Navigating UnexpectedAlertPresentException in Selenium
|
When you perform WebDriver operations, if an alert, confirmation, or prompt box appears unexpectedly, the script is not prepared to handle that. Then, the script moves on to the next execution step, and UnexpectedAlertPresentException is thrown in Selenium. You need to handle these pop-up alerts as they can block further execution.
Read this article to learn more about alerts and handling NoAlertPresentException in Selenium.
Why UnexpectedAlertPresentException Happens?
Below are a few reasons why you might encounter UnexpectedAlertPresentException in Selenium:
Unhandled Alerts in Script
This is the most common reason for the exception. When an alert/confirmation/prompt box appears on the screen, and the WebDriver does not handle it, i.e., accept, dismiss, or read its content. The test script then tries to execute the following command, and UnexpectedAlertPresentException is thrown.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); driver.findElement(By.id("triggerAlertButton")).click(); // This triggers an alert // Attempt to click another button without handling the alert driver.findElement(By.id("otherButton")).click(); // This will throw UnexpectedAlertPresentException
Asynchronous Operations Trigerring Alerts
Asynchronous operations such as AJAX calls may trigger JavaScript alerts unexpectedly. If the delay is not managed in the script for such alerts, then the exception is thrown by Selenium.
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); driver.findElement(By.id("triggerAjaxCall")).click(); // This triggers an AJAX call that will eventually show an alert // Assuming the AJAX call will complete in 5 seconds Thread.sleep(5000); // Poor way to handle timing, just for demonstration // Trying to interact with the page without checking for alert driver.findElement(By.id("afterAjax")).click(); // Might throw UnexpectedAlertPresentException if the alert is present
Browser-specific Alerts/Behavior
Different browsers may handle alerts differently. A few scripts may work fine in one browser but may encounter unexpected alerts in another. These alerts might be unhandled by the script and cause UnexpectedAlertPresentException.
Example: A test script executes smoothly in Chrome but faces unexpected alerts in Firefox, and then UnexpectedAlertPresentException occurs.
Alert Timing Issues
Alerts that appear on page load or due to some background operations might not be appropriately synchronized with the WebDriver’s commands. This may lead to unexpected alerts and then UnexpectedAlertPresentException eventually.
Example: An alert appears during a page load, but the script starts interacting with the page elements before handling the alert.
How to Fix UnexpectedAlertPresentException?
Here are a few resolutions that might help you to fix UnexpectedAlertPresentException in Selenium.
Handle Alerts Explicitly
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); driver.findElement(By.id("triggerAlertButton")).click(); // This triggers an alert try { Alert alert = driver.switchTo().alert(); alert.accept(); // or alert.dismiss(); to cancel the alert } catch (NoAlertPresentException e) { System.out.println("No alert was present"); } // Continue with other actions
Wait for Alerts in Asynchronous Operations
WebDriver driver = new ChromeDriver(); WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); driver.get("http://example.com"); driver.findElement(By.id("triggerAjaxCall")).click(); // This triggers an AJAX call that may eventually show an alert try { wait.until(ExpectedConditions.alertIsPresent()); Alert alert = driver.switchTo().alert(); alert.accept(); // Handle the alert } catch (TimeoutException e) { System.out.println("Alert did not appear within the specified time"); } // Continue with other actions
Handle Alerts After Page Load
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); try { Alert alert = driver.switchTo().alert(); alert.accept(); // Handling alert on page load } catch (NoAlertPresentException e) { System.out.println("No alert on page load"); } // Now safe to proceed with other actions
Use Try-Catch Blocks
WebDriver driver = new ChromeDriver(); driver.get("http://example.com"); try { driver.findElement(By.id("someElement")).click(); // More actions... } catch (UnexpectedAlertPresentException e) { Alert alert = driver.switchTo().alert(); System.out.println("Unexpected alert handled: " + alert.getText()); alert.accept(); // Optionally, retry the action or perform alternative steps }
Cross-browser Testing
Test your scripts across different browsers to handle browser-specific alert behaviors and rectify any errors and exceptions accordingly for smooth execution. Read more about cross-browser testing.
Bypass UnexpectedAlertPresentException with testRigor
You may have understood the reasons and resolutions to UnexpectedAlertPresentException in Selenium. However, it is easier said than done, and if you want to bypass all these exceptions and just focus on software testing, then we have a powerful solution for you.
login click "Men Clothing" scroll down click "Men's cargo shorts" click "brown" click "Size 34" click "Add to cart" check that page contains "Your order is nearly complete!" accept prompt with value "Order Successful!"
This is the simplicity of test cases in testRigor, where you can use the UI text that you see on the screen as identifiers for elements. No more unstable CSS/XPath locators to put you in trouble with maintenance and frequent script updates. With testRigor, you need not add explicit waits to wait for the page elements to load completely; testrigor is intelligent enough to handle these scenarios automatically.
Perform web, mobile, desktop, API, cross-browser, and cross-platform testing singlehandedly with testRigor in plain English. It has supportive integrations with all significant test management, defect management, infrastructure provider, ERP, and CRM tools. It’s time to bid goodbye to the irritating Selenium exceptions forever!
Conclusion
Selenium has been everyone’s test automation partner for a long. But the current Agile and DevOps faster release cycles demand more. It is not worth spending hours handling Selenium errors and exceptions when that effort and time could bring out something more productive and valuable.
If there are intelligent alternatives available, why not use them for the benefit of every stakeholder involved? Put minimum effort into script maintenance with testRigor, which has in-built self-healing capabilities. Build robust tests faster with ease and attain more test coverage to release a high-quality product within budget.
Achieve More Than 90% Test Automation | |
Step by Step Walkthroughs and Help | |
14 Day Free Trial, Cancel Anytime |
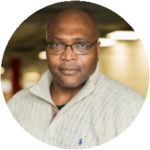