Troubleshooting InvalidElementStateException in Selenium
|
In Selenium, exceptions are part of its error-handling mechanism, and when an error occurs during test execution, exceptions are thrown. They indicate an unexpected condition that has stopped the test execution flow.
InvalidElementStateException is one of the common exceptions in Selenium. Let’s go ahead and understand more about this exception: the root cause and how to mitigate it.
What is InvalidElementStateException?
InvalidElementStateException occurs when the script performs an action on the web element, such as clicking or typing, but the element is not in the required state to accept the action. This scenario typically occurs when an element is not visible, enabled, or clickable to accept the action.
Here’s a more detailed explanation of InvalidElementStateException with an example:
Consider writing a test script to interact with a web application’s login page. The login page has two input fields: username and password and a “Login” button. The test script enters the correct credentials and then clicks the “Login” button to log in.
from selenium import webdriver # Create a WebDriver instance (e.g., ChromeDriver) driver = webdriver.Chrome() # Navigate to the login page driver.get("https://example.com/login") # Locate the username and password input fields username_field = driver.find_element_by_id("username") password_field = driver.find_element_by_id("password") # Enter valid credentials username_field.send_keys("your_username") password_field.send_keys("your_password") # Attempt to click the "Login" button login_button = driver.find_element_by_id("login_button") login_button.click()
This script navigates to the login page, locates the username and password input fields, and enters valid credentials. However, suppose the “Login” button is disabled or not clickable for some reason (e.g., due to an ongoing validation process or an error on the page). In that case, a click on the “Login” button raises an InvalidElementStateException.
from selenium.common.exceptions import InvalidElementStateException try: login_button = driver.find_element_by_id("login_button") login_button.click() except InvalidElementStateException as e: print(f"Failed to click the 'Login' button: {e}")
This example shows catching the InvalidElementStateException exception and prints an error message to indicate that clicking the “Login” button failed due to its invalid state.
Reasons for InvalidElementStateException
-
Element Not Enabled: One of the most common reasons for an InvalidElementStateException is when we try to interact with an element (e.g., clicking a button or inputting text) that is disabled (not enabled yet) on the web page. Web elements often have an “enabled” or “disabled” attribute determining whether they can receive user input. In the below example, the “Login” button is disabled, and attempting to click it would result in an InvalidElementStateException.
<button id="login_button" disabled>Login</button>
-
Element Not Visible: Another common reason is when an element is invisible or hidden. Elements with a CSS property like display: none or visibility: hidden cannot be interacted with directly.
<input type="text" id="hidden_input" style="display: none;">
Attempting to send keys to this example’s “hidden_input” field would result in an InvalidElementStateException. -
Element Not Clickable: Some elements may be technically enabled and visible but are not clickable due to overlapping elements or other factors that prevent user interaction. This can lead to an InvalidElementStateException when attempting to click such elements.
-
Element State Changes: Elements on a web page can change their state dynamically. For example, a button that initially triggers a form submission might change into a “loading” state after clicking, preventing further interaction until the loading is complete. Such state changes can result in an InvalidElementStateException if you attempt to interact with the element at the wrong time.
Resolving InvalidElementStateException
-
Using Explicit Waits: Use explicit waits to ensure that the element you want to interact with becomes clickable, visible, or reaches the desired state before proceeding. Explicit waits allow you to specify conditions that must be met before interacting with an element. The WebDriverWait class can be used for this purpose.Here is an example using explicit wait to wait for an element to be clickable:
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC # Wait for the "Login" button to become clickable login_button = WebDriverWait(driver, 10).until( EC.element_to_be_clickable((By.ID, "login_button")) ) login_button.click()
-
Check Element State Before Interaction: Before interacting with an element, you can check its state using conditions provided by Selenium’s Expected Conditions. For example, you can use element_to_be_enabled to ensure that the element is enabled or element_to_be_clickable to ensure that it’s enabled and clickable.Let us review an example:
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC # Check if the "Login" button is clickable login_button = driver.find_element(By.ID, "login_button") WebDriverWait(driver, 10).until(EC.element_to_be_clickable(login_button)) login_button.click()
-
Error Handling and Logging: We can capture the exceptions in our automation scripts using proper error handling and logging strategies. Using these logs, we can analyze the exception and the root cause.
-
Handle AJAX and JavaScript Delays: If the web application contains JavaScript or AJAX requests, then it takes time to execute the script. In that case, we may need to add additional waits. Also, use ExpectedConditions provided by Selenium or custom JavaScript waits if necessary.
Advantage of Using testRigor
As we know, Selenium was a popular automation tool during the starting periods of test automation. However, many underlying things could have been improved with the tool. One major drawback was the need for good programming skills to use it. So, the tester must be proficient in the required programming language to use Selenium. Not only that, as the test script creation progresses, the code repository also gets complex, making it an arduous task to debug in case of failure. Creating a framework with Selenium is another time-consuming job with many disadvantages.
Simple, better, and more powerful options are available for end-to-end testing. You can read more about the 11 reasons why not to use Selenium anymore. With all these drawbacks, there has been a surge in the need for one advanced automation tool that can leverage AI’s power to simplify automation. The answer is testRigor. Its incredible generative AI-powered features are changing the testing game for many organizations today.
Here are a few of its features to bypass InvalidElementStateException:
- Independent from Programming Languages: testRigor helps to create test scripts in plain English, making it easy to use for everyone in the team: testers, project managers, business analysts, and other stakeholders.
- Auto Wait: testRigor provides auto wait for the page to load. So, there is no need to add a custom wait in the script and delay the execution time. Once the page is loaded fully, then only testRigor starts executing the scripts.
- Generative AI: Using generative AI, testRigor helps generate tests by providing the test description, thereby saving testers time and effort to create test scripts manually.
- Custom Element locators: testRigor doesn’t depend on flaky XPaths; it has its way of identifying elements called testRigor locators, which is exactly like how humans identify an element on the screen. Use the UI text or the relative position of an element; for example, click “cart”
click on button “Delete” below “Section Name”
EndNotes
When we consider today’s world, the demand put forward by consumers is high. So, to meet that, the companies need to release more frequently. To meet the required quality of these releases, it’s mandatory that the testing also should be completed in significantly less time.
The automation tool should be intelligent and modern to support this whole process seamlessly. Tools like Selenium couldn’t sustain and meet these demanding requirements of today, because of its own complexity, errors, and exceptions. That’s where testRigor makes it very easy for everyone to write and execute tests and achieve great coverage quickly.
Sign up for a free trial to discover its powerful features.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
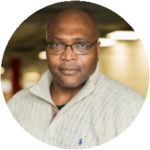