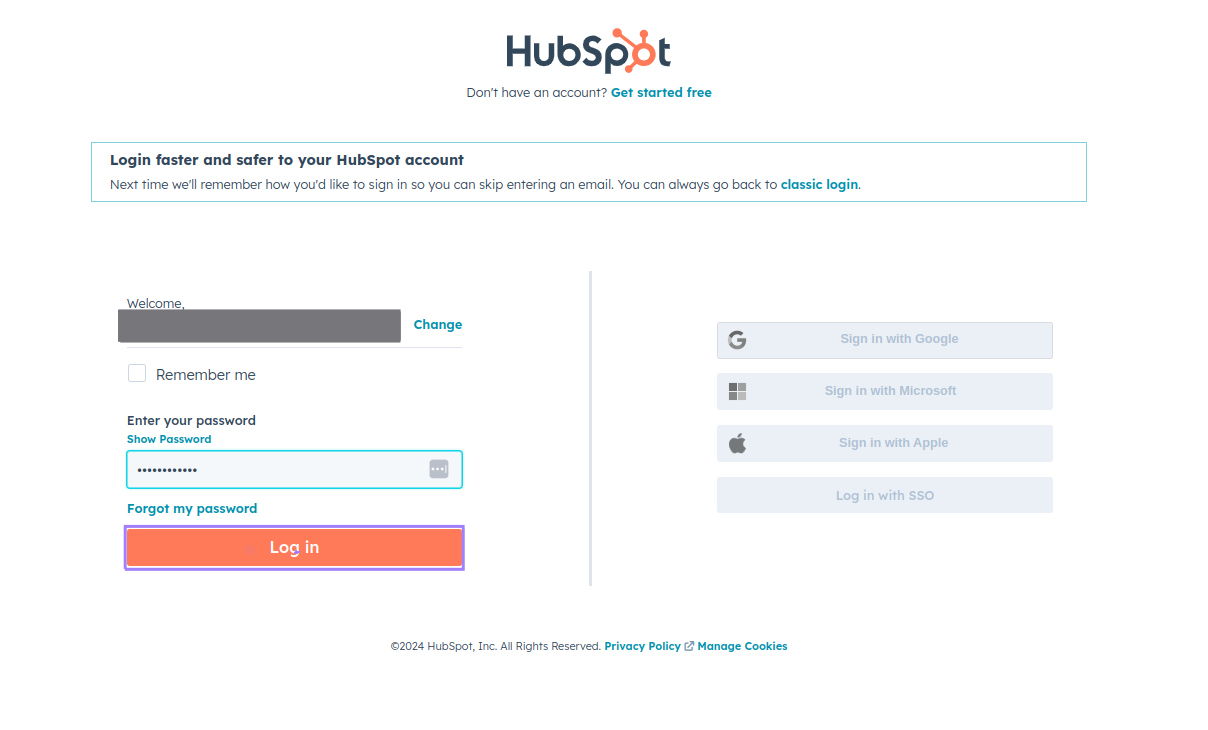
Step 7: In this end-to-end test case, we want to create a new deal in hubspot CRM . To reduce the dependency on any test case, we create a new deal for every end-to-end test case. Since this deal creation is a repetitive task, we can create a plain English ‘Reusable Rule‘ in testRigor for it, which is similar to a subroutine in programming. We will just insert the name of this reusable rule in every test case whenever we need to create a new deal.
Here are the test steps for creating the reusable rule ‘create deal’.
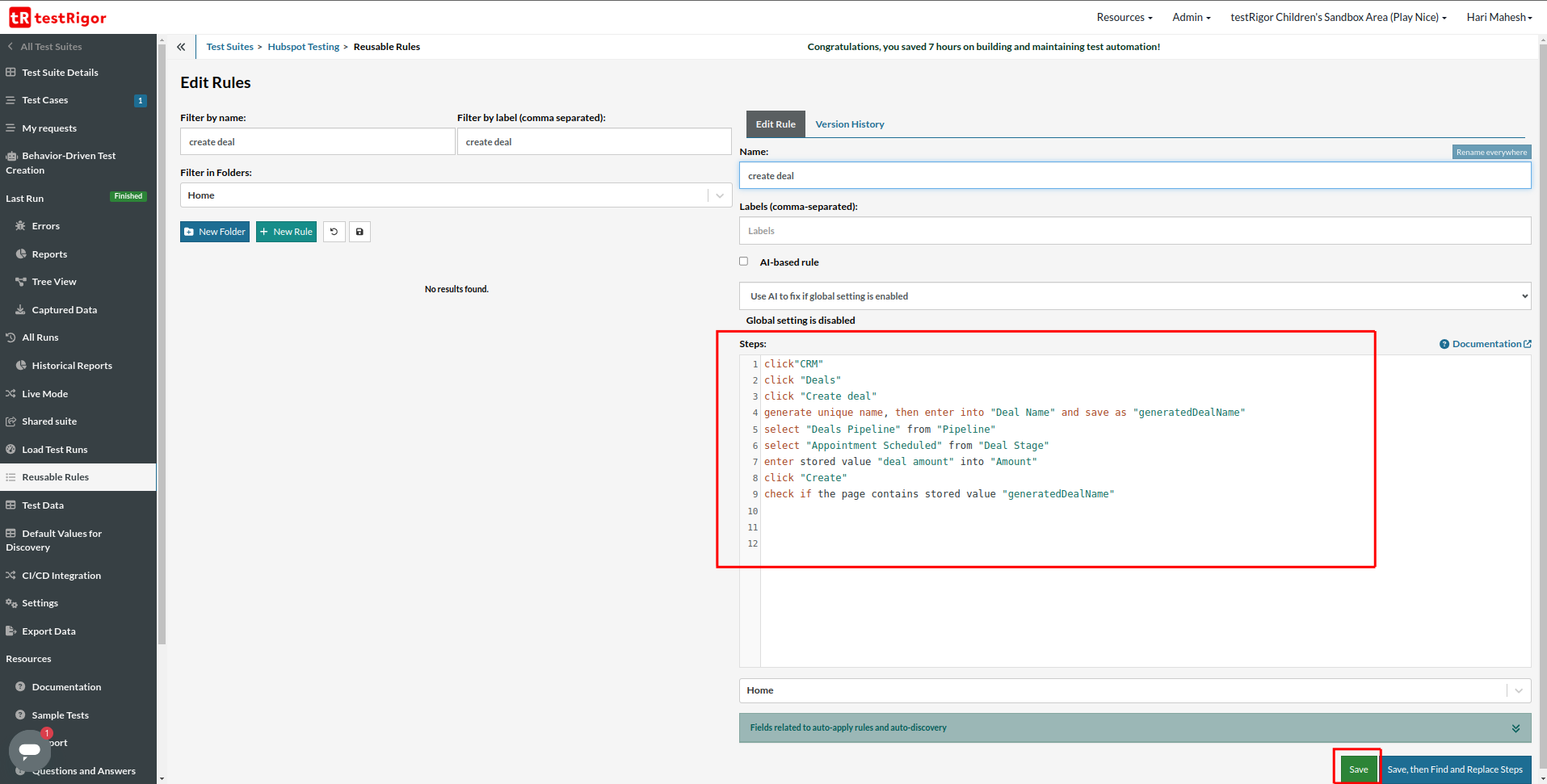
login create deal
In a nutshell the ‘create deal‘ test steps are working as:
Step 8.1: click “CRM”
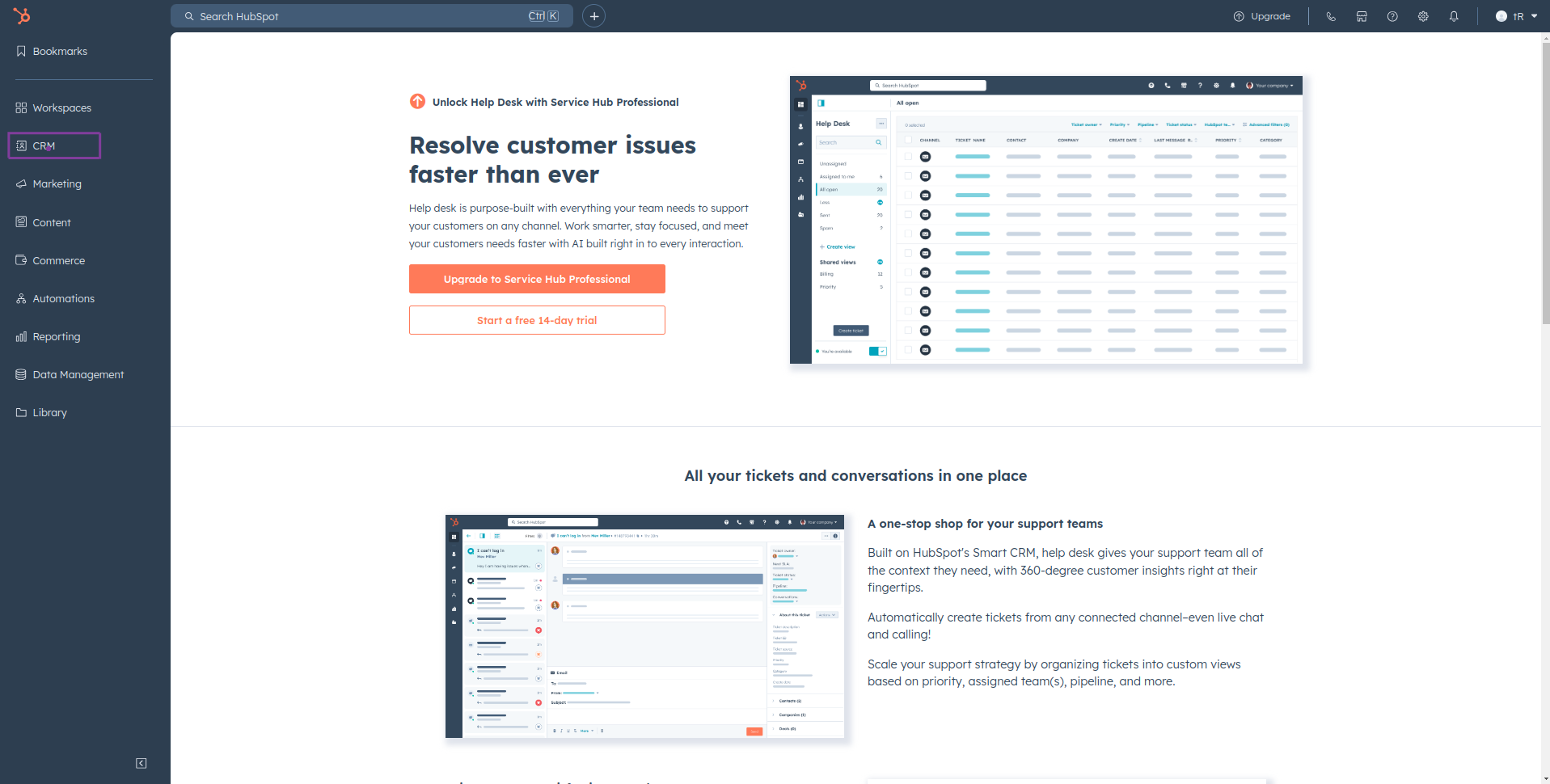
Step 8.2: click “deals”
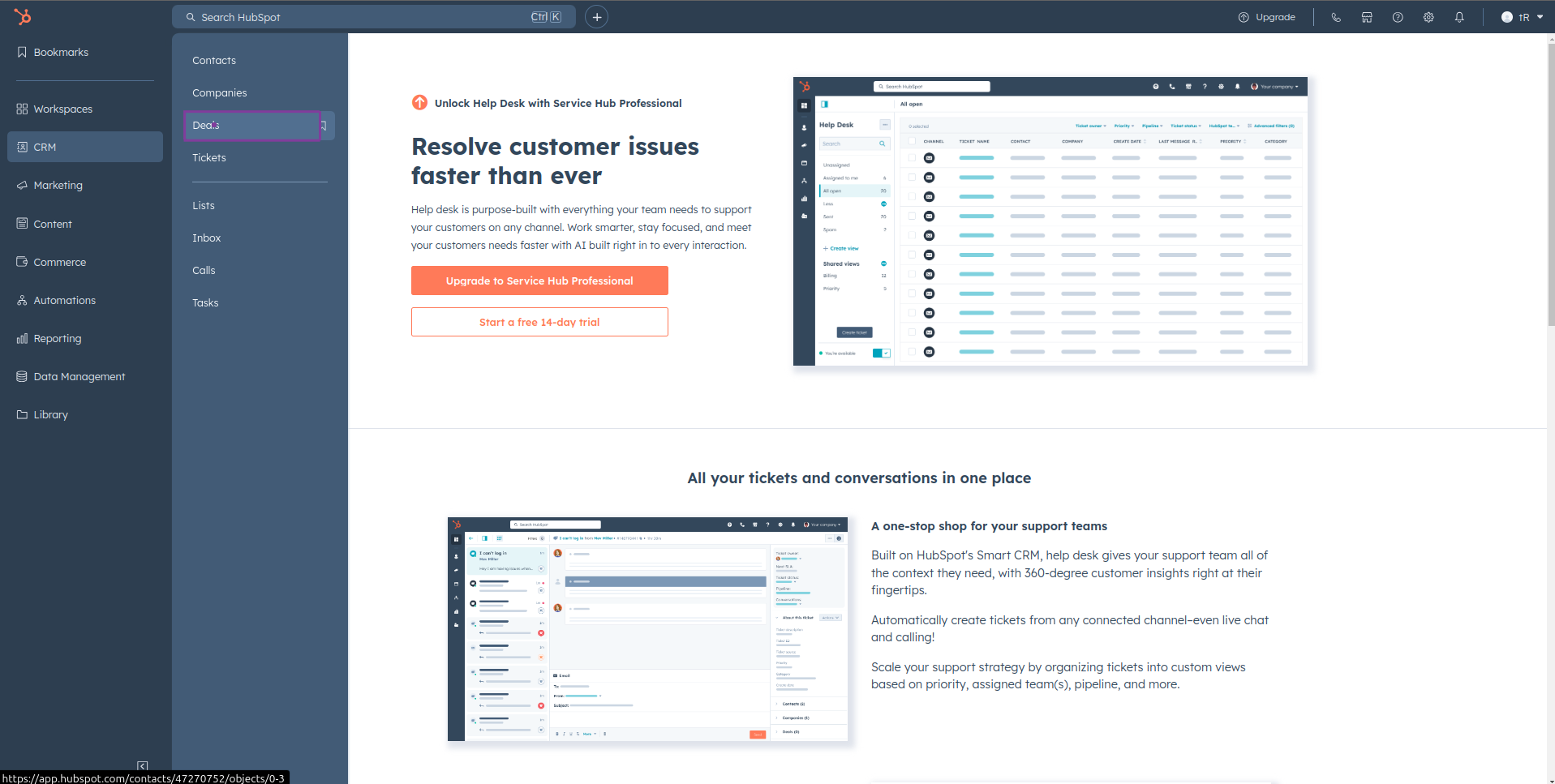
Step 8.3: click "Create deal"
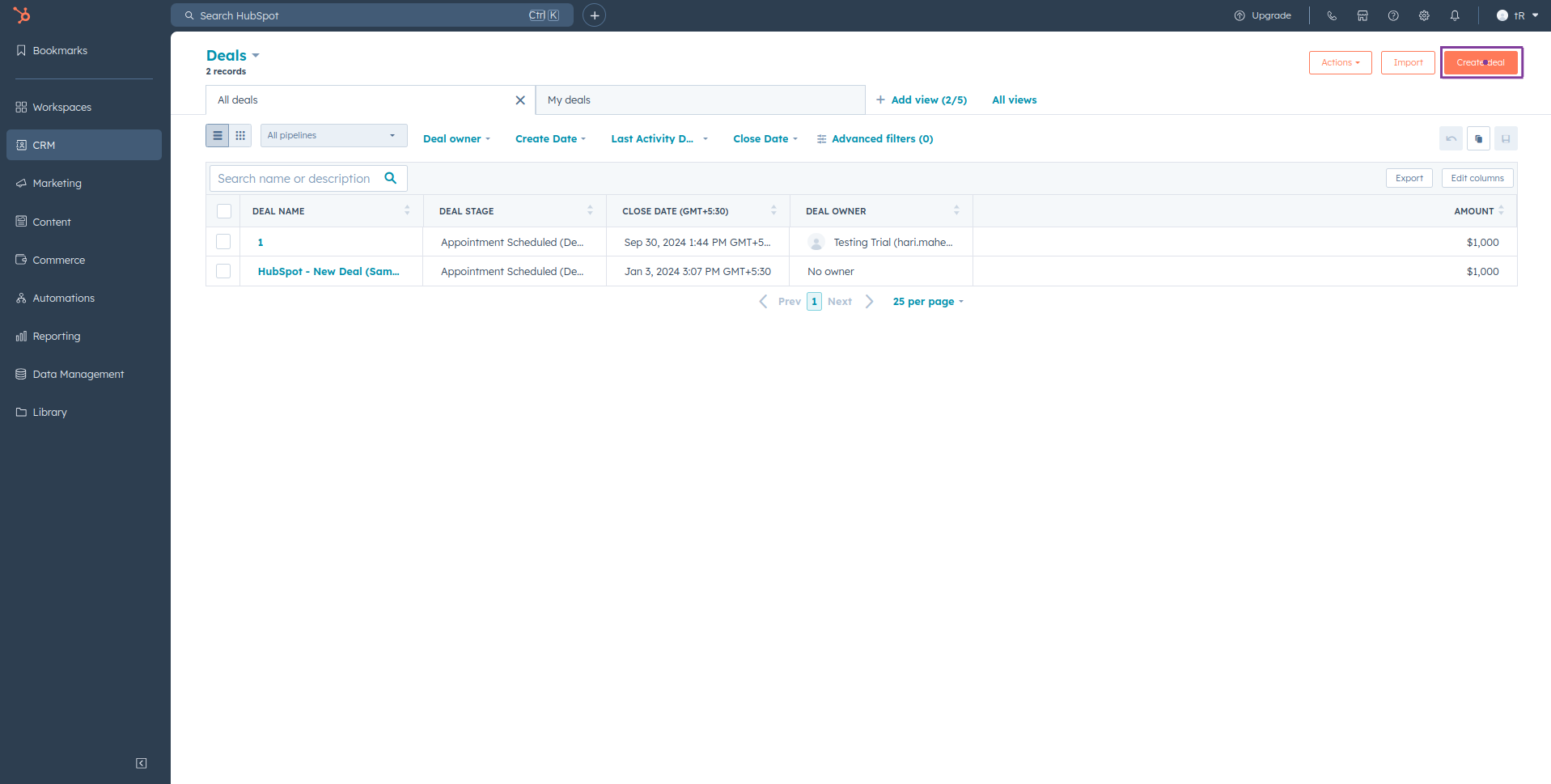
Step 8.4: click "generate unique name, then enter into "Deal Name" and save as "generatedDealName"
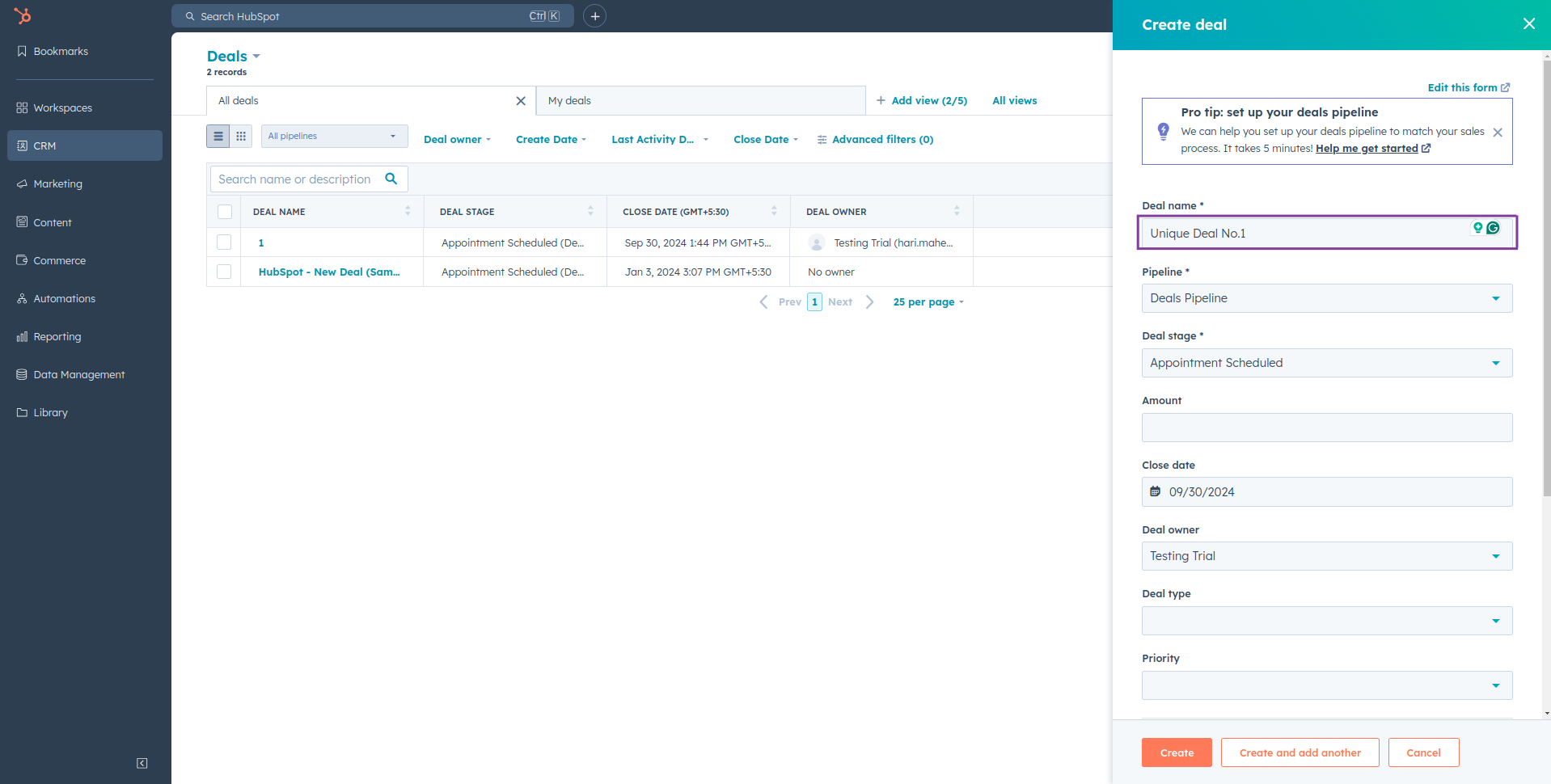
Step 8.5: select "Deals Pipeline" from "Pipeline"
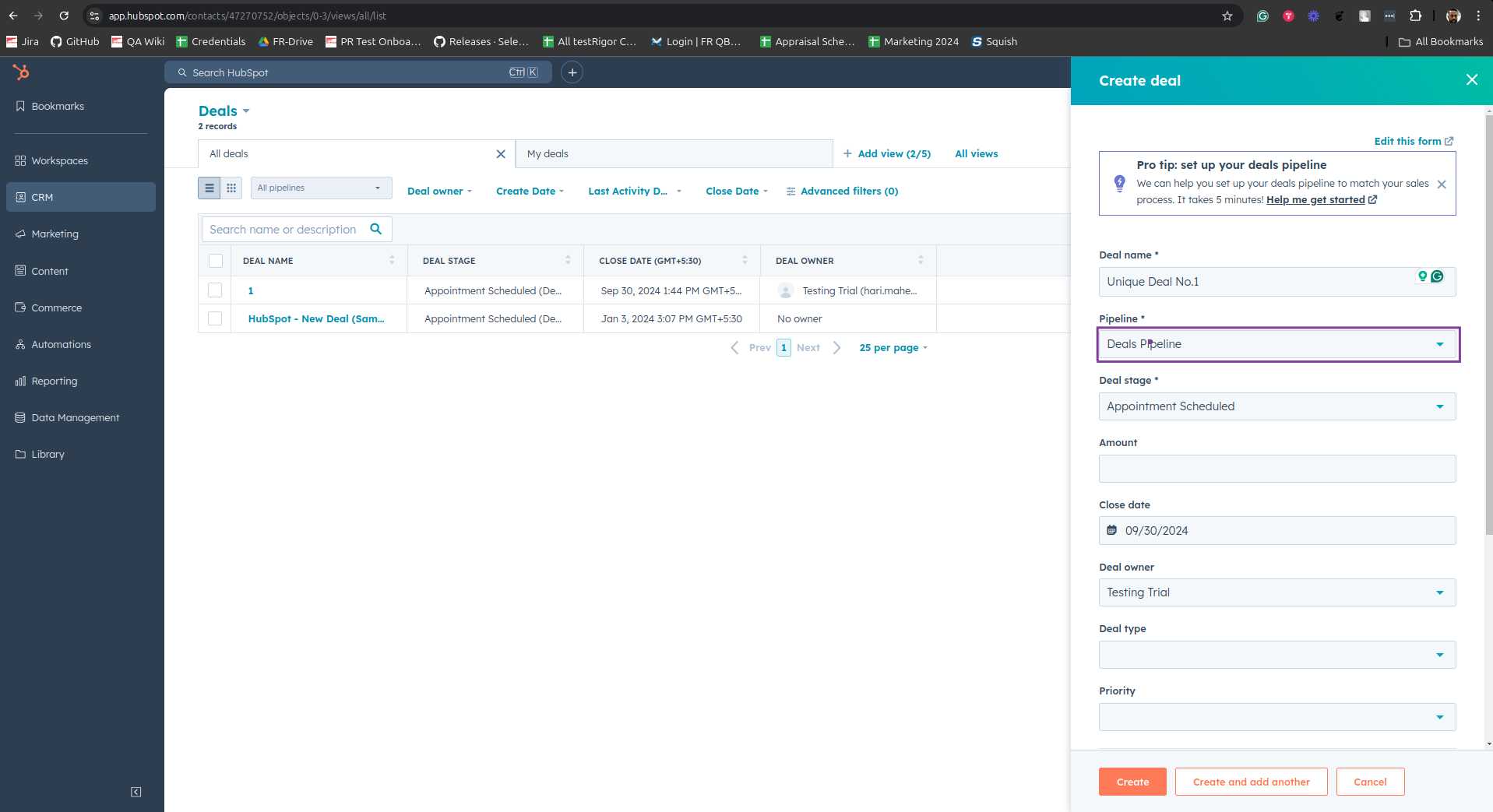
Step 8.6: select "Appointment Scheduled" from "Deal Stage"
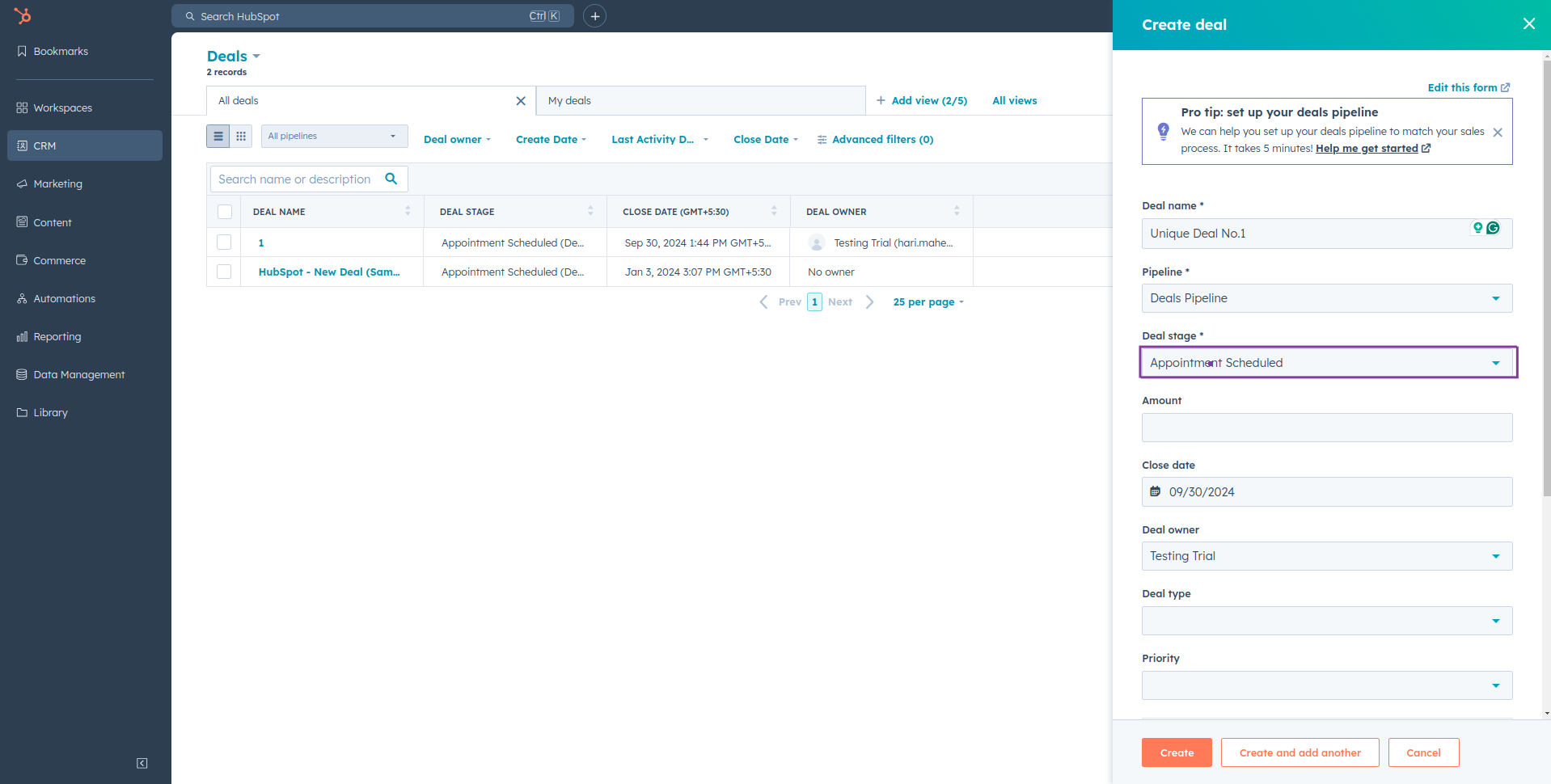
Step 8.7: enter stored value "deal amount" into "Amount"
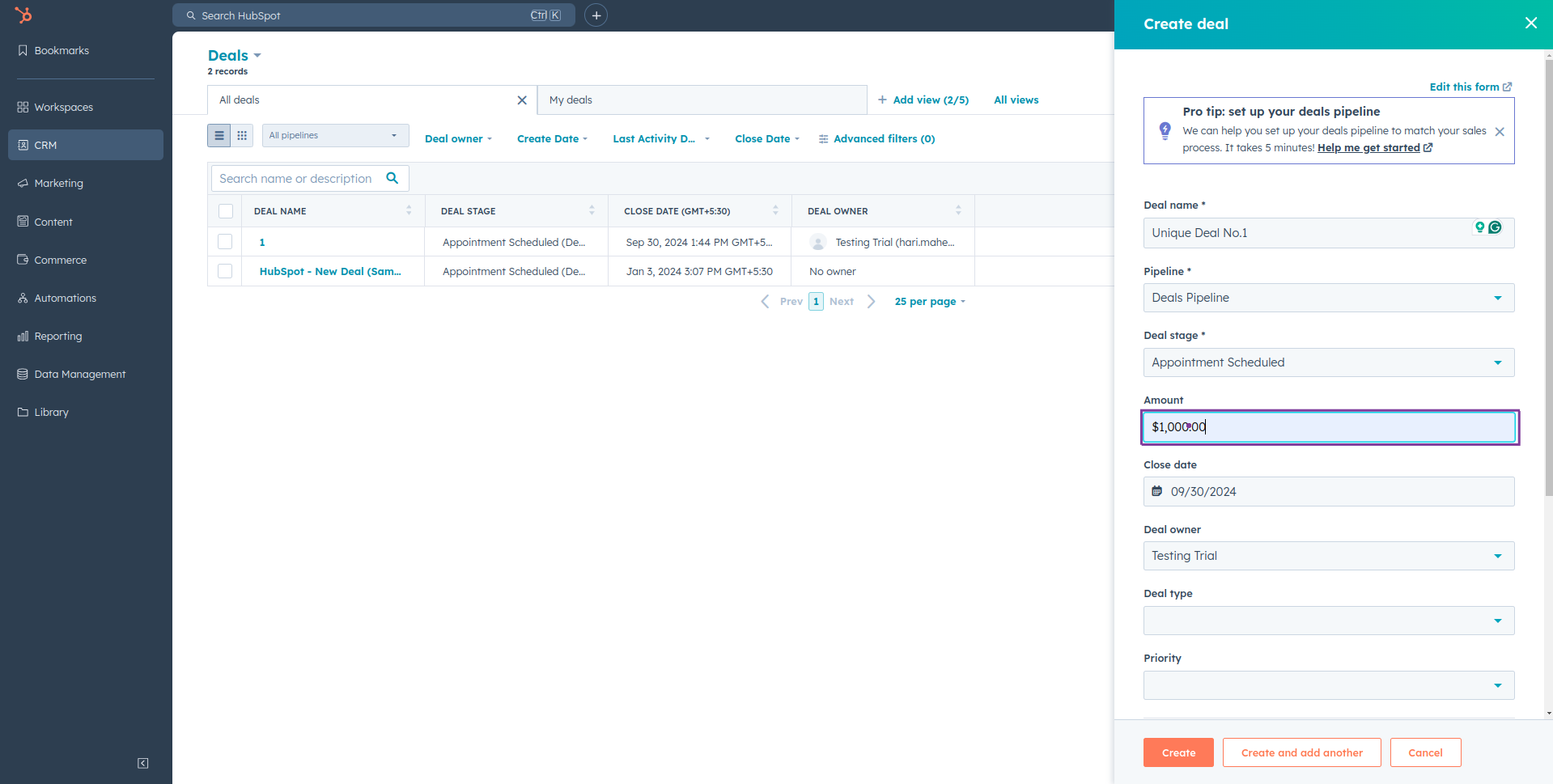
Step 8.8: click "Create"
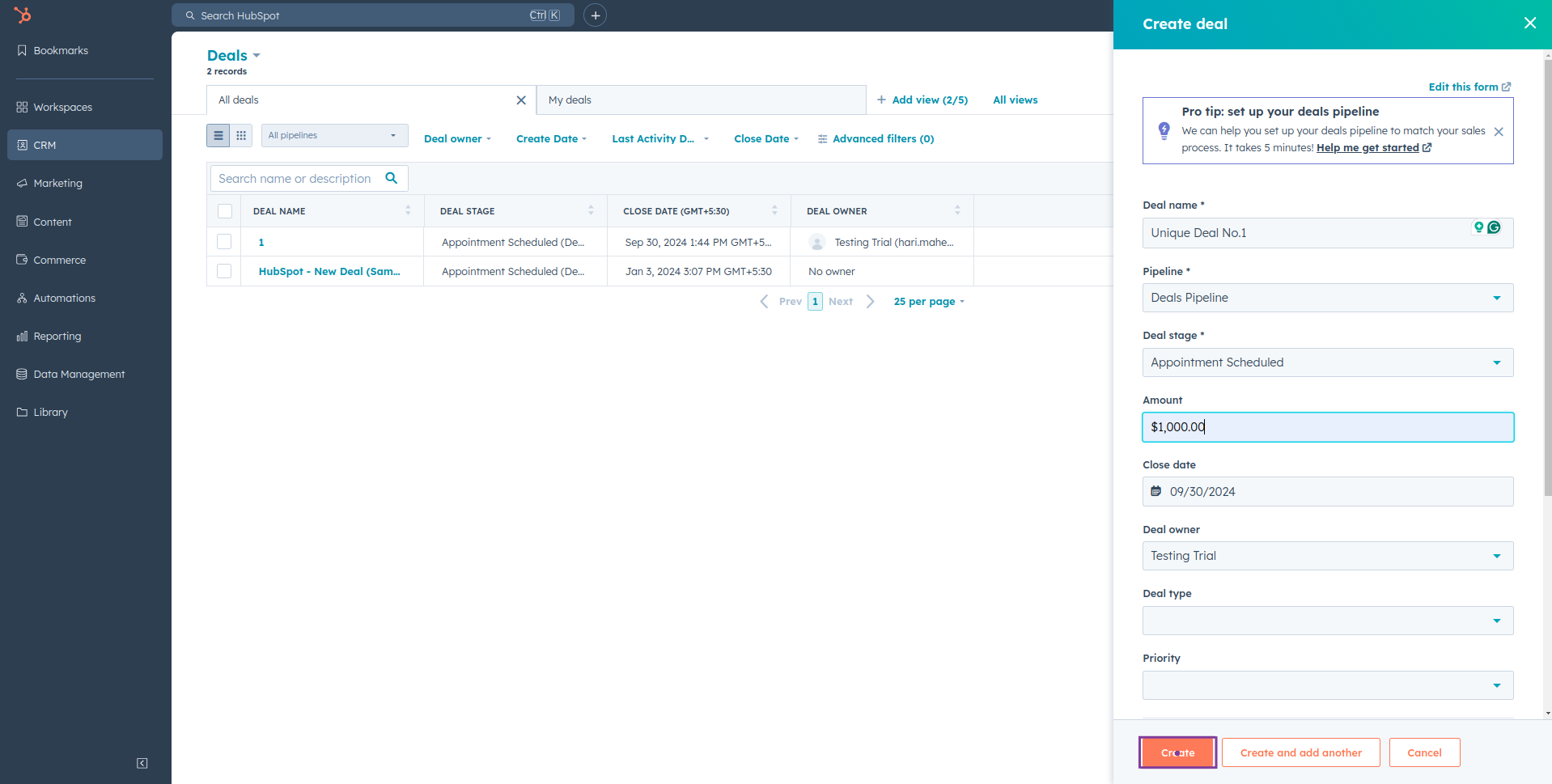
Step 8.9: check if the page contains stored value "generatedDealName"
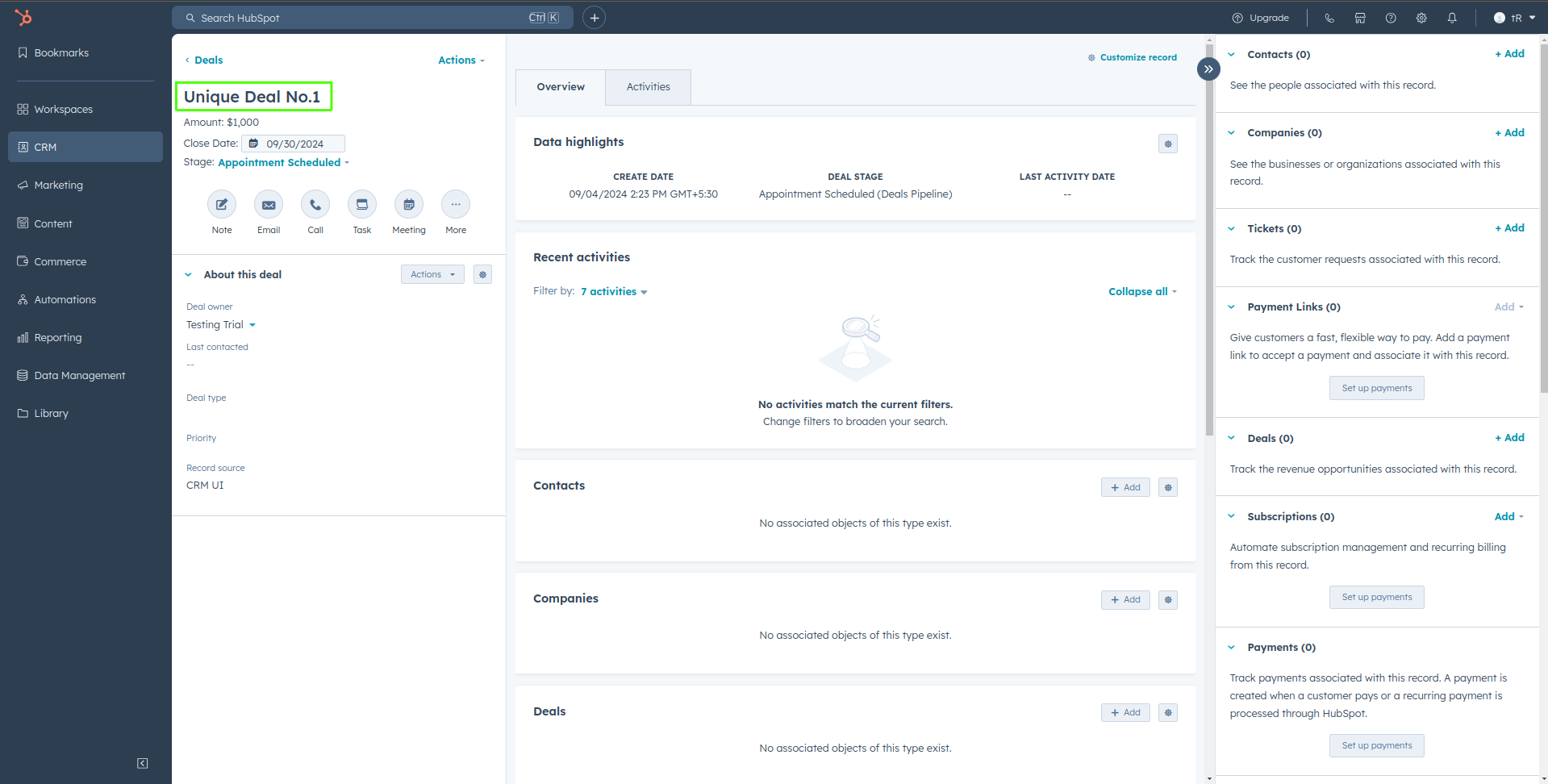
Step 9: Now, we have a new deal created using the reusable rule ‘create deal’. We will add the remaining test steps to update the company with this deal. The complete test case will look like this:
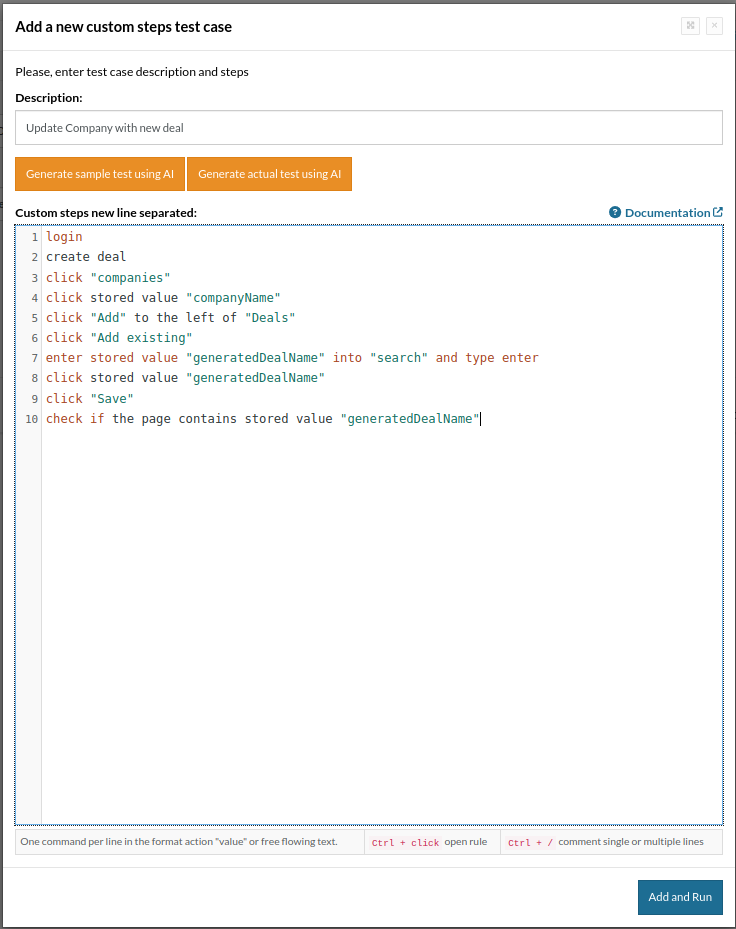
Click ‘Add and Run‘ to start the test execution.
Here is a step-by-step guide to help you perform end-to-end testing using testRigor.
HubSpot Marketplace Certification process
Once you’re confident about your app’s functionality through internal testing, you can submit it for certification through the HubSpot App Marketplace. Here’s what to expect:
- Provide Testing Credentials: HubSpot requires you to provide them with access to a test account or environment within your app. They’ll use this to verify your app’s functionality and adherence to their guidelines. You can use the email address [email protected] to invite HubSpot for testing purposes.
- Review Process: HubSpot will review your app for functionality, security and compliance with their App Marketplace guidelines. This may involve additional testing on their end.
- Feedback and Iteration: You might receive feedback from HubSpot on areas for improvement or potential issues identified during their review. Be prepared to address their concerns and resubmit your app for final approval.
Additional Resources
- HubSpot App Marketplace listing requirements: https://developers.hubspot.com/docs/api/app-marketplace-listing-requirements
- App Marketplace | Certification requirements: https://developers.hubspot.com/docs/api/certification-requirements
- Provide testing credentials for your app: https://developers.hubspot.com/docs/api/provide-testing-credentials-for-your-app
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
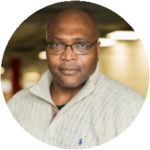